Instructions
Objective
Write an Assembly language assignment to add variables of different sizes.
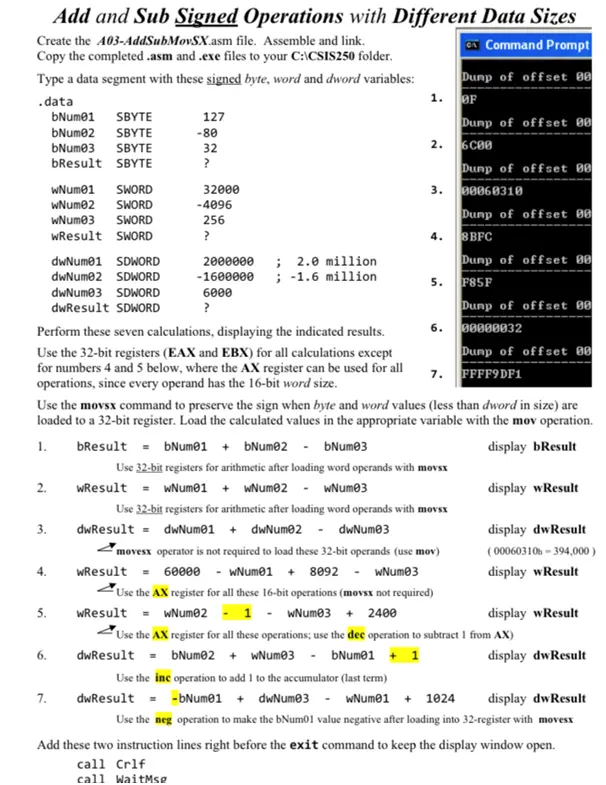
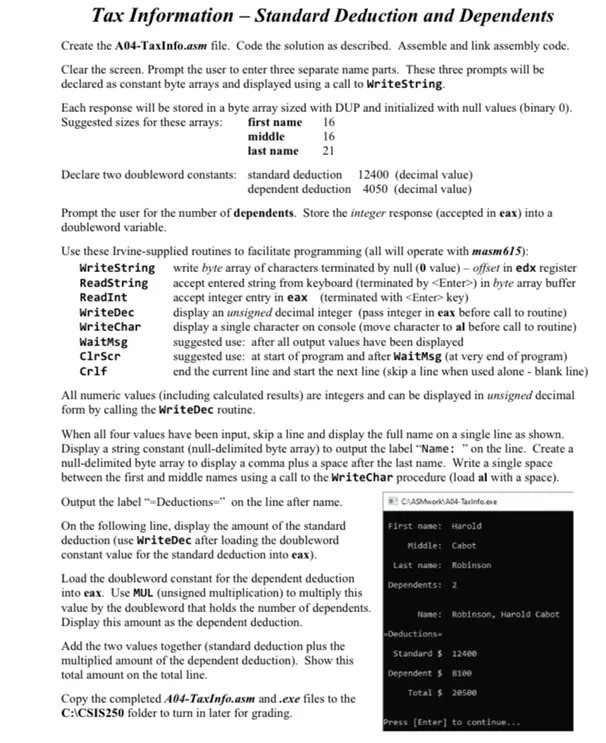
Screenshots of output
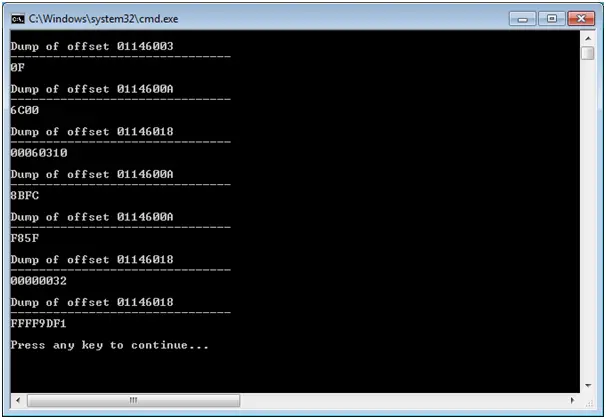
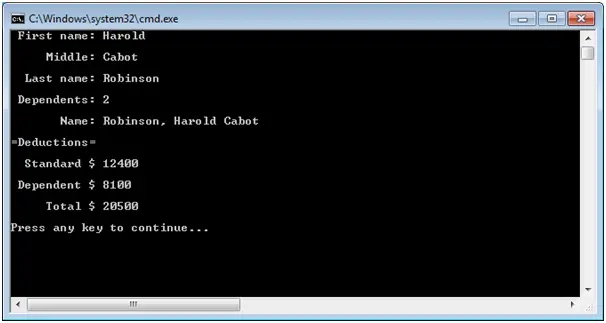
Source Code
Add sub movies
INCLUDE Irvine32.inc
.data
bNum01 SBYTE 127
bNum02 SBYTE -80
bNum03 SBYTE 32
bResult SBYTE ?
wNum01 SWORD 32000
wNum02 SWORD -4096
wNum03 SWORD 256
wResult SWORD ?
dwNum01 SDWORD 2000000 ; 2million
dwNum02 SDWORD -1600000 ; -1.6million
dwNum03 SDWORD 6000
dwResult SDWORD ?
.code
main PROC
movsx eax, [bNum01] ; load first byte value, extend to dword
movsx ebx, [bNum02] ; load second byte value, extend to dword
add eax, ebx ; add second to first
movsx ebx, [bNum03] ; load third byte value, extend to dword
sub eax, ebx ; subtract third from sum
mov [bResult],al ; save result as byte
mov esi, OFFSET bResult ; starting offset of result
mov ecx, 1 ; number of units
mov ebx, TYPE BYTE ; byte format
call DumpMem ; display result
movsx eax, [wNum01] ; load first word value, extend to dword
movsx ebx, [wNum02] ; load second word value, extend to dword
add eax, ebx ; add second to first
movsx ebx, [wNum03] ; load third word value, extend to dword
sub eax, ebx ; subtract third from sum
mov [wResult],ax ; save result as word
mov esi, OFFSET wResult ; starting offset of result
mov ecx, 1 ; number of units
mov ebx, TYPE WORD ; word format
call DumpMem ; display result
mov eax, [dwNum01] ; load first dword value
add eax, [dwNum02] ; add second dword value
sub eax, [dwNum03] ; subtract third from sum
mov [dwResult],eax ; save result as dword
mov esi, OFFSET dwResult ; starting offset of result
mov ecx, 1 ; number of units
mov ebx, TYPE DWORD ; dword format
call DumpMem ; display result
mov ax, 60000 ; load 60000
sub ax, [wNum01] ; subtract 60000 - first word
add ax, 8092 ; add 8092 to difference
sub ax, [wNum03] ; subtract third word from previous result
mov [wResult],ax ; save result as word
mov esi, OFFSET wResult ; starting offset of result
mov ecx, 1 ; number of units
mov ebx, TYPE WORD ; word format
call DumpMem ; display result
mov ax, [wNum02] ; load second word value
dec ax ; subtract 1 from second
sub ax, [wNum03] ; subtract third word from previous result
add ax, 2400 ; add 2400 to previous result
mov [wResult],ax ; save result as word
mov esi, OFFSET wResult ; starting offset of result
mov ecx, 1 ; number of units
mov ebx, TYPE WORD ; word format
call DumpMem ; display result
movsx eax, [bNum02] ; load second byte value, extend to dword
movsx ebx, [wNum03] ; load third word value, extend to dword
add eax, ebx ; add second byte to third word
movsx ebx, [bNum01] ; load first byte value, extend to dword
sub eax, ebx ; subtract first byte from previous result
inc eax ; add 1 to result
mov [dwResult],eax ; save result as dword
mov esi, OFFSET dwResult ; starting offset of result
mov ecx, 1 ; number of units
mov ebx, TYPE DWORD ; dword format
call DumpMem ; display result
movsx eax, [bNum01] ; load first byte value, extend to dword
neg eax ; convert to negative
add eax, [dwNum03] ; add third dword value
movsx ebx, [wNum01] ; load first word value, extend to dword
sub eax, ebx ; subtract first word from previous result
add eax, 1024 ; add 1024 to result
mov [dwResult],eax ; save result as dword
mov esi, OFFSET dwResult ; starting offset of result
mov ecx, 1 ; number of units
mov ebx, TYPE DWORD ; dword format
call DumpMem ; display result
call CrLf
call WaitMsg
exit ; terminate the program
main ENDP
END main
Tax infos
INCLUDE Irvine32.inc
.data
promptFirst BYTE ' First name: ', 0
promptMiddle BYTE ' Middle: ', 0
promptLast BYTE ' Last name: ', 0
promptDepend BYTE ' Dependents: ', 0
printName BYTE ' Name: ', 0
printDeduct BYTE '=Deductions= ', 0
printStd BYTE ' Standard $ ', 0
printDep BYTE ' Dependent $ ', 0
printTotal BYTE ' Total $ ', 0
comma BYTE ', ', 0
firstName BYTE 16 DUP(0)
middleName BYTE 16 DUP(0)
lastName BYTE 21 DUP(0)
stdDeduct DWORD 12400
depDeduct DWORD 4050
total DWORD ?
dependents DWORD ?
.code
main PROC
call ClrScr ; clear the screen
mov edx, OFFSET promptFirst ; load address of prompt
call WriteString ; print prompt on screen
mov edx, OFFSET firstName ; load address of name array
mov ecx, 16 ; size of array
call ReadString ; read the string and save as first name
call CrLf ; leave empty line
mov edx, OFFSET promptMiddle ; load address of prompt
call WriteString ; print prompt on screen
mov edx, OFFSET middleName ; load address of middle array
mov ecx, 16 ; size of array
call ReadString ; read the string and save in middle name
call CrLf ; leave empty line
mov edx, OFFSET promptLast ; load address of prompt
call WriteString ; print prompt on screen
mov edx, OFFSET lastName ; load address of name array
mov ecx, 16 ; size of array
call ReadString ; read the string and save as last name
call CrLf ; leave empty line
mov edx, OFFSET promptDepend ; load address of prompt
call WriteString ; print prompt on screen
call ReadDec ; read dependents
mov [dependents],eax ; save dependents in variable
call CrLf ; leave empty line
mov edx, OFFSET printName ; load address of name message
call WriteString ; print message for name on screen
mov edx, OFFSET lastName ; load address of last name
call WriteString ; print last name on screen
mov edx, OFFSET comma ; load address comma string
call WriteString ; print separating comma on screen
mov edx, OFFSET firstName ; load address of first name
call WriteString ; print first name on screen
mov al, ' ' ; load space character in al
call WriteChar ; print space on screen
mov edx, OFFSET middleName ; load address of middle name
call WriteString ; print middle name on screen
call CrLf ; jump to next line
call CrLf ; leave empty line
mov edx, OFFSET printDeduct ; load address of deductions message
call WriteString ; print message for deductions on screen
call CrLf ; jump to next line
call CrLf ; leave empty line
mov edx, OFFSET printStd ; load address of standard message
call WriteString ; print message for standard on screen
mov eax, [stdDeduct] ; load standard deduction amount
call WriteDec ; print standard deduction amount on screen
call CrLf ; jump to next line
call CrLf ; leave empty line
mov edx, OFFSET printDep ; load address of dependent message
call WriteString ; print message for dependent on screen
mov eax, [depDeduct] ; load dependent deduction amount
mul [dependents] ; multiply by number of dependents
mov [total], eax ; save multiplication result in total
call WriteDec ; print dependent deduction amount on screen
call CrLf ; jump to next line
call CrLf ; leave empty line
mov edx, OFFSET printTotal ; load address of total message
call WriteString ; print message for total on screen
mov eax, [stdDeduct] ; load standard deduction
add eax, [total] ; add to dependent total
call WriteDec ; print total deduction amount on screen
call CrLf ; jump to next line
call CrLf
call WaitMsg
exit ; terminate program
main ENDP
END main
Related Samples
Access our free assembly language assignment samples to enhance your understanding and skills. Explore a variety of examples, detailed explanations, and practical applications to assist you in mastering assembly language programming. Get started now and improve your coding proficiency!
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language