Claim Your Discount Today
Ring in Christmas and New Year with a special treat from www.programminghomeworkhelp.com! Get 15% off on all programming assignments when you use the code PHHCNY15 for expert assistance. Don’t miss this festive offer—available for a limited time. Start your New Year with academic success and savings. Act now and save!
We Accept
- Understanding the Problem Statement
- Read Carefully
- Identify Key Requirements
- Clarify Doubts
- Setting Up Your Development Environment
- Choose the Right Tools
- Configure Your Project
- Organize Your Files
- Initial Project Setup
- Create a New Project
- Add Dependencies
- Create Required Classes
- Implementing Core Functionality
- Start Simple
- Follow Guidelines
- Write Helper Methods
- Implement Comparable
in Bus Class - Build the BusStopTree
- Testing Your Code
- Write Test Cases
- Test Incrementally
- Use Provided Utilities
- Debugging
- Systematic Debugging
- Print Statements
- Optimization and Refinement
- Optimize Code
- Refactor
- Improve Documentation
- Submission Preparation
- Review Requirements
- Final Testing
- Package and Submit
- Example: Implementing a Binary Search Tree for Bus Schedules
- Step-by-Step Implementation
- Implement Comparable
in Bus Class - Build the BusStopTree
- Test Your Implementation
- Implement Comparable
Programming assignments can often seem overwhelming, especially when they involve intricate projects with multiple components. Solving java assignments especially those involving complex projects like implementing a Bus Stop Tree in Java, can often seem daunting. However, by following a systematic approach, you can tackle these assignments efficiently and effectively. This guide aims to provide you with a comprehensive strategy to solve your programming assignment, using a sample project to illustrate the steps. This guide will be structured into several sections to make it easy to follow.
Understanding the Problem Statement
The first and most crucial step in solving any programming assignment is to understand the problem statement thoroughly. This step lays the foundation for all subsequent work and helps prevent misunderstandings that could lead to incorrect solutions.
Read Carefully
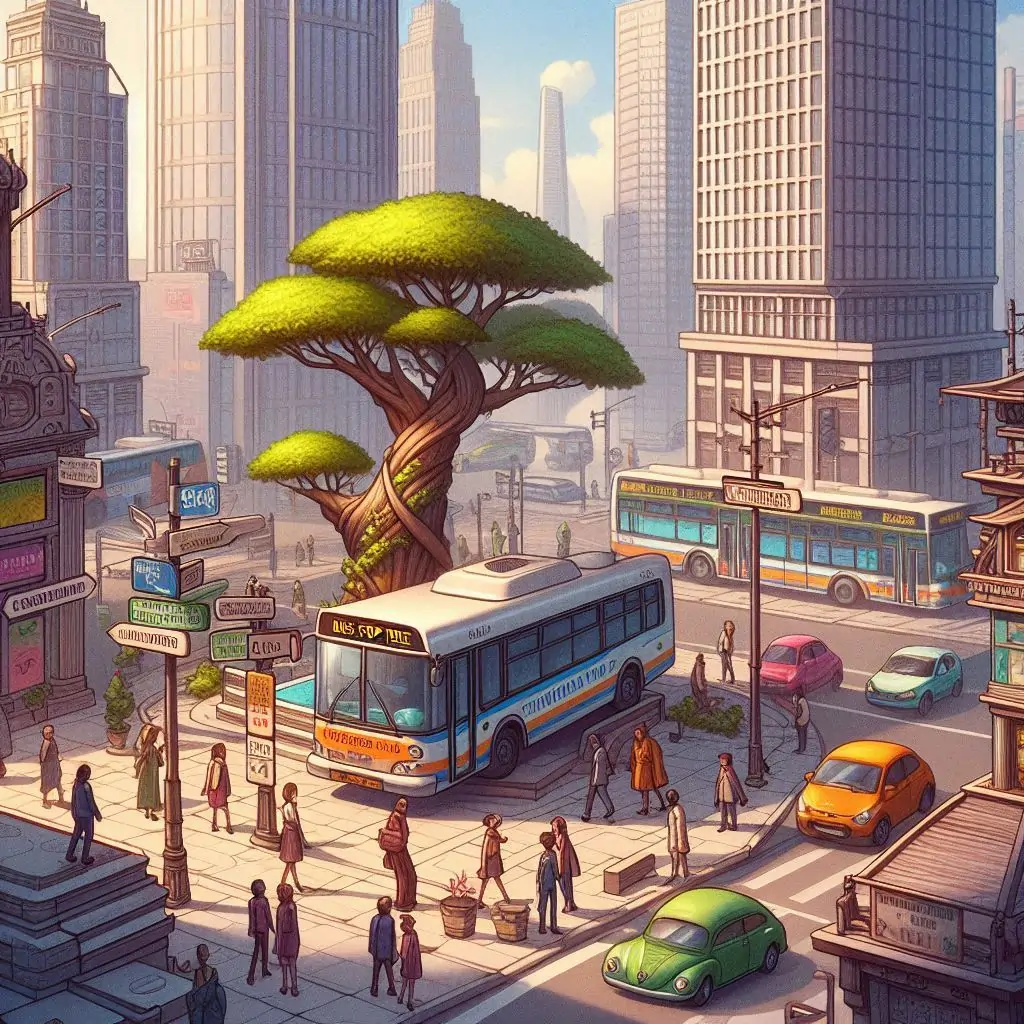
Begin by reading the problem statement and any provided documentation meticulously. Pay attention to every detail and ensure that you understand the requirements clearly. It’s essential to grasp the overall objective of the assignment as well as the specific tasks you need to accomplish.
Identify Key Requirements
Once you have read the problem statement, highlight the key requirements. These requirements are the main tasks you need to complete, such as implementing specific classes, methods, or algorithms. By identifying these requirements, you can break down the assignment into manageable parts and plan your work accordingly.
Clarify Doubts
If any part of the problem statement is unclear, don’t hesitate to seek clarification. You can ask your instructor, classmates, or seek help from online forums. It’s better to clarify doubts at the beginning than to proceed with incorrect assumptions.
Setting Up Your Development Environment
Setting up a suitable development environment is the next critical step. A well-configured environment can significantly enhance your productivity and help you avoid technical issues that could impede your progress.
Choose the Right Tools
Ensure that you are using the correct development environment and tools for your project. For example, if you are working on a Java project, Eclipse is a popular and powerful integrated development environment (IDE) that you can use. Make sure you have the necessary software installed and configured correctly.
Configure Your Project
Set up your project according to the specifications provided in the assignment. This may include setting the correct JDK version, configuring build paths, and setting up any required libraries or frameworks. For instance, if the assignment specifies using Java 17, make sure your project is configured to use this version.
Organize Your Files
Proper organization of your project files is crucial for maintaining clarity and ease of navigation. Download all necessary files, such as libraries, provided source files, and data sets, and arrange them logically within your project structure. This organization will make it easier to find and manage files as you work on the assignment.
Initial Project Setup
Once your development environment is ready, the next step is to set up your project. This involves creating the necessary project structure, adding dependencies, and ensuring everything is correctly configured.
Create a New Project
In your IDE, create a new project and configure it as instructed in the assignment. For example, if you are working on an Eclipse project, you would create a new Java project and set the appropriate JDK version.
Add Dependencies
Include any required libraries or JAR files in your project’s build path. These dependencies are often essential for the functionality of your project and must be correctly referenced.
Create Required Classes
Set up the files you will need to implement. Create the classes as specified in the assignment and add any stubs or skeletons required to ensure the project compiles. This step provides a framework within which you can begin implementing the actual logic.
Implementing Core Functionality
With the initial setup complete, you can now start implementing the core functionality of the assignment. This step involves writing the code to fulfill the main requirements of the project.
Start Simple
Begin with basic implementations and gradually add complexity. For instance, if you need to implement several interfaces and methods, start with the simpler ones and ensure they work correctly before moving on to more complex tasks. This incremental approach helps you build a solid foundation and makes debugging easier.
Follow Guidelines
Adhere to any specific implementation guidelines provided in the assignment. These guidelines might include coding standards, method signatures, or algorithmic requirements. Following these guidelines ensures that your solution meets the expectations and requirements of the assignment.
Write Helper Methods
Break down complex methods into smaller, manageable helper methods. This modular approach makes your code more readable, easier to debug, and more maintainable. Helper methods can also be reused across different parts of your project, promoting code reuse and efficiency.
Implement Comparable<Bus> in Bus Class
To illustrate, let's look at implementing the Comparable<Bus> interface in a Bus class:
public class Bus implements Comparable<Bus> {
// Fields for arrival time, route, etc.
@Override
public int compareTo(Bus other) {
return this.arrivalTime.compareTo(other.arrivalTime);
}
// Other methods
}
Build the BusStopTree
Next, you would build the BusStopTree, a binary search tree (BST) that orders buses by their arrival times. Here’s a basic implementation:
public class BusStopTree {
private Node<Bus> root;
private static class Node<Bus> {
Bus data;
Node<Bus> left;
Node<Bus> right;
Node(Bus data) {
this.data = data;
this.left = this.right = null;
}
}
public void addBus(Bus bus) {
root = addBusHelper(root, bus);
}
private Node<Bus> addBusHelper(Node<Bus> node, Bus bus) {
if (node == null) {
return new Node<>(bus);
}
if (bus.compareTo(node.data) < 0) {
node.left = addBusHelper(node.left, bus);
} else {
node.right = addBusHelper(node.right, bus);
}
return node;
}
// Other methods
}
Testing Your Code
Thorough testing is critical to ensure that your implementation works as expected. Developing a comprehensive suite of test cases helps identify and fix bugs early in the development process.
Write Test Cases
Develop thorough test cases for each method you implement. These test cases should cover a range of scenarios, including edge cases and typical use cases. By writing detailed tests, you can ensure that your code handles all possible inputs correctly.
Test Incrementally
Test your code in small increments. After implementing a method, write test cases for it and ensure it works correctly before moving on to the next method. This incremental testing approach makes debugging easier and ensures each part of your code is robust before you proceed.
Use Provided Utilities
Leverage any provided utilities or dummy data to facilitate testing. For example, if the assignment includes methods for creating dummy bus routes or bus stops, use these methods to simplify the creation of test objects. This approach helps you focus on testing your implementation rather than spending time setting up test data.
Debugging
Despite careful planning and testing, bugs are inevitable in programming. Effective debugging techniques can help you quickly identify and fix these issues.
Systematic Debugging
Use the debugging tools in your IDE to step through your code and identify issues. Set breakpoints at critical points in your code and inspect the values of variables to understand the program’s state. This systematic approach helps you pinpoint the exact location and cause of bugs.
Print Statements
Add print statements to your code to understand the flow of your program and the state of your variables at various points. Print statements can be especially helpful for debugging complex logic or recursive methods. However, remember to remove or comment out these statements once you have resolved the issues.
Optimization and Refinement
Once your basic implementation is working correctly, you can focus on optimizing and refining your code to improve its performance, readability, and maintainability.
Optimize Code
Look for ways to optimize your code for better performance. This may involve improving the efficiency of algorithms, reducing the complexity of methods, or optimizing memory usage. Efficient code not only runs faster but also consumes fewer resources.
Refactor
Refactor your code to improve its structure and eliminate redundancy. Refactoring involves cleaning up your code, organizing it more logically, and making it easier to understand. This step is crucial for maintaining the quality and readability of your code, especially in larger projects.
Improve Documentation
Enhance the documentation of your code by adding comments and writing detailed documentation. Comments should explain the purpose of classes, methods, and complex logic. Additionally, provide user guides or API documentation if required. Good documentation helps others (and your future self) understand and use your code effectively.
Submission Preparation
The final step is to prepare your project for submission. This involves reviewing the assignment requirements, performing final testing, and packaging your project correctly.
Review Requirements
Ensure you have met all the requirements of the assignment. Revisit the problem statement and check that you have implemented all the necessary features and followed all guidelines. This review helps you avoid missing any critical elements.
Final Testing
Run a final round of testing to ensure everything works as expected. This last round of testing should cover all aspects of your project and verify that there are no remaining bugs. Thorough final testing ensures that your project is ready for submission.
Package and Submit
Package your project as required (e.g., in a ZIP file) and submit it through the appropriate channel. Ensure that all necessary files are included and that the project structure is correct. Double-check the submission guidelines to make sure you haven’t missed any steps.
Example: Implementing a Binary Search Tree for Bus Schedules
To illustrate the process, let's walk through how you would approach a specific part of a sample project: implementing a Binary Search Tree (BST) for sorting bus schedules.
Step-by-Step Implementation
Implement Comparable<Bus> in Bus Class
Begin by implementing the Comparable<Bus> interface in the Bus class. This interface allows Bus objects to be compared based on their arrival times, which is crucial for building the BST.
public class Bus implements Comparable<Bus> {
private String arrivalTime;
private String route;
@Override
public int compareTo(Bus other) {
return this.arrivalTime.compareTo(other.arrivalTime);
}
// Constructor, getters, setters, etc.
}
Build the BusStopTree
Next, implement the BusStopTree class, which represents a BST of Bus objects. This class will include methods for adding buses, retrieving the first bus, and validating the BST structure.
public class BusStopTree {
private Node<Bus> root;
private static class Node<Bus> {
Bus data;
Node<Bus> left;
Node<Bus> right;
Node(Bus data) {
this.data = data;
this.left = this.right = null;
}
}
public void addBus(Bus bus) {
root = addBusHelper(root, bus);
}
private Node<Bus> addBusHelper(Node<Bus> node, Bus bus) {
if (node == null) {
return new Node<>(bus);
}
if (bus.compareTo(node.data) < 0) {
node.left = addBusHelper(node.left, bus);
} else {
node.right = addBusHelper(node.right, bus);
}
return node;
}
// Other methods such as getFirstBus, isValidBST, etc.
}
Test Your Implementation
Finally, write test cases to ensure your implementation works as expected. Create a BusStopTreeTester class to systematically test the methods of the BusStopTree class.
public class BusStopTreeTester {
public static void main(String[] args) {
BusStopTree tree = new BusStopTree();
Bus bus1 = new Bus("08:00", "Route 1");
Bus bus2 = new Bus("09:00", "Route 2");
Bus bus3 = new Bus("07:30", "Route 3");
tree.addBus(bus1);
tree.addBus(bus2);
tree.addBus(bus3);
// Test getFirstBus method
assert tree.getFirstBus().equals(bus3);
// Test isValidBST method
assert tree.isValidBST();
}
}
By following these steps, you can systematically approach and solve complex programming assignments, ensuring a thorough understanding and robust implementation of the required functionality.