Instructions
Requirements and Specifications
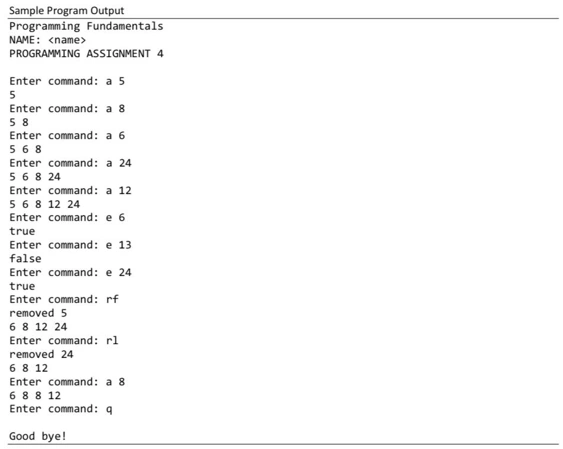
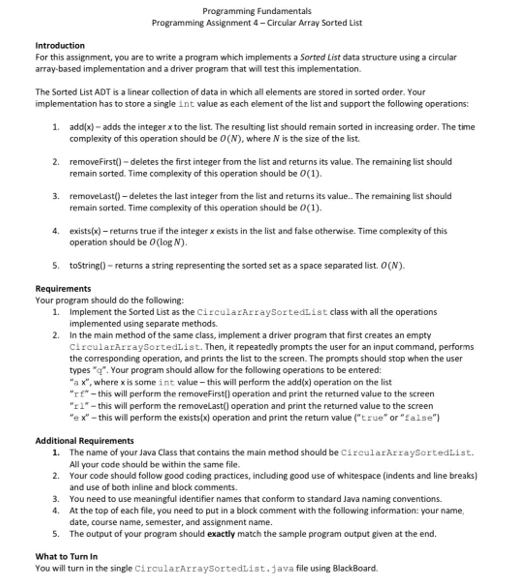
Source Code
import java.util.ArrayList;
import java.util.List;
public class CircularArraySortedList
{
List array;
public CircularArraySortedList()
{
array = new ArrayList ();
}
// getters
public int size() {return array.size();}
public void add(int x)
{
/*
* Adds an element in the array such that the elements are sorted
*
*/
if(size() == 0)
{
array.add(x);
}
else
{
// if the element x is higher than the last element in the list, then just add it
if(x >= array.get(size()-1)) {
array.add(x);
}
else
{
for(int i = 0; i < size(); i++)
{
if(x < array.get(i))
{
array.add(i, x);
break;
}
}
}
}
}
public int removeFirst() {
/*
* Removes the first element in the array and returns it
*/
return array.remove(0);
}
public int removeLast() {
return array.remove(size()-1);
}
public boolean exist(int x)
{
/*
* Given an integer x, check if it exists in the array
*/
return array.indexOf(x) >= 0;
}
@Override
public String toString()
{
/*
* Return an string containing the elements in the list
*/
String result = "";
for(Integer x: array) {
result += x + " ";
}
return result;
}
}
Related Samples
Explore our Java Assignments sample section designed to elevate your programming proficiency. Dive into topics like object-oriented programming, data structures, algorithms, and more. Each assignment offers comprehensive explanations and solutions to enhance your Java skills. Master Java programming with our expertly curated assignments and excel in your coursework effortlessly.
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java