Instructions
Objective
Write a python assignment program to convert english to morse code.
Requirements and Specifications
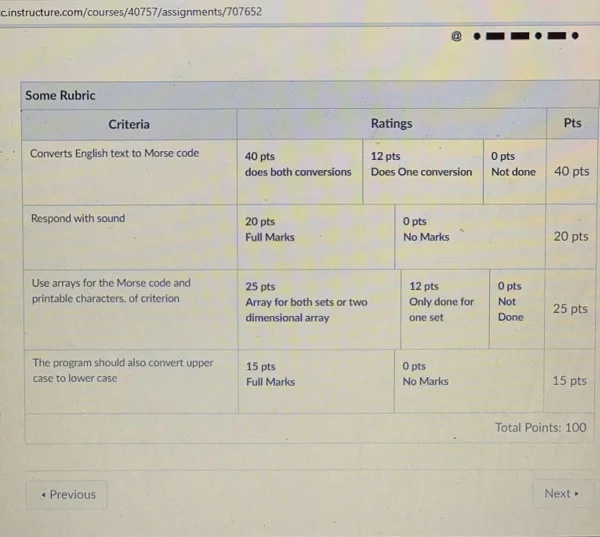
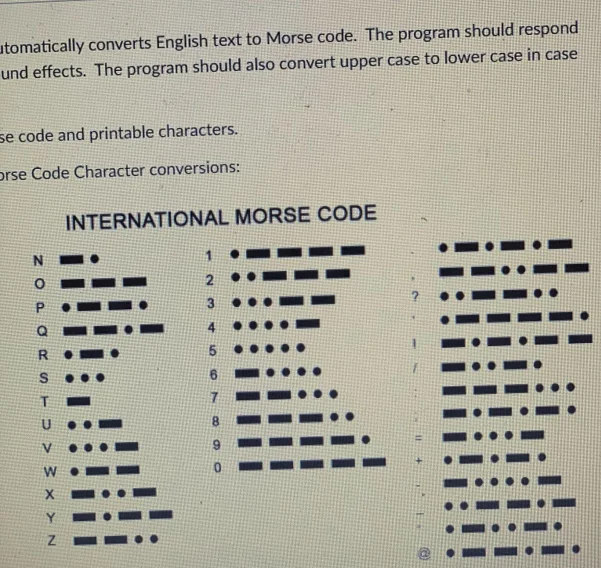
Source Code
import winsound # used to reproduce the morse code with sound
import time
# Dictionary representing the morse code chart
MORSE_CODE_ALPHABET = {
'a':'.-',
'b':'-...',
'c':'-.-.',
'd':'-..',
'e':'.',
'f':'..-.',
'g':'--.',
'h':'....',
'i':'..',
'j':'.---',
'k':'-.-',
'l':'.-..',
'm':'--',
'n':'-.',
'o':'---',
'p':'.--.',
'q':'--.-',
'r':'.-.',
's':'...',
't':'-',
'u':'..-',
'v':'...-',
'w':'.--',
'x':'-..-',
'y':'-.--',
'z':'--..',
'1':'.----',
'2':'..---',
'3':'...--',
'4':'....-',
'5':'.....',
'6':'-....',
'7':'--...',
'8':'---..',
'9':'----.',
'0':'-----',
', ':'--..--',
'.':'.-.-.-',
'?':'..--..',
'/':'-..-.',
'-':'-....-',
'(':'-.--.',
')':'-.--.-',
'!': '-.-.--',
':': '---...',
';': '-.-.-.',
'=': '-...-',
'+': '.-.-.',
'@': '.--.-.'}
def textToMorse(message):
"""
This function translates a text to morse code
:param message: string containing text
:return: string containing the message in morse
"""
if len(message) < 1: # message is empty
return ""
# First, convert the message to lower case
message = message.lower()
result = [] # here we will store the encrypted message
# Iterate through each char
for c in message:
if c != ' ':
if c in MORSE_CODE_ALPHABET:
result.append(MORSE_CODE_ALPHABET[c])
return result
def beep(duration):
"""
This function plays a beep
:return: None
"""
frequency = 550
winsound.Beep(frequency, duration)
def sleep(duration):
"""
This function pauses the code. Used to pause the sound between beeps
:param duration: integer containing the duration in ms
:return:
"""
time.sleep(duration/1000)
def playMorseCode(morse_array):
"""
This function received a string containing a morse code and plays it
:param message: list containing the morse-code of each letter
:return: None
"""
N = len(morse_array)
if N < 1:
return None
for letter in morse_array:
for c in letter: # iterate through the dots and dash of each letter
if c == '.': # char is dot
beep(60)
sleep(100)
elif c == '-': # char is dash
beep(300)
sleep(100)
sleep(700) # sleep 700 ms between each word
if __name__ == '__main__':
morse = textToMorse("Hello, this is a test for the morse code!")
print(morse)
playMorseCode(morse)
Similar Samples
Explore our high-quality programming assignments and solutions, crafted by experts. Each sample showcases our dedication to helping you excel in your coding journey, offering clarity and understanding in various programming languages and concepts.
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python