Claim Your Discount Today
Ring in Christmas and New Year with a special treat from www.programminghomeworkhelp.com! Get 15% off on all programming assignments when you use the code PHHCNY15 for expert assistance. Don’t miss this festive offer—available for a limited time. Start your New Year with academic success and savings. Act now and save!
We Accept
- Step 1: Understand the Problem Statement
- Step 2: Plan Your Approach
- Step 3: Implement the Solution
- Data Reading and Parsing
- Graph Construction
- Graph Algorithms
- User Interaction
- Step 4: Optimize for Efficiency
- Data Structures
- Algorithm Efficiency
- Memory Usage
- Step 5: Test Thoroughly
- Unit Testing
- Integration Testing
- Performance Testing
- Step 6: Document and Submit
- Code Comments
- README File
- Submission
- Example Breakdown: Enron Email Dataset
- Conclusion
Tackling programming assignments that involve manipulating and analyzing large datasets with graph algorithms can initially appear daunting. However, by breaking the task into manageable steps and adhering to best practices, you can efficiently address these challenges while showcasing your mastery of essential computer science concepts. Start by thoroughly understanding the problem statement and planning your approach, including data reading, parsing, graph construction, and implementing the necessary algorithms. Optimize your solution for efficiency, choosing the right data structures and ensuring algorithm performance. Testing is crucial—unit tests for individual components and integration tests for the complete application ensure accuracy and robustness. Document your code comprehensively, with clear comments and a detailed README file. For instance, analyzing the Enron email dataset involves parsing email files, constructing a graph of email interactions, identifying key connectors using Depth-First Search (DFS), and responding to user queries about the dataset. By systematically addressing each part of the problem, you can effectively manage complex programming assignments involving graphs and data analysis, turning a daunting task into a manageable and rewarding project. You can solve your programming assignment efficiently with these steps and turn complex tasks into rewarding projects.
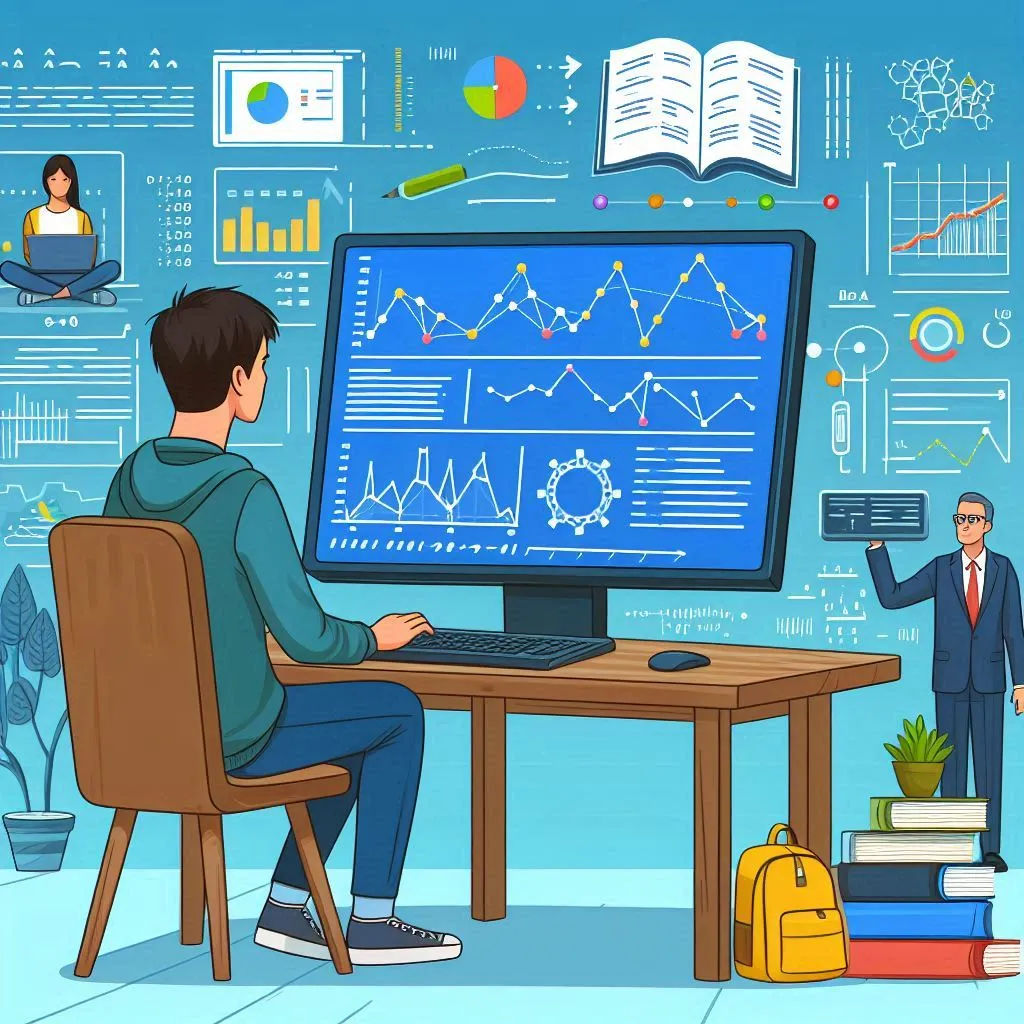
Step 1: Understand the Problem Statement
To begin tackling any programming assignment effectively, start by thoroughly comprehending the problem statement. This involves breaking down the assignment requirements into specific components:
- Functional Requirements: Identify what functionalities your program needs to implement. For instance, this may include tasks such as reading a dataset, constructing a graph from the data, identifying key connectors in the graph structure, and generating responses to user queries based on the analysis.
- Constraints and Restrictions: Understand any limitations or constraints imposed on your solution. This could include restrictions on the use of external libraries, requirements for certain performance benchmarks, or specific guidelines for memory usage and runtime efficiency.
- Expected Output: Clarify how your program should present its results. Determine whether the output should be displayed directly (e.g., printed to the console) or saved to a file. This step ensures that your implementation aligns with the expected deliverables and meets the assignment's specifications accurately.
By structuring your approach around these key aspects of the problem statement, you lay a solid foundation for developing a comprehensive and effective solution. Understanding these details upfront helps in crafting a clear and targeted strategy for your programming assignment.
Step 2: Plan Your Approach
When planning your approach to a programming assignment, it's crucial to break down the problem into smaller, manageable parts. This structured approach helps in tackling complex tasks effectively:
- Data Reading and Parsing: Develop code to read and parse the dataset. For instance, in the case of the Enron dataset, this involves extracting information from email files, such as sender, recipient, and timestamp details.
- Graph Construction: Conceptualize the problem as a graph. In the Enron scenario, individuals can be represented as vertices, while email exchanges form the edges connecting these vertices. Implementing this graph structure lays the foundation for further analysis.
- Graph Algorithms: Implement essential graph algorithms tailored to your assignment's requirements. For example, utilize Depth-First Search (DFS) to identify connectors within the graph. Understanding and applying these algorithms are crucial for deriving meaningful insights from the dataset.
- User Interaction: Design an intuitive interface for interacting with your program's output. Consider how users will query and interact with the dataset analysis results. This step ensures your program not only computes results accurately but also presents them in a user-friendly manner.
By breaking down the assignment into these systematic components, you streamline the development process and ensure each aspect of the problem is addressed comprehensively. This structured approach enhances clarity, efficiency, and the overall quality of your solution.
Step 3: Implement the Solution
Implementing the solution involves executing a structured approach to handle the programming assignment effectively:
Data Reading and Parsing
- File I/O Operations: Utilize file operations to read the dataset efficiently. For instance, when dealing with the Enron dataset, employ file handling techniques to access and retrieve email files.
- Email Parsing: Extract essential information like sender, recipient, and timestamp from email files. This step is crucial for subsequent data analysis and graph construction.
- Data Structures: Employ appropriate data structures such as HashMaps for storing extracted information. HashMaps facilitate quick data lookups and are instrumental in managing large datasets.
Graph Construction
- Graph Representation: Develop a dedicated class to represent the graph structure. Define methods within this class to facilitate graph operations and interactions.
- Adjacency List or Matrix: Choose between an adjacency list or matrix to store graph edges based on the assignment requirements. Ensure the chosen structure efficiently supports operations like edge addition and traversal.
- Edge Management: Ensure the integrity of the graph by preventing duplicate edges and self-loops, maintaining consistency in graph representation.
Graph Algorithms
- Depth-First Search (DFS): Implement DFS algorithm to traverse the constructed graph. Track dfsnum and back values during traversal to identify pivotal connectors within the graph structure.
- Handling Edge Cases: Address unique scenarios such as disconnected components within the graph. Develop strategies to manage these edge cases effectively during algorithm execution.
User Interaction
- Interface Design: Design an intuitive user interface (UI) to facilitate interaction with the graph analysis results. Consider user-friendly features and functionalities that enhance usability.
- Query Methods: Implement methods within the UI to compute and display pertinent information, such as the number of unique email addresses a person has interacted with. Ensure these methods provide accurate and meaningful insights from the analyzed dataset.
By meticulously implementing each component outlined in this approach, you can navigate through complex programming assignments involving graphs and data analysis methodically. This structured implementation ensures clarity, efficiency, and robustness in addressing the assignment requirements.
Step 4: Optimize for Efficiency
Efficiency optimization is crucial in handling programming assignments involving graphs and data analysis. Follow these guidelines to ensure your solution performs optimally:
Data Structures
- Selecting Efficient Structures: Choose data structures such as HashMaps and HashSets based on their average O(1) time complexity for operations like insertions and lookups. These structures are essential for managing and accessing data swiftly, especially in large datasets.
Algorithm Efficiency
- Optimizing Algorithm Performance: Ensure your implemented algorithms, such as Depth-First Search (DFS), operate efficiently within the assignment's constraints. DFS, for example, runs in O(V + E) time complexity, making it suitable for traversing large graphs without performance bottlenecks.
Memory Usage
- Managing Memory Consumption: Be cautious of memory utilization, particularly when processing extensive datasets. Optimize data storage practices to minimize redundancy and unnecessary duplication. Efficient memory management enhances the scalability and performance of your solution, enabling it to handle large datasets seamlessly.
By prioritizing these efficiency strategies during implementation, you enhance the responsiveness and scalability of your solution. This approach not only meets the assignment's performance requirements but also prepares you for tackling future challenges in graph and data analysis tasks effectively.
Step 5: Test Thoroughly
Testing is a critical phase in developing a robust and reliable solution for programming assignments involving graphs and data analysis. Here’s how to approach testing comprehensively:
Unit Testing
- Component Testing: Write unit tests for each individual component of your solution. Focus on testing functionalities such as graph construction, the implementation of DFS algorithms, and any helper functions. This ensures that each part of your code works correctly in isolation.
Integration Testing
- Comprehensive Testing: Conduct integration tests by running the complete application with a variety of inputs. This step is crucial to verify that all components interact seamlessly and that the system handles different scenarios correctly. Test edge cases, typical inputs, and boundary conditions to ensure robustness.
Performance Testing
- Full Dataset Testing: Run performance tests using the entire dataset to evaluate your solution’s efficiency and scalability. Monitor the application’s performance, including runtime and memory usage, to ensure it meets the constraints and performs optimally under load. This step helps identify any performance bottlenecks or memory issues that need addressing.
By thoroughly testing your solution through these stages, you can ensure its correctness, reliability, and efficiency, making it well-prepared to handle complex graph and data analysis tasks.
Step 6: Document and Submit
Effective documentation and submission practices are crucial for presenting your programming assignment professionally and ensuring its clarity and usability:
Code Comments
- Clarity and Understanding: Enhance code readability by adding concise comments to explain the purpose of classes, methods, and critical sections of your code. This practice aids in understanding and maintaining the codebase over time.
README File
- Usage Instructions: Create a comprehensive README file detailing how to execute your program. Include instructions on setting up dependencies, running the application, and any specific configurations required. Clear and precise documentation ensures that others can easily replicate your setup and use your program effectively.
Submission
- Follow Guidelines: Adhere to the submission guidelines provided by your instructor or assignment requirements. This typically involves pushing your code to a version control system like GitHub and submitting the repository link through the designated platform. Verify that your submission meets all specified criteria to avoid any delays or issues.
By documenting your code effectively and following proper submission protocols, you demonstrate professionalism and ensure that your hard work is presented clearly and accessibly. This approach not only facilitates evaluation but also prepares your solution for potential future iterations or collaborations.
Example Breakdown: Enron Email Dataset
Let's break down how to approach the Enron email dataset assignment using structured steps:
- Read Data: Develop a method to read email files from the dataset directory. Extract crucial details such as sender, recipient, and relevant metadata required for further analysis.
- Construct Graph: Establish a Graph class tailored to represent the dataset's email interactions. Utilize an adjacency list to efficiently manage and store graph edges. Implement methods to seamlessly incorporate vertices and edges into the graph structure.
- Identify Connectors: Implement a specialized method utilizing Depth-First Search (DFS) to pinpoint connectors within the email communication network. Monitor and record dfsnum and back values during traversal to identify pivotal connectors based on defined criteria.
- User Queries: Introduce a dedicated method to address user queries regarding email interactions within the dataset. Calculate essential metrics such as the count of sent and received emails by individuals. Determine the team size by identifying clusters of individuals who have exchanged emails, regardless of direct interaction.
By meticulously following these steps, you can effectively handle and analyze the Enron email dataset assignment. This approach ensures systematic progress from data ingestion to complex graph analysis, culminating in insightful results that demonstrate proficiency in programming and data manipulation techniques.
Conclusion
By following these steps and systematically addressing each part of the problem, you can effectively tackle any similar programming assignment involving graphs, algorithms, and large datasets. Understanding the problem statement thoroughly, planning a structured approach, implementing efficient data parsing and graph construction techniques, and optimizing algorithms for performance are key. Testing your solution rigorously ensures it handles diverse inputs accurately. Documenting your code with clear comments and instructions enhances readability and maintainability. Whether analyzing the Enron email dataset or other complex tasks, mastering these skills not only helps in achieving assignment goals but also deepens your understanding of graph theory and data analysis. With practice, you'll build confidence in solving computational problems, making significant strides in your programming journey.