Instructions
ObjectiveWrite an Assembly language assignment program to shift string by n bits.
Requirements and Specifications
ObjectiveFor this programming assignment, you will write an ARM program that does the following:
- Prints "input a string".
- Reads the input string.
- Calculates the length of the string.
- Outputs the string length to the console.
- Determines if the string is a palindrome.
- Outputs whether the string is a palindrome
Screenshots of output
.webp)
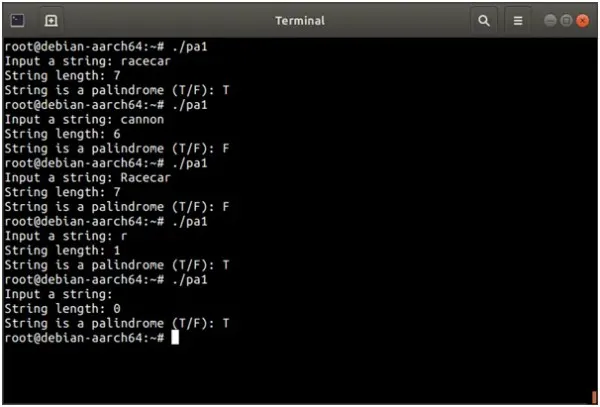
Source Code
.section .data
input_prompt : .asciz "Input a string: "
input_spec : .asciz "%[^\n]"
length_spec : .asciz "String length: %d\n"
palindrome_spec : .asciz "String is a palindrome (T/F): %c\n"
input: .space 10 // place to save string
.section .text
.global main
# program execution begins here
main:
ldr x0, =input_prompt // load address of prompt string
bl printf // print the prompt
ldr x0, =input_spec // load address of spec to read a string
ldr x1, =input // load address of place to save string
bl scanf // read the string
# calculate length
ldr x0, =input // load input string address
mov x19, 0 // start length in zero
loop1:
ldrb w1, [x0, x19] // load character from string
cmp w1, 0 // if the character is end of string
beq endloop1 // terminate loop
add x19, x19, 1 // else increment string length
b loop1 // repeat loop
endloop1:
# print length
ldr x0, =length_spec // load address of string to print
mov w1, w19 // copy length to w1
bl printf // print the length message
# if length is <= 1, string is palindrome
cmp x19, 1
ble palindrome
# else, check if string is palindrome
ldr x0, =input // load input string address
mov w1, 'F' // by defaulf we assume string is not a palindrome
mov x2, 0 // index from start of string (0)
sub x3, x19, 1 // index from end of string (length - 1)
loop2:
ldrb w4, [x0, x2] // load character from start of string
ldrb w5, [x0, x3] // load character from end of string
cmp w4, w5 // if the characters are not equal
bne printtf // print the result, not palindrome
add x2, x2, 1 // advance start index to next char in string
add x3, x3, -1 // move end index to previous char in string
cmp x2, x3 // compare indices
blt loop2 // continue in loop if indices don't cross
// if indices cross, all other chars in front and back
// were equal so it's a palindrome
palindrome:
mov w1, 'T' // is a palindrome
printtf:
# print palindrome result T or F
ldr x0, =palindrome_spec // load address of string to print
bl printf // print the palindrome message
exit:
mov x0, 0
mov x8, 93
svc 0
ret
Related Samples
At ProgrammingHomeworkHelp.com, we offer comprehensive assignment support to students, including detailed samples of Assembly Language projects. Our expertly crafted samples demonstrate best practices and provide insights into efficient coding techniques, helping students understand complex concepts and improve their programming skills. Whether you're tackling basic Assembly tasks or advanced projects, our resources are designed to guide you through every step of your assignment. Trust ProgrammingHomeworkHelp.com to be your partner in mastering Assembly Language and achieving academic success.
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language