Instructions
Objective
Write an x86 assembly language assignment that converts an integer from -100 to 100 to text.
Screenshots of output
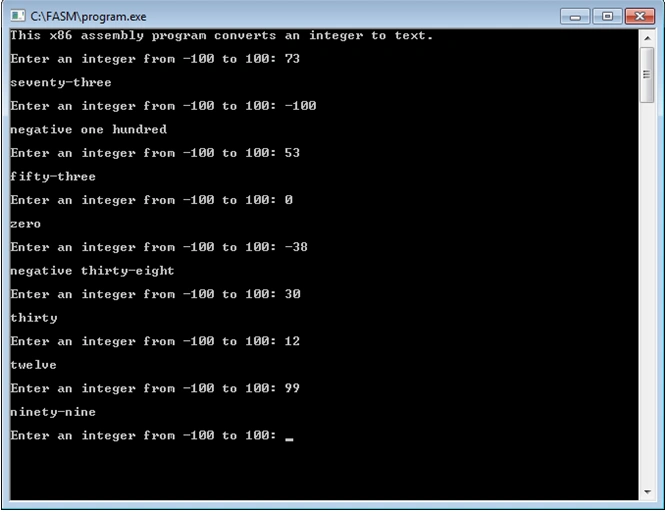
Source Code
format PE console
entry _start
include 'win32ax.inc'
Number DD 0
Quotient DD 0
Remainder DD 0
section '.text' code readable executable
_start:
push ebp
mov ebp, esp
cinvoke printf, "This x86 assembly program converts an integer to text.%c%c%c%c", 10, 13, 10, 13
readLoop:
cinvoke printf, "Enter an integer from -100 to 100: "
cinvoke scanf, "%d", Number ; read the integer
call [getchar] ; read enter from scanf
cinvoke printf, "%c%c", 10, 13 ; jump to next line
cmp DWORD[Number], -100 ; check if number is in range
jl endProgram ; if not, end program
cmp DWORD[Number], 100 ; check if number is in range
jg endProgram ; if not, end program
translate:
cmp DWORD[Number], 0 ; check if it's a negative number
jge positive ; if not, print positive
cinvoke printf, "negative " ; else, print number is negative
neg DWORD[Number] ; convert number to positive
positive:
mov eax, [Number] ; load number in eax
cmp eax, 100 ; if 100
jne get2digits ; if not, print 2 digits
cinvoke printf, "one hundred" ; else, print hundred
jmp ending
get2digits:
mov ebx, 10 ; load 10 to make division
mov edx, 0 ; clear edx before division
idiv ebx ; divide number by 10
mov [Quotient], eax ; save quotient
mov [Remainder], edx ; save remainder
cmp DwORD[Quotient], 0 ; if quotient 0
je Single
cmp DwORD[Quotient], 1 ; if quotient 1
je Tens
cmp DwORD[Quotient], 1 ; if quotient 1
je Tens
cmp DwORD[Quotient], 2 ; if quotient 2
je Twenty
cmp DwORD[Quotient], 3 ; if quotient 3
je Thirty
cmp DwORD[Quotient], 4 ; if quotient 4
je Forty
cmp DwORD[Quotient], 5 ; if quotient 5
je Fifty
cmp DwORD[Quotient], 6 ; if quotient 6
je Sixty
cmp DwORD[Quotient], 7 ; if quotient 7
je Seventy
cmp DwORD[Quotient], 8 ; if quotient 8
je Eighty
cmp DwORD[Quotient], 9 ; if quotient 9
je Ninety
Twenty:
cinvoke printf, "twenty"
jmp OnesDigit
Thirty:
cinvoke printf, "thirty"
jmp OnesDigit
Forty:
cinvoke printf, "forty"
jmp OnesDigit
Fifty:
cinvoke printf, "fifty"
jmp OnesDigit
Sixty:
cinvoke printf, "sixty"
jmp OnesDigit
Seventy:
cinvoke printf, "seventy"
jmp OnesDigit
Eighty:
cinvoke printf, "eighty"
jmp OnesDigit
Ninety:
cinvoke printf, "ninety"
OnesDigit:
cmp DwORD[Remainder], 0 ; if remainder 0 in 2 digit numbers
je ending ; don't print more
cinvoke printf, "-" ; else, print dash
Single:
cmp DwORD[Remainder], 0 ; if remainder 0
je Zero
cmp DwORD[Remainder], 1 ; if remainder 1
je One
cmp DwORD[Remainder], 2 ; if remainder 2
je Two
cmp DwORD[Remainder], 3 ; if remainder 3
je Three
cmp DwORD[Remainder], 4 ; if remainder 4
je Four
cmp DwORD[Remainder], 5 ; if remainder 5
je Five
cmp DwORD[Remainder], 6 ; if remainder 6
je Six
cmp DwORD[Remainder], 7 ; if remainder 7
je Seven
cmp DwORD[Remainder], 8 ; if remainder 8
je Eight
cmp DwORD[Remainder], 9 ; if remainder 9
je Nine
Zero:
cinvoke printf, "zero"
jmp ending
One:
cinvoke printf, "one"
jmp ending
Two:
cinvoke printf, "two"
jmp ending
Three:
cinvoke printf, "three"
jmp ending
Four:
cinvoke printf, "four"
jmp ending
Five:
cinvoke printf, "five"
jmp ending
Six:
cinvoke printf, "six"
jmp ending
Seven:
cinvoke printf, "seven"
jmp ending
Eight:
cinvoke printf, "eight"
jmp ending
Nine:
cinvoke printf, "nine"
jmp ending
Tens:
cmp DwORD[Remainder], 0 ; if remainder 0
je Ten
cmp DwORD[Remainder], 1 ; if remainder 1
je Eleven
cmp DwORD[Remainder], 2 ; if remainder 2
je Twelve
cmp DwORD[Remainder], 3 ; if remainder 3
je Thirteen
cmp DwORD[Remainder], 4 ; if remainder 4
je Fourteen
cmp DwORD[Remainder], 5 ; if remainder 5
je Fifteen
cmp DwORD[Remainder], 6 ; if remainder 6
je Sixteen
cmp DwORD[Remainder], 7 ; if remainder 7
je Seventeen
cmp DwORD[Remainder], 8 ; if remainder 8
je Eighteen
cmp DwORD[Remainder], 9 ; if remainder 9
je Nineteen
Ten:
cinvoke printf, "ten"
jmp ending
Eleven:
cinvoke printf, "eleven"
jmp ending
Twelve:
cinvoke printf, "twelve"
jmp ending
Thirteen:
cinvoke printf, "thirteen"
jmp ending
Fourteen:
cinvoke printf, "fourteen"
jmp ending
Fifteen:
cinvoke printf, "fifteen"
jmp ending
Sixteen:
cinvoke printf, "sixteen"
jmp ending
Seventeen:
cinvoke printf, "seventeen"
jmp ending
Eighteen:
cinvoke printf, "eighteen"
jmp ending
Nineteen:
cinvoke printf, "nineteen"
ending:
cinvoke printf, "%c%c%c%c", 10, 13, 10, 13 ; leave empty line
jmp readLoop ; read another number
endProgram:
call [getchar] ; wait until user presses a key
mov esp, ebp
pop ebp
call [ExitProcess] ; exit the program
; Import section
section '.idata' import data readable
library kernel32, 'kernel32.dll', \
msvcrt,'msvcrt.dll'
import kernel32, \
import msvcrt, \
printf, 'printf', scanf, 'scanf', \
getchar, 'getchar'
Related Samples
Dive into our Assembly Language Assignments samples for comprehensive solutions crafted to aid students. Navigate intricate concepts from basic instructions to complex system calls with clarity. Explore concise, annotated code snippets that unravel the nuances of Assembly Language, empowering students to master low-level programming effortlessly.
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language