Instructions
Objective
Write a program to merge 2 arrays in assembly language (both arrays are sorted, merged array should be in order), using Irvine library in Assembly language.
Requirements and Specifications
Description:
Write an x86 assembler program that will merge two (2) presorted lists of unsigned numbers (up to 32-bits in size). All lists will have items sorted in ascending order. Each list will have at least one value; there is no other restriction on the size of the lists.
The input lists for this program will be declared internally in the data segment of your program. Do not prompt the user for the input. An example of this declaration is given below. Note that in the final sorted list, all items in the input lists must be present
Caveats:
- This program must include at least one procedure beyond MAIN.
- Be sure to thoroughly test this program as you will need this code for a subsequent assignment.
- In the above example, the 2nd list is larger than the first. However, when I test your solution, either list may be larger than the other.
- Just upload a zip file with the ASM source file for this program.
Screenshots of output
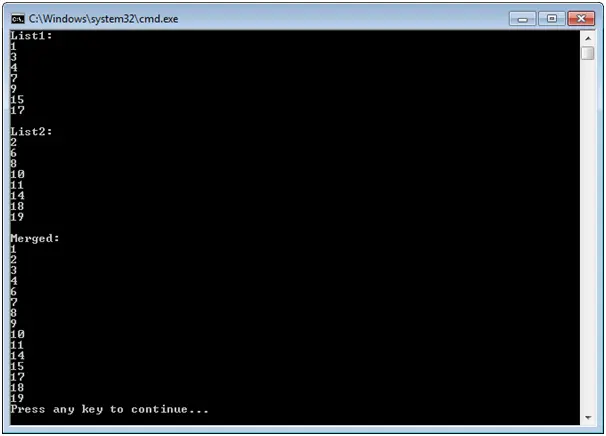
Source Code
Title ArrayMerge
include irvine32.inc
.data
a1 dword 1,3,4,7,9,15,17
a2 dword 2,6,8,10,11,14,18,19
a3 dword 20 dup(0)
p1 byte "List1:",0
p2 byte "List2:",0
p3 byte "Merged:",0
.code
main proc
sub EAX,EAX
mov ESI,OFFSET a1 ; list 1
mov EDI,OFFSET a2 ; list 2
mov EDX,OFFSET a3 ; merged list
mov EBX, lengthof a1 ; elements in list 1
mov ECX, lengthof a2 ; elements in list 2
call Merge
mov EDX, offset p1
call writestring
call crlf
mov ECX,lengthof a1
mov EDX,offset a1
call Display
call crlf
mov EDX, offset p2
call writestring
call crlf
mov ECX,lengthof a2
mov EDX,offset a2
call Display
call crlf
mov EDX, offset p3
call writestring
call crlf
mov ECX,lengthof a1
add ECX,lengthof a2
mov EDX,offset a3
call Display
exit
main endp
;=====================================================================
Merge proc
TheLoop:
cmp ebx, 0
je AddA2
cmp ecx, 0
je AddA1
mov EAX,[ESI]
cmp EAX,[EDI]
jl AddA1 ;Jump if less
jmp AddA2 ;Jump if greater or equal
AddA1:
cmp ebx, 0
je EndJump
mov EAX,[ESI]
mov [EDX],ax
add ESI,4
add EDX,4
dec EBX
jmp TheLoop
AddA2:
cmp ecx, 0
je EndJump
mov EAX,[EDI]
mov [EDX],ax
add EDI,4
add EDX,4
dec ECX
jmp TheLoop
EndJump:
ret
Merge endp
;==================================================================
Display proc
DisplayLoop:
mov EAX,[EDX]
add EDX, 4
call writedec
call crlf
loop DisplayLoop
ret
Display endp
;===================================================================
end main
Similar Samples
Explore our curated selection of programming homework samples at ProgrammingHomeworkHelp.com. From Python projects to Java assignments and more, each sample is crafted to illustrate practical solutions and coding techniques. Whether you're a student or professional, our diverse samples provide valuable insights to enhance your programming skills and academic success.
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language
Assembly Language