Instructions
Objective
Write a program that will implement file and string parsing in C language.
Requirements and Specifications
Assignment Overview
OVERVIEW
Write a C assignment program to generate sport team win-loss records from game results in files.
NOTE: This is a harder assignment than the previous major assignments.
OBJECTIVES
- Use file I/O (text mode).
- Use C-style strings and string functions.
- Use best practices for magic numbers.
- Design computer programs for modularity and maintainability.
- Demonstrate the development cycle for C programs.
- Follow stated requirements.
ACADEMIC INTEGRITY AND LATE PENALTIES
- Link to Academic Integrity Information
- Link to Late Policy
Screenshots
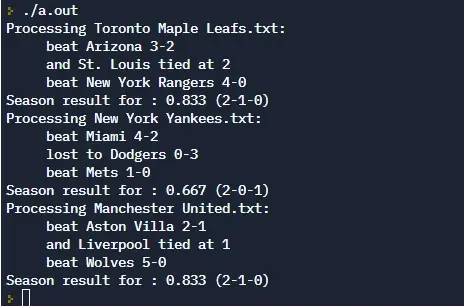
Source Code
#include
#include
#include
#define TEAMS_FILENAME "teams.txt"
int isBlank(char* line) {
int len = strlen(line), i;
for (i = 0; i
if (line[i] != ' ') {
return 0;
}
}
return 1;
}
int parseLine(char* line, char* opponent, int* score, int* oppScore) {
int len = strlen(line);
int bufferSize = 255;
char resultStr[bufferSize];
char scoreStr[bufferSize];
char oppScoreStr[bufferSize];
char* delPtr = strchr(line, ',');
if (delPtr == NULL) {
return 0;
}
int index = delPtr - line;
strncpy(opponent, line, index);
opponent[index] = 0;
memcpy(resultStr, delPtr + 1, len - (index + 1));
resultStr[len - index - 1] = 0;
len = strlen(resultStr);
delPtr = strchr(resultStr, '-');
if (delPtr == NULL) {
return 0;
}
index = delPtr - resultStr;
strncpy(scoreStr, resultStr, index);
scoreStr[index] = 0;
*score = atoi(scoreStr);
memcpy(oppScoreStr, delPtr + 1, len - (index + 1));
oppScoreStr[len - index - 1] = 0;
*oppScore = atoi(oppScoreStr);
return 1;
}
int processGames(char* filename) {
FILE *f = fopen(filename, "r");
int bufferSize = 255;
char line[bufferSize];
char name[bufferSize];
char opp[bufferSize];
int wins = 0, ties = 0, losses = 0, score, oppScore;
double percentage;
if (!f) {
return 0;
}
printf("Processing %s:\n", filename);
while(fgets(line, bufferSize, f)) {
line[strlen(line) - 1] = 0;
if (isBlank(line)) {
continue;
}
if (parseLine(line, opp, &score, &oppScore)) {
if (score > oppScore) {
printf("\t%s beat %s %d-%d\n", name, opp, score, oppScore);
wins++;
}
else if (oppScore > score) {
printf("\t%s lost to %s %d-%d\n", name, opp, score, oppScore);
losses++;
}
else {
printf("\t%s and %s tied at %d\n", name, opp, score);
ties++;
}
}
}
if (wins + ties + losses == 0) {
return 0;
}
percentage = (2*wins + ties)/(2.0*(wins + ties + losses));
printf("Season result for %s: %.3f (%d-%d-%d)\n", name, percentage, wins, ties, losses);
fclose(f);
return 1;
}
int main(void) {
FILE *fTeams;
int bufferSize = 255;
char line[bufferSize];
fTeams = fopen(TEAMS_FILENAME, "r");
while(fgets(line, bufferSize, fTeams)) {
if (line[strlen(line) - 1] == '\n') {
line[strlen(line) - 1] = 0;
}
if (isBlank(line)) {
continue;
}
processGames(line);
}
fclose(fTeams);
return 0;
}
Similar Samples
Explore our extensive collection of programming homework samples at ProgrammingHomeworkHelp.com. Our examples cover a wide range of topics and languages, including Java, Python, C++, and more. Each sample showcases our dedication to delivering clear, well-commented solutions that meet academic standards. Dive into our samples to see how we can assist you in mastering programming concepts effectively.
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C