Instructions
Objective
Write a program to calculate drivers fine in python language.
Requirements and Specifications
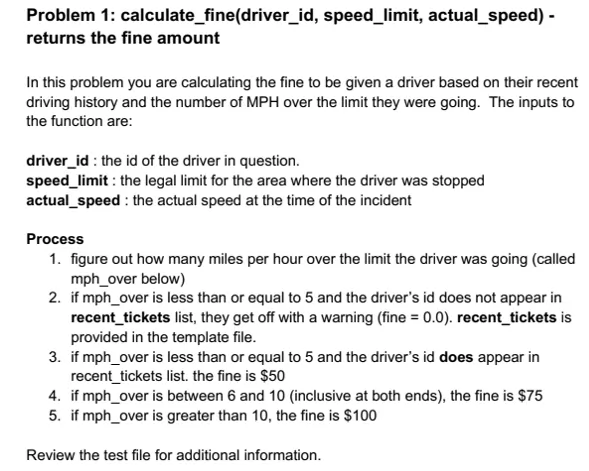
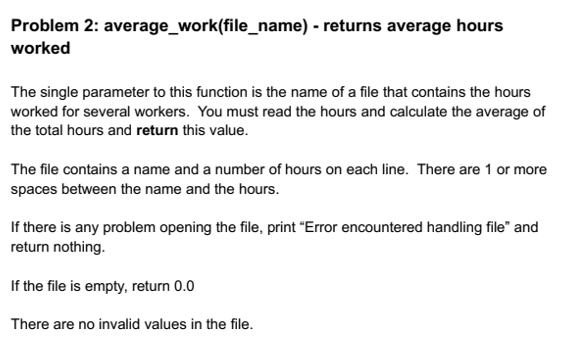
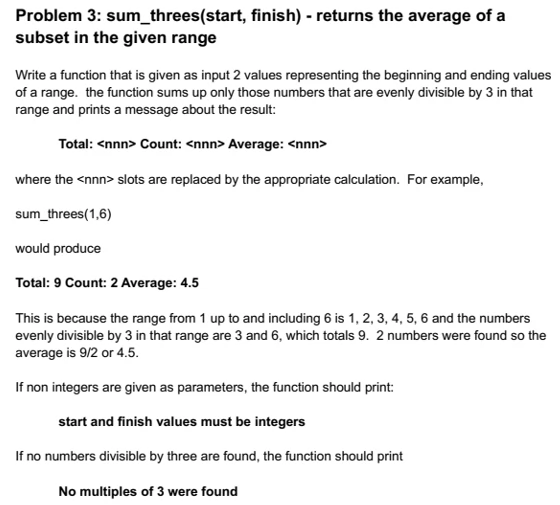
Source Code
# CSC-401 Midterm
# Due: July 2, 2021 11:59 PM
# Author:
#
# Don't forget to delete 'pass' from each function
# Don't forget function descriptions
### WARNING: you must not add any imports ###
def calculate_fine(driver_id, speed_limit, actual_speed):
'''
This function calculate a driver's fine if it's speed is over the limit
The fine is calculated depending of the amount of mph over the limit
'''
recent_tickets = ['1234', '0101','2222'] # has a ticket in the last year
# If the actual speed is lower than the speed limit, then there is no fine
if actual_speed <= speed_limit:
return 0
# Calculate the number of mph over the limit
mph_over = actual_speed - speed_limit
fine = 0
if mph_over <= 5 and driver_id not in recent_tickets: # only warning
fine = 0
elif mph_over <= 5 and driver_id in recent_tickets:
fine = 50
elif mph_over >= 6 and mph_over <= 10:
fine = 75
elif mph_over > 10:
fine = 100
return fine
def average_work(file_name):
'''
This function calculates the average amount of the number of hours reportd by workers
The name and number of hours for each worker is inside a file given by file_name
'''
# Read file
total_hours = 0.0
n_workers = 0.0
try:
f = open(file_name, 'r')
# read lines
lines = f.readlines()
for line in lines:
# Split line by space
data = line.split(" ")
name = data[0]
hours = int(data[1])
total_hours += hours
n_workers += 1.0
# calculate average of there is at least one worker
avg_work = total_hours
if n_workers > 0:
avg_work = total_hours / n_workers
return avg_work
except Exception:
print("Error encountered handling file")
def sum_threes(start, finish):
'''
This function calculates the sum of all numbers divisible by 3 in the range [start, finish] (inclusive)
'''
# Check that both start and finish are numeric
if isinstance(start, str) or isinstance(finish, str):
print("start and finish values must be integers")
return
# Check if both start and finish are integers
if not isinstance(start, int) or not isinstance(finish, int):
print("start and finish values must be integers")
return
n_threes = 0 # variable to count the amount of numbers divisible by 3
sum_threes = 0 # variable to store the sum of threes
avg_threes = 0 # variable to store the avg "" ""
for n in range(start, finish + 1):
if n % 3 == 0: # divisible by 3
n_threes += 1
sum_threes += n
# Calculate average
if n_threes > 0:
avg_threes = sum_threes / n_threes
# Print
print("Total: {} Count: {} Average: {:.1f}".format(sum_threes, n_threes, avg_threes))
else:
print("No multiples of 3 were found")
def switch(file_name):
'''
This function will keep prompting the user for a string (until he/she enters an empty line)
and then that string is split in half. Then, the function will write to a file (given by file_name) the string
but with the two halfs switched
Example: input: 1234
output: 34 | 12
'''
# Create a loop to keep user entering a string
while True:
string = input("Enter text: ")
if len(string) > 0: # Not empty
# Get length of string
N = len(string)
# Chop
left = string[:N // 2]
right = string[N // 2:]
# Open file
f = open(file_name, 'a+')
f.write(right + " | " + left + "\n")
f.close()
else: # empty string, break loop
break
# The following code is for Part 5
def f(x):
'''
return the count of all of the event numbers from 0 upto and including x
'''
cnt = 0
for i in range(0, x+1): # The first error was here. x is an integer not a range.
if i%2 == 0:
cnt += 1
# return cnt -> The second error was here. This line is now disabled (commented). The function was returning the value of cnt after the first even number
if cnt > 10:
print("more than 10 where found") # Third error was here. The formatted string was not written correctly
return cnt
'''
-------------------------------------------------------
The first error as in the for-loop. That for loop was handling the parameter x as a list or range
but the parameter x is actually an integer. The second error was that inside the loop, after
the first even number, the variable cnt was returned so it would only return 1 if there were 1 or more
even numbers from 0 to x. The third error was in the print function. The formatted string was wrong.
-------------------------------------------------------
'''
# --------------------------------------------
# STOP! Do not change anything below this line
# The code below makes this file 'self-testing'
# --------------------------------------------
def show_file(file_name):
with open(file_name, 'r') as result_file:
print(result_file.read())
if __name__ == "__main__":
# creates an empty file used in a test
empty_file = open('no_work.txt','w')
empty_file.close()
import doctest
doctest.testfile('csc401_sum2021_midterm_test.txt', verbose = True)
Related Samples
Explore our Python programming homework samples for a glimpse into our expertise. Our curated examples showcase comprehensive solutions to Python assignments, ensuring clarity and depth in coding concepts. See firsthand how our expert team tackles challenges, delivering top-notch assistance tailored to your academic needs.
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python