Instructions
Objective
Write a java assignment program to calculate using math functions in java language.
Requirements and Specifications
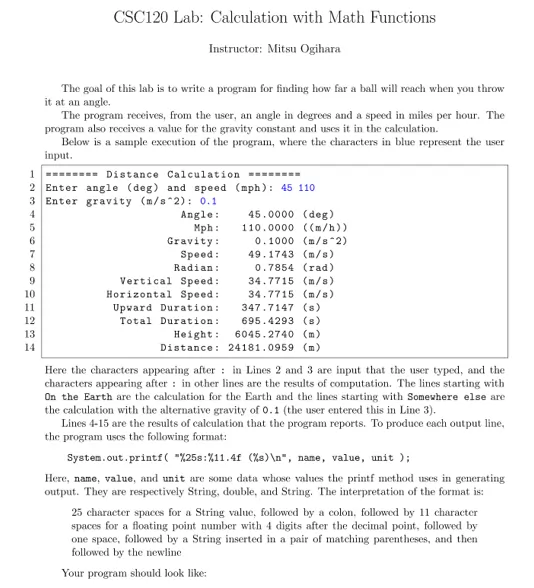
Source Code
import java.util.Scanner;
public class App {
public static void main(String[] args) throws Exception {
// Create scanner
Scanner sc = new Scanner(System.in);
// Create variables to hold inputs
double angle;
double speed;
double g;
// Create variables to hold calculations
double speed_mps;
double angle_rad;
double speed_y;
double speed_x;
double upward_t;
double total_t;
double height;
double distance;
// Display header
System.out.println("========= Distance Calculation ========");
// Ask user for angle and speed
System.out.print("Enter angle (deg) and speed (mph): ");
angle = sc.nextDouble();
speed = sc.nextDouble();
// Ask for gravity
System.out.print("Enter gravity (m/s^2): ");
g = sc.nextDouble();
// Now, do calculations
// Let's be sure that the angle is between 0.0 and 90.0
angle = Math.max(0.0, angle);
angle = Math.min(angle, 90.0);
speed_mps = speed*1609.34/3600;
angle_rad = angle*Math.PI/180;
speed_y = speed_mps*Math.sin(angle_rad);
speed_x = speed_mps*Math.cos(angle_rad);
upward_t = speed_y/g;
total_t = 2*upward_t;
height = speed_y*upward_t - 0.5*g*Math.pow(upward_t, 2.0);
distance = speed_x*total_t;
// Now, print
System.out.printf("%25s:%11.4f (%s)\n", "Angle", angle, "deg");
System.out.printf("%25s:%11.4f (%s)\n", "Mph", speed, "m/h");
System.out.printf("%25s:%11.4f (%s)\n", "Gravity", g, "m/s^2");
System.out.printf("%25s:%11.4f (%s)\n", "Speed", speed_mps, "m/s");
System.out.printf("%25s:%11.4f (%s)\n", "Radian", angle_rad, "rad");
System.out.printf("%25s:%11.4f (%s)\n", "Vertical Speed", speed_y, "m/s");
System.out.printf("%25s:%11.4f (%s)\n", "Horizontal Speed", speed_x, "m/s");
System.out.printf("%25s:%11.4f (%s)\n", "Upward Duration", upward_t, "s");
System.out.printf("%25s:%11.4f (%s)\n", "Total Duration", total_t, "s");
System.out.printf("%25s:%11.4f (%s)\n", "Height", height, "m");
System.out.printf("%25s:%11.4f (%s)\n", "Distance", distance, "m");
sc.close();
}
}
Related Samples
Explore our collection of free Java assignment samples. These samples showcase practical applications and solutions in Java programming, offering insights and examples to support your learning and development in Java programming.
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java
Java