Instructions
Objective
Write a program to create a histogram of random numbers chosen with normal distribution using Fortran 95 assignment solution.
Requirements and Specifications
.webp)
Screenshots of output
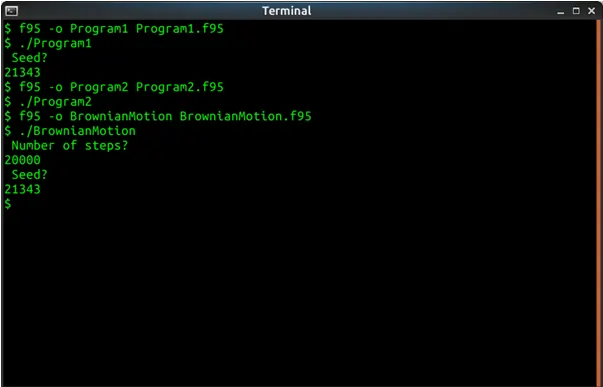
Source Code
PROGRAM Program1
IMPLICIT NONE
REAL, PARAMETER :: PI=3.14159265358979323846
INTEGER, PARAMETER :: NGEN1 = 13000
REAL, PARAMETER :: AVG1 = 22.0
REAL, PARAMETER :: STDDEV1 = 2.5
INTEGER, PARAMETER :: NGEN2 = 7000
REAL, PARAMETER :: AVG2 = 15.5
REAL, PARAMETER :: STDDEV2 = 1.0
INTEGER :: i
REAL :: n1, n2, ngauss
INTEGER :: new_seed;
! Read seed
print*, "Seed?"
read*, new_seed
! set seed for random generator
call srand(new_seed)
open(11,FILE="Bumps.txt", STATUS='REPLACE', ACCESS='SEQUENTIAL',ACTION='WRITE')
! Generate 13000 numbers with gaussian distribution
! with average= 22.0 and stddev = 2.5
do i = 1, NGEN1
! generate two numbers
n1 = rand()
n2 = rand()
! generate normally distributed number
ngauss = boxMuller(n1, n2, AVG1, STDDEV1)
write(11, *) ngauss
end do
! Generate 7000 numbers with gaussian distribution
! with average= 15.5 and stddev = 1.0
do i = 1, NGEN2
! generate two numbers
n1 = rand()
n2 = rand()
! generate normally distributed number
ngauss = boxMuller(n1, n2, AVG2, STDDEV2)
write(11, *) ngauss
end do
close(11)
CONTAINS
! generate a random number with gaussian distribution
! and given average m and standard deviation s, using
! the Box-Muller algorithm based on the uniformly
! distributed numbers n1 and n2
REAL FUNCTION boxMuller(n1, n2, m, s)
REAL, INTENT(IN) :: n1, n2, m, s
REAL :: r, z1
r = sqrt(-2.0 * log(n1))
z1 = r*cos(2.0 * PI * n2)
! transform to given median and deviation
boxMuller = m + s*z1
END FUNCTION boxMuller
END PROGRAM Program1
PROGRAM Program2
IMPLICIT NONE
INTEGER, PARAMETER :: NNUMBERS = 20000
REAL, PARAMETER :: HIST_MIN = 10
REAL, PARAMETER :: HIST_MAX = 30
INTEGER, PARAMETER :: HIST_NBOXES = 100
INTEGER :: i, box
REAL :: n, boxwid, start
INTEGER :: histogram(100)
! initialize histogram to zero
do i = 1, HIST_NBOXES
histogram(i) = 0
end do
! box size
boxwid = (HIST_MAX - HIST_MIN) / HIST_NBOXES
! Read the 20000 numbers from the file and
! build histogram with the data
open(11,FILE="Bumps.txt")
do i = 1, NNUMBERS
read(11, *) n
if (n >= HIST_MIN .and. n <= HIST_MAX) then
box = (n - HIST_MIN)/boxwid + 1
histogram(box) = histogram(box) + 1
end if
end do
close(11)
! write histogram to a file
open(12,FILE="BumpsHist.txt", STATUS='REPLACE', ACCESS='SEQUENTIAL',ACTION='WRITE')
do i = 1, HIST_NBOXES
start = (i - 1)*boxwid + HIST_MIN
write(12, *) start, histogram(i)
end do
close(12)
END PROGRAM Program2
Contact Details
Related Samples
At ProgrammingHomeworkHelp.com, we provide comprehensive assignment support to students, including related samples of Fortran programming assignments. Our website is designed to help students excel in their programming courses by offering expertly crafted examples and detailed solutions. Whether you're struggling with Fortran code or need guidance on complex programming concepts, our resources are here to assist you. Explore our sample assignments to gain insights and improve your understanding, ensuring you achieve academic success in your programming studies.
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming
Programming