Instructions
Objective
Write a java homework program to create course planning.
Requirements and Specifications
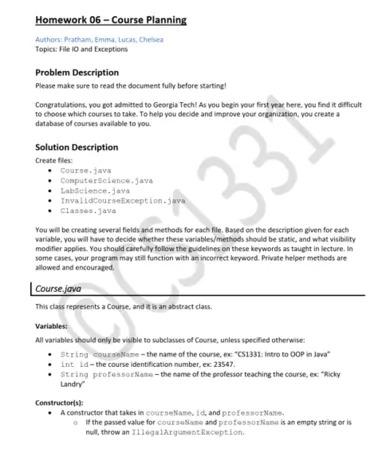
Source Code
CLASSES
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.PrintWriter;
import java.util.ArrayList;
import java.util.Scanner;
/**
* This class represents the Driver program.
* @author: NAME
* @version: 1.0
*/
public class Classes {
/**
* Method that reads a file with courses data.
* Returns an array list of curses
* @param fileName String
* @return ArrayList of Course objects
* @throws FileNotFoundException Exception
* @throws InvalidCourseException Exception
*/
public static ArrayList outputCourses(String fileName) throws FileNotFoundException,
InvalidCourseException {
if (fileName == null || fileName.isEmpty()) {
throw new FileNotFoundException();
}
File file = new File(fileName);
if (!file.exists()) {
throw new FileNotFoundException();
}
// Create list
ArrayList courses = new ArrayList();
// Read file
Scanner reader = new Scanner(file);
String line;
Course course = null;
while (reader.hasNextLine()) {
line = reader.nextLine();
// Split
String[] row = line.split(",");
String courseType = row[0];
String courseName = row[1];
int id = Integer.valueOf(row[2]);
String professorName = row[3];
if (courseType.equals("ComputerScience")) {
String language = row[4];
course = new ComputerScience(courseName, id, professorName, language);
} else if (courseType.equals("LabScience")) {
boolean labCoatRequired = Boolean.valueOf(row[4]);
course = new LabScience(courseName, id, professorName, labCoatRequired);
} else {
throw new InvalidCourseException();
}
if (course != null) {
courses.add(course);
course = null;
}
}
reader.close();
return courses;
}
/**
* Method that writes a given array list of courses to a file.
* @param fileName File name
* @param courses ArrayList of courses
* @return boolean
*/
public static boolean writeCourses(String fileName, ArrayList courses) {
boolean success = false;
try {
// Open or create file
PrintWriter writer = new PrintWriter(new FileOutputStream(new File(fileName), true));
// Loop through courses
for (Course course: courses) {
; writer.println(course.toString());
}
writer.close();
success = true;
} catch (Exception ex) {
System.out.println(ex.getMessage());
} finally {
return success;
}
}
/**
* Method that read the courses from a file and checks if a given course exists.
* If the course exists in the file, this method returns an array list with
* the number of the lines where the course is located at
* @param fileName String
* @param course Course
* @return ArrayList of lines id
* @throws FileNotFoundException Exception
* @throws InvalidCourseException Exception
*/
public static ArrayList readCourses(String fileName, Course course)
throws FileNotFoundException, InvalidCourseException {
if (fileName == null || fileName.isEmpty()) {
throw new FileNotFoundException();
}
File file = new File(fileName);
if (!file.exists()) {
throw new FileNotFoundException();
}
int lineid = 1;
ArrayList lines = new ArrayList();
// Read file
Scanner reader = new Scanner(file);
String line;
Course courseRead = null;
while (reader.hasNextLine()) {
line = reader.nextLine();
// Split
String[] row = line.split(",");
String courseType = row[0];
String courseName = row[1];
int id = Integer.valueOf(row[2]);
String professorName = row[3];
if (courseType.equals("ComputerScience")) {
String language = row[4];
courseRead = new ComputerScience(courseName, id, professorName, language);
} else if (courseType.equals("LabScience")) {
boolean labCoatRequired = Boolean.valueOf(row[4]);
courseRead = new LabScience(courseName, id, professorName, labCoatRequired);
}
if (courseRead != null && courseRead.equals(course)) {
lines.add(lineid);
}
lineid += 1;
}
reader.close();
// if the ArrayList is empty, it means the course was not found
if (lines.size() == 0) {
throw new InvalidCourseException();
} else {
return lines;
}
}
/**
* Main method. Driver of the program.
* @param args String array with arguments
*/
public static void main(String[] args) {
// Create three computer science objects
Course cs1 = new ComputerScience("CS1", 15002, "Mr. Johnson", "C++");
Course cs2 = new ComputerScience("CS2", 25002, "Ms. Park", "Java");
Course cs3 = new ComputerScience("CS3", 35002, "Mr. Diktovich", "Python");
// LabScience courses
Course ls1 = new LabScience("LS001", 11001, "Mr. Karl", true);
Course ls2 = new LabScience("LS002", 11002, "Mr. Sagan", false);
Course ls3 = new LabScience("LS003", 11003, "Mr. Holland", true);
// add to an arraylist
ArrayList courses = new ArrayList();
courses.add(cs1);
courses.add(cs2);
courses.add(cs3);
courses.add(ls1);
courses.add(ls2);
courses.add(ls3);
writeCourses("TestCourses.csv", courses);
try {
ArrayList readCourses = outputCourses("TestCourses.csv");
for (Course c: readCourses) {
System.out.println(c.toString());
}
} catch (Exception ex) {
System.out.println(ex.getMessage());
}
}
}
COMPUTER SCIENCE
/**
* Class that represents a ComputerScience course object.
* @author: NAME
* @version: 1.0
*/
public class ComputerScience extends Course {
/**
* String that represents the language of the course.
*/
private String language;
/**
* Parametrized constructor.
* @param courseName String
* @param id integer
* @param professorName String
* @param language String
*/
public ComputerScience(String courseName, int id, String professorName, String language) {
super(courseName, id, professorName);
if (language == null || language.isEmpty()) {
throw new IllegalArgumentException();
}
this.language = language;
}
/**
* Returns a String representation of the object.
*/
@Override
public String toString() {
return String.format("ComputerScience,%s,%s", super.toString(), getLanguage());
}
/**
* Checks if two ComputerScience objects are equal.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof ComputerScience)) {
return false;
}
ComputerScience other = (ComputerScience) o;
return super.equals(other) && getLanguage().equals(other.getLanguage());
}
/**
* Getter method for the language.
* @return String
*/
public String getLanguage() {
return this.language;
}
}
COURSE
/**
* This class represents a Course object.
* @author: NAME
* @version: 1.0
*/
public abstract class Course {
/**
* String that represents the course name.
*/
protected String courseName;
/**
* Integer that represents the course id.
*/
protected int id;
/**
* String that represents the professor name.
*/
protected String professorName;
/**
* OVerloaded constructor.
* Given course name, id and professor name
* @param courseName String
* @param id integer
* @param professorName String
*/
public Course(String courseName, int id, String professorName) {
if (courseName == null || courseName.isEmpty() || professorName == null || professorName.isEmpty()) {
throw new IllegalArgumentException();
}
if (id < 0 || id < 10000) {
throw new IllegalArgumentException();
}
this.courseName = courseName;
this.professorName = professorName;
this.id = id;
}
/**
* Returns a string representation of the object.
*/
@Override
public String toString() {
return String.format("%s,%d,%s", getCourseName(), getId(), getProfessorName());
}
/**
* Method that checks if two course objects are equal.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof Course)) {
return false;
}
Course other = (Course) o;
return getCourseName().equals(other.getCourseName())
&& getProfessorName().equals(other.getProfessorName())
&& getId() == other.getId();
}
/**
* Getter method for course name.
* @return String
*/
public String getCourseName() {
return this.courseName;
}
/**
* Getter method for professor name.
* @return String
*/
public String getProfessorName() {
return this.professorName;
}
/**
* Getter method for course id.
* @return Integer
*/
public int getId() {
return this.id;
}
}
INVALID COURSE EXCEPTION
/**
* Class that represents an InvalidCourseException
* This is a custom exception
* @author: NAME
* @version: 1.0
*/
public class InvalidCourseException extends RuntimeException {
/**
* Parametrized constructor.
* @param message String
*/
public InvalidCourseException(String message) {
super(message);
}
/**
* Default constructor.
*/
public InvalidCourseException() {
super("Invalid course type!");
}
}
LAB SCIENCE
/**
* Class that represents a LabSience course.
* @author: NAME
* @version: 1.0
*/
public class LabScience extends Course {
/**
* Parameter that defines is the lab requires coat.
*/
private boolean labCoatRequired;
/**
* Parametrized constructor.
* @param courseName String
* @param id Integer
* @param professorName String
* @param labCoatRequired Boolean
*/
public LabScience(String courseName, int id, String professorName, boolean labCoatRequired) {
super(courseName, id, professorName);
this.labCoatRequired = labCoatRequired;
}
/**
* Returns a string representation of the object.
*/
@Override
public String toString() {
return String.format("LabScience,%s,%s", super.toString(), getLabCoatRequired());
}
/**
* Checks if two LabScience course are equal.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof LabScience)) {
return false;
}
LabScience other = (LabScience) o;
return super.equals(other) && getLabCoatRequired() == other.getLabCoatRequired();
}
/**
* Getter method for the boolean parameter.
* @return Boolean
*/
public boolean getLabCoatRequired() {
return this.labCoatRequired;
}
}
Related Samples
Explore our free programming assignment samples to see examples of our expertly solved problems across various programming languages and topics. These samples showcase our commitment to quality and thorough understanding of student needs in programming education.
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming