Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 10% off on your all programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
- 1. Understanding the Problem Statement
- 2. Design the Solution
- Algorithm Selection:
- Data Structures:
- Modular Design:
- Testing and Validation:
- 3. Implement the Solution in Lisp
- Set Up Your Environment:
- Write Modular Code:
- Test Incrementally:
- 4. Document Your Work
- Code Comments:
- Report Preparation:
- 3. Instructions on How to Run Your Code:
- 5. Debugging and Validation
- Debugging:
- Validation:
- 6. Submitting Your Work
- Source Code:
- Submission Format:
- Additional Tips
- Research and Resources:
- Avoiding Plagiarism:
- Conclusion
Programming assignments frequently present intricate and multifaceted challenges that require a methodical and systematic approach to problem-solving. These tasks often involve a diverse range of algorithms, complex puzzles, and advanced logical constructs that can be particularly daunting. This blog is dedicated to offering a comprehensive and detailed framework for tackling such assignments, with a special emphasis on using Common Lisp—a language renowned for its unique capabilities and powerful abstraction techniques. Common Lisp's rich feature set makes it particularly well-suited for solving problems involving recursive algorithms, symbolic computation, and dynamic data structures.
However, the principles and strategies outlined here extend well beyond the confines of Common Lisp, providing valuable insights applicable across various programming languages and paradigms. Whether you’re working with Python, Java, C++, or any other language, the systematic approach discussed will equip you with the skills to break down complex problems, design effective algorithms, and implement solutions efficiently.
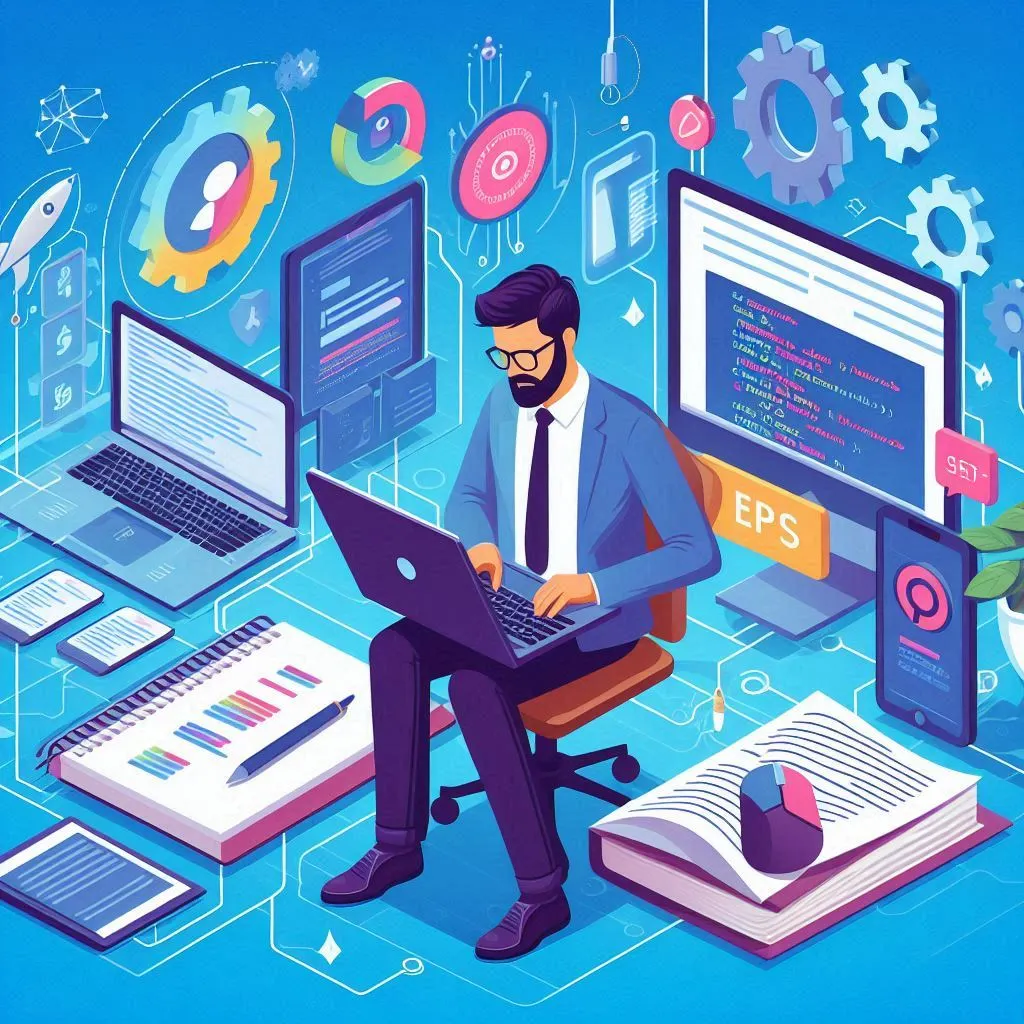
By adopting a structured methodology, students and programmers can enhance their problem-solving capabilities, manage their assignments more effectively, and develop a deeper understanding of the underlying principles that govern algorithm design and implementation. This blog will guide you through a step-by-step approach to mastering assignments that demand a blend of analytical thinking and programming expertise, offering practical advice and actionable strategies to help you succeed in your academic and professional endeavors. For those seeking additional support, consider leveraging a Lisp programming homework helper to aid in navigating complex tasks. Here’s a comprehensive approach to navigating and excelling in assignments that require a sophisticated interplay of algorithmic thinking and coding proficiency.
1. Understanding the Problem Statement
Before diving into the coding phase, it is crucial to gain a deep and comprehensive understanding of the problem at hand. This foundational step sets the stage for effective problem-solving and implementation. Here's how you can approach this process: If you need further assistance, consider reaching out to a programming homework helper. Gaining a thorough grasp of the problem will enhance your ability to develop a robust solution and make the coding process more efficient and manageable.
- Problem Definition: Begin by clearly defining the core problem you are tasked with solving. This involves understanding the primary objective and the end goal. For instance, the Missionaries and Cannibals problem requires you to devise a strategy for transporting a group of missionaries and cannibals across a river using a boat, ensuring that at no point do the cannibals outnumber the missionaries on either side of the river. Similarly, the 8-Puzzle problem involves rearranging tiles on a 3x3 grid to achieve a specific goal state by sliding tiles into the blank space. Clearly articulating the problem helps you focus on what needs to be accomplished and serves as a blueprint for designing your solution.
- Constraints and Requirements: Identify and document all constraints and requirements that impact the problem. Constraints are limitations or rules that must be adhered to while solving the problem. For example, in the Missionaries and Cannibals problem, the boat has a maximum capacity, and there are restrictions on the number of individuals that can be on the boat or on either side of the river at any given time. These constraints ensure that the solution remains feasible and adheres to the problem’s rules. Similarly, for the 8-Puzzle problem, the constraints include the permissible moves (sliding tiles horizontally or vertically) and the requirement to reach a goal state from a given start state. Understanding these constraints helps in formulating a viable approach and avoiding potential pitfalls in your solution.
- Input and Output: Clarify the format of the input data and the expected output. For instance, in the Missionaries and Cannibals problem, the input might include the number of missionaries, cannibals, and the boat’s capacity, while the output would be the sequence of moves required to solve the problem. For the 8-Puzzle problem, the input consists of the initial configuration of the puzzle, and the output is the sequence of moves to reach the goal configuration. Knowing the format of the input and output helps in designing appropriate data structures and functions for processing and generating results.
- Edge Cases and Exceptions: Consider any potential edge cases or exceptions that might arise. These are unusual or boundary scenarios that could affect the solution’s accuracy. For example, what happens if there are no missionaries or no cannibals? How should the solution handle an unsolvable puzzle configuration? Addressing these edge cases ensures that your solution is robust and can handle a variety of inputs effectively.
By thoroughly understanding and documenting the problem definition, constraints, requirements, input and output formats, and potential edge cases, you lay a strong foundation for developing a well-structured and effective solution. This comprehensive approach not only helps in devising a strategy but also in implementing and testing your solution with confidence.
2. Design the Solution
Creating a high-level design for your solution is a critical step in addressing complex programming problems. This phase involves outlining a structured approach to solving the problem, selecting appropriate algorithms, and choosing the right data structures. Here’s a detailed look at how to design your solution effectively:
Algorithm Selection:
Selecting the right algorithm is crucial for solving the problem efficiently. Analyze the nature of the problem to determine which algorithm will provide the best balance between performance and accuracy.
- An Algorithm*: For problems like the 8-Puzzle, the A* algorithm is particularly effective. It is a pathfinding algorithm that uses heuristics to estimate the cost of reaching the goal from a given state, making it efficient for solving puzzles and finding the shortest path. Its efficiency comes from combining the benefits of Dijkstra's algorithm (which finds the shortest path) and greedy best-first search (which uses heuristics to guide the search).
- Search Strategies: In other problems, different search strategies might be appropriate. For instance, Depth-First Search (DFS) might be used for exploring all possible solutions in a systematic manner, while Breadth-First Search (BFS) is useful for finding the shortest path in unweighted graphs.
Data Structures:
Choosing the right data structures is essential for efficiently managing and manipulating data. The data structures you select should align with the requirements of the algorithm and the problem’s constraints.
- State Representation: For the 8-Puzzle problem, you can use arrays or lists to represent the puzzle state. Each configuration of the puzzle can be stored as a 2D array or a flat list, with each element corresponding to a tile in the puzzle. This representation makes it easy to perform operations like sliding tiles and checking for goal states.
- Priority Queues: In algorithms like A*, a priority queue is often used to manage the open set of states. This data structure allows you to efficiently retrieve the state with the lowest estimated cost, which is critical for guiding the search towards the optimal solution.
- Graphs and Trees: For problems involving state exploration, such as the Missionaries and Cannibals problem, representing states as nodes in a graph or tree can be useful. Each node represents a possible state of the problem, and edges represent valid transitions between states.
- Hash Tables: To keep track of visited states and avoid redundant computations, hash tables can be used. They allow for efficient lookup and insertion, which is crucial for managing large state spaces and preventing infinite loops or redundant searches.
Modular Design:
Design your solution in a modular fashion by breaking it down into smaller, manageable components. This approach helps in simplifying development and debugging.
- Function Decomposition: Divide the problem into distinct functions or modules, each responsible for a specific task. For example, in the 8-Puzzle problem, you might have separate functions for generating possible moves, evaluating the heuristic, and checking the goal state.
- Encapsulation: Encapsulate related functionalities within classes or modules. For instance, in object-oriented programming, you might create classes for different components of the problem, such as a Puzzle class for managing puzzle states and a Solver class for implementing the algorithm.
Testing and Validation:
Ensure that your design includes provisions for testing and validating your solution.
- Unit Testing: Develop unit tests for individual functions or modules to verify their correctness. Testing components in isolation helps in identifying and fixing issues early.
- Integration Testing: Test the integrated solution to ensure that all components work together as expected. Validate the overall functionality by running test cases that simulate real-world scenarios.
By carefully designing your solution with appropriate algorithms, data structures, and a modular approach, you set a solid foundation for implementing an effective and efficient solution to complex programming problems. This thoughtful design phase not only enhances the quality of your code but also improves your ability to tackle similar challenges in the future.
3. Implement the Solution in Lisp
When implementing your solution in Common Lisp, it's important to follow a structured approach to ensure that your code is both functional and efficient. Here’s a detailed breakdown of the steps you should take:
Set Up Your Environment:
- Install and Configure Common Lisp: Begin by ensuring that you have a Common Lisp environment properly set up. Common Lisp implementations such as CLISP, SBCL (Steel Bank Common Lisp), or Allegro CL can be used. Install the chosen implementation and configure your environment to facilitate coding and debugging.
- Choose an Integrated Development Environment (IDE): Select an IDE or editor that supports Common Lisp. Emacs with SLIME (Superior Lisp Interaction Mode), LispWorks, and Portacle are popular choices that offer features like syntax highlighting, debugging, and REPL integration.
- Verify Installation: Run basic Lisp commands to verify that your environment is correctly set up and functioning. This can include simple operations like evaluating expressions and running test scripts.
Write Modular Code:
- Function Decomposition: Structure your code by breaking it down into discrete functions or modules. This modular approach simplifies development and debugging. For example, in the Missionaries and Cannibals problem, you might create the following functions:
- move-people: Handles the logic for moving individuals across the river.
- check-constraints: Ensures that the movement adheres to the problem’s constraints.
- generate-moves: Produces all possible valid moves from a given state.
- Encapsulation: Use Lisp’s ability to define functions and macros to encapsulate related functionalities. This makes your code more organized and easier to manage. For instance, encapsulate state management within a class or structure if your solution involves complex state handling.
- Data Structures: Utilize Lisp’s built-in data structures such as lists, arrays, and hash tables effectively. For example, use lists to represent states and arrays to manage the 8-Puzzle board configuration.
- Error Handling: Incorporate error handling to manage unexpected situations and invalid inputs gracefully. Use Common Lisp’s condition system to handle errors and exceptions effectively.
Test Incrementally:
- Unit Testing: Develop and execute unit tests for individual functions or components. Start by testing small, isolated pieces of functionality to ensure they work as intended. For example, test the generate-moves function separately to verify that it correctly produces all valid moves.
- Integration Testing: Once individual functions are validated, test them in combination to ensure they work together seamlessly. For example, test the interaction between move-people and check-constraints to ensure that moves are valid and constraints are properly enforced.
- Debugging: Use debugging tools and techniques available in your Common Lisp environment to identify and fix issues. Common Lisp environments typically offer debugging features like breakpoints, step-through execution, and variable inspection.
- Test Cases and Validation: Create a set of test cases that cover various scenarios, including edge cases and typical use cases. Validate your solution against these test cases to ensure that it produces correct results and behaves as expected under different conditions.
- Iterative Refinement: Continuously refine and improve your code based on test results and feedback. Iteratively add features, enhance performance, and resolve any issues that arise during testing.
By following these steps, you ensure a well-organized and effective implementation of your solution in Common Lisp. Modular code, incremental testing, and a solid development environment will contribute to the success of your programming assignments and projects.
4. Document Your Work
Proper documentation is essential for conveying the purpose, functionality, and usage of your code. It aids in understanding, maintaining, and troubleshooting the solution. Here’s how to effectively document your work:
Code Comments:
1. Purpose and Functionality: Provide comments that explain the overall purpose of your functions and modules. For example, if you have a function that generates valid moves for the Missionaries and Cannibals problem, include a comment describing its role and the logic behind it.
;; Generates all possible moves from the current state.
;; Moves include combinations of missionaries and cannibals
;; moving across the river, while adhering to constraints.
(defun generate-moves (state)
;; Function implementation here
)
2. Non-Obvious Logic: Document any complex or non-intuitive logic within your code. This helps others (and future you) understand why certain decisions were made or how specific parts of the code function.
;; Checks if the current state is valid by ensuring that
;; the number of cannibals does not exceed the number of
;; missionaries on either side of the river.
(defun check-constraints (state)
;; Function implementation here
)
3. Assumptions and Constraints: Clearly state any assumptions made and constraints considered while developing your solution. This can be particularly useful for understanding the boundaries within which your code operates.
;; Assumes that the boat can carry a maximum of two people.
;; Constraints ensure that cannibals do not outnumber missionaries
;; on either side of the river.
4. Function Usage: Include comments on how each function should be used, including parameters and return values.
;; Moves the specified number of missionaries and cannibals
;; from the current side of the river to the other side.
;; Parameters:
;; - missionaries: number of missionaries to move
;; - cannibals: number of cannibals to move
;; Returns: New state after the move
(defun move-people (missionaries cannibals)
;; Function implementation here
)
Report Preparation:
1. Description of the Implemented Solution:
Provide a comprehensive overview of your solution. Describe the problem you are addressing and outline the approach you took to solve it. Include details on the algorithms used, data structures chosen, and how the overall design supports the problem requirements.
Example:
The implemented solution addresses the Missionaries and Cannibals problem by utilizing a breadth-first search algorithm to explore possible states and transitions. The solution ensures that the constraints are met at each step, and the sequence of moves is generated to successfully transport all individuals across the river. The code uses lists to represent the state and hash tables to track visited states, ensuring efficient performance.
2. Known Issues or Limitations:
Document any issues or limitations of your solution. This can include performance concerns, edge cases that are not handled, or known bugs. Providing this information helps in understanding the constraints of your solution and areas where further improvements might be needed.
Example:
Known issues:
- The solution currently does not handle scenarios with more than 20 missionaries and 20 cannibals efficiently due to memory constraints.
- The program may enter an infinite loop if the heuristic function for the A* algorithm is not properly implemented.
3. Instructions on How to Run Your Code:
Provide clear and concise instructions for running your code. Include details on how to compile and execute the program, as well as any necessary setup steps. Ensure that anyone using your code can easily follow these instructions to replicate your results.
Example:
To run the Missionaries and Cannibals solution, follow these steps:
- Compile the source code using CLISP with the command: `clisp -compile mission-cannibals.lisp`
- Execute the program using the command: `clisp mission-cannibals.lisp`
- The output will be displayed in the terminal, showing the sequence of moves.
- 4. Output Examples:
Include examples of the expected output to demonstrate the functionality of your code. This helps users understand what results to expect and verifies that the solution is working correctly.
Example:
Example Output:
Move 1: Boat moves with 2 missionaries
Move 2: Boat returns with 1 missionary
Move 3: Boat moves with 2 cannibals
...
By providing detailed comments in your code and preparing a thorough report, you make your work more accessible and understandable to others. Good documentation also enhances maintainability and supports future modifications or debugging efforts.
5. Debugging and Validation
Ensuring that your solution is both correct and efficient involves a rigorous process of debugging and validation. Here’s how to approach these critical steps:
Debugging:
1. Use Debugging Tools:
- Integrated Debuggers: Utilize the debugging features available in your Common Lisp environment or IDE. These tools can help you set breakpoints, step through code, and inspect variable values during execution.
- Interactive Development: Leverage the REPL (Read-Eval-Print Loop) to interactively test and debug small portions of your code. This allows for immediate feedback and rapid iteration.
2. Print Statements:
- Trace Outputs: Insert print statements or logging calls to track the flow of execution and monitor variable values. For instance, print the state of the puzzle at each step to ensure the transitions are correct.
- Error Messages: Use informative print statements to output error messages or unexpected values, which can help in diagnosing issues.
;; Example of debugging output
(print (format nil "Current state: ~a" state))
3. Validate Functions Independently:
- Unit Testing: Test each function or module in isolation to ensure it performs as expected. For example, validate the move-people function independently to ensure it correctly updates the state.
- Edge Cases: Test your functions with edge cases and unusual inputs to verify robustness and correctness.
Validation:
1. Compare Results with Expected Outputs:
- Known Solutions: Compare the results of your program with known solutions or benchmarks. For the Missionaries and Cannibals problem, ensure that the solution correctly transports all individuals without violating constraints.
- Output Consistency: Validate that your program’s output matches the expected format and values. For instance, check that the sequence of moves is logically sound and meets the problem’s requirements.
2. Test Against Various Scenarios:
- Test Cases: Run your code with a variety of test cases to cover different scenarios, including normal cases, edge cases, and boundary conditions.
- Performance Testing: Evaluate the performance of your solution with larger inputs or more complex scenarios to ensure it performs efficiently and within acceptable limits.
(defun validate-solution (solution)
;; Check that the solution meets all constraints
(assert (check-constraints solution))
;; Further validation steps here
)
6. Submitting Your Work
Proper submission of your work ensures that your solution is evaluated correctly and that you meet all assignment requirements. Follow these steps to prepare your submission:
Source Code:
1. Organize Code:
- Structure: Ensure that your code is well-organized with clear separation between different modules or functions. Use consistent naming conventions and formatting to enhance readability.
- Comments: Include comments in your code to explain key functionalities and logic, making it easier for reviewers to understand your work.
2. Code Quality:
- Review and Refactor: Review your code for any potential improvements or optimizations. Refactor if necessary to enhance readability and performance.
- Remove Debugging Artifacts: Ensure that any debugging print statements or temporary code are removed before submission.
Submission Format:
1. Prepare the Zip File:
- Directory Structure: Create a directory with your last name and student ID number, as specified in the assignment instructions. Place all relevant files, including source code and reports, into this directory.
- Include All Required Files: Make sure to include all necessary files such as source code files, documentation, and any additional materials requested in the assignment.
2. Create the Zip Archive:
- Compression: Zip the directory into a single archive file. Ensure that the zip file includes all required contents and follows the naming conventions provided in the assignment guidelines.
- Check Submission Requirements: Review any specific submission instructions or guidelines provided by your instructor or institution. Ensure compliance with file formats, naming conventions, and submission platforms.
# Example of creating a zip file on Unix-based systems
zip -r lastname_studentID.zip lastname_studentID/
3. Submit Your Work:
- Upload: Submit the zip file through the specified platform, such as an online submission portal or learning management system.
- Confirm Submission: Verify that your submission was received and confirm that all files are accessible and correctly uploaded.
Additional Tips
To enhance your approach and ensure the success of your programming assignments, consider the following additional tips:
Research and Resources:
1. Textbooks and Academic Materials:
- Foundational Knowledge: Refer to textbooks and academic materials on Common Lisp to build a strong understanding of the language and its features. Books such as "Practical Common Lisp" by Peter Seibel or "Common Lisp: A Gentle Introduction to Symbolic Computation" by David S. Touretzky can be invaluable.
- Problem-Specific Literature: Look into literature that addresses specific problems like the Missionaries and Cannibals or the 8-Puzzle. Understanding classic algorithms and problem-solving techniques can provide valuable insights.
2. Online Tutorials and Documentation:
- Official Documentation: Consult the official Common Lisp documentation for details on language features, functions, and best practices. The Common Lisp HyperSpec is an excellent resource.
- Online Courses: Take advantage of online courses and tutorials that focus on Lisp programming or problem-solving strategies. Websites like Coursera, Udemy, or Khan Academy offer relevant courses.
- Video Tutorials: Platforms like YouTube have tutorials and lectures on Common Lisp and algorithms. These can provide visual explanations and practical examples.
3. Forums and Community Support:
- Online Forums: Engage with programming communities on forums such as Stack Overflow, Reddit, or Lisp-related discussion groups. Asking questions and discussing with peers can help clarify doubts and provide new perspectives.
- Lisp Communities: Join Lisp-specific forums and mailing lists, such as the Common Lisp Forum or the Lisp subreddit, to connect with other Lisp programmers and gain insights from their experiences.
Avoiding Plagiarism:
1. Original Code Development:
- Write Your Own Code: Develop your own code to solve the problems, ensuring that it reflects your understanding and skills. Use resources to learn and understand concepts but avoid copying code verbatim.
- Build from Scratch: Start from scratch when implementing algorithms and solutions. This helps you learn the underlying principles and avoid accidental plagiarism.
2. Citing Sources:
- Proper Attribution: If you refer to external sources for ideas or concepts, properly cite them in your report. This demonstrates academic integrity and acknowledges the contributions of others.
- Learning and Applying: Use resources to learn and apply concepts rather than copying code. For example, if you understand the A* algorithm from a tutorial, implement it yourself to reinforce your learning.
3. Understand and Implement:
- Comprehend Concepts: Make sure you thoroughly understand the concepts and algorithms before implementing them. This helps you write original code and apply the knowledge effectively.
- Practice Coding: Regular practice and coding exercises can help you become more proficient and reduce the temptation to copy code. Work on similar problems to strengthen your problem-solving skills.
4. Checking for Plagiarism:
- Plagiarism Detection Tools: Some educational institutions use plagiarism detection tools to ensure originality. Familiarize yourself with these tools and check your work if needed.
- Code Reviews: Perform self-reviews or peer reviews to ensure that your code is original and adheres to academic integrity standards.
By leveraging resources effectively and ensuring originality in your work, you can enhance your understanding and performance in programming assignments. These practices not only contribute to academic success but also help you develop strong programming skills and ethical standards.
Conclusion
Successfully navigating programming assignments, particularly those involving complex algorithms and puzzles, demands a comprehensive approach that combines understanding, design, implementation, and validation. By following a structured methodology—starting with a thorough comprehension of the problem, designing a robust solution, implementing it effectively in Lisp, and documenting your work meticulously—you set yourself up for success. Debugging and validating your solution ensures its correctness and efficiency, while proper preparation and submission align with academic guidelines.
Additionally, leveraging research and resources can greatly enhance your understanding and problem-solving skills. Using textbooks, online tutorials, and community forums provides valuable insights and support throughout your assignment. At the same time, maintaining academic integrity by writing original code and properly citing sources helps uphold ethical standards in your work.
In conclusion, the combination of a systematic approach, effective use of resources, and adherence to academic principles not only helps in completing assignments but also fosters a deeper understanding of programming concepts. This approach not only prepares you for academic success but also equips you with the skills necessary for real-world problem-solving in the field of programming. Embracing these practices will enable you to tackle assignments with confidence, creativity, and integrity, ultimately contributing to your growth as a skilled and ethical programmer.