Claim Your Discount Today
Take your coding game to new heights with expert help at unbeatable prices. Got a tricky project or a tight deadline? We’ve got you covered! Use code PHHBF10 at checkout and save BIG. Don’t wait—this exclusive Black Friday deal won’t last long. Secure your academic success today!
We Accept
- Understanding the Problem Statement
- Game Dynamics:
- Input:
- Output:
- Pathway to Finding a Solution
- 1. Parsing the Input
- 2. Initializing Variables
- 3. Processing Each Play
- 4. Handling Edge Cases
- Steps in Detail
- Advanced Considerations
- Testing and Debugging
- Conclusion
When designing software to simulate competitive games, accuracy and clarity are essential. This involves translating game rules into precise algorithms and ensuring that the program correctly reflects the dynamics of the game. Assignments involving programming challenges are a staple in computer science and software engineering education. These assignments not only test your understanding of coding concepts but also hone your problem-solving skills. One such assignment type could be simulating a volleyball game, like the one described in the example. This guide will walk you through how to approach and solve your game design assignment, focusing on general problem-solving strategies, programming techniques, and best practices. By the end of this guide, you should be well-equipped to tackle similar Computer science assignments with confidence.
Understanding the Problem Statement
When tackling a game simulation problem, it's crucial to understand the game rules and how they affect the outcome. In our example, the game is volleyball, and the scoring system has specific rules:
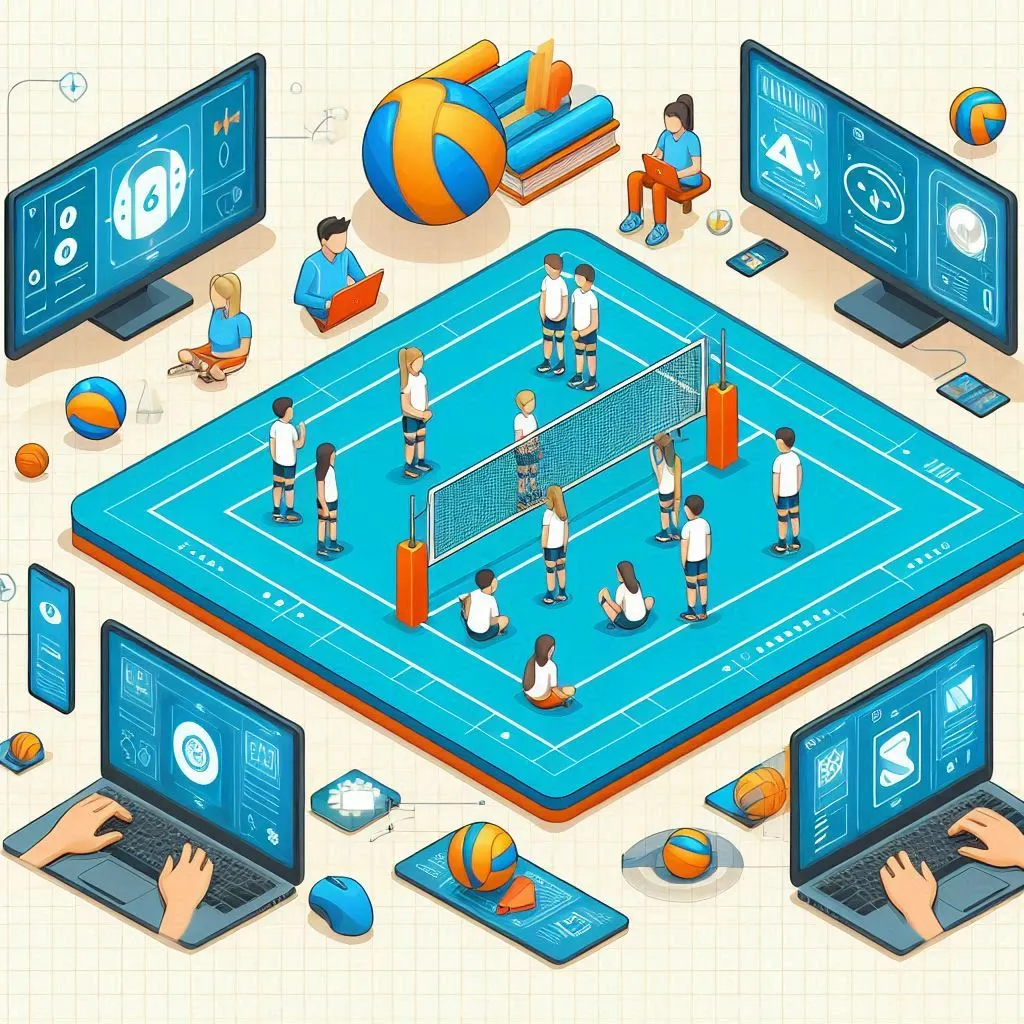
Game Dynamics:
- Two teams, A and B, are playing volleyball.
- Team A always starts serving.
- A team earns a point only if it is serving and wins the rally.
- If the non-serving team wins the rally, it gains the right to serve but doesn’t score a point.
- The game is won by the first team to reach 5 points, but they must win by at least a 2-point margin.
- The game continues until one team has a 2-point lead and at least 5 points.
Input:
A sequence of strings representing which team won each rally (either "A" or "B").
Output:
- The program should print the start of the match.
- For each rally, indicate which team served, which team won, and the score after the rally.
- Print the final result when a team wins.
Pathway to Finding a Solution
1. Parsing the Input
The input will typically be a sequence of characters, each denoting the winner of a play. This sequence needs to be read and processed in the order it is given.
Example:
plays = ['A', 'B', 'A', 'A', 'A', 'B', 'B', 'A', 'A', 'A']
2. Initializing Variables
Before processing the input, initialize variables to track the state of the game:
- Scores: Maintain scores for both teams.
- Serving Team: Track which team is currently serving.
Example Initialization:
score = {'A': 0, 'B': 0}
serving_team = 'A'
3. Processing Each Play
Iterate through the plays to update scores and handle serving changes. Here’s a step-by-step breakdown:
- Determine the Serving Team: Based on the current state of the game.
- Update Scores: If the serving team wins, increase their score. If not, switch the serving team.
- Check for Match End: Determine if either team has won based on the rules.
Detailed Code Example:
def simulate_match(plays):
score = {'A': 0, 'B': 0}
serving_team = 'A'
print("EMPIEZA")
for play in plays:
if play == serving_team:
# Serving team wins
score[serving_team] += 1
print(f"SACA {serving_team}")
print(f"GANA {serving_team}")
else:
# Serving team loses, change serve
serving_team = 'B' if serving_team == 'A' else 'A'
print(f"SACA {serving_team}")
print(f"GANA {serving_team}")
print(f"A {score['A']} B {score['B']}")
# Check if match is over
if score['A'] >= 5 and score['A'] - score['B'] >= 2:
print("FINAL")
break
elif score['B'] >= 5 and score['B'] - score['A'] >= 2:
print("FINAL")
break
4. Handling Edge Cases
Consider and handle potential edge cases:
- Immediate End of Game: If the input sequence ends before a team wins, ensure the code handles it correctly.
- Unusual Input Sequences: Input sequences where one team consistently wins or loses, affecting the serving team’s continuity.
5. Output Format
Ensure the output matches the required format:
- Start: Print "EMPIEZA" before any other output.
- Play Results: After each play, indicate the serving team, the winner, and the current score.
- End: Print "FINAL" when the match concludes.
Output Example:
EMPIEZA
SACA A
GANA A
A 1 B 0
SACA A
GANA B
A 1 B 0
SACA B
GANA B
A 1 B 1
SACA B
GANA B
A 1 B 2
...
FINAL
Detailed Example
To illustrate the entire process, let’s walk through a detailed example using a specific sequence of plays.
Example Input
A
B
A
A
B
B
A
A
A
B
Steps in Detail
1. Initialize:
score = {'A': 0, 'B': 0}
serving_team = 'A'
2. Process Each Play:
- Play 1: A wins, serving team is A, score: A 1 B 0.
- Play 2: B wins, change serve to B, score: A 1 B 1.
- Play 3: A wins, serving team remains A, score: A 2 B 1.
- Play 4: A wins, serving team remains A, score: A 3 B 1.
- Play 5: B wins, change serve to B, score: A 3 B 2.
- Play 6: B wins, serving team remains B, score: A 3 B 3.
- Play 7: A wins, change serve to A, score: A 4 B 3.
- Play 8: A wins, serving team remains A, score: A 5 B 3.
- Play 9: The match ends as A has at least 5 points and a 2-point lead.
Final Output
EMPIEZA
SACA A
GANA A
A 1 B 0
SACA A
GANA B
A 1 B 1
SACA B
GANA B
A 1 B 2
SACA B
GANA A
A 2 B 2
SACA A
GANA A
A 3 B 2
SACA A
GANA A
A 4 B 2
SACA A
GANA A
A 5 B 2
FINAL
Advanced Considerations
Scalability
For large inputs or more complex games, consider optimizing the simulation:
- Efficient Data Structures: Use efficient data structures to track scores and serving teams.
- Optimized Algorithms: Ensure the algorithm handles large sequences without excessive computation.
Extending the Framework
This framework can be adapted for various games with different rules:
- Different Scoring Systems: Modify the score tracking and winning conditions based on the game’s rules.
- Multiple Teams: Adapt the logic to handle more than two teams and complex serving rules.
Testing and Debugging
Ensure thorough testing with various scenarios:
- Normal Cases: Standard sequences of plays.
- Edge Cases: Unusual or unexpected sequences, such as continuous wins by one team or very short input sequences.
Conclusion
Python assignments involving simulating a volleyball game can be challenging, but with a systematic approach, you can tackle them successfully. By carefully analyzing the problem, planning your solution, writing clean and efficient code, and thoroughly testing it, you will not only solve the assignment but also enhance your problem-solving skills.
Remember, the key to success in programming assignments lies in understanding the problem, breaking it down into manageable parts, and approaching it methodically. By following the steps outlined in this guide, you’ll be well-equipped to do your programming assignments in your coursework and beyond.
This structured approach will also serve you well in competitive programming, technical interviews, and real-world software development, where problem-solving skills are paramount. Keep practicing, stay curious, and don’t be afraid to experiment with new ideas and techniques.