Instructions
Requirements and Specifications
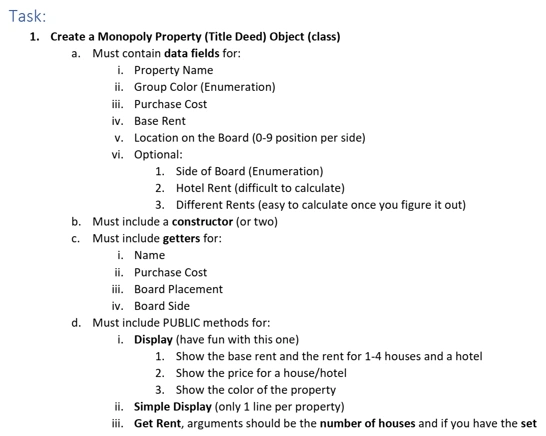
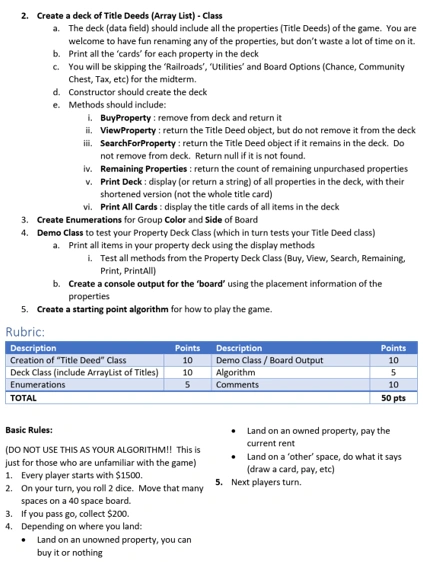
Source Code
COLOR
/**
* Property groups, represented by colors
*/
public enum Color {
RED,
ORANGE,
YELLOW,
GREEN,
BLUE,
PURPLE,
BLACK,
WHITE,
}
DECK
import java.util.ArrayList;
public class Deck {
/**
* Collection, containing all TitleDeeds, which are not bought year
*/
private final ArrayListtitleDeeds;
/**
* No-args constructor - creates deck with full set of properties
*/
public Deck() {
titleDeeds = new ArrayList<>();
// iterating over all colors and creating whole color groups one by one
for (int j = 0; j
Color color = Color.values()[j];
Side side = null;
switch (j / 2) {
case 0:
side = Side.NORTH;
break;
case 1:
side = Side.EAST;
break;
case 2:
side = Side.SOUTH;
break;
case 3:
side = Side.WEST;
break;
default:
throw new IllegalStateException();
}
String colorStr = color.name();
for (int i = 0; i<3; i++) {
// calculating reasonable locations
int loc = (j % 2) * 5 + i * 2;
// making simple property names out of color name and number
titleDeeds.add(new TitleDeed(colorStr + " house #" + (i+1), color, side, loc));
}
}
}
/**
* Method for buying property from the deck.
* If found, removes property from deck and returns it
* @param name of property to buy
* @return bought property or null, if property with such name does not exist in deck
*/
public TitleDeed buyProperty(String name) {
TitleDeed target = searchForProperty(name);
if (target == null) {
return null;
}
titleDeeds.remove(target);
return target;
}
/**
* Method for getting string details of property from the deck.
* @param name of property to get details
* @return details of property or null, if property with such name does not exist in deck
*/
public String viewProperty(String name) {
TitleDeed target = searchForProperty(name);
if (target == null) {
return null;
}
return target.display();
}
/**
* Method for getting property object from the deck by name.
* @param name of property to search for
* @return property with given name or null, if it does not exist
*/
public TitleDeed searchForProperty(String name) {
return titleDeeds.stream().filter(td -> td.getName().equals(name)).findAny().orElse(null);
}
/**
* Method for getting property object from the deck by its location.
* @param side of property to search for
* @param loc location of property to search for
* @return property with given position or null, if it does not exist
*/
public TitleDeed searchForProperty(Side side, int loc) {
return titleDeeds.stream().filter(td -> td.getSide() == side && td.getLocation() == loc).findAny().orElse(null);
}
/**
* Method for getting current deck size
* @return deck size
*/
public int remainingProperties() {
return titleDeeds.size();
}
/**
* Method for getting deck string representation, obtained from simple string representation
* for each card in deck
* @return compact string representation
*/
public String printDeck() {
StringBuilder builder = new StringBuilder();
for (TitleDeed td : titleDeeds) {
builder.append(td.simpleDisplay()).append(System.lineSeparator());
}
return builder.toString();
}
/**
* Method for getting deck string representation, obtained from full string representation
* for each card in deck
* @return full string representation
*/
public String printAllCards() {
StringBuilder builder = new StringBuilder();
for (TitleDeed td : titleDeeds) {
builder.append(td.display()).append(System.lineSeparator()).append(System.lineSeparator());
}
return builder.toString();
}
}
DEMO
public class Demo {
/**
* Method for showing console representation of game board
* @param deck to show board for
*/
private static void printBoard(Deck deck) {
// iterating over all sides, showing properties for each side
for (Side side : Side.values()) {
System.out.println("Side: " + side.name());
// iterating over all valid locations, showing property on each location, if exists
for (int i = 0; i < 10; i++) {
TitleDeed td = deck.searchForProperty(side, i);
System.out.print(i + ": ");
if (td == null) {
System.out.println();
} else {
System.out.println(td.simpleDisplay());
}
}
}
}
public static void main(String[] args) {
// checking printing methods
Deck deck = new Deck();
System.out.println(deck.printDeck());
System.out.println();
System.out.println(deck.printAllCards());
System.out.println();
printBoard(deck);
// covering all methods in Deck class
int size = deck.remainingProperties();
System.out.println("Deck size: " + size);
if (size == 24) {
System.out.println("Size of deck is correct. CORRECT");
} else {
System.out.println("Deck size is incorrect. FAIL!!!");
return;
}
System.out.println("Searching for Property with name \"foo\"");
TitleDeed foo = deck.searchForProperty("foo");
if (foo != null) {
System.out.println("Foo was found. FAIL!!!");
return;
} else {
System.out.println("Foo was not found. CORRECT");
}
System.out.println("Searching for Property with name \"RED house #2\"");
TitleDeed redHouse2 = deck.searchForProperty("RED house #2");
if (redHouse2 != null) {
System.out.println("Found. CORRECT");
} else {
System.out.println("Existing property was not. FAIL!!!");
return;
}
System.out.println("Getting info for this property:");
System.out.println(deck.viewProperty(redHouse2.getName()));
System.out.println("Trying to by this property:");
TitleDeed redHouse2Bought = deck.buyProperty(redHouse2.getName());
if (redHouse2 != redHouse2Bought) {
System.out.println("Incorect return value of bought method. FAIL!!!");
return;
} else {
System.out.println("Correct value returned by buy method. CORRECT");
}
int newSize = deck.remainingProperties();
System.out.println("Deck size: " + newSize);
if (size == newSize + 1) {
System.out.println("Size of deck is correct. CORRECT");
} else {
System.out.println("Deck size is incorrect. FAIL!!!");
return;
}
}
}
SIDE
/**
* Four sides of Monopoly board
*/
public enum Side {
NORTH,
SOUTH,
EAST,
WEST;
}
TITLE DEED
public class TitleDeed {
/**
* Property name
*/
private final String name;
/**
* Color of property
*/
private final Color groupColor;
/**
* Monopoly board side of property
*/
private final Side side;
/**
* Location of the property on the side: integer from 0 to 9
*/
private final int location;
/**
* Cost of property
*/
private final double purchaseCost;
/**
* Rent for property without any upgrades (houses/hotel)
*/
private final double baseRent;
/**
* Array, representing rent amount for given number of houses:
* housesRent[0] - rent with 1 house
* housesRent[1] - rent with 2 houses
* etc
*/
private final double[] housesRent;
/**
* Rent amount of property with hotel
*/
private final double hotelRent;
/**
* Constructor, creating Property with default prices
* @param name property name
* @param groupColor property color
* @param side property position side
* @param location property position on the side
*/
public TitleDeed(String name, Color groupColor, Side side, int location) {
this(name, groupColor, side, location, 100.0, 10.0, new double[]{50, 100, 150, 200}, 400);
}
/**
* All args constructor for Property
* @param name property name
* @param groupColor property color
* @param side propertt position side
* @param location property position on the side
* @param purchaseCost property price
* @param baseRent property rent without upgrades
* @param housesRent array, represetning rent amount for number of houses
* @param hotelRent rent amount with hotel
*/
public TitleDeed(String name, Color groupColor, Side side, int location, double purchaseCost, double baseRent, double[] housesRent, double hotelRent) {
this.name = name;
this.groupColor = groupColor;
this.side = side;
this.location = location;
this.purchaseCost = purchaseCost;
this.baseRent = baseRent;
this.housesRent = housesRent;
this.hotelRent = hotelRent;
}
/**
* Getter for name field
* @return property name
*/
public String getName() {
return name;
}
/**
* Getter for purchaseCost field
* @return property price
*/
public double getPurchaseCost() {
return purchaseCost;
}
/**
* Getter for side field
* @return propert position side
*/
public Side getSide() {
return side;
}
/**
* Getter for location field
* @return property position on the side
*/
public int getLocation() {
return location;
}
/**
* Method for getting full string representation of the property
* @return full string representation of the property
*/
public String display() {
StringBuilder builder = new StringBuilder("\"" + name + "\"");
builder.append(System.lineSeparator());
builder.append("Side: ").append(side).append(" Location: ").append(location).append(System.lineSeparator());
builder.append("Price $: ").append(purchaseCost).append(System.lineSeparator());
builder.append("Rent $: ").append(baseRent).append(System.lineSeparator());
for (int i = 0; i<4; i++) {
builder.append("With " + (i+1) + " house(s): $" + housesRent[i]).append(System.lineSeparator());
}
builder.append("With hotel: $" + hotelRent);
return builder.toString();
}
/**
* Method for getting short string representation of the property
* @return short string representation of the property
*/
public String simpleDisplay() {
return "\"" + name + "\" Price: $" + purchaseCost;
}
/**
* Method for calculate current rent of property
* @param houses number of houses
* @param hasHotel if property has hotel or not
* @return rent amount
*/
public double getRent(int houses, boolean hasHotel) {
if (hasHotel) {
return hotelRent;
}
if (houses == 0) {
return baseRent;
}
return housesRent[houses-1];
}
}
Related Samples
Explore our Java Assignments sample section, where we offer expertly crafted solutions to enhance your understanding and proficiency in Java programming. Whether you're tackling basic syntax or complex algorithms, find comprehensive examples designed to support your learning and excel in your coursework.
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java