Instructions
Objective
Write a C++ assignment program to create a battleship game in C++. In this assignment, your task is to implement a program that simulates a battleship game using C++. You will need to design the game board, allow players to place their battleships, and take turns guessing the coordinates to attack the opponent's ships. This assignment will test your understanding of C++ programming concepts such as classes, objects, arrays, loops, and conditional statements. By completing this assignment, you will not only enhance your C++ programming skills but also gain experience in designing and implementing interactive games.
Requirements and Specifications
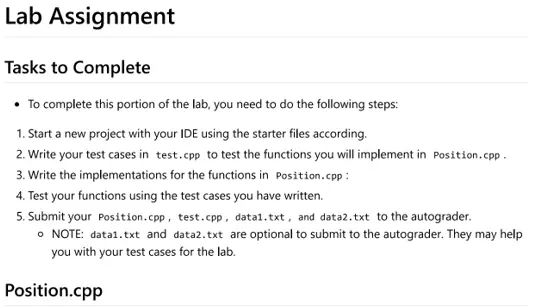
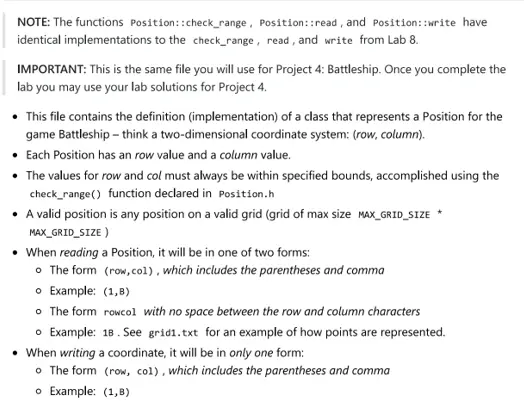
Source Code
/**
* Copyright 2019 University of Michigan EECS183
*
* Position.cpp
* Project UID 8885f2d9f10d2f99bc099aa9c3fc0543
*
* <#Name#>
* <#Uniqname#>
*
* Project 4: Battleship
*
* <#description#>
*/
#include "Position.h"
#include "utility.h"
Position::Position() {
// TODO: write implementation here.
row = 0;
col = 0;
}
Position::Position(int row_in, int col_in) {
// TODO: write implementation here.
row = row_in;
col = col_in;
}
Position::Position(char row_in, char col_in) {
// TODO: write implementation here.
// First, convert both char values to integers
int row_inte = row_in - '0';
int col_inte = col_in - '0';
// If the values are between 65 and 72 it is because these are A, B, ... H
if(row_inte >= 65 && row_inte <= 72) {
row_inte -= 65;
}
else if(row_inte >= 97 && row_inte <= 104) { // these are a, b, c.. h
row_inte -= 97;
} else if(row_inte >= 1 && row_inte <= 8) {
row_inte -= 1;
}
if(col_inte >= 65 && col_inte <= 72) {
col_inte -= 65;
}
else if(col_inte >= 97 && col_inte <= 104) { // these are a, b, c.. h
col_inte -= 97;
} else if(col_inte >= 1 && col_inte <= 8) {
col_inte -= 1;
}
// Finally, set the valeus using the check_range function
row = check_range(row_inte);
col = check_range(col_inte);
}
int Position::get_row() {
// TODO: write implementation here.
return row;
}
void Position::set_row(int row_in) {
// TODO: write implementation here.
row = row_in;
}
int Position::get_col() {
// TODO: write implementation here.
return col;
}
void Position::set_col(int col_in) {
// TODO: write implementation here.
col = col_in;
}
void Position::read(istream &is) {
// TODO: write implementation here.
// First, read the line
std::string raw_pos;
is >> raw_pos;
// Check if it contains parenthesys
char rowc, colc;
if(raw_pos[0] == '(')
{
rowc = raw_pos.at(1);
colc = raw_pos.at(3);
}
else {
rowc = raw_pos[0];
colc = raw_pos[1];
}
// Instantiante a new POsition
Position p(rowc, colc);
row = p.get_row();
col = p.get_col();
return;
}
void Position::write(ostream &os) {
// TODO: write implementation here.
// Convert col to be in the range [1,8]
int row_new = row+1;
// Convert column to char
char col_new = (char)(col + 65);
// Write
os << "(" << row_new << "," << col_new << ")";
return;
}
int Position::check_range(int val) {
// TODO: write implementation here.
if(val >= 0 && val < MAX_GRID_SIZE) {
return val;
}
return 0;
}
// Your code goes above this line.
// Don't change the implementations below!
istream &operator>>(istream &is, Position &pos) {
pos.read(is);
return is;
}
ostream &operator<<(ostream &os, Position pos) {
pos.write(os);
return os;
}
Similar Samples
Explore our comprehensive collection of programming homework samples across various disciplines including Java, Python, C++, and more. Each sample exemplifies our expertise in solving diverse coding challenges with clarity and precision. Whether you're working on algorithms, data structures, or software projects, our samples demonstrate our commitment to delivering reliable solutions tailored to your academic needs.
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++
C++