Instructions
Objective
Write a program to store and retrieve data in python.
Requirements and Specifications
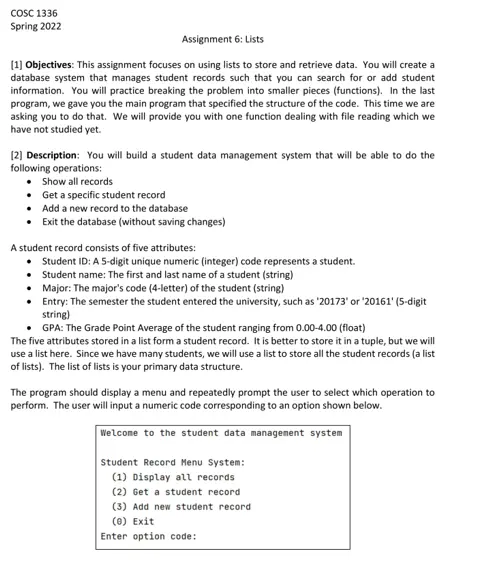
Source Code
import csv
def read_file(record_file):
dblist = []
with open(record_file, 'r') as stu_file:
records = csv.reader(stu_file)
for row in records:
dblist.append([int(row[0]), row[1], row[2], row[3], float(row[4])])
return dblist
def read_menu_option():
while True:
print('Student Record Menu System:')
print(' (1) Display all records')
print(' (2) Get a student record')
print(' (3) Add new student record')
print(' (0) Exit')
try:
choice = int(input('Enter option code: '))
if choice < 0 or choice > 3:
raise Exception()
return choice
except:
print('Invalid input')
print()
def display_all_records(db):
print('Display all records.')
print()
print('{:<6} {:<20} {:<5} {:<5} {:<4}'.format('Stu ID', 'Name', 'Major', 'Entry', 'GPA'))
for record in db:
print('{:>6} {:<20} {:<5} {:<5} {:.2f}'.format(str(record[0]), record[1], record[2], record[3], record[4]))
def _find_record(db, id_str):
found_index = -1
result = None
for i in range(len(db)):
if str(db[i][0]) == id_str:
found_index = i
result = db[i]
break
return found_index, result
def get_record(db):
id = input('Enter a student ID: ')
search_result = _find_record(db, id)
print()
if search_result[0] >= 0:
result = search_result[1]
print('Record found with index', search_result[0])
print('{:<6} {:<20} {:<5} {:<5} {:<4}'.format('Stu ID', 'Name', 'Major', 'Entry', 'GPA'))
print('{:>6} {:<20} {:<5} {:<5} {:.2f}'.format(str(result[0]), result[1], result[2], result[3],
result[4]))
else:
print('Student ID', id, 'not in the list.')
def add_record(db):
id = input('Enter the student ID for the new record: ')
search_result = _find_record(db, id)
print()
if search_result[0] >= 0:
result = search_result[1]
print('Record found with index', search_result[0])
print('{:<6} {:<20} {:<5} {:<5} {:<4}'.format('Stu ID', 'Name', 'Major', 'Entry', 'GPA'))
print('{:>6} {:<20} {:<5} {:<5} {:.2f}'.format(str(result[0]), result[1], result[2], result[3],
result[4]))
print('Can not add student with such ID')
else:
print('Student ID', id, 'not in the list.')
print()
name = input('Enter student\'s name: ')
major = input('Enter student\'s major: ')
semester = input('Enter student\'s semester of entry: ')
gpa = input('Enter student GPA: ')
db.append([int(id), name, major, semester, float(gpa)])
print('Record added.')
def main():
print('Welcome to the student data management system')
print()
student_db = read_file('student_records.csv')
is_over = False
while not is_over:
choice = read_menu_option()
if choice == 1:
display_all_records(student_db)
elif choice == 2:
get_record(student_db)
elif choice == 3:
add_record(student_db)
else:
is_over = True
print()
print('Exiting the database.')
if __name__ == '__main__':
main()
Similar Samples
Explore our extensive collection of programming homework samples at ProgrammingHomeworkHelp.com. From Java and Python to Machine Learning and Data Structures, our samples showcase our proficiency in delivering solutions tailored to your programming challenges. Each example exemplifies our commitment to clarity, accuracy, and academic excellence. Dive into our samples to see how we can assist you in mastering programming concepts and achieving your academic goals.
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python
Python