Instructions
Objective
Write a java assignment program to create department management system.
Requirements and Specifications
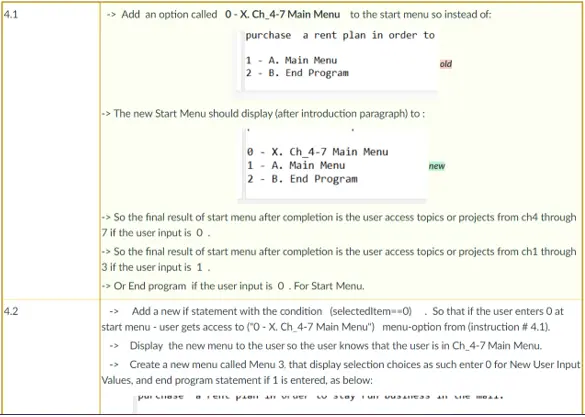
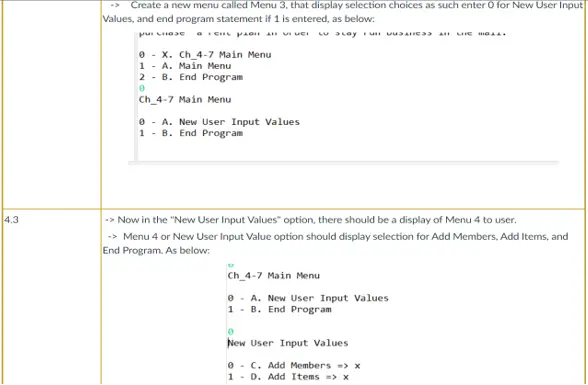
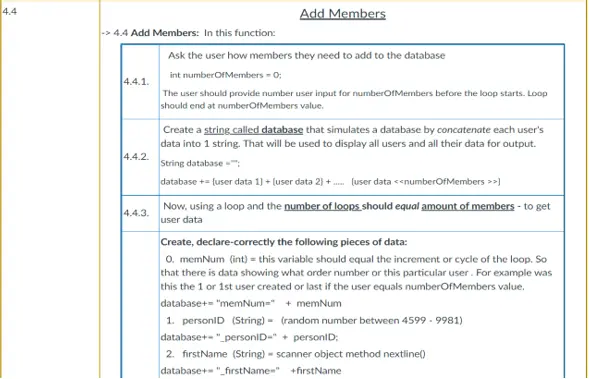
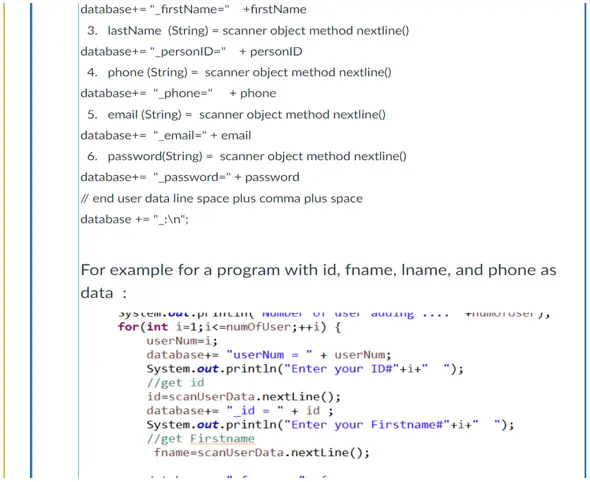
.webp)
Source Code
package crn23004.MOD_03.ClothingDepartmentStore;
import java.util.Random;
import java.util.Scanner;
public class ManagementSystem_EJP3_MainMenu {
public static void main(String[] args) {
// Initialize all variables needed for the program
Scanner scanner = new Scanner(System.in);
final double TRANSACTION_TAX = 0.0825f;
int menuOption = 0;
long customerID = 0;
short itemID = 0;
byte itemQuantity = 0;
double itemPrice = 0;
double itemTotalLinePrice = 0;
double pricePerTransaction = 0;
// Display the introduction
System.out.println("Welcome to Maria's Clothing Department Store:");
System.out.println("We have various elegant clothes with affordable price.");
System.out.println("Today is the last day of the discount of our new arrival products.");
System.out.println("Our staff is friendly and helpful.");
System.out.println("===================================================");
// Display user option
System.out.println("0. Ch_4-7 Main Menu");
System.out.println("1. Main Menu");
System.out.println("2. End Program");
System.out.print("Option: ");
menuOption = Integer.parseInt(scanner.nextLine());
if (menuOption == 0) {
// processing new menu option
// showing submenu for new submenu
System.out.println("\n===================================================");
System.out.println("Ch_4-7 Main Menu\n");
System.out.println("0. New User Input Values");
System.out.println("1. End Program");
System.out.print("Option: ");
// parsing user choice input
menuOption = Integer.parseInt(scanner.nextLine());
if (menuOption == 0) {
// processing submenu option
System.out.println("\n===================================================");
System.out.println("New User Input Values\n");
int loopMem = 0;
loopMem = 1;
// keep iterating until user wants to quit
while (loopMem == 1) {
System.out.println("0. Add Members => X");
System.out.println("1. Add Items => X");
System.out.print("Option: ");
menuOption = Integer.parseInt(scanner.nextLine());
if (menuOption == 0) {
// processing "Add members" suboption
Random random = new Random();
System.out.println("\n===================================================");
System.out.println("Add Members");
// asking user for number of users to add
System.out.println("How many user to add to the database: ");
int numberOfMembers = Integer.parseInt(scanner.nextLine());
int memNum;
String database = "";
String personID, firstName, lastName, phone, email, password;
// adding entered number of users
System.out.println("Number of user adding ...." + numberOfMembers);
for (int i = 1; i <= numberOfMembers; i++) {
// reading each member attribute
// accumulating result string value in database variable
memNum = i;
database += "memnum = " + memNum;
personID = Integer.toString(random.nextInt(5383) + 4599);
database += "_personID = " + personID;
System.out.println("Enter your Firstname#" + i);
firstName = scanner.nextLine();
database += "_firstName = " + firstName;
System.out.println("Enter your Lastname#" + i);
lastName = scanner.nextLine();
database += "lastName = " + lastName;
System.out.println("Enter your Phone#" + i);
phone = scanner.nextLine();
database += "_phone = " + phone;
System.out.println("Enter your Email#" + i);
email = scanner.nextLine();
database += "_email = " + email;
System.out.println("Enter your Password#" + i);
password = scanner.nextLine();
database += "_password = " + password + System.lineSeparator();
System.out.println("****User Update***");
}
// outputting result database string
System.out.print(database);
} else if (menuOption == 1) {
// processing "Add Items" suboption
System.out.println("\n===================================================");
System.out.println("Add Items");
// initializing values of items
boolean outOfStock = false;
boolean addItem = true;
long availableQuantity = 10;
long qtyCase1 = availableQuantity;
long qtyCase2 = availableQuantity;
long qtyCase3 = availableQuantity;
long qtyCase4 = availableQuantity;
long qtyCase5 = availableQuantity;
// keep asking for items until user wants
while (addItem) {
// Create variables of some data type with default value
byte lineItem = 0;
// Zero-out previous variables
customerID = 0;
itemID = 0;
itemQuantity = 0;
itemPrice = 0;
itemTotalLinePrice = 0;
pricePerTransaction = 0;
System.out.println("Shop & Checkout");
// Display items to choose from
System.out.println("Select an item, select line 1 - 5 from the menu below:");
System.out.println("Line Item: " + (++lineItem)
+ ", ID: 100, Name: Shirt, Description: Upper Garment, Available Quantity: " + qtyCase1 + ", Price Per Item: $5.00");
System.out.println("Line Item: " + (++lineItem)
+ ", ID: 200, Name: Pants, Description: Lower Garment, Available Quantity: " + qtyCase2 + ", Price Per Item: $6.00");
System.out.println("Line Item: " + (++lineItem)
+ ", ID: 300, Name: Boxers, Description: Male Underwear, Available Quantity: " + qtyCase3 + ", Price Per Item: $2.50");
System.out.println("Line Item: " + (++lineItem)
+ ", ID: 400, Name: Socks, Description: Foot Garment, Available Quantity: " + qtyCase4 + ", Price Per Item: $1.50");
System.out.println("Line Item: " + (++lineItem)
+ ", ID: 500, Name: Belt, Description: Waist Garment, Available Quantity: " + qtyCase5 + ", Price Per Item: $1.00");
System.out.print("Line Item Option: ");
lineItem = Byte.parseByte(scanner.nextLine());
// Set the quantity and price per item based on selected line item
switch (lineItem) {
case 1:
itemID = 100;
availableQuantity = qtyCase1;
itemPrice = 5;
break;
case 2:
itemID = 200;
availableQuantity = qtyCase2;
itemPrice = 6;
break;
case 3:
itemID = 300;
availableQuantity = qtyCase3;
itemPrice = 2.5f;
break;
case 4:
itemID = 400;
availableQuantity = qtyCase4;
itemPrice = 1.5f;
break;
case 5:
itemID = 500;
availableQuantity = qtyCase5;
itemPrice = 1;
break;
default:
lineItem = -1;
}
System.out.println();
if (lineItem != -1) {
// Display the selected item
System.out.print(lineItem + " - " + itemID + " - ");
switch (lineItem) {
case 1:
System.out.print("Shirt - Upper Garment");
break;
case 2:
System.out.print("Pants - Lower Garment");
break;
case 3:
System.out.print("Boxers - Male Underwear");
break;
case 4:
System.out.print("Socks - Foot Garment");
break;
case 5:
System.out.print("Belt - Waist Garment");
break;
}
System.out.println(" - " + availableQuantity + " - " + itemPrice);
// Ask for the customer's ID
System.out.print("Enter customer ID: ");
customerID = Long.parseLong(scanner.nextLine());
// Ask for the quantity
System.out.print("Enter quantity to purchase: ");
itemQuantity = Byte.parseByte(scanner.nextLine());
if (itemQuantity > availableQuantity) {
// Check if the available quantity is enough
System.out.println("Out of stock, transaction cannot be processed!");
System.out.println("\n===================================================");
System.out.println("Thank you for purchasing in Maria's Clothing Department Store.");
System.out.println("Have a great day with your new style!");
System.out.println("End of program.");
outOfStock = true;
break;
} else if (itemQuantity > 0 && itemQuantity <= availableQuantity) {
// If there's available quantity then process it
System.out.println("Item is available for purchase!");
availableQuantity -= itemQuantity;
switch (lineItem) {
case 1:
qtyCase1 -= itemQuantity;
break;
case 2:
qtyCase2 -= itemQuantity;
break;
case 3:
qtyCase3 -= itemQuantity;
break;
case 4:
qtyCase4 -= itemQuantity;
break;
case 5:
qtyCase5 -= itemQuantity;
break;
}
// Display the available quantity left after purchase
if (availableQuantity == 0)
System.out.println("NOW - out of stock, current # of items left: " + availableQuantity);
else
System.out.println("Item is available, current # of items left: " + availableQuantity);
// Calculate the total
itemTotalLinePrice = itemPrice * itemQuantity;
System.out.printf("\n%d's transactional balance equals $%.2f.\n", customerID, itemTotalLinePrice);
pricePerTransaction = itemTotalLinePrice + (itemTotalLinePrice * TRANSACTION_TAX);
System.out.printf("\nCustomer Identification Number: %d\n", customerID);
System.out.printf("Transaction payment: $%.2f.\n", pricePerTransaction);
} else {
System.out.println("Invalid quantity.");
}
}
// asking if user wants add another item
System.out.println("Another item to add to cart? \n1 - Yes or \n2 - No");
int selectAnotherItem = Integer.parseInt(scanner.nextLine());
addItem = selectAnotherItem == 1;
}
if (!outOfStock) {
System.out.printf("Amount Due: $%.2f%n", pricePerTransaction);
}
}
System.out.println("1 - Again\n2 - Exit");
loopMem = Integer.parseInt(scanner.nextLine());
}
}
} else if (menuOption == 1) {
// Show an inner menu
System.out.println("\n===================================================");
System.out.println("1. Print user info");
System.out.println("2. Shop & Checkout");
System.out.println("3. End Program");
System.out.print("Option: ");
menuOption = Integer.parseInt(scanner.nextLine());
if (menuOption == 1) {
// Print all variable's values
System.out.println("customerID = " + customerID);
System.out.println("itemID = " + itemID);
System.out.println("itemQuantity = " + itemQuantity);
System.out.println("itemPrice = " + itemPrice);
System.out.println("itemTotalLinePrice = " + itemTotalLinePrice);
System.out.println("pricePerTransaction = " + pricePerTransaction);
} else if (menuOption == 2) {
// Create variables of some data type with default value
byte lineItem = 0;
long availableQuantity = 0;
// Zero-out previous variables
customerID = 0;
itemID = 0;
itemQuantity = 0;
itemPrice = 0;
itemTotalLinePrice = 0;
pricePerTransaction = 0;
// Display more options
System.out.println("Select an item, select line 1 - 5 from the menu below:");
System.out.println("Line Item: " + (++lineItem)
+ ", ID: 100, Name: Shirt, Description: Upper Garment, Available Quantity: 1, Price Per Item: $5.00");
System.out.println("Line Item: " + (++lineItem)
+ ", ID: 200, Name: Pants, Description: Lower Garment, Available Quantity: 2, Price Per Item: $6.00");
System.out.println("Line Item: " + (++lineItem)
+ ", ID: 300, Name: Boxers, Description: Male Underwear, Available Quantity: 3, Price Per Item: $2.50");
System.out.println("Line Item: " + (++lineItem)
+ ", ID: 400, Name: Socks, Description: Foot Garment, Available Quantity: 4, Price Per Item: $1.50");
System.out.println("Line Item: " + (++lineItem)
+ ", ID: 500, Name: Belt, Description: Waist Garment, Available Quantity: 5, Price Per Item: $1.00");
System.out.print("Line Item Option: ");
lineItem = Byte.parseByte(scanner.nextLine());
// Set the quantity and price per item based on selected line item
switch (lineItem) {
case 1:
itemID = 100;
availableQuantity = 1;
itemPrice = 5;
break;
case 2:
itemID = 200;
availableQuantity = 2;
itemPrice = 6;
break;
case 3:
itemID = 300;
availableQuantity = 3;
itemPrice = 2.5f;
break;
case 4:
itemID = 400;
availableQuantity = 4;
itemPrice = 1.5f;
break;
case 5:
itemID = 500;
availableQuantity = 5;
itemPrice = 1;
break;
default:
lineItem = -1;
}
System.out.println();
if (lineItem != -1) {
// Display the selected item
System.out.print(lineItem + " - " + itemID + " - ");
switch (lineItem) {
case 1:
System.out.print("Shirt - Upper Garment");
break;
case 2:
System.out.print("Pants - Lower Garment");
break;
case 3:
System.out.print("Boxers - Male Underwear");
break;
case 4:
System.out.print("Socks - Foot Garment");
break;
case 5:
System.out.print("Belt - Waist Garment");
break;
}
System.out.println(" - " + availableQuantity + " - " + itemPrice);
// Ask for the customer's ID
System.out.print("Enter customer ID: ");
customerID = Long.parseLong(scanner.nextLine());
// Ask for the quantity
System.out.print("Enter quantity to purchase: ");
itemQuantity = Byte.parseByte(scanner.nextLine());
if (itemQuantity > availableQuantity) {
// Check if the available quantity is enough
System.out.println("Out of stock, transactoin cannot be processed!");
System.out.println("\n===================================================");
System.out.println("Thank you for purchasing in Maria's Clothing Department Store.");
System.out.println("Have a great day with your new style!");
System.out.println("End of program.");
} else if (itemQuantity > 0 && itemQuantity <= availableQuantity) {
// If there's available quantity then process it
System.out.println("Item is available for purchase!");
availableQuantity -= itemQuantity;
// Display the available quantity left after purchase
if (availableQuantity == 0)
System.out.println("NOW - out of stock, current # of items left: " + availableQuantity);
else
System.out.println("Item is available, current # of items left: " + availableQuantity);
// Calculate the total
itemTotalLinePrice = itemPrice * itemQuantity;
System.out.printf("\n%d's transactional balance equals $%.2f.\n", customerID, itemTotalLinePrice);
pricePerTransaction = itemTotalLinePrice + (itemTotalLinePrice * TRANSACTION_TAX);
System.out.printf("\nCustomer Identification Number: %d\n", customerID);
System.out.printf("Transaction payment: $%.2f.\n", pricePerTransaction);
} else {
System.out.println("Invalid quantity.");
}
}
} else if (menuOption != 3) {
System.out.println("Invalid option.");
}
} else if (menuOption != 2) {
System.out.println("Invalid option.");
}
// Exit with a message
System.out.println("\n===================================================");
System.out.println("Thank you for purchasing in Maria's Clothing Department Store.");
System.out.println("Have a great day with your new style!");
System.out.println("Program Shutdown.");
System.out.println("\n===================================================");
}
}
Similar Samples
Discover the quality of our work through these detailed samples. Each example showcases our expertise in various programming languages and problem-solving skills. Whether you're looking for assistance in Python, Java, C++, or any other programming language, our samples highlight the precision and proficiency you can expect from our services.
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java