Instructions
Objective
Write a java assignment program to create a car tracker system.
Requirements and Specifications
.webp)
Screenshot
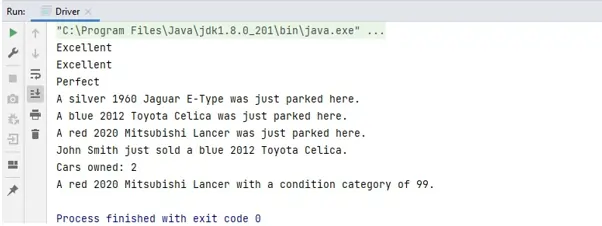
Source Code
public class Car {
private int year;
private String make;
private String model;
private String color;
private int conditionCategory;
private boolean isRestored;
public Car(int year, String make, String model, String color, int conditionCategory) {
this.year = year;
this.make = make;
this.model = model;
this.color = color;
this.conditionCategory = (conditionCategory > 100 || conditionCategory < 40) ? 80 : conditionCategory;
if (conditionCategory >= 90) {
this.isRestored = true;
System.out.println("Perfect");
} else {
this.isRestored = false;
if (conditionCategory >= 80) {
System.out.println("Excellent");
} else if (conditionCategory >= 70) {
System.out.println("Fine");
} else if (conditionCategory >= 60) {
System.out.println("Very Good");
} else if (conditionCategory >= 50) {
System.out.println("Good");
} else {
System.out.println("Driver");
}
}
}
public Car(int year, String make, String model) {
this(year, make, model, "blue", 80);
}
public Car() {
this(1960, "Jaguar", "E-Type", "silver", 89);
}
public int getYear() {
return year;
}
public String getMake() {
return make;
}
public String getModel() {
return model;
}
public int getConditionCategory() {
return conditionCategory;
}
public String getColor() {
return color;
}
}
DRIVER
public class Driver {
public static void main(String[] args) {
GarageOwner garageOwner = new GarageOwner("John Smith", 29);
Garage garage = new Garage(garageOwner, new Car[3]);
Car car1 = new Car();
Car car2 = new Car(2012, "Toyota", "Celica");
Car car3 = new Car(2020, "Mitsubishi", "Lancer", "red", 99);
garage.addCar(0, car1);
garage.addCar(1, car2);
garage.addCar(2, car3);
garage.sellCar(1);
System.out.println("Cars owned: " + garageOwner.getCarsOwned());
garage.showCertainCars(89);
}
}
GARAGE
public class Garage {
private GarageOwner theOwner;
private Car[] carCatalogue;
public Garage(GarageOwner theOwner, Car[] carCatalogue) {
this.theOwner = theOwner;
this.carCatalogue = carCatalogue;
}
public Garage() {
this(new GarageOwner("Enzo Ferrari", 35), new Car[4]);
}
public Car addCar(int index, Car car) {
if (index < 0 || index >= carCatalogue.length) {
System.out.println("Cannot add a car to this spot.");
return null;
}
Car prev = carCatalogue[index];
if (prev == null) {
System.out.printf("A %s %d %s %s was just parked here.%n",
car.getColor(), car.getYear(), car.getMake(), car.getModel());
theOwner.setCarsOwned(theOwner.getCarsOwned() + 1);
} else {
System.out.printf("A %s %d %s %s was just parked here.%n",
prev.getColor(), prev.getYear(), prev.getMake(), prev.getModel());
}
carCatalogue[index] = car;
return prev;
}
public Car sellCar(int index) {
if (index < 0 || index >= carCatalogue.length || carCatalogue[index] == null) {
System.out.println("There is no car to sell.");
return null;
}
Car car = carCatalogue[index];
System.out.printf("%s just sold a %s %d %s %s.%n",
theOwner.getName(), car.getColor(), car.getYear(), car.getMake(), car.getModel());
theOwner.setCarsOwned(theOwner.getCarsOwned() - 1);
carCatalogue[index] = null;
return car;
}
public void showCertainCars(int conditionalCategory) {
for (int i = 0; i < carCatalogue.length; i++) {
Car car = carCatalogue[i];
if (car == null || car.getConditionCategory() <= conditionalCategory) {
continue;
}
System.out.printf("A %s %d %s %s with a condition category of %d.%n",
car.getColor(), car.getYear(), car.getMake(), car.getModel(), car.getConditionCategory());
}
}
}
Related Samples
At ProgrammingHomeworkHelp.com, we offer comprehensive assignment support for students, featuring a variety of expertly crafted Java assignment samples. These samples provide valuable insights into solving complex Java problems, helping students enhance their coding skills and understanding of Java programming concepts. Whether you're tackling basic syntax or advanced algorithms, our detailed examples serve as a reliable guide. Explore our Java samples today to gain the confidence and knowledge needed to excel in your coursework. Let ProgrammingHomeworkHelp.com be your trusted partner in academic success.
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java