Instructions
Requirements and Specifications
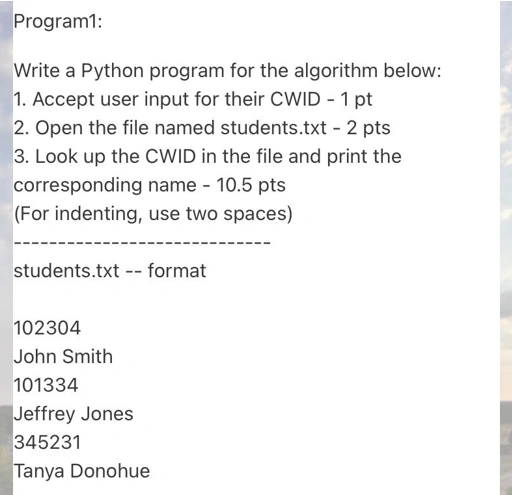
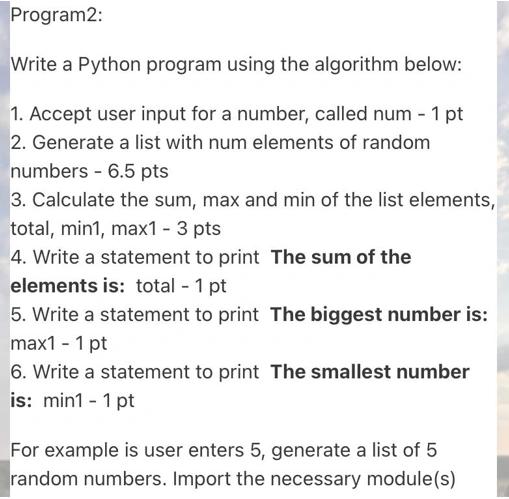
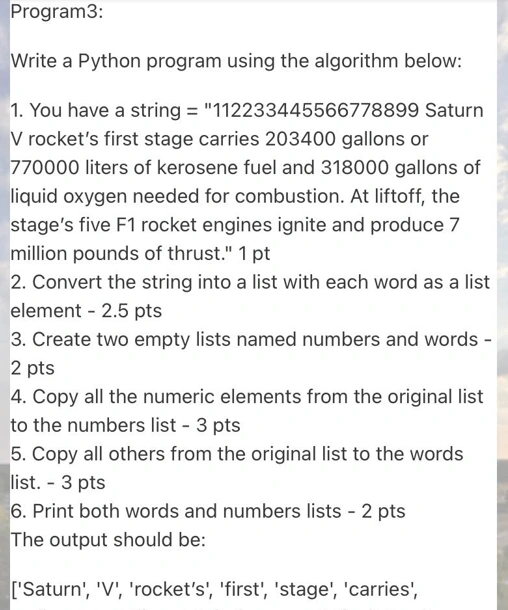
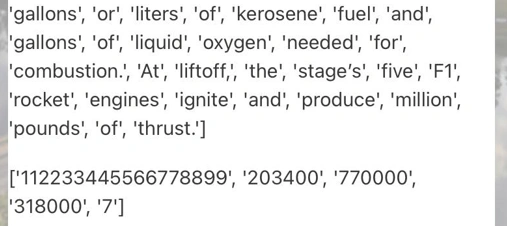
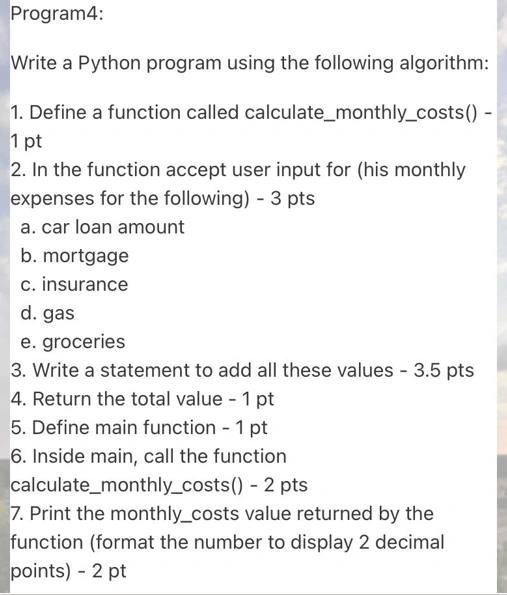
Source Code
PROGRAM 1
cwid = input('Please, enter CWID: ')
f = open('students.txt', 'r')
lines = f.read().splitlines()
line_counter = 0
found_name = None
while line_counter < len(lines):
if lines[line_counter] == cwid:
found_name = lines[line_counter + 1]
break
line_counter += 2
if found_name:
print(found_name)
PROGRAM 2
import random
num = int(input('Please, enter the number: '))
rand_list = []
for i in range(num):
rand_list.append(random.randint(0, 1000))
total = 0
min1 = None
max1 = None
for a in rand_list:
total += a
if not min1 or min1 > a:
min1 = a
if not max1 or max1 < a:
max1 = a
print('The sum of the elements is:', total)
print('The biggest number is:', max1)
print('The smallest number is:', min1)
PROGRAM 3
s = "112233445566778899 Saturn V rocket's first stage carries 203400 gallons or 770000 liters of kerosene fuel and " \
"318000 gallons of liquid oxygen needed for combustion. At liftoff, the stage's five F1 rocket engines ignite and " \
"produce 7 million pounds of thrust. "
tokens = s.split()
numbers = []
words = []
for token in tokens:
if token.isnumeric():
numbers.append(token)
else:
words.append(token)
print(words, '\n')
print(numbers)
PROGRAM 4
def calculate_monthly_costs(car_loan, mortgage, insurance, gas, groceries):
total = car_loan + mortgage + insurance + gas + groceries
return total
def main():
car_loan = 50.0
mortgage = 40.0
insurance = 20.0
gas = 30.0
groceries = 10.0
monthly_cost = calculate_monthly_costs(car_loan, mortgage, insurance, gas, groceries)
print('{:.2f}'.format(monthly_cost))
main()
Related Samples
Discover our Python Assignments samples showcasing foundational topics like loops, functions, data structures, and OOP concepts. Dive into annotated code examples covering practical applications in data manipulation, web scraping, and more. Enhance your Python programming skills with curated assignments designed to accelerate your learning and academic success.</
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python