Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 10% off on your all programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
- Understanding Time Complexity
- Part 1: Analyzing Time Complexity in Loops
- General Approach
- Example 1: Sum Every 7th Element
- Example 2: Exponential Growth Loop
- Example 3: Nested Loops
- Part 2: Analyzing Recursive Algorithms
- Example 1: Power of 5
- Example 2: QuickSort Algorithm
- Example 3: Towers of Hanoi
- Conclusion
Understanding time complexity is fundamental for any programmer seeking to optimize their code and solve problems efficiently. When tackling C++ programming assignments, especially those involving time complexity analysis, it's crucial to understand how to evaluate the performance of algorithms. Time complexity analysis helps us determine how the runtime of an algorithm scales with the size of the input. This detailed guide will delve into the principles of time complexity analysis, using a series of data structures and algorithms assignment as examples to illustrate key concepts. We will cover both iterative and recursive algorithms to provide a thorough understanding of how to analyze and evaluate their efficiency.
Understanding Time Complexity
Time Complexity is a measure of the amount of computational time that an algorithm takes as a function of the size of its input. It helps us understand the efficiency of an algorithm and predict how it will perform as the input size grows. The key concepts involved are:
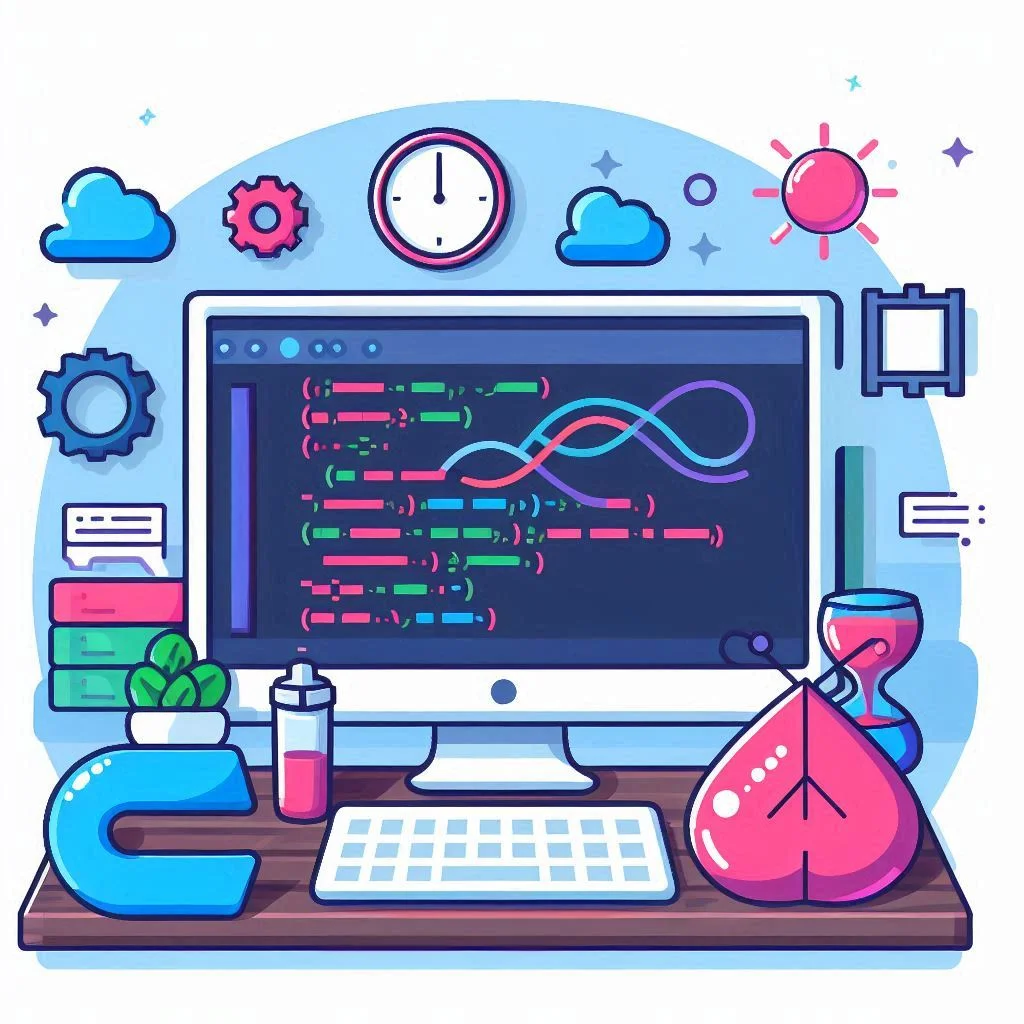
- Big O Notation (O): Describes the upper bound of the time complexity. It provides an asymptotic analysis of the algorithm, focusing on the worst-case scenario.
- Big Theta Notation (Θ): Represents the exact bound of the time complexity, indicating both upper and lower bounds.
- Big Omega Notation (Ω): Describes the lower bound of the time complexity, focusing on the best-case scenario.
Part 1: Analyzing Time Complexity in Loops
For-loops are one of the most common constructs in programming. To analyze their time complexity, follow these steps:
General Approach
- Initialization: Examine how the loop variables are initialized.
- Condition: Determine the condition under which the loop continues to execute.
- Update: Analyze how the loop variables are updated in each iteration.
- Operations Inside Loop: Evaluate the time complexity of operations performed inside the loop.
Loops are one of the most common structures in programming, and understanding their time complexity is crucial for optimizing algorithms.
Example 1: Sum Every 7th Element
Let’s begin with a simple loop that sums every 7th element in an array:
double sum_skip7 (double array[], int n) {
double sum = 0;
for (int i = 0; i < n; i = i + 7)
sum = sum + array[i];
return sum;
}
Analysis:
1. Loop Analysis:
- The loop variable i starts at 0 and increments by 7 in each iteration until it reaches or exceeds n.
- Since i is incremented by 7 in each step, the loop runs n/7 times.
2. Time Taken per Iteration:
- The operation inside the loop (i.e., sum = sum + array[i];) takes constant time, denoted as O(1).
3. Total Time Complexity:
- The total time complexity is calculated by multiplying the time taken per iteration by the number of iterations.
- Number of iterations: n/7.
- Time per iteration: O(1).
- Therefore, the total time complexity is O(n/7), which simplifies to O(n) because constant factors are ignored in Big-O notation.
This example illustrates that even though the loop iterates fewer times due to the increment by 7, the time complexity is still linear, i.e., O(n). This is because Big-O notation abstracts away constant factors, focusing only on how the algorithm scales with input size.
Example 2: Exponential Growth Loop
Consider the following loop that sums powers of 10 up to n:
double sum_exponentials(int n) {
int sum = 0;
for (int i = 1; i < n; i = i * 10)
sum = sum + i;
return sum;
}
Analysis:
1. Loop Analysis:
- The loop variable i starts at 1 and is multiplied by 10 in each iteration.
- The loop runs as long as i is less than n.
2. Number of Iterations:
- The loop iterates for each power of 10 less than n.
- The number of iterations is the number of times you can multiply 1 by 10 before exceeding n, which is log10(n).
3. Time Taken per Iteration:
- The operation inside the loop takes constant time, O(1).
4. Total Time Complexity:
- Number of iterations: O(log10(n)).
- Time per iteration: O(1).
- Therefore, the total time complexity is O(log10(n)), which simplifies to O(log n) since Big-O notation typically abstracts away the base of the logarithm.
This loop demonstrates a logarithmic time complexity, indicating that the algorithm's execution time grows slowly as the input size increases. Logarithmic time complexities are efficient, especially for large inputs.
Example 3: Nested Loops
Nested loops can often lead to higher time complexities. Let’s analyze the following code with nested loops:
for (int i = 0; i < n; i++)
for (int j = n; j > i; j--)
cout << i << "," << j << endl;
Analysis:
1. Outer Loop Analysis:
- The outer loop runs n times, with i taking values from 0 to n-1.
2. Inner Loop Analysis:
- For each value of i, the inner loop runs from j = n down to i + 1.
- The number of iterations for the inner loop decreases as i increases.
3. Total Number of Iterations:
- The total number of operations (or iterations of the inner loop) is the sum of the first n natural numbers:
- Total iterations = (n) + (n-1) + (n-2) + ... + 1 = n(n+1)/2.
- This simplifies to O(n^2) time complexity.
4. Time Complexity:
- Each operation inside the inner loop takes constant time, O(1).
- Therefore, the overall time complexity of the nested loops is O(n^2).
Nested loops, where the number of iterations depends on another loop variable, often result in quadratic time complexity, O(n^2). This means that the algorithm's running time grows rapidly as the input size increases, which can become inefficient for large inputs.
Part 2: Analyzing Recursive Algorithms
Recursive algorithms are a common source of complex time complexities, and they require a different approach for analysis. To analyze the time complexity of recursive algorithms, we often use recurrence relations, which express the time complexity of a problem in terms of smaller instances of the same problem.
Example 1: Power of 5
Consider the following recursive function that calculates powers of 5:
int pow_5(int n) {
if (n == 1)
return 5;
if (n > 1)
return (5 * pow_5(n - 1));
}
Analysis:
1. Base Case:
- The base case is when n == 1, which takes constant time, O(1).
2. Recursive Case:
- The function calls itself with n - 1, and the time complexity of each recursive call includes the time taken by the call itself plus the time taken by all subsequent recursive calls.
3. Recurrence Relation:
- The time complexity can be expressed as T(n) = T(n - 1) + O(1).
4. Solving the Recurrence:
- Expanding the recurrence:
- T(n) = T(n - 1) + O(1)
- T(n - 1) = T(n - 2) + O(1)
- T(n - 2) = T(n - 3) + O(1)
- ...
- T(1) = O(1).
- Adding these up gives:
- T(n) = O(n).
5. Total Time Complexity:
- The function has a linear time complexity, O(n), which means the time required increases linearly with n.
This example demonstrates a simple linear recursion, where the time complexity grows directly with the input size. Recursive functions like this are straightforward to analyze, but more complex recursions require more advanced techniques.
Example 2: QuickSort Algorithm
QuickSort is a widely-used sorting algorithm that relies on the divide-and-conquer strategy. Let's analyze the time complexity of QuickSort in different scenarios.
algorithm quicksort(A, lo, hi) {
if (lo < hi) {
p := partition(A, lo, hi);
quicksort(A, lo, p - 1);
quicksort(A, p + 1, hi);
}
}
1. Partitioning Process:
- The partition function rearranges the elements of the array such that elements smaller than the pivot are on one side and those greater are on the other. The pivot is then placed in its correct position.
- The partitioning itself takes O(n) time because it involves scanning and comparing each element in the array segment being considered.
2. Best Case Analysis:
- Scenario:The pivot always splits the array into two equal halves.
- Recurrence Relation:
- T(n) = 2T(n/2) + O(n).
- Time Complexity:
- This solves to T(n) = O(n log n) using the Master Theorem for Divide and Conquer recurrences.
3. Worst Case Analysis:
- Scenario: The pivot always splits the array such that one side has all but one element, resulting in maximum imbalance.
- Recurrence Relation:
- T(n) = T(n - 1) + O(n).
- Time Complexity:
- This solves to T(n) = O(n^2).
- Explanation:
- The worst-case scenario typically occurs when the array is already sorted (or reverse sorted), causing the partition to perform poorly.
4. 40:60 Partition Analysis:
- Scenario: The partitioning consistently results in a 40:60 split.
- Recurrence Relation:
- T(n) = T(0.4n) + T(0.6n) + O(n).
- Time Complexity:
- This case still results in O(n log n) time complexity, though the constants involved differ.
QuickSort’s time complexity varies significantly depending on how well the pivot divides the array. In the best and average cases, QuickSort is highly efficient with O(n log n) complexity. However, in the worst case, the time complexity can degrade to O(n^2), which makes the choice of the pivot crucial in practical implementations.
Example 3: Towers of Hanoi
The Towers of Hanoi is a classic problem that demonstrates exponential time complexity. The problem involves moving a set of disks from one rod to another, with the constraint that a larger disk can never be placed on top of a smaller one.
Algorithm MoveTower(disk, source, dest, spare) {
IF disk == 0 THEN {
move disk from source to dest;
} ELSE {
MoveTower(disk - 1, source, spare, dest);
move disk from source to dest;
MoveTower(disk - 1, spare, dest, source);
}
}
Analysis:
1. Base Case:
- The base case occurs when there is only one disk (disk == 0). This takes constant time, O(1).
2. Recursive Case:
- The algorithm involves three steps:
- Move n-1 disks from the source to the spare rod.
- Move the nth disk (largest) from the source to the destination rod.
- Move the n-1 disks from the spare rod to the destination rod.
- This recursive process doubles the problem size for each step, plus the time to move the single disk.
3. Recurrence Relation:
- The time complexity can be expressed as T(n) = 2T(n - 1) + O(1).
4. Solving the Recurrence:
- Expanding the recurrence:
- T(n) = 2T(n - 1) + O(1)
- T(n - 1) = 2T(n - 2) + O(1)
- ...
- T(1) = O(1).
- This simplifies to T(n) = O(2^n).
5. Total Time Complexity:
- The time complexity of the Towers of Hanoi problem is O(2^n), indicating that the time required doubles with each additional disk.
This example illustrates exponential time complexity, where the running time grows rapidly with the size of the input. Algorithms with exponential time complexity are generally infeasible for large inputs due to their extremely high time requirements.
Conclusion
Understanding time complexity is fundamental for evaluating and optimizing algorithms. By analyzing loops and recursive algorithms, you can determine how their runtime scales with input size and make informed decisions about which algorithms to use in different scenarios. Whether you're dealing with simple loops or complex recursive functions, a thorough understanding of time complexity will help you write more efficient code and solve programming assignments effectively.