Tip of the day
News
Instructions
Objective
Write a program that allows users to type a character and display it in upper and lower case, and the ascii code in hex and decimal in Pep9 assembly language.
Requirements and Specifications
Write the following program using Pep9 using Assembly Language
- First load the value FFFF in the A register.
- Save the value in A to memory and display the value using decimal out. ( This should display a -1 then an LF )
- Next, get a character from the user using character input. For simplicity, we will assume that the user types in a lower case alpha character.
- Display the following properties of the character: Original Character then LF Character as Uppercase then LF Decimal ASCII value of the Character then LF Decimal ASCII value of the Uppercase Letter then LF Hex value of the original lowercase letter then LF Other requirements for this program: Put the data section in low memory and Branch around it. Use the A register. DO NOT use the X register Print a LF after each output. This program should work for any lowercase letter submitted by the user. This program should work running it several times changing the input. That is, it should not work only the first time you run it. Run it a couple of times changing the input each time Deliverables: Turn in the following electronically: file with your assembly code with the pep extension: eg. prog1.pep Hints: When you save the assembly code, it saves a .pep file. You may need to load and store 1 and 2 byte values using Load/Store and LoadByte and StoreByte. Remember to put a stop instruction at the end of your program to complete the assembly language assignment. Remember that you are to work individually on your programs. You should not discuss your ideas and algorithms with other people. Sample input/output: Input: j Output: -1 j J 106 74 006A *Note that this program should work for any lowercase input. (Not just lower case j)
Screenshots of output
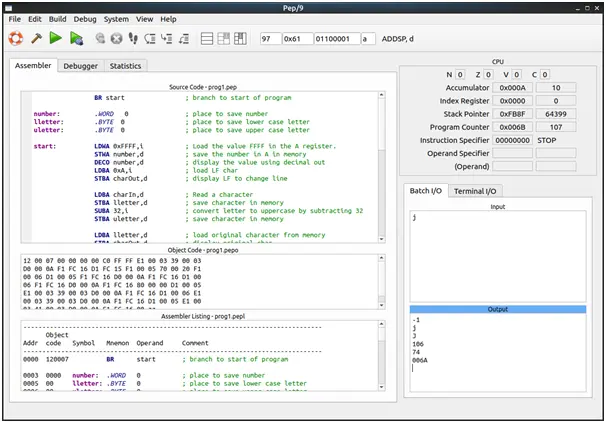
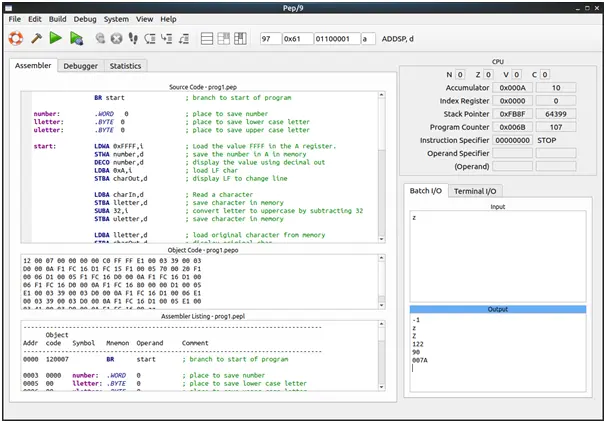
Source Code
BR start ; branch to start of programnumber: .WORD 0 ; place to save numberlletter: .BYTE 0 ; place to save lower case letteruletter: .BYTE 0 ; place to save upper case letter start: LDWA 0xFFFF,i ; Load the value FFFF in the A register. STWA number,d ; save the number in A in memory DECO number,d ; display the value using decimal out LDBA 0xA,i ; load LF char STBA charOut,d ; display LF to change line LDBA charIn,d ; Read a character STBA lletter,d ; save character in memory SUBA 32,i ; convert letter to uppercase by subtracting 32 STBA uletter,d ; save character in memory LDBA lletter,d ; load original character from memory STBA charOut,d ; display original char LDBA 0xA,i ; load LF char STBA charOut,d ; display LF to change line LDBA uletter,d ; load uppercase character from memory STBA charOut,d ; display uppercase char LDBA 0xA,i ; load LF char STBA charOut,d ; display LF to change line ANDA 0,i ; clear A to zero LDBA lletter,d ; load lowercase character from memory STWA number,d ; save the value as a 2 byte number DECO number,d ; display lowercase ASCII value LDBA 0xA,i ; load LF char STBA charOut,d ; display LF to change line LDBA uletter,d ; load uppercase character from memory STWA number,d ; save the value as a 2 byte number DECO number,d ; display uppercase ASCII value LDBA 0xA,i ; load LF char STBA charOut,d ; display LF to change line LDBA lletter,d ; load lowercase character from memory STWA number,d ; save the value as a 2 byte number HEXO number,d ; display lowercase char hex value LDBA 0xA,i ; load LF char STBA charOut,d ; display LF to change line STOP ; End the program .END
Related Samples
Explore our Assembly Language Assignment Samples for precise solutions to low-level programming challenges. Covering concepts such as registers, memory management, and bitwise operations, these examples offer clear explanations and practical implementations. Perfect for students aiming to master assembly language programming and excel in their coursework with structured, educational content.
Assembly Language
Word Count
11673 Words
Writer Name:Dr. Denise M. Lopez
Total Orders:500
Satisfaction rate:
Assembly Language
Word Count
3655 Words
Writer Name:Dr. John L. Sanders
Total Orders:900
Satisfaction rate:
Assembly Language
Word Count
11176 Words
Writer Name:Rehana Magnus
Total Orders:750
Satisfaction rate:
Assembly Language
Word Count
1782 Words
Writer Name:Rehana Magnus
Total Orders:750
Satisfaction rate:
Assembly Language
Word Count
7747 Words
Writer Name:Rehana Magnus
Total Orders:750
Satisfaction rate:
Assembly Language
Word Count
2371 Words
Writer Name:Rehana Magnus
Total Orders:750
Satisfaction rate:
Assembly Language
Word Count
1270 Words
Writer Name:Rehana Magnus
Total Orders:750
Satisfaction rate:
Assembly Language
Word Count
5650 Words
Writer Name:Rehana Magnus
Total Orders:750
Satisfaction rate:
Assembly Language
Word Count
5768 Words
Writer Name:Rehana Magnus
Total Orders:750
Satisfaction rate:
Assembly Language
Word Count
1586 Words
Writer Name:Rehana Magnus
Total Orders:750
Satisfaction rate:
Assembly Language
Word Count
2204 Words
Writer Name:Rehana Magnus
Total Orders:750
Satisfaction rate:
Assembly Language
Word Count
3452 Words
Writer Name:Rehana Magnus
Total Orders:750
Satisfaction rate:
Assembly Language
Word Count
6803 Words
Writer Name:Rehana Magnus
Total Orders:750
Satisfaction rate:
Assembly Language
Word Count
5272 Words
Writer Name:Rehana Magnus
Total Orders:750
Satisfaction rate:
Assembly Language
Word Count
2752 Words
Writer Name:Rehana Magnus
Total Orders:750
Satisfaction rate:
Assembly Language
Word Count
10514 Words
Writer Name:Rehana Magnus
Total Orders:750
Satisfaction rate:
Assembly Language
Word Count
2587 Words
Writer Name:Rehana Magnus
Total Orders:750
Satisfaction rate:
Assembly Language
Word Count
15048 Words
Writer Name:Rehana Magnus
Total Orders:750
Satisfaction rate:
Assembly Language
Word Count
4268 Words
Writer Name:Rehana Magnus
Total Orders:750
Satisfaction rate:
Assembly Language
Word Count
4470 Words
Writer Name:Rehana Magnus
Total Orders:750
Satisfaction rate: