Instructions
Objective
Write a python assignment program to create an email validation using regular expression.
Requirements and Specifications
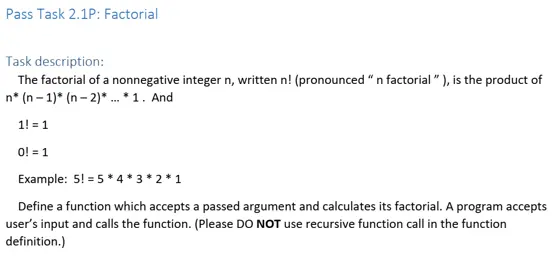
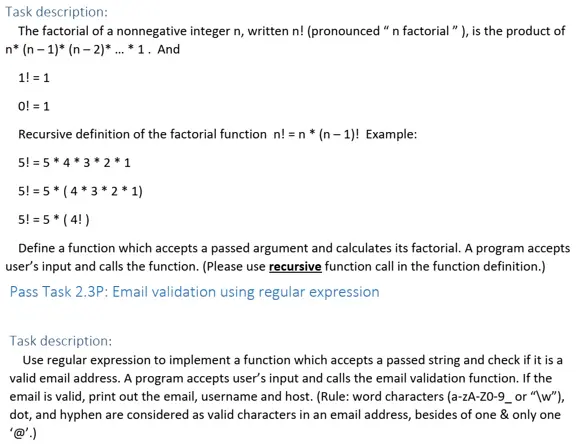
Screenshot
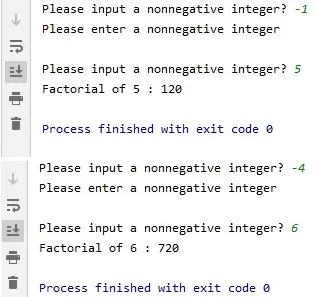
Source Code
TASK 1
def factorial(n):
# starting with value 1
result = 1
# keep multiplying by consecutive integers including n
for i in range(n):
result *= (i + 1)
return result
def main():
# keep asking user until nonnegative value is entered
while True:
n = int(input('Please input a nonnegative integer? '))
if n >= 0:
# calculating factorial of given integer
print('Factorial of', n, ':', factorial(n))
return
else:
# showing error message
print('Please enter a nonnegative integer')
print()
if __name__ == '__main__':
main()
TASK 2
def factorial(n):
# if n == 0, returning 1
if n == 0:
return 1
# returning result as a product of (n-1)! and n
return factorial(n-1) * n
def main():
# keep asking user until nonnegative value is entered
while True:
n = int(input('Please input a nonnegative integer? '))
if n >= 0:
# calculating factorial of given integer
print('Factorial of', n, ':', factorial(n))
return
else:
# showing error message
print('Please enter a nonnegative integer')
print()
if __name__ == '__main__':
main()
TASK 3
import re
def check_email(email):
# using following regex:
# - positive number of symbols: alphanumeric/-/.
# - single @
# - positive number of symbols: alphanumeric/-/.
regex = '^[\w\-.]+[@][\w\-.]+$'
if re.search(regex, email):
# if email matches pattern, searching for index of '@'
idx = email.index('@')
# returning separated username and host, as a tuple
return email[:idx], email[idx+1:]
# if valid is not valid, returning None
return None
def main():
# keep asking user until value email is entered
while True:
result = check_email(input('Please input your email address:').strip())
if result:
# outputting result of email parsing
print('email: ', result[0] + '@' + result[1], ',', 'username: ', result[0], ',', 'host: ', result[1])
return
else:
# showing error message
print('Not a valid email.')
print()
if __name__ == '__main__':
main()
Similar Samples
Discover our sample programming assignments at ProgrammingHomeworkHelp.com. These exemplify our dedication to delivering top-notch solutions across various languages and complexities. Each sample demonstrates our proficiency in problem-solving and adherence to quality standards, ensuring your academic or professional programming needs are met with excellence.
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python