Instructions
Requirements and Specifications
- The file contains floating point numbers that represent grades out of 100.0%.
- The contents of the file are in the following format:
- numberX :NNN.NN
- X – represent a value from 0-999
- NNN.NN – represents a number in the range (0.00 – 100.00) inclusive
- Your program will perform the following actions:
- Look for numbers below and equal to 60.00, and do the following:
- Open a new file called below60.txt, in text mode
- Write the numbers into a file 10 per row and separated by space(s)
- Look for numbers above 60.00, and do the following:
- Open a file called above60.txt
- Write the numbers into a file 10 per row and separated by space
- Close all files on completion and print the following information:
- A count of the number of floats in the file numbers.txt
- The count of numbers in the file below60.txt
- The count of numbers in the file above60.txt
- Look for numbers below and equal to 60.00, and do the following:
Problem 2 (40):
Write a C program that will open one of the files labelled: filea.hex, fileb.hex, filec.hex, filed.hex, filee.hex
- Ask the user to enter the name of the file to open. The user can choose any of the files listed above. (filea, fileb etc).
- Read the contents of the file and produce the following output format
- Offset is the hexadecimal representation of the first value in each line. The value 00000 is the offset of value 7f – which is the control sequence DEL. The value 00010 is the offset of the value 03 which is the control sequence ETX.
- Hexadecimal Data represents the hexadecimal values of each character in the text file.
- Character Format represents the characters that were read from the file.
- Print a ‘.’ when there isn’t an ASCII representation of the retrieved character from the file.
- You should print a final summarization of the contents of the file; based on the following criteria
- Print the value of the first 4 bytes of the file as a hexadecimal value. Remember that the bytes are placed in the file in little endian format.
- If 75 – 100% of the bytes in the file fall in the range of printable ascii characters (0x20 – 0x7E) then conclude that this was a Text file
- If 25 – 74% of the bytes in the file fall in the range of printable ascii characters (0x20 – 0x7E) then conclude that this was possibly a Text file
- If less than 25% of the bytes in the file fall in the range of printable ascii characters (0x20 – 0x7E) then conclude that this is a Binary file.
Screenshots of output
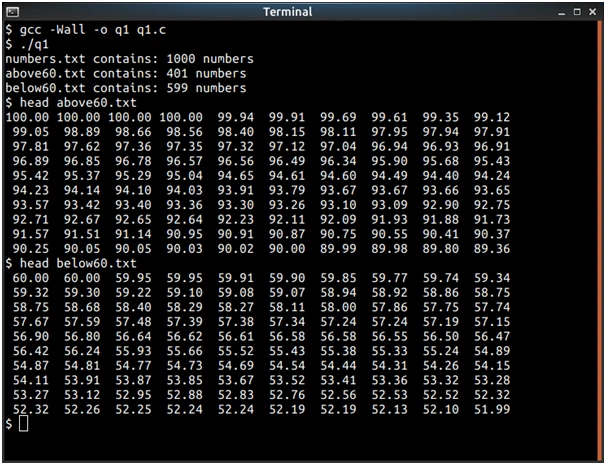
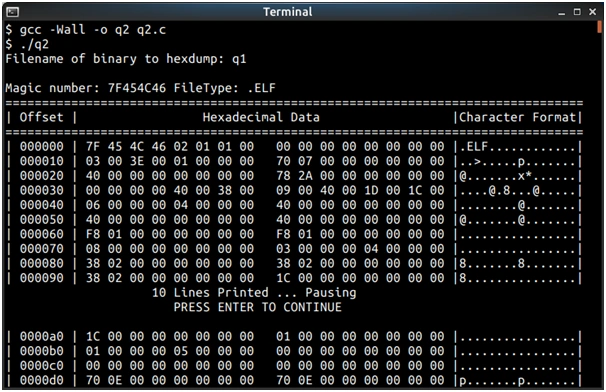
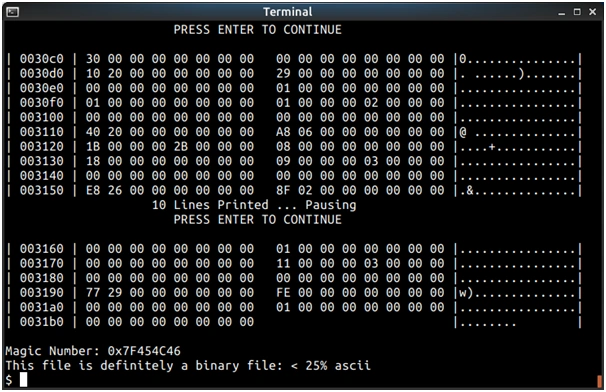
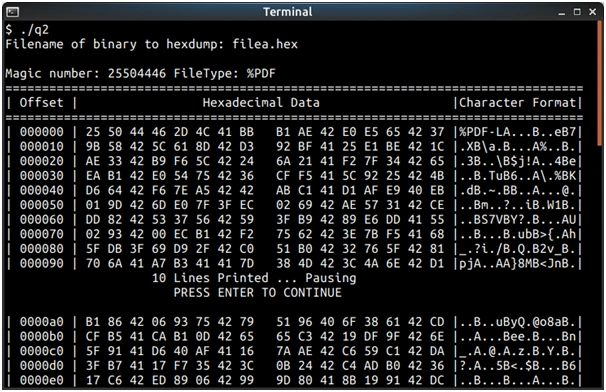
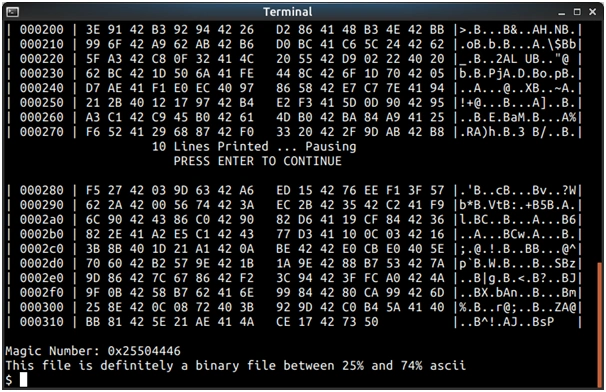
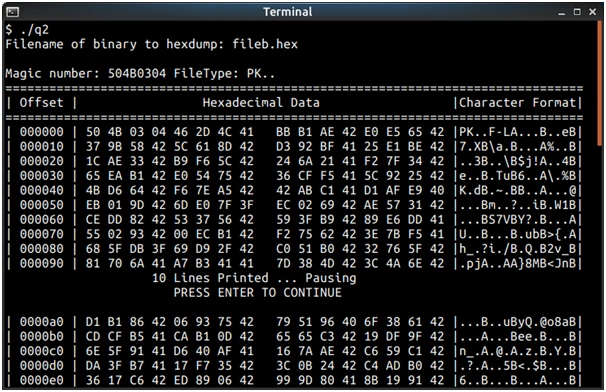
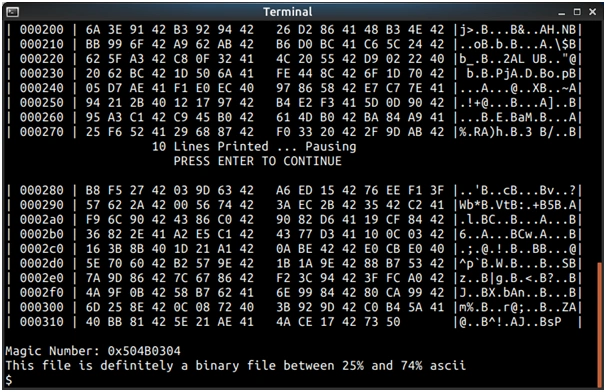
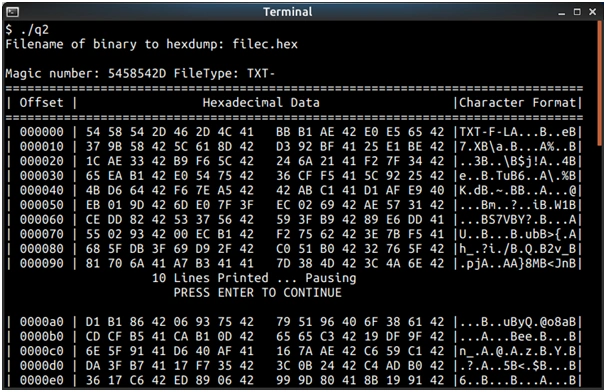
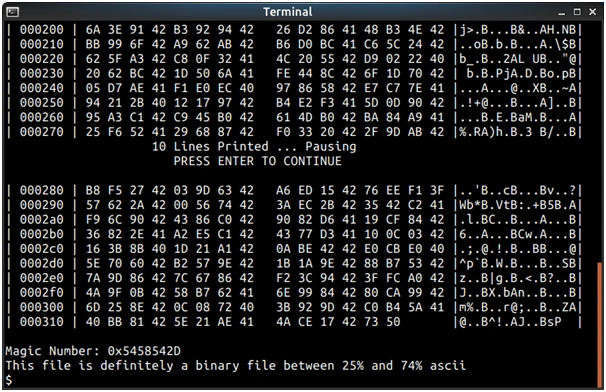
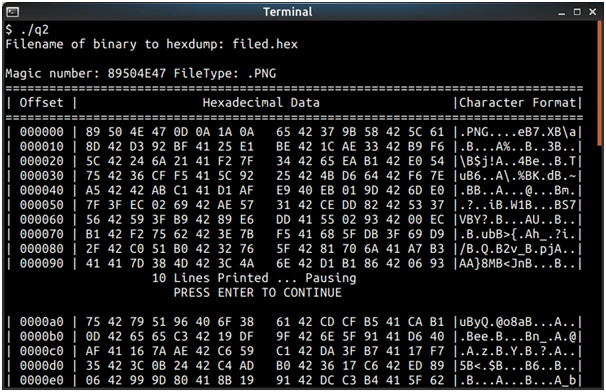
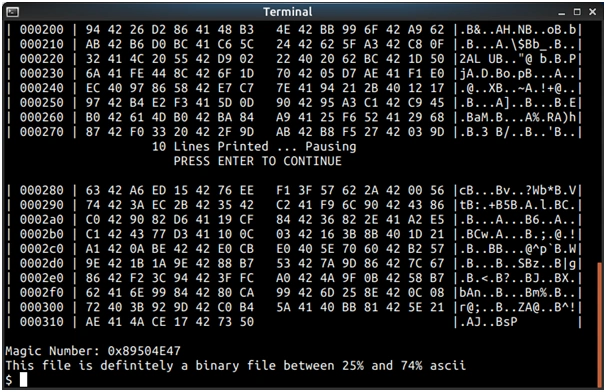
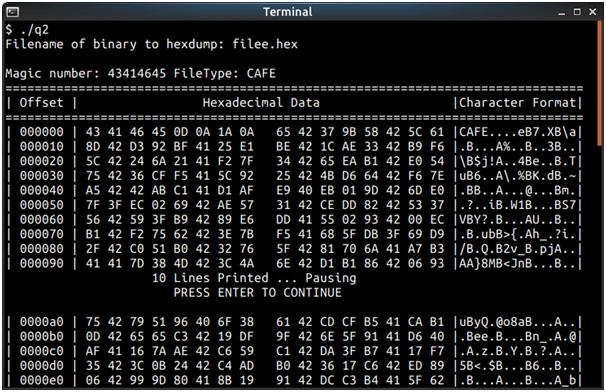
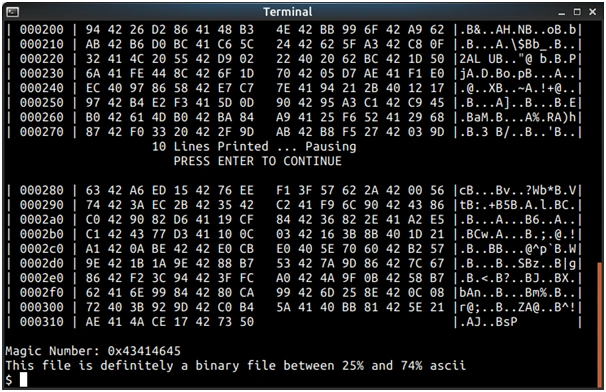
Source Code
Question 1
/**
* Author: ??????
* Date: April 8, 2022
* Description: Simple program that reads a file of numbers and saves them in
* two new files
*/
#include
#include
/**
* Function to compare two elements in the array for the qsort
*/
int compfun(const void *a, const void *b)
{
float diff;
diff = (*(float *)(b) - *(float *)(a)); // subtract in reverse order
if (diff < 0)
return (-1);
else if (diff > 0)
return (1);
else
return (0);
}
int main()
{
FILE *finput, *fabove60, *fbelow60;
int i, j;
int n;
int numtotal;
int numabove60;
int numbelow60;
float readnum;
float above60[1000]; // array to save read numbers (maximum 1000)
float below60[1000]; // array to save read numbers (maximum 1000)
finput = fopen("numbers.txt", "rt"); // open input file
numtotal = 0; // initialize total number of numbers
numbelow60 = 0; // initialize total number of numbers below 60
numabove60 = 0; // initialize total number of numbers above 60
// read while there are numbers with the given format
while (fscanf(finput, "number%d :%f ", &n, &readnum) == 2)
{
if (readnum <= 60.00) // if below 60
{
below60[numbelow60] = readnum; // copy to array
numbelow60++;
}
else // if above 60
{
above60[numabove60] = readnum; // copy to array
numabove60++;
}
numtotal++; // increment total read
}
fclose(finput); // close file
// sort numbers in both arrays
qsort(below60, numbelow60, sizeof(float), compfun);
qsort(above60, numabove60, sizeof(float), compfun);
fbelow60 = fopen("below60.txt", "wt"); // open output file
for (i = 0; i < numbelow60; )
{
for (j = 0; j < 10 && i < numbelow60; i++, j++) // print 10 numbers
{
fprintf(fbelow60, "%6.2f ", below60[i]);
}
fprintf(fbelow60, "\n");
}
fclose(fbelow60); // close file
fabove60 = fopen("above60.txt", "wt"); // open output file
for (i = 0; i < numabove60; )
{
for (j = 0; j < 10 && i < numabove60; i++, j++) // print 10 numbers
{
fprintf(fabove60, "%6.2f ", above60[i]);
}
fprintf(fabove60, "\n");
}
fclose(fabove60); // close file
printf("numbers.txt contains: %d numbers\n", numtotal);
printf("above60.txt contains: %d numbers\n", numabove60);
printf("below60.txt contains: %d numbers\n", numbelow60);
return (0);
}
Question 2
/**
* Author: ??????
* Date: April 8, 2022
* Description: Simple program that reads a file and display the contents in hex
* and ascii
*/
#include
#include
// Function prototypes
void print_ascii(char c);
void print_magic_hex(unsigned char *magic);
void print_magic_ascii(unsigned char *magic);
void print_separator();
int print_hex8(unsigned char *line, int start, int len);
void print_ascii16(unsigned char *line, int len);
void print_line(FILE *f, unsigned int offset);
void print_contents(FILE *f);
void print_summary();
// Global variables
int totalbytes = 0; // number of bytes in the file
int numprintables = 0; // number of printable ascii bytes in the file
int main()
{
char filename[100];
unsigned char magic[4];
FILE *input;
printf("Filename of binary to hexdump: ");
fgets(filename, 100, stdin); // read string
filename[strlen(filename) - 1] = '\0'; // remove ending enter
input = fopen(filename, "rb"); // open file as binary
if (input == NULL) // if error opening
{
printf("Error opening file \"%s\"\n", filename);
printf("Terminating program\n");
return (1);
}
fread(magic, 1, 4, input); // read 4 bytes from file (magic number)
printf("\nMagic number: ");
print_magic_hex(magic); // print magic as hex
printf(" FileType: ");
print_magic_ascii(magic); // print magic as ascii
printf("\n");
fseek(input, 0, SEEK_SET); // move file pointer back to start of file
print_contents(input); // print all file contents
// print magic number as int
printf("Magic Number: 0x");
print_magic_hex(magic); // print magic as hex
printf("\n");
print_summary();
fclose(input);
return (0);
}
/**
* Print a byte as ascii, if not printable, prints a dot
*/
void print_ascii(char c)
{
if (c >= 32 && c < 127) // if it's a printable ascii
{
printf("%c", c); // print byte as ascii
}
else
{
printf(".");
}
}
/**
* Print the magic number (first 4 bytes) of the file as hex
*/
void print_magic_hex(unsigned char *magic)
{
int i;
for (i = 0; i < 4; i++)
{
printf("%02X", magic[i]); // print bytes as hex
}
}
/**
* Print the magic number (first 4 bytes) of the file as ascii
*/
void print_magic_ascii(unsigned char *magic)
{
int i;
for (i = 0; i < 4; i++)
{
print_ascii(magic[i]); // print bytes as ascii
}
}
/**
* Prints a horizontal separator
*/
void print_separator()
{
int i;
for (i = 0; i < 79; i++)
{
printf("=");
}
printf("\n");
}
/**
* Prints 8 bytes of the line of length len starting at given start position
* returns the last position in the line
*/
int print_hex8(unsigned char *line, int start, int len)
{
int i;
int j = start; // position in line
for (i = 0; i < 8; i++)
{
if (j < len) // print only bytes in line length
{
printf(" %02X", line[j]); // print as hex
j++;
}
else
{
printf(" "); // if printing beyond end of file, use spaces
}
}
return j; // return last position
}
/**
* Prints 16 bytes of the line of lenght len
*/
void print_ascii16(unsigned char *line, int len)
{
int i;
int j = 0; // position in line
for (i = 0; i < 16; i++)
{
if (j < len) // print only bytes in line length
{
print_ascii(line[j]); // print as ascii
if (line[j] >= 32 && line[j] < 127) // if it's a printable ascii
numprintables++; // increment number of printables
j++;
}
else
{
printf(" "); // if printing beyond end of file, use spaces
}
}
}
/**
* Print a line of at most 16 bytes from the file, format it to show offset,
* hex values and ascii values
*/
void print_line(FILE *f, unsigned int offset)
{
unsigned char line[16]; // bytes in line
int nread;
int pos;
printf("|");
printf(" %06x ", offset); // print offset as hexadecimal
printf("|");
nread = fread(line, 1, 16, f); // read 16 bytes
totalbytes += nread; // add read bytes to total
pos = print_hex8(line, 0, nread); // print first 8 bytes as hex
printf(" ");
print_hex8(line, pos, nread); // print last 8 bytes as hex
printf(" |");
print_ascii16(line, nread); // print allbytes as ascii
printf("|\n");
}
/**
* Print all the contents of the file as hex and ascii, pauses every 10 lines
*/
void print_contents(FILE *f)
{
unsigned int offset = 0; // starting offset
int nline;
print_separator();
printf("| Offset |");
printf(" Hexadecimal Data ");
printf("|Character Format|\n");
print_separator();
while(!feof(f)) // print all contents until EOF
{
for (nline = 0; nline < 10 && !feof(f); nline++) // print 10 lines at a time
{
print_line(f, offset);
offset += 16; // advance offset by 16 bytes
}
if (!feof(f)) // only wait if there is more content
{
printf(" 10 Lines Printed ... Pausing\n");
printf(" PRESS ENTER TO CONTINUE\n");
getchar();
}
}
printf("\n");
}
/**
* Print a summary of the file based on the printable content
*/
void print_summary()
{
int percentage;
percentage = 100 * numprintables / totalbytes;
printf("This file is definitely a ");
if (percentage >= 75)
{
printf("text file: >= 75%% ascii\n");
}
else if (percentage >= 25)
{
printf("binary file between 25%% and 74%% ascii\n");
}
else
{
printf("binary file: < 25%% ascii\n");
}
}
Related Samples
Explore our C Assignments samples, featuring essential concepts like loops, functions, arrays, and pointers. Master programming fundamentals with clear, annotated code examples. Enhance your understanding of C language intricacies through practical assignments tailored for educational purposes. Level up your programming skills and excel in C programming effortlessly.
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C