Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 10% off on your all programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
- Why Choose a Traffic Light Controller for Your Assignment?
- Understanding the Basics of a Traffic Light Controller System
- Step-by-Step Guide to Creating the System
- Step 1: Define the FSM
- Step 2: Determine Inputs and Outputs
- Step 3: Write the Verilog Code
- Step 4: Simulate and Test the System
- Additional Features to Enhance Your Project
- Debugging Tips
- Conclusion
Traffic light controllers are a classic project for students studying digital design and computer engineering. These systems combine concepts like finite state machines (FSM), combinational and sequential logic, and hardware description languages (HDLs) such as Verilog. In this blog, we will dive into how to create a traffic light controller system using Verilog, ideal for your college assignment. If you’re looking for Verilog assignment help or just want to sharpen your skills, this guide is for you.
Why Choose a Traffic Light Controller for Your Assignment?
A traffic light controller is a great choice for a college project because it is both practical and educational. It demonstrates key digital design concepts while being simple enough for beginners to grasp. Moreover, creating this project provides an opportunity to learn how to translate real-world scenarios into code—a skill highly valued in engineering and programming assignments.
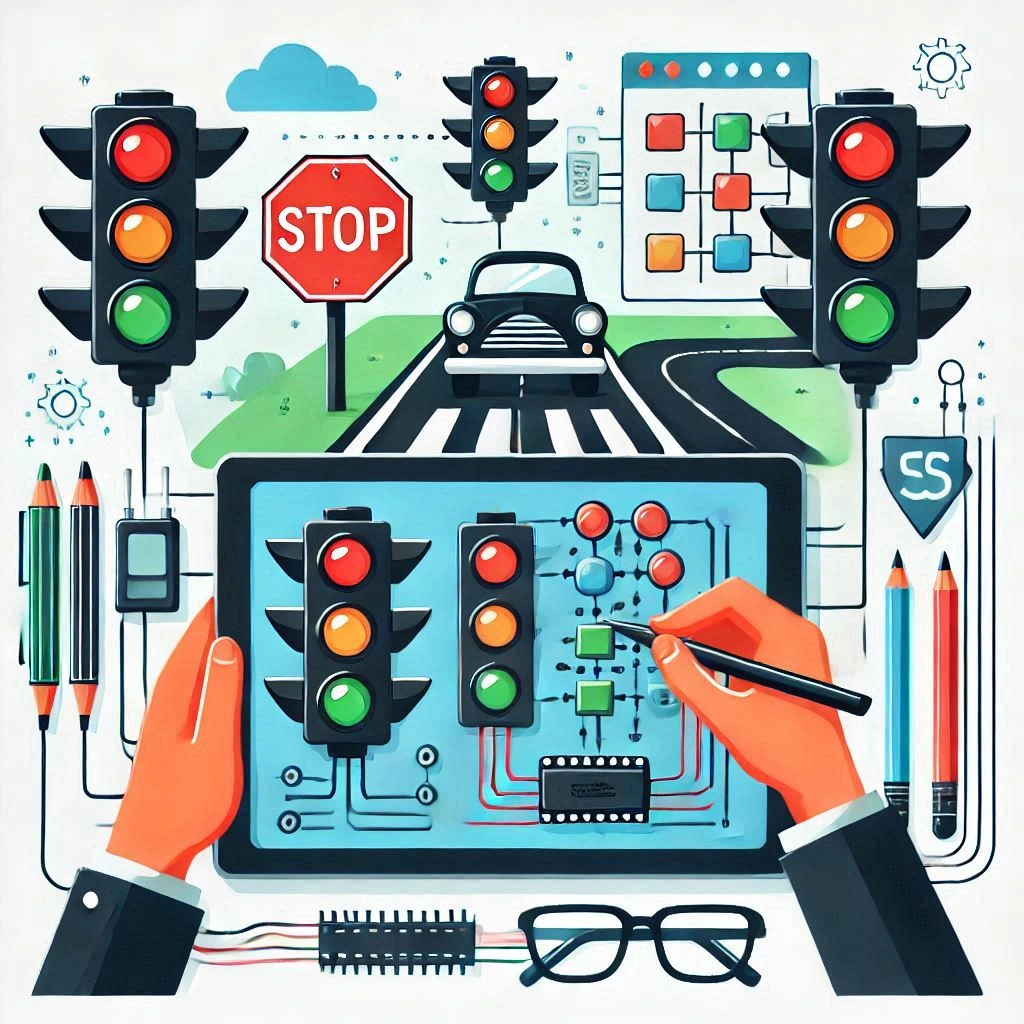
If you’re new to hardware description languages or struggling with concepts, seeking programming assignment help can bridge the gap between theoretical understanding and practical implementation. Now, let’s get started!
Understanding the Basics of a Traffic Light Controller System
Before jumping into the Verilog code, let’s understand what a traffic light controller does. For simplicity, consider a system that manages traffic lights at a four-way intersection. It must:
- Control the red, yellow, and green lights for two directions (e.g., north-south and east-west).
- Allow for smooth transitions between states (e.g., green to yellow, then yellow to red).
- Operate on a time-based system with clear intervals for each light.
- Optionally, include a pedestrian crossing signal or emergency override system.
This behavior can be implemented using a finite state machine (FSM) model, where each state corresponds to a specific light configuration.
Step-by-Step Guide to Creating the System
Step 1: Define the FSM
The FSM for a basic traffic light controller might include the following states:
- State 1 (NS_Green_EW_Red): North-south direction has a green light, and east-west has a red light.
- State 2 (NS_Yellow_EW_Red): North-south direction transitions to yellow, while east-west remains red.
- State 3 (NS_Red_EW_Green): North-south direction has a red light, and east-west has a green light.
- State 4 (NS_Red_EW_Yellow): North-south remains red while east-west transitions to yellow.
Each state transition is time-based, with specific delays for green, yellow, and red lights.
Step 2: Determine Inputs and Outputs
Inputs:
- Clock: Provides the timing for state transitions.
- Reset: Resets the system to the initial state.
- Sensor (Optional): Detects traffic density to adjust timings dynamically.
Outputs:
- NS_Red, NS_Yellow, NS_Green: Control lights for the north-south direction.
- EW_Red, EW_Yellow, EW_Green: Control lights for the east-west direction.
Step 3: Write the Verilog Code
Here’s an example of a simple Verilog implementation for a traffic light controller:
module Traffic_Light_Controller(
input clk,
input reset,
output reg NS_Red,
output reg NS_Yellow,
output reg NS_Green,
output reg EW_Red,
output reg EW_Yellow,
output reg EW_Green
);
// State encoding
typedef enum logic [1:0] {
STATE_NS_GREEN_EW_RED = 2'b00,
STATE_NS_YELLOW_EW_RED = 2'b01,
STATE_NS_RED_EW_GREEN = 2'b10,
STATE_NS_RED_EW_YELLOW = 2'b11
} state_t;
state_t current_state, next_state;
// Timing counters
reg [3:0] timer;
// Parameters for time intervals
parameter GREEN_TIME = 10;
parameter YELLOW_TIME = 3;
parameter RED_TIME = 10;
// State transition logic
always @(posedge clk or posedge reset) begin
if (reset) begin
current_state <= STATE_NS_GREEN_EW_RED;
timer <= 0;
end else begin
if (timer == 0) begin
current_state <= next_state;
case (current_state)
STATE_NS_GREEN_EW_RED: timer <= GREEN_TIME;
STATE_NS_YELLOW_EW_RED: timer <= YELLOW_TIME;
STATE_NS_RED_EW_GREEN: timer <= GREEN_TIME;
STATE_NS_RED_EW_YELLOW: timer <= YELLOW_TIME;
endcase
end else begin
timer <= timer - 1;
end
end
end
// Next state logic
always @(*) begin
case (current_state)
STATE_NS_GREEN_EW_RED: next_state = STATE_NS_YELLOW_EW_RED;
STATE_NS_YELLOW_EW_RED: next_state = STATE_NS_RED_EW_GREEN;
STATE_NS_RED_EW_GREEN: next_state = STATE_NS_RED_EW_YELLOW;
STATE_NS_RED_EW_YELLOW: next_state = STATE_NS_GREEN_EW_RED;
default: next_state = STATE_NS_GREEN_EW_RED;
endcase
end
// Output logic
always @(*) begin
// Default all outputs to 0
NS_Red = 0; NS_Yellow = 0; NS_Green = 0;
EW_Red = 0; EW_Yellow = 0; EW_Green = 0;
case (current_state)
STATE_NS_GREEN_EW_RED: begin
NS_Green = 1;
EW_Red = 1;
end
STATE_NS_YELLOW_EW_RED: begin
NS_Yellow = 1;
EW_Red = 1;
end
STATE_NS_RED_EW_GREEN: begin
NS_Red = 1;
EW_Green = 1;
end
STATE_NS_RED_EW_YELLOW: begin
NS_Red = 1;
EW_Yellow = 1;
end
endcase
end
endmodule
Step 4: Simulate and Test the System
Simulation is crucial for verifying the functionality of your traffic light controller. Use tools like ModelSim or Xilinx Vivado to simulate the Verilog code. Here’s an example testbench:
module Traffic_Light_Controller_tb;
reg clk;
reg reset;
wire NS_Red, NS_Yellow, NS_Green;
wire EW_Red, EW_Yellow, EW_Green;
// Instantiate the module
Traffic_Light_Controller uut (
.clk(clk),
.reset(reset),
.NS_Red(NS_Red),
.NS_Yellow(NS_Yellow),
.NS_Green(NS_Green),
.EW_Red(EW_Red),
.EW_Yellow(EW_Yellow),
.EW_Green(EW_Green)
);
// Clock generation
initial begin
clk = 0;
forever #5 clk = ~clk; // 10 time units period
end
// Test sequence
initial begin
reset = 1;
#10 reset = 0;
#200 $stop; // End simulation after 200 time units
end
endmodule
Additional Features to Enhance Your Project
Once the basic traffic light controller is working, you can add more features to make your assignment stand out:
- Pedestrian Crossing: Add buttons and signals for pedestrians to request crossing.
- Traffic Sensors: Adjust light durations dynamically based on traffic density.
- Emergency Override: Implement an emergency mode that gives priority to one direction.
- Multilane Support: Extend the system to manage multilane intersections.
Debugging Tips
- Check State Transitions: Ensure your FSM transitions match the intended logic.
- Verify Timing: Use simulation waveforms to verify timing intervals.
- Test Edge Cases: Test reset functionality and ensure all outputs behave as expected in every state.
- Seek Help if Needed: If you’re stuck, don’t hesitate to seek Verilog assignment help or consult with peers and professors.
Conclusion
Designing a traffic light controller using Verilog is an excellent way to enhance your HDL skills and gain hands-on experience in digital design. By understanding FSMs, modular coding practices, and simulation techniques, you’ll be well-equipped to handle similar projects in the future. Whether you’re doing this project independently or with programming assignment help, following the structured approach outlined in this guide will set you up for success.