Claim Your Offer
New semester, new challenges—but don’t stress, we’ve got your back! Get expert programming assignment help and breeze through Python, Java, C++, and more with ease. For a limited time, enjoy 10% OFF on all programming assignments with the Spring Special Discount! Just use code SPRING10OFF at checkout! Why stress over deadlines when you can score high effortlessly? Grab this exclusive offer now and make this semester your best one yet!
We Accept
- Understanding the Assignment
- Identifying Key Tasks
- Understanding Constraints
- Focusing on Output
- Planning Your Approach
- Breaking Down the Problem
- Pseudocode First
- Writing the Code
- Class Design and Implementation
- Testing and Debugging
- Optimizing Your Code
- Documenting and Submitting Your Assignment
- Commenting Your Code
- Writing a ReadMe File
- Following Submission Guidelines
- Conclusion
Programming assignments are a staple in any computer science curriculum. They test not only your understanding of theoretical concepts but also your ability to apply these concepts practically to solve real-world problems. One common type of assignment involves text analysis using hash tables, like the one described in the project summary above. Whether you’re seeking help with C++ assignments involving word frequency counts, text parsing, or other forms of data analysis, understanding the underlying principles and approaches is crucial for success. These assignments typically require students to count word frequencies in a text, a task that is fundamental in fields such as linguistics and data analysis. This blog aims to guide you through the process of tackling programming assignments effectively, using a structured approach that will help you understand the problem, plan your solution, write efficient code, and ensure your program meets all requirements.
Understanding the Assignment
Understanding your assignment thoroughly is the first crucial step towards a successful solution. This section will break down the typical requirements and constraints of a text analysis project using hash tables, and provide a roadmap for planning your approach.
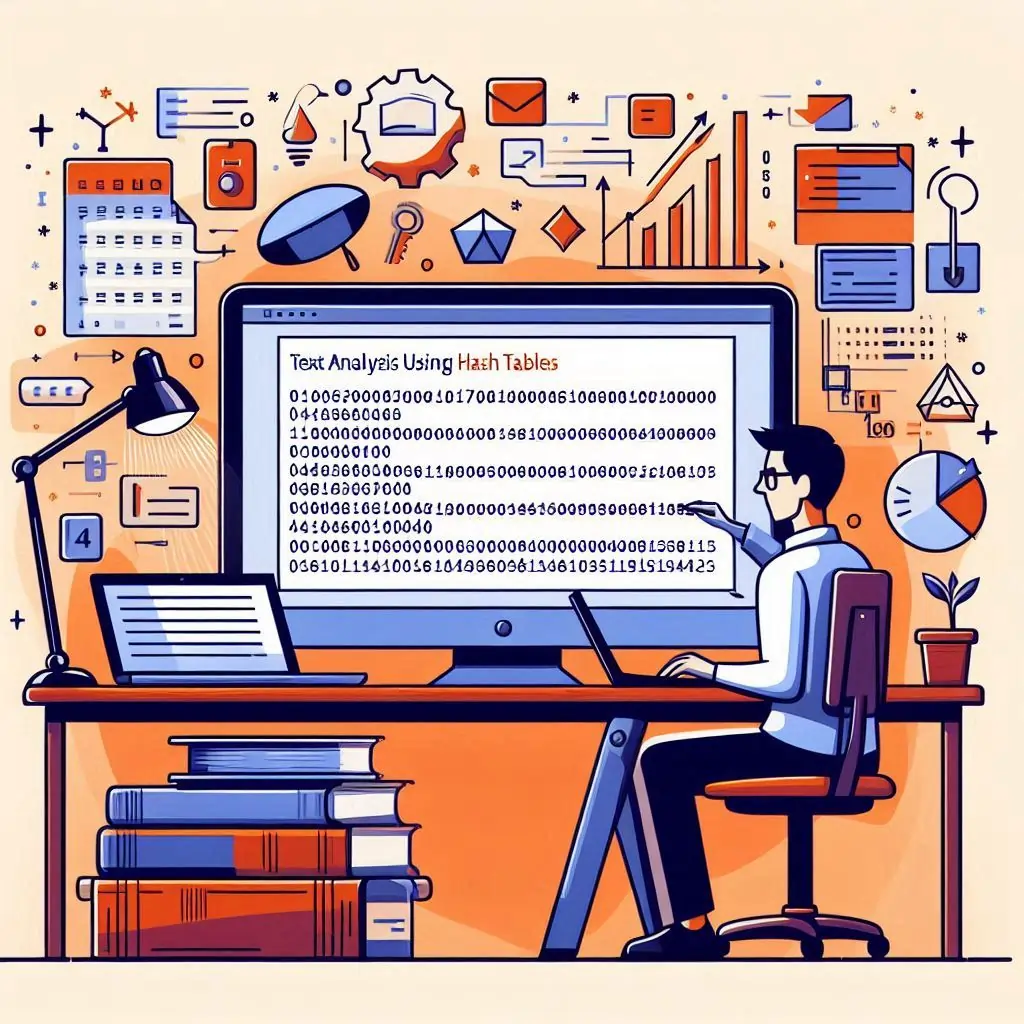
Identifying Key Tasks
When faced with a text analysis project, your primary goal is to understand what the assignment asks you to do. For instance, in a project where you need to count word frequencies, the key tasks may include:
- Reading Input: Your program will need to read text from a file or standard input. The text can be large, so handling file I/O efficiently is important.
- Sanitizing Words: Words must be cleaned of punctuation and other non-alphabetic characters, and converted to a standard format (e.g., lowercase) to ensure accurate counting.
- Counting Frequencies: Each unique word needs to be counted, and the counts stored in a data structure that allows for efficient lookups and updates.
Understanding Constraints
Assignments often come with specific constraints that you must adhere to. These might include:
- Input and Output Requirements: You may be required to read from a specific file or output your results in a particular format, such as a text file with word counts.
- Coding Standards: Certain libraries or functions might be restricted, and coding standards, such as avoiding the using namespace std; directive in C++, may be enforced.
- Efficiency Constraints: You might need to ensure your solution runs within a certain time or memory limit, especially when processing large texts.
Focusing on Output
Knowing what your final output should look like is essential for guiding your solution. In a word frequency assignment, you might be asked to produce a list of words and their counts, or identify the most frequent word. Clear requirements will help you structure your code and validate your results effectively.
Planning Your Approach
Planning is key to managing the complexity of a programming assignment. By breaking down the problem into manageable tasks and outlining a clear strategy, you can approach your coding with confidence.
Breaking Down the Problem
Dividing the assignment into smaller tasks makes it easier to tackle each part methodically. For a text analysis project, you might break down the problem as follows:
Text Input and Processing
- Read Text: Develop a function to read text from a file or input stream.
- Tokenize Words: Split the text into individual words, handling punctuation and case sensitivity.
- Sanitize Words: Clean each word to remove unwanted characters and normalize the case.
Word Counting and Storage
- Initialize Data Structure: Use a hash table (e.g., std::unordered_map in C++) to store word counts.
- Count Words: Iterate over the list of sanitized words, updating the count for each word in the hash table.
- Handle Edge Cases: Ensure your program can handle empty inputs, very large words, or special characters.
Output and Analysis
- Generate Output: Create a function to write the word counts to a file or display them in the required format.
- Identify Most Frequent Word: Implement logic to find and return the word with the highest count.
- Optimize Performance: Consider ways to improve the efficiency of your code, such as reducing memory usage or optimizing loops.
Pseudocode First
Writing pseudocode helps you outline the logic of your solution without worrying about syntax. For example, a pseudocode for the word counting function might look like this:
function countWords(text):
initialize empty hash table wordCounts
for each word in text:
sanitize the word
if word is not empty:
if word is in wordCounts:
increment wordCounts[word]
else:
add word to wordCounts with count 1
return wordCounts
Pseudocode makes it easier to identify logical errors and refine your approach before diving into actual coding.
Writing the Code
Once you have a clear plan, it's time to start coding. Focus on implementing your functions one at a time, testing as you go to ensure correctness. This section will guide you through the core functions typically required in a text analysis project.
Class Design and Implementation
In a project where you analyze text and count word frequencies, you might create a class to encapsulate the logic and data structures used. For instance, a WordFrequency class in C++ could handle reading text, sanitizing words, and counting frequencies.
Text Input and Word Sanitization
Your class might start by implementing functions to read text and sanitize words. Sanitization involves removing unwanted characters and normalizing the case of each word. Here's an example in C++:
#include <iostream>
#include <unordered_map>
#include <string>
#include <algorithm>
#include <cctype>
class WordFrequency {
private:
std::unordered_map<std::string, int> wordMap;
std::string sanitize(const std::string& word) {
std::string sanitized;
std::copy_if(word.begin(), word.end(), std::back_inserter(sanitized), [](char c) {
return std::isalnum(c) || c == '\''; // Keep intra-word punctuation like apostrophes
});
std::transform(sanitized.begin(), sanitized.end(), sanitized.begin(), ::tolower); // Convert to lowercase
return sanitized;
}
public:
WordFrequency(std::istream& input = std::cin) {
std::string word;
while (input >> word) {
word = sanitize(word);
if (!word.empty()) {
++wordMap[word];
}
}
}
};
This code reads words from an input stream, sanitizes each word, and stores the word counts in an unordered_map.
Counting Words
The word counting function updates the count for each sanitized word in the hash table. The hash table ensures efficient lookups and updates, which is crucial for processing large texts:
void addWord(const std::string& word) {
std::string sanitizedWord = sanitize(word);
if (!sanitizedWord.empty()) {
wordMap[sanitizedWord]++;
}
}
Output and Analysis
Finally, you need functions to generate the required output, such as writing word counts to a file or finding the most frequent word. Here's how you might implement these functions:
int numberOfWords() const {
return wordMap.size();
}
int wordCount(const std::string& word) const {
auto it = wordMap.find(word);
return it != wordMap.end() ? it->second : 0;
}
std::string mostFrequentWord() const {
int maxCount = 0;
std::string mostFrequent;
for (const auto& pair : wordMap) {
if (pair.second > maxCount) {
maxCount = pair.second;
mostFrequent = pair.first;
}
}
return mostFrequent;
}
void writeOutput(const std::string& filename) const {
std::ofstream outputFile(filename);
for (const auto& pair : wordMap) {
outputFile << pair.first << " " << pair.second << std::endl;
}
}
These functions provide the necessary functionality to count words, identify the most frequent word, and write the results to a file.
Testing and Debugging
Testing your code is a critical step in ensuring its correctness and robustness. This section covers the essential practices for testing and debugging your text analysis project.
Testing with Sample Data
To validate your program, test it with various input cases:
- Normal Text: Use a text file with a variety of words and punctuation.
- Edge Cases: Test with empty files, files with only punctuation, and files with large words.
- Special Characters: Ensure your program handles non-alphabetic characters and numbers correctly.
Creating a test suite with different types of input helps ensure your program can handle a wide range of scenarios.
Using Debugging Tools
Debugging tools can help you identify and fix issues in your code. Use a debugger to step through your program, inspect variables, and understand the flow of execution. Common debugging techniques include:
- Breakpoints: Set breakpoints to pause execution and inspect the state of your program.
- Watch Variables: Monitor the values of variables to detect unexpected changes.
- Step Through Code: Execute your code line-by-line to observe its behavior and identify where errors occur.
Debugging effectively requires a methodical approach and patience, but it can save you time and effort in the long run.
Optimizing Your Code
Efficiency is crucial, especially when processing large texts. Optimizing your code can improve its performance and reduce resource usage.
Algorithm Efficiency
Choose algorithms that minimize time complexity. For example, using a hash table for word counting provides average O(1) time complexity for insertions and lookups, which is much more efficient than a linear search.
Memory Management
In languages like C++, managing memory efficiently is important to prevent leaks and ensure your program runs smoothly. Use smart pointers or ensure proper deletion of dynamic memory to avoid memory issues.
Code Refactoring
Refactor your code to improve readability and maintainability. Simplify complex functions, remove redundant code, and adhere to coding standards. Clear and well-organized code is easier to debug, test, and optimize.
Documenting and Submitting Your Assignment
Proper documentation and adherence to submission guidelines are critical for presenting your work professionally and ensuring it is graded correctly.
Commenting Your Code
Include comments throughout your code to explain the purpose of each function and the logic of complex sections. Comments should be concise but informative, providing enough context for someone else to understand your code.
// This function sanitizes a word by removing non-alphanumeric characters
// and converting it to lowercase.
std::string sanitize(const std::string& word) {
// Implementation here...
}
Writing a ReadMe File
A well-written ReadMe file provides an overview of your project and instructions for building and running your program. Include sections such as:
- Project Description: A brief summary of what the program does.
- Requirements: List any software or libraries needed to compile and run the program.
- Installation Instructions: Provide step-by-step instructions for setting up the development environment and compiling the code.
- Usage: Explain how to run the program and use its features.
- Output: Describe the expected output and where it can be found.
Following Submission Guidelines
Adhere strictly to the submission guidelines provided in your assignment. This includes:
- File Naming and Formats: Ensure your files are named correctly and submitted in the required formats.
- Output Requirements: Verify that your program produces the required output files and formats.
- Code Modifications: Only modify designated sections of the code if specified, and avoid changes that are not allowed.
Double-check your submission to ensure all files are included and meet the assignment requirements.
Conclusion
Mastering programming assignments, especially those involving text analysis using hash tables, requires a structured approach and attention to detail. By understanding the assignment requirements, planning your approach, writing efficient code, and thoroughly testing your solution, you can tackle these projects with confidence and skill. Remember, each assignment is an opportunity to improve your programming abilities and deepen your understanding of key concepts.
For those who find programming assignments challenging, resources like ProgrammingHomeworkHelp.com can provide the guidance and support you need to succeed. Whether you need help understanding the assignment or developing a solution, expert assistance is just a click away.