Instructions
Objective
Write a program to create contact service application in java language.
Requirements and Specifications
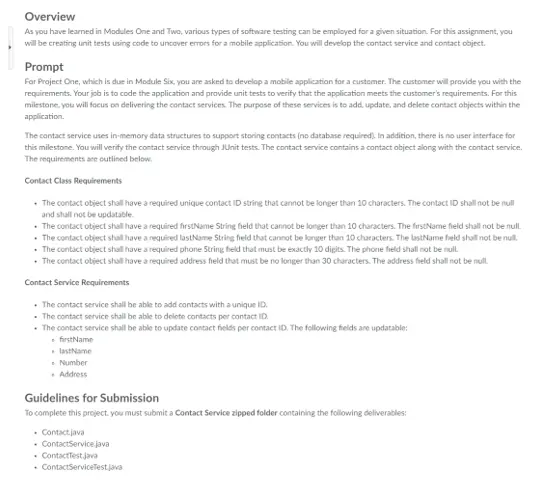
Source Code
CONTACT
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package com.mycompany.contacttests;
public class Contact {
private String contactId;
private String firstName;
private String lastName;
private String address;
private String phoneNumber;
public Contact(String contactId, String firstName, String lastName, String address, String phoneNumber) {
//validate inputs against requirements
boolean isValid = validateInput(contactId, 10);
if(isValid) {
this.contactId = contactId;
}
isValid = isValid && setContactFirstName(firstName);
isValid = isValid && setContactLasttName(lastName);
isValid = isValid && setAddress(address);
isValid = isValid && setPhoneNumber(phoneNumber);
if(!isValid) {
throw new IllegalArgumentException("Invalid input");
}
}
public boolean setContactFirstName(String firstName) {
boolean isValid = validateInput(firstName, 10);
if(isValid) {
this.firstName = firstName;
}
return isValid;
}
public boolean setContactLasttName(String lastName) {
boolean isValid = validateInput(lastName, 10);
if(isValid) {
this.lastName = lastName;
}
return isValid;
}
public boolean setAddress(String address) {
boolean isValid = validateInput(address, 20);
if(isValid) {
this.address = address;
}
return isValid;
}
public boolean setPhoneNumber(String phoneNumber) {
boolean IsValid = phoneNumber.matches("\\d{10}");
if(IsValid) {
this.phoneNumber = phoneNumber;
}
return IsValid;
}
public String getContactId() {
return contactId;
}
public String getFirstName() {
return firstName;
}
public String getLastName() {
return lastName;
}
public String getAddress() {
return address;
}
public String getPhoneNumber() {
return phoneNumber;
}
private boolean validateInput(String item, int length) {
return (item != null && item.length() <= length && item.length() > 0);
}
}
CONTACT SERVICE
package com.mycompany.contacttests;
/*
* To change this license header, choose License Headers in Project Properties.
;* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
import java.util.HashMap;
import java.util.Map;
public class ContactService {
private static ContactService reference = new ContactService();
private final Map contacts;
ContactService() {
this.contacts = new HashMap();
}
//Create a singleton Contact Service
public static ContactService getService() {
return reference;
}
public boolean addContact(Contact contact) {
boolean isSuccess = false;
if(!contacts.containsKey(contact.getContactId())) {
contacts.put(contact.getContactId(), contact);
isSuccess = true;
}
return isSuccess;
}
public boolean deleteContact(String contactId) {
return contacts.remove(contactId) != null;
}
public Contact getContact(String contactId) {
return contacts.get(contactId);
}
}
Similar Samples
Explore a variety of programming assignment samples at ProgrammingHomeworkHelp.com. Our samples cover Java, Python, C++, SQL, and more, showcasing our expertise in delivering precise solutions. Whether you need help with algorithms, databases, or web development, our examples demonstrate our commitment to excellence in programming assistance.
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java