Instructions
Objective
Write a python assignment program to create a webcam selling system.
Requirements and Specifications
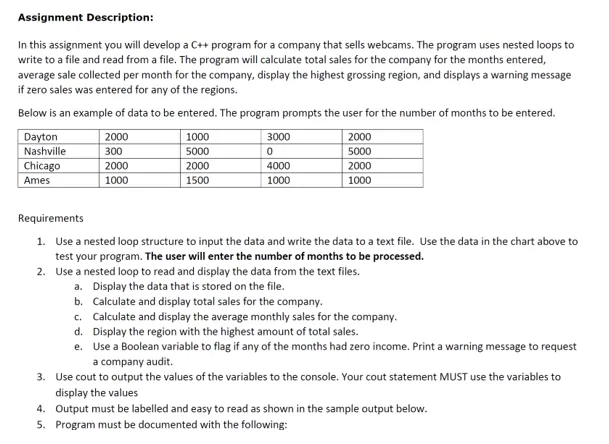
Source Code
// Name:
// Date: 06/06/2021
// Program Name:
// Description: This program records the number of sales per region, per month
#include
#include
#include
#include
using namespace std;
// define a struct named region
struct Region
{
string name;
float month_sales[12]; // max 12 months
};
int main()
{
int n_months; // variable to store number of months
nt n_regions; // variable to store number of regions
float month_sale; // variable to store the number of sales inputted by user
cout << "Enter number of regions ";
cin >> n_regions;
cout << "Enter number of months ";
cin >> n_months;
// create an array to store regions
struct Region regions[100]; // max 100 regions
struct Region region; // region object to store a region info
string region_name;
cin.ignore();
for (int i = 0; i < n_regions; i++)
{
cout << "Enter region name" << endl;
getline(cin, region_name);
region.name = region_name;
for (int j = 0; j < n_months; j++)
{
cout << "Enter month " << (j + 1) << " sales" << endl;
cin >> month_sale;
region.month_sales[j] = month_sale;
cin.ignore();
}
regions[i] = region;
}
cout << endl << endl;
// print regions
cout << fixed << setprecision(2);
for (int i = 0; i < n_regions; i++)
{
cout << left << setw(25) << regions[i].name << setw(10);
for (int j = 0; j < n_months; j++)
{
cout << regions[i].month_sales[j] << setw(10);
}
cout << endl;
}
cout << endl;
// Do calculations
float total_sales = 0;
float average_sales = 0.0;
int highest_sale_idx = -1;
float highest_sale = 0;
float highest_sale_region = 0;
// check regions with zero sales
int zero_sales = 0;
for (int i = 0; i < n_regions; i++)
{
highest_sale_region = 0;
for (int j = 0; j < n_months; j++)
{
if (regions[i].month_sales[j] == 0)
{
zero_sales = 1;
}
total_sales += regions[i].month_sales[j];
highest_sale_region += regions[i].month_sales[j];
}
if (highest_sale_region > highest_sale)
{
highest_sale = highest_sale_region;
highest_sale_idx = i;
}
}
average_sales = total_sales / (float)n_regions;
// Now print info
if (zero_sales == 1) // there is at least one region with zero sales
cout << "One of the regions recorded zero sales\nA company audit is required" << endl << endl;
// Print total, average and the region with the highest sales
cout << "Total sales for the company " << setw(10) << total_sales << endl;
cout << "Average region sales: " << setw(10) << average_sales << endl << endl;
cout << "The complex with the highest sales is " << regions[highest_sale_idx].name << " with " << highest_sale << endl;
// Now, write file
ofstream output_file("output.txt");
if (output_file.is_open())
{
// write
output_file << fixed << setprecision(2);
output_file << setw(70) << "Region Total" << endl;
for (int i = 0; i < n_regions; i++)
{
output_file << left << setw(25) << regions[i].name << setw(10);
for (int j = 0; j < n_months; j++)
{
output_file << regions[i].month_sales[j] << setw(10);
}
if (highest_sale_idx == i)
output_file << setw(30) << "Highest";
output_file << endl;
}
output_file << left << setw(70) << "Total Sales" << setw(10) << total_sales << endl;
output_file << left << setw(70) << "Average" << setw(10) << average_sales << endl;
output_file.close();
}
else
cout << "Error: Could not write the file output.txt" << endl;
}
Related Samples
View our free Python assignment samples to gain clarity and improve your understanding. These samples offer clear explanations and practical examples, making complex Python concepts easier to grasp.
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python