Tip of the day
News
Instructions
Objective
Write a program to implement basic functions using the LISP programming language.
Requirements and Specifications
Function intmax to return the greatest number among two integers.
Function dup which takes an element and returns a tuple with the element duplicated .
Recursive function to add up all numbers from 1 to a given upper limit.
Function backwards to return the reverse order list of a given input list but no sublists .
Function totalbackwards to return total reverse order list .
Function with algorithm to detect palindrome.
Screenshots of output
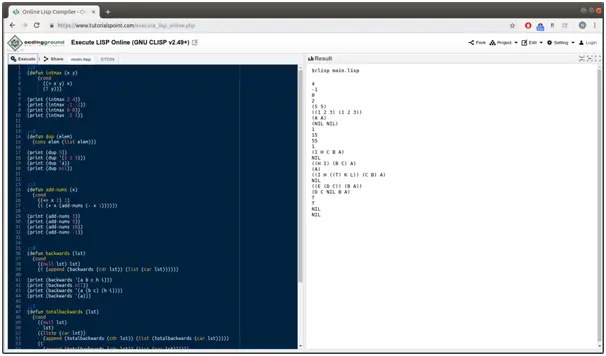
Source Code
;;1(defun intmax (x y) (cond ((> x y) x) (T y)))(print (intmax 2 4))(print (intmax -1 -2))(print (intmax 0 0))(print (intmax -2 2));;2(defun dup (elem) (cons elem (list elem)))(print (dup 5))(print (dup '(1 2 3)))(print (dup 'a))(print (dup nil));;3(defun add-nums (x) (cond ((<= x 1) 1) (t (+ x (add-nums (- x 1))))))(print (add-nums 1))(print (add-nums 5))(print (add-nums 10))(print (add-nums -1));;4(defun backwards (lst) (cond ((null lst) lst) (t (append (backwards (cdr lst)) (list (car lst))))))(print (backwards '(a b c h i)))(print (backwards nil))(print (backwards '(a (b c) (h i))))(print (backwards '(a)));;5(defun totalbackwards (lst) (cond ((null lst) lst) ((listp (car lst)) (append (totalbackwards (cdr lst)) (list (totalbackwards (car lst))))) (t (append (totalbackwards (cdr lst)) (list (car lst))))))(print (totalbackwards '(a (b c) ((l k (t)) h i))))(print (totalbackwards nil))(print (totalbackwards '((a b) ((c d) e))))(print (totalbackwards '(a b () c d)));;6(defun palindrome (lst) (equal lst (backwards lst)))(print (palindrome '(r o t o r)))(print (palindrome '(r a c e c a r)))(print (palindrome '(p a l i n d r o m e)))(print (palindrome '(r a c e c a r s)))
Related Samples
Explore Lisp Assignment Samples: Delve into our curated selection showcasing Lisp solutions covering recursion, list manipulation, and functional programming paradigms. From basic exercises to complex algorithms, each sample offers insights into Lisp programming. Strengthen your Lisp skills and excel in functional programming with our comprehensive examples.
Lisp
Word Count
4794 Words
Writer Name:Megan Talbot
Total Orders:366
Satisfaction rate:
Lisp
Word Count
6523 Words
Writer Name:Chloe Martin
Total Orders:1422
Satisfaction rate:
Lisp
Word Count
4639 Words
Writer Name:Megan Talbot
Total Orders:366
Satisfaction rate:
Lisp
Word Count
3893 Words
Writer Name:Chloe Martin
Total Orders:1422
Satisfaction rate:
Lisp
Word Count
3235 Words
Writer Name:Dr. Michelle Wong
Total Orders:509
Satisfaction rate:
Lisp
Word Count
3967 Words
Writer Name:Megan Talbot
Total Orders:366
Satisfaction rate:
Lisp
Word Count
2178 Words
Writer Name:Megan Talbot
Total Orders:366
Satisfaction rate: