Tip of the day
News
Instructions
Objective
To complete a C++ assignment, you'll need to write a program that functions as a basketball statistics analyzer. This program should be designed to efficiently gather and analyze basketball game data, providing insights into player performance, team statistics, and other relevant metrics. By utilizing C++ language features, you can develop a robust and user-friendly application that handles data input, processing, and output effectively. This assignment will not only test your coding skills but also your ability to design a practical solution for real-world data analysis needs.
Requirements and Specifications
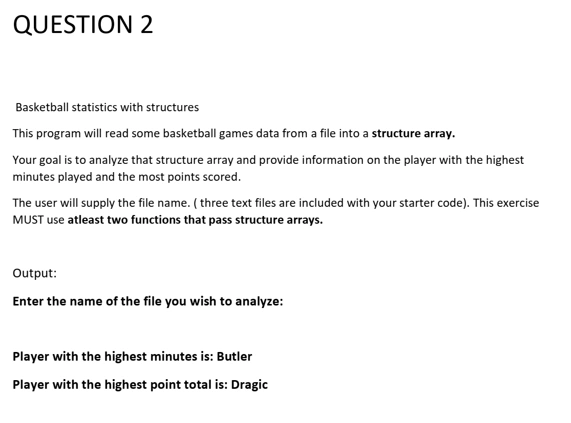
Source Code
#include <iostream.h>
#include <fstream.h>
// Structure that stores information about model houses
struct Game {
char name[100];
int minutes;
int points;
};
// identifiers
struct Game getLongestGame(struct Game games[1000], int n_games);
struct Game getHigherScoreGame(struct Game games[1000], int n_games);
int main() {
// char array to store file names
char file_name[100];
// array fo struct to store games
struct Game games[1000];
// Ask for file name
std::cout << "Enter the name of the file you wish to analyze: ";
std::cin.getline(file_name, 100, '\n');
// variable to count the number of games read
int n_games = 0;
// read file
FILE* myFile;
myFile = fopen(file_name, "r");
// check that file opened correctly
if(myFile == NULL) {
printf("File %s could not be opened.", file_name);
return 1;
}
char name[100];
char line[100];
int minutes, points;
bool isInt = false;
int line_id = 0;
while(fscanf(myFile, "%s %i %i", &name, &minutes, &points) == 3)
{
// Create game
struct Game game;
memcpy(&game.name, &name, 100);
game.minutes = minutes;
game.points = points;
games[n_games] = game;
n_games++;
}
fclose(myFile);
// get longest game
struct Game longest_game = getLongestGame(games, n_games);
// Get game with higher score
struct Game higher_score_game = getHigherScoreGame(games, n_games);
std::cout << "Player with the highest minutes is: " << longest_game.name << std::endl;
std::cout << "Player with the highest point total is: " << higher_score_game.name << std::endl;
std::cout << std::endl << std::endl;
system("pause");
return 0;
}
struct Game getLongestGame(struct Game games[1000], int n_games)
{
// get game with the longest duration
Game ret_game = games[0];
for(int i = 1; i < n_games; i++)
{
if(games[i].minutes > ret_game.minutes) {
ret_game = games[i];
}
}
return ret_game;
}
struct Game getHigherScoreGame(struct Game games[1000], int n_games)
{
// get game with the higher score
Game ret_game = games[0];
for(int i = 1; i < n_games; i++)
{
if(games[i].points > ret_game.points) {
ret_game = games[i];
}
}
return ret_game;
}
Related Samples
Explore C++ Assignment Examples: Delve into our carefully selected collection highlighting C++ solutions for diverse topics, including data structures and algorithms. From foundational concepts to complex projects, each example illustrates effective coding techniques. Improve your C++ proficiency and excel in programming with practical samples crafted to support student learning.
C++
Word Count
6780 Words
Writer Name:Alexander Gough
Total Orders:2435
Satisfaction rate:
C++
Word Count
6185 Words
Writer Name:Neven Bell
Total Orders:2376
Satisfaction rate:
C++
Word Count
5749 Words
Writer Name:Professor Kevin Tan
Total Orders:569
Satisfaction rate:
C++
Word Count
4786 Words
Writer Name:Dr. Isabelle Taylor
Total Orders:580
Satisfaction rate:
C++
Word Count
3997 Words
Writer Name:Professor Oliver Bennett
Total Orders:304
Satisfaction rate:
C++
Word Count
6830 Words
Writer Name:Dr. Maddison Todd
Total Orders:865
Satisfaction rate:
C++
Word Count
4424 Words
Writer Name:Dr. Alexandra Mitchell
Total Orders:589
Satisfaction rate:
C++
Word Count
7250 Words
Writer Name:Mr. Harrison Yu
Total Orders:311
Satisfaction rate:
C++
Word Count
4894 Words
Writer Name:James Miller
Total Orders:824
Satisfaction rate:
C++
Word Count
5544 Words
Writer Name:Callum Chambers
Total Orders:932
Satisfaction rate:
C++
Word Count
3533 Words
Writer Name:Aaliyah Armstrong
Total Orders:658
Satisfaction rate:
C++
Word Count
3645 Words
Writer Name:Len E. Villasenor
Total Orders:489
Satisfaction rate:
C++
Word Count
4033 Words
Writer Name:Daniel M. Harrison
Total Orders:700
Satisfaction rate:
C++
Word Count
3576 Words
Writer Name:Dr. Isabella Wong
Total Orders:652
Satisfaction rate:
C++
Word Count
3600 Words
Writer Name:Prof. Liam Wilson
Total Orders:554
Satisfaction rate:
C++
Word Count
1035 Words
Writer Name:Professor Marcus Tan
Total Orders:544
Satisfaction rate:
C++
Word Count
6288 Words
Writer Name:Dr. Stephanie Kim
Total Orders:812
Satisfaction rate:
C++
Word Count
1586 Words
Writer Name:Ethan Patel
Total Orders:556
Satisfaction rate:
C++
Word Count
3852 Words
Writer Name:Sarah Rodriguez
Total Orders:689
Satisfaction rate:
C++
Word Count
4701 Words
Writer Name:Harry F. Grimmett
Total Orders:342
Satisfaction rate: