Instructions
Objective
Write a python homework to calculate average and standard deviation of image in python language.
Requirements and Specifications
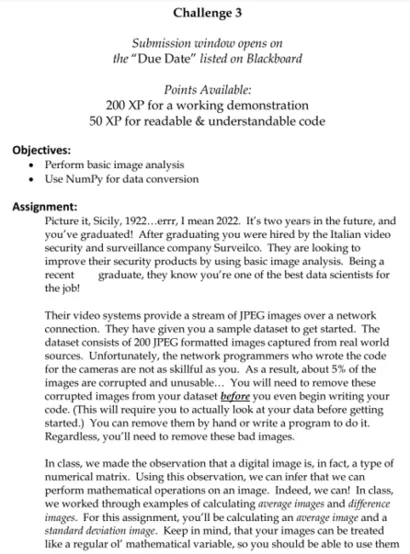
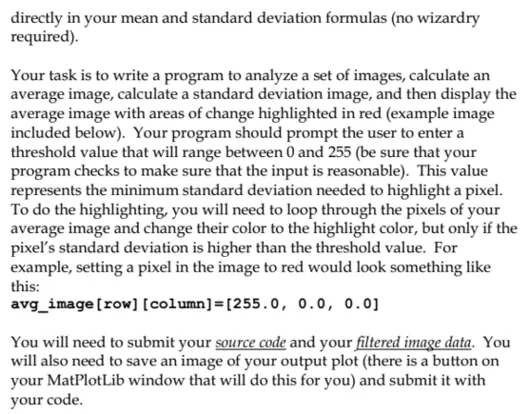
Source Code
import matplotlib.pyplot as mplot
import numpy as np
#import image
#might get error so use import below
from PIL import Image
import matplotlib.pyplot as plt
import os
files=os.listdir("./data")
N_images = len(files)
# Load the first image and get its size
img = np.array(Image.open("./data/" + files[0])).astype('float')
(h,w,layers) = img.shape
avg_image = np.zeros(img.shape).astype('float')
print("Loading images... ", end = "")
for filename in files:
img_dir = "./data/" + filename
img=np.array(Image.open("./data/"+filename))
avg_image += img
print(f"done. A total of {N_images} images loaded.")
# Calculate average image
print("Calculating average image... ", end="")
avg_image = avg_image / float(N_images)
print("done.")
# Calculate standard deviation image
# Create an array with the same size of the avg image
print("Calculating std image... ", end="")
std_image = np.zeros(avg_image.shape).astype('float')
# Now calculate the std of each pixel for all images
for filename in files:
img_dir = "./data/" + filename
img=np.array(Image.open("./data/"+filename))
std_image += (np.square(img - avg_image) / float(N_images))
std_image = np.sqrt(std_image)
print("done.")
std_image2 = avg_image.std()
# Ask user for treshold
while True:
threshold = input("Enter STD treshold: ")
if threshold.isnumeric():
threshold = float(threshold)
if threshold >= 0.0 and threshold <= 255.0:
break
else:
print("Please enter a value between 0-255")
else:
print("Please enter a numeric treshold value.")
# Now, highlight areas in avg_image
avg_image_highlighted = avg_image.copy()
for row in range(h):
for col in range(w):
if std_image[row][col].mean() > threshold: # if pixel's std is higher than treshold
avg_image_highlighted[row][col] = [255.0, 0.0, 0.0]
fig, axes = plt.subplots(nrows = 1, ncols = 2)
axes[0].imshow(avg_image.astype(np.uint8))
axes[0].set_title('Average Image')
axes[1].imshow(avg_image_highlighted.astype(np.uint8))
axes[1].set_title('Average Image Highlighted')
plt.show()
Related Samples
Access our free Python assignment samples to enhance your coding skills and knowledge. Explore a variety of projects and examples that cover fundamental to advanced topics in Python programming. Perfect for students and enthusiasts looking to learn and improve.
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python