Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 10% off on your all programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
- Understanding the Assignment
- Using Syscalls for Input and Output
- Implementing Mathematical Functions
- Structuring Your Program
- Building and Linking the Program
- Compiling Assembly Code
- Compiling C/C++ Code
- Linking the Object Files
- Conclusion
Assembly language programming can seem daunting at first, but with practice and understanding, it becomes a valuable skill. This blog aims to guide you through the process of tackling assignments involving computation in assembly language, specifically those requiring the use of syscalls for I/O operations and custom mathematical functions like sin(x). By breaking down complex problems into manageable steps, you can gain confidence and proficiency in assembly language. You'll learn how to use syscalls to handle input and output, ensuring that your program can interact with users and display results effectively. Additionally, implementing mathematical functions, such as the sine function using the Taylor series expansion, will deepen your understanding of both mathematics and low-level programming. These skills are not only crucial for completing specific assignments but also provide a solid foundation for understanding how computers execute instructions at the hardware level. As you progress, you'll find that the seemingly cryptic syntax of assembly language becomes more intuitive, enabling you to write more efficient and optimized code. This blog will provide practical examples and detailed explanations to help you master these concepts, making assembly language a powerful tool in your programming repertoire. With dedication and practice, you'll be able to tackle any assembly language assignment with confidence. Mastering these techniques will not only help you complete your assembly language assignment efficiently but also pave the way for advanced learning and application in computer science.
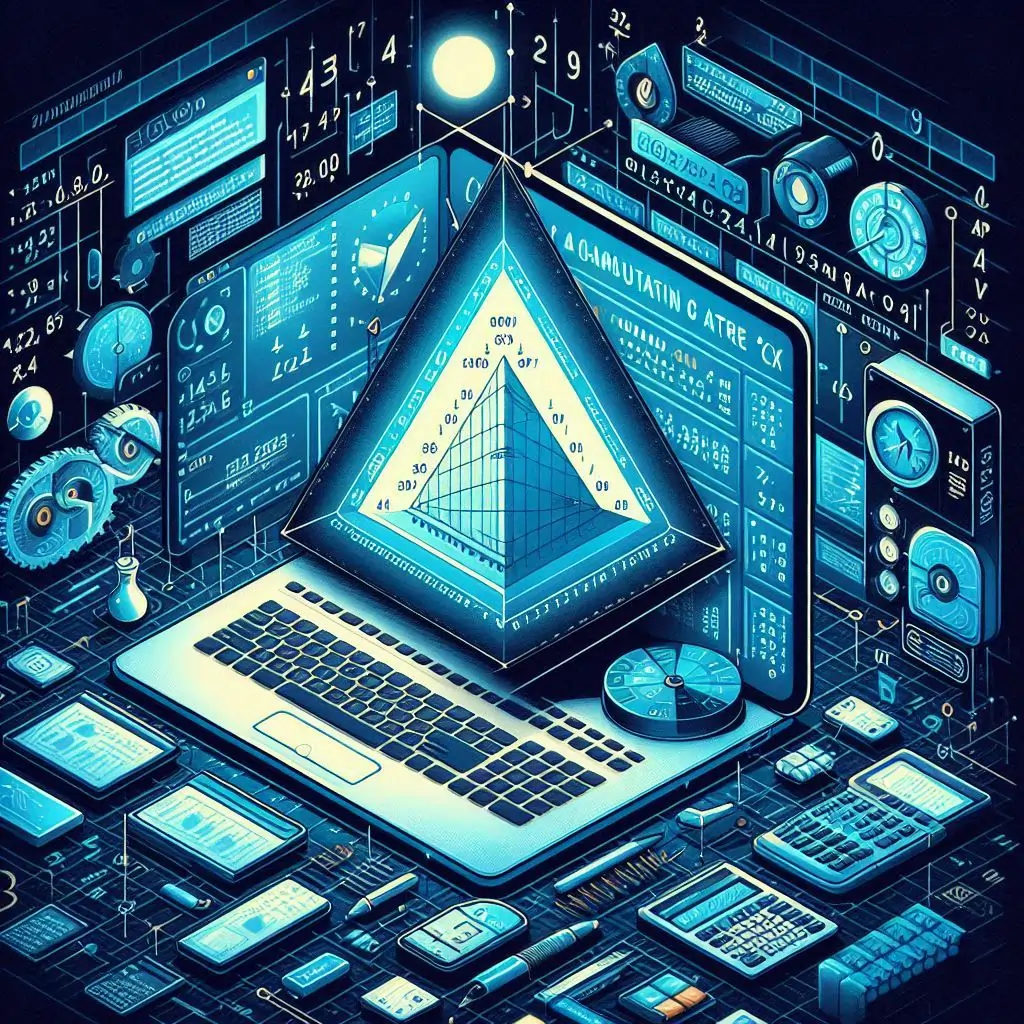
Understanding the Assignment
Programming Assignments that require you to compute the area of a triangle in assembly language typically involve several key tasks. You need to use syscalls for input and output operations, and you must implement mathematical functions such as the sine function directly in assembly. This section will break down the assignment into smaller, manageable steps.
Using Syscalls for Input and Output
Syscalls, or system calls, are a way for your program to interact with the operating system. In assembly language, syscalls are used to perform input and output operations. This is crucial for any program that requires user interaction or needs to display results.
Syscalls for Outputting Strings
To output strings in assembly language, you need to use the sys_write syscall. Here's a basic example of how to output a string:
section .data
msg db 'Hello, World!', 0 ; Null-terminated string
section .text
global _start
_start:
mov eax, 4 ; syscall number for sys_write
mov ebx, 1 ; file descriptor 1 (stdout)
mov ecx, msg ; pointer to message
mov edx, 13 ; message length
int 0x80 ; call kernel
mov eax, 1 ; syscall number for sys_exit
xor ebx, ebx ; exit code 0
int 0x80 ; call kernel
In this example, the message "Hello, World!" is stored in the data section. The syscall for writing (sys_write) is invoked by moving the appropriate values into the registers: eax for the syscall number, ebx for the file descriptor, ecx for the message pointer, and edx for the message length. Finally, an interrupt (int 0x80) is called to execute the syscall.
Syscalls for Reading Input
Reading input in assembly is handled by the sys_read syscall. Here's an example:
section .bss
buffer resb 128 ; buffer for input
section .text
global _start
_start:
mov eax, 3 ; syscall number for sys_read
mov ebx, 0 ; file descriptor 0 (stdin)
mov ecx, buffer ; pointer to buffer
mov edx, 128 ; buffer size
int 0x80 ; call kernel
; Additional code to process input
mov eax, 1 ; syscall number for sys_exit
xor ebx, ebx ; exit code 0
int 0x80 ; call kernel
In this code, a buffer is reserved in the bss section to store the input. The sys_read syscall reads from the standard input (stdin) into this buffer. The same interrupt mechanism (int 0x80) is used to execute the syscall.
Combining Input and Output
Combining both reading and writing operations allows you to create interactive programs. For example, you can prompt the user for input and then display a response based on the input received. This is crucial for assignments where user interaction is required.
Implementing Mathematical Functions
For tasks that require mathematical computations, such as computing the sine of an angle, you can implement these functions directly in assembly. Using the Taylor series expansion is a common method for approximating functions like sin(x).
The Taylor Series Expansion
The Taylor series expansion for sin(x) is: sin(x)=x−x33!+x55!−x77!+⋯\sin(x) = x - \frac{x^3}{3!} + \frac{x^5}{5!} - \frac{x^7}{7!} + \cdotssin(x)=x−3!x3+5!x5−7!x7+⋯
This series provides a way to approximate the sine function to a desired level of precision by summing a finite number of terms.
Implementing Sine Function in Assembly
To implement the sine function in assembly, you start by initializing the result and iteratively calculating each term of the series. Here's a simplified version:
section .data
pi dd 3.14159265358979323846
precision dd 20
section .text
global sin
sin:
; Assume x is passed in st0
; Initialize result
fldz ; st0 = 0 (result)
; Perform the Taylor series calculation
; (For simplicity, only a few terms are shown here)
fld st1 ; st0 = x, st1 = x
fadd ; st0 = x + 0 (initial term)
; Next term - x^3/3!
fld st1 ; st0 = x, st1 = x, st2 = x
fmul st0, st1 ; st0 = x^2, st1 = x, st2 = x
fmul st0, st1 ; st0 = x^3, st1 = x, st2 = x
fidiv dword [factorial_3] ; st0 = x^3/3!, st1 = x, st2 = x
fsub ; st0 = x - x^3/3!
; Continue adding more terms for higher precision
; Return result in st0
ret
section .data
factorial_3 dd 6
; Add more factorials for higher terms
This example shows the basic structure for calculating sin(x) using the Taylor series. You can extend this to include more terms for higher precision.
Structuring Your Program
Dividing your program into distinct modules helps manage complexity and improves readability. Here’s how you can structure your program for computing the area of a triangle:
Director Module
The Director module, often written in C or C++, handles the main workflow and user interaction. It prompts the user for input, calls the Producer module for computation, and displays the results.
Producer Module
The Producer module, written in assembly, handles the core logic. It uses syscalls for input and output, calls the sine function, and computes the area of the triangle. The formula for the area of a triangle given two sides aaa and bbb and the angle CCC between them is: Area=12absin(C)\text{Area} = \frac{1}{2}ab \sin(C)Area=21absin(C)
Sine Function Module
The Sine Function module, also in assembly, implements the sine function using the Taylor series expansion. This module is called by the Producer module to compute the sine of the given angle.
Building and Linking the Program
Once you have your modules, you need to compile and link them. This can be done using tools like nasm for assembling the assembly code and gcc or g++ for linking.
Compiling Assembly Code
Use nasm to compile your assembly modules:
nasm -f elf32 producer.asm -o producer.o
nasm -f elf32 sin.asm -o sin.o
Compiling C/C++ Code
If your Director module is in C or C++, compile it using gcc or g++:
gcc -m32 -c director.c -o director.o
Linking the Object Files
Link the object files together to create the final executable:
gcc -m32 director.o producer.o sin.o -o triangle_area
This command links the object files and produces an executable named triangle_area.
Conclusion
Mastering assembly language programming requires breaking down problems into manageable pieces and understanding core concepts like syscalls and mathematical computations. By practicing regularly, you will find that working with assembly becomes more intuitive over time. Don't hesitate to seek help from resources or professionals if you encounter difficulties. Remember, persistence and consistent effort are key to success in assembly programming.
For additional programming assistance, visit ProgrammingHomeworkHelp.com. With dedication and the right support, you'll become proficient in tackling complex assignments.