Claim Your Discount Today
Ring in Christmas and New Year with a special treat from www.programminghomeworkhelp.com! Get 15% off on all programming assignments when you use the code PHHCNY15 for expert assistance. Don’t miss this festive offer—available for a limited time. Start your New Year with academic success and savings. Act now and save!
We Accept
- 1. Understanding the Problem Domain
- Core Concepts
- Objectives
- Inputs and Outputs
- 2. Define Data Structures
- Substance Class
- Chemist Class
- 3. Implement Functionalities
- Loading Substances
- Add Substance
- Display Inventory
- Search for Elements
- Merge Substances
- Calculate Score
- 4. Implement User Interface
- 5. Detailed Explanation
- 1. Data Structures
- 2. Functionalities
- 3. User Interface
- 6. Testing and Debugging
- Incremental Testing
- Handling Edge Cases
- Debugging
- Conclusion
Imagine having the power to create and manage a chemical laboratory entirely through code. A place where elements are discovered, compounds are synthesized, and every aspect of a chemist's work is simulated in a virtual environment. This kind of project not only stretches the limits of programming skills but also offers a unique challenge that blends theoretical knowledge with practical application.
In this blog, we will delve into a C++ programming assignment that focuses on designing and implementing a sophisticated chemical laboratory simulation. This project requires more than just a grasp of coding—it demands a thorough understanding of the underlying concepts and a methodical approach to problem-solving. Whether you're an experienced developer or tackling your first C++ assignment, building a chemical laboratory simulation can be a rewarding experience that sharpens your technical abilities and expands your creative horizons.
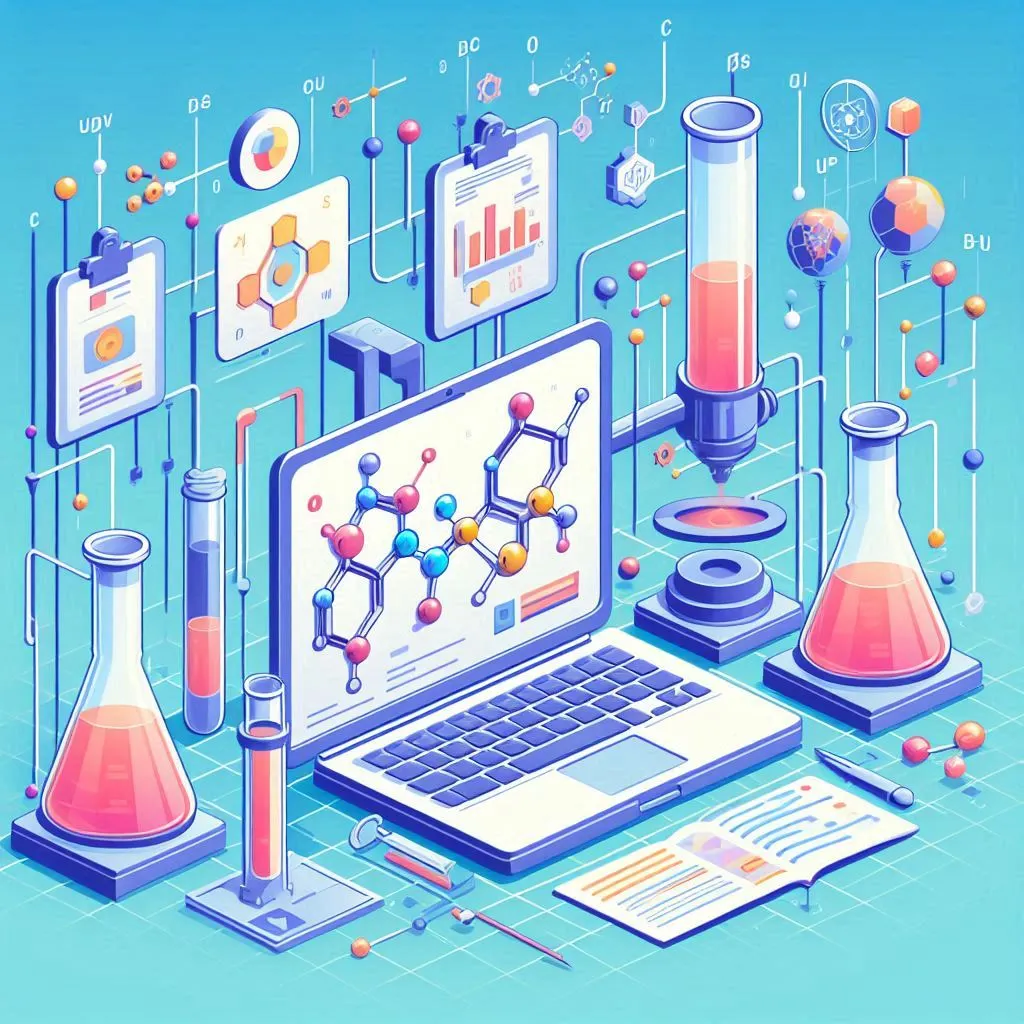
We’ll guide you through the entire process, exploring key concepts such as elements, compounds, and chemical bonds. We'll define crucial data structures, implement essential functionalities, and develop an intuitive user interface. Along the way, we'll share best practices for testing and debugging to ensure your simulation operates seamlessly.
Join us as we break down the steps and strategies for crafting a virtual chemical laboratory in C++, and unlock the secrets to tackling complex programming challenges with confidence and creativity.
1. Understanding the Problem Domain
Core Concepts
To effectively approach this assignment, you need to understand the core concepts involved in the simulation:
- Elements: Fundamental substances that can be found through searching or discovery. They are the building blocks for all other substances.
- Compounds: Substances formed by combining two or more elements or existing compounds. Compounds require a specific combination of elements and/or compounds to be created.
- Chemical Bonds: The forces holding atoms together in a compound. There are primary bonds (ionic, covalent, metallic) and secondary bonds (dipole-dipole interactions, hydrogen bonds, etc.). However, for this assignment, focus on primary bonds as they dictate how substances combine.
Objectives
The primary objectives are:
- To find elements randomly.
- To create compounds by combining elements or other compounds.
- To manage a chemist's inventory and update it based on actions (finding elements, creating compounds).
- To calculate and display the percentage of substances the chemist has discovered relative to the total available.
Inputs and Outputs
- Input File: Contains data about substances including their names, types (element or compound), formulas, and components required to create them. Example format:
Name,Type,Formula,Component1,Component2
- Outputs: Includes displaying the chemist’s current inventory, searching for elements, merging substances, and showing the overall score.
2. Define Data Structures
Substance Class
We need a Substance class to represent elements and compounds. Each substance has a name, type (element or compound), formula, and possibly components.
#include <iostream>
#include <fstream>
#include <sstream>
#include <string>
#include <vector>
class Substance {
public:
std::string name;
std::string type; // "element" or "compound"
std::string formula;
std::string component1; // For compounds
std::string component2; // For compounds
Substance() : name(""), type(""), formula(""), component1(""), component2("") {}
Substance(const std::string& n, const std::string& t, const std::string& f,
const std::string& c1 = "", const std::string& c2 = "")
: name(n), type(t), formula(f), component1(c1), component2(c2) {}
};
Chemist Class
The Chemist class manages the inventory of substances and operations like searching for elements and merging substances.
#include <iostream>
#include <fstream>
#include <sstream>
#include <string>
#include <vector>
class Substance {
public:
std::string name;
std::string type; // "element" or "compound"
std::string formula;
std::string component1; // For compounds
std::string component2; // For compounds
Substance() : name(""), type(""), formula(""), component1(""), component2("") {}
Substance(const std::string& n, const std::string& t, const std::string& f,
const std::string& c1 = "", const std::string& c2 = "")
: name(n), type(t), formula(f), component1(c1), component2(c2) {}
};
3. Implement Functionalities
Loading Substances
Read substances from a file. This involves parsing lines to create Substance objects and storing them in the Chemist's list.
void Chemist::loadSubstances(const std::string& filename) {
std::ifstream infile(filename);
std::string line;
substances.clear();
quantities.clear();
numSubstances = 0;
while (std::getline(infile, line)) {
std::stringstream ss(line);
std::string name, type, formula, component1, component2;
std::getline(ss, name, ',');
std::getline(ss, type, ',');
std::getline(ss, formula, ',');
std::getline(ss, component1, ',');
std::getline(ss, component2);
substances.emplace_back(name, type, formula, component1, component2);
quantities.push_back(0); // Initialize quantity to 0
numSubstances++;
}
}
Add Substance
Add substances to the inventory with specified quantities.
void Chemist::addSubstance(const Substance& s, int quantity) {
for (size_t i = 0; i < substances.size(); ++i) {
if (substances[i].name == s.name) {
quantities[i] += quantity;
return;
}
}
// If substance not found, add it
substances.push_back(s);
quantities.push_back(quantity);
}
Display Inventory
Print out all substances and their quantities.
void Chemist::displayInventory() const {
std::cout << "Chemist's Inventory:\n";
for (size_t i = 0; i < substances.size(); ++i) {
std::cout << substances[i].name << " ("
<< substances[i].type << "): "
<< quantities[i] << " units\n";
}
}
Search for Elements
Randomly select an element and add it to the inventory. We use a random number generator for this.
void Chemist::searchForElement() {
std::vector<int> elementIndices;
for (size_t i = 0; i < substances.size(); ++i) {
if (substances[i].type == "element") {
elementIndices.push_back(i);
}
}
if (elementIndices.empty()) {
std::cout << "No elements available for search.\n";
return;
}
int index = elementIndices[rand() % elementIndices.size()];
std::cout << "Found element: " << substances[index].name << "\n";
addSubstance(substances[index], 1);
}
Merge Substances
Combine two substances if they are valid for merging. This requires checking if the selected substances can be merged and then updating the inventory.
void Chemist::mergeSubstances(int index1, int index2) {
if (index1 >= numSubstances || index2 >= numSubstances) {
std::cout << "Invalid substance indices.\n";
return;
}
// Check if substances can be merged
const auto& s1 = substances[index1];
const auto& s2 = substances[index2];
if (s1.type == "element" || s2.type == "element") {
std::cout << "Cannot merge elements directly.\n";
return;
}
// Check if substances are compatible for merging
if (s1.component1 == s2.name || s1.component2 == s2.name ||
s2.component1 == s1.name || s2.component2 == s1.name) {
// Create a new compound or increase the quantity of an existing compound
std::string newName = s1.name + "+" + s2.name; // Example name
std::string newFormula = s1.formula + "+" + s2.formula;
addSubstance(Substance(newName, "compound", newFormula), 1);
std::cout << "Merged substances to create: " << newName << "\n";
} else {
std::cout << "Substances cannot be merged.\n";
}
}
Calculate Score
Calculate the percentage of discovered substances relative to the total number available.
float Chemist::calculateScore() const {
int discoveredCount = 0;
for (size_t i = 0; i < quantities.size(); ++i) {
if (quantities[i] > 0) {
discoveredCount++;
}
}
return (static_cast<float>(discoveredCount) / numSubstances) * 100;
}
4. Implement User Interface
Create a simple menu to interact with the chemist’s laboratory.
int main() {
Chemist chemist;
chemist.loadSubstances("proj2_data.txt"); // Load substances from file
int choice;
do {
std::cout << "\nMenu:\n";
std::cout << "1. Display Inventory\n";
std::cout << "2. Search for Element\n";
std::cout << "3. Merge Substances\n";
std::cout << "4. Calculate Score\n";
std::cout << "5. Exit\n";
std::cin >> choice;
switch (choice) {
case 1:
chemist.displayInventory();
break;
case 2:
chemist.searchForElement();
break;
case 3: {
int index1, index2;
std::cout << "Enter indices of substances to merge: ";
std::cin >> index1 >> index2;
chemist.mergeSubstances(index1, index2);
break;
}
case 4:
std::cout << "Score: " << chemist.calculateScore() << "%\n";
break;
case 5:
std::cout << "Exiting...\n";
break;
default:
std::cout << "Invalid choice. Try again.\n";
break;
}
} while (choice != 5);
return 0;
}
5. Detailed Explanation
1. Data Structures
- Substance Class: Represents both elements and compounds. It includes attributes like name, type, formula, and component1/component2 for compounds.
- Chemist Class: Manages substances and their quantities. It includes methods to load substances from a file, add substances to inventory, display inventory, search for elements, merge substances, and calculate the score.
2. Functionalities
- Loading Substances: Reads from a file and creates Substance objects. Initializes the substances and quantities vectors.
- Add Substance: Updates the quantity of a substance in the inventory. If the substance is not already in inventory, it is added.
- Display Inventory: Prints out all substances and their quantities, showing what the chemist currently has.
- Search for Element: Randomly selects an element and adds it to the inventory. This simulates finding a new element in the laboratory.
- Merge Substances: Combines two substances to form a new compound. Checks if the substances are compatible and updates the inventory with the new compound.
- Calculate Score: Computes the percentage of discovered substances relative to the total number of substances available.
3. User Interface
- Menu: Provides options for the user to interact with the system, including displaying inventory, searching for elements, merging substances, and calculating the score.
6. Testing and Debugging
Incremental Testing
Test individual components of your program to ensure each part works correctly before integrating them. For example:
- Test the file reading function to ensure it correctly parses the input.
- Verify that the search functionality correctly updates the chemist’s inventory.
- Ensure the merging logic accurately updates quantities and inventory.
Handling Edge Cases
Consider edge cases such as:
- Attempting to merge substances that cannot be combined.
- Searching for elements when none are available.
- Handling invalid user input gracefully.
Debugging
Use debugging tools or add print statements to trace the flow of execution and identify issues. This will help ensure that all functionalities work as expected.
Conclusion
Creating a simulation like a chemical laboratory involves understanding the problem domain, designing a robust solution, implementing key features, and thoroughly testing your code. This project is an excellent way to apply concepts from data structure assignments, demonstrating how to manage complex data in a structured and efficient manner. By breaking down the problem into manageable components and following a systematic approach, you can effectively solve your programming assignments and develop a functional and well-structured application. Good luck with your project, and remember that systematic problem-solving and rigorous testing are keys to success in programming.