Question:
Write a C assignment program for a mile-to-kilometer conversion to run on ocelot. (1 Mile = 1.60934 Kilometers)
Create a nicely formatted chart with the conversions of each of the following values from Miles to Kilometers. There is no user input, these values can be constants in the program.
- 2
- 5.65
- 300
- 1845
Solution:
#include
void convert(float miles[]){
float kilometeres[4];
for(int i=0; i < 4 ; ++i)
{
kilometeres[i] = miles[i] * 1.60934; //converts miles to Kilometeres
}
printf("Miles to Kilometeres chart using c.\n");
printf("____________________________________________________\n"); //design for printing chart
printf("| Miles | Kilometeres |\n");
printf("----------------------------------------------------\n");
for(int i =0 ; i < 2; i ++)
{
printf("| %.5f | %.5f |\n", miles[i], kilometeres[i]);
}
printf("| %.5f | %.5f |\n", miles[2], kilometeres[2]);
printf("| %.5f | %.5f |\n", miles[3], kilometeres[3]);
printf("----------------------------------------------------");
}
int main()
{
// Declaring an array of input values provided
float miles[4] = {2.0, 5.65, 300, 1845};
// An array to store kilometer values, once converted
float kilometers[4];
for(int i=0; i < 4 ; ++i)
{
// Conversion of miles to kilometers
kilometers[i] = miles[i] * 1.60934;
}
// Displaying the information in a table
printf("\t CONVERTING MILES\n");
printf("%15s%15s\n", "Miles", "Kilometers");
for(int i=0; i < 4 ; ++i)
{
printf("%15.5f%15.5f\n", miles[i], kilometers[i]);
}
return 0;
}
Output:
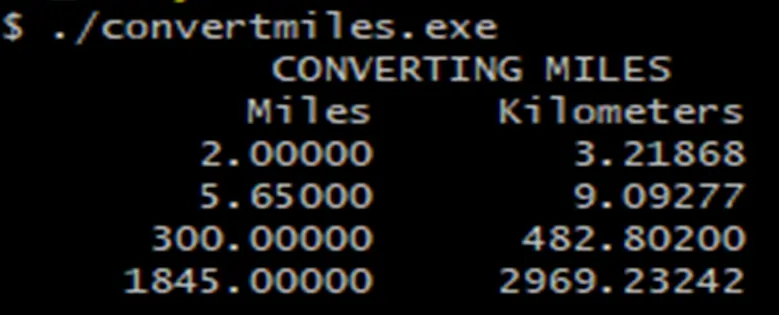
Related Samples
Explore our collection of free C programming samples showcasing practical solutions and examples. Discover efficient coding techniques and best practices tailored to enhance your programming skills. Dive into our comprehensive library today!
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C