Claim Your Discount Today
Ring in Christmas and New Year with a special treat from www.programminghomeworkhelp.com! Get 15% off on all programming assignments when you use the code PHHCNY15 for expert assistance. Don’t miss this festive offer—available for a limited time. Start your New Year with academic success and savings. Act now and save!
We Accept
- Introduction to Designing a Test and Survey System
- Understanding the Problem
- High-Level Design
- UML Diagrams
- Class Diagram
- Sequence Diagram
- Implementing the Solution
- Survey and Test Classes
- 2. Question Class and Subclasses
- 3. Grading Class
- 4. Storage Class
- 5. Results Module
- Testing and Validation
- Unit Testing
- Integration Testing
- Conclusion
Creating a generic system for surveys and tests involves several fundamental software engineering principles, including modularity, abstraction, and separation of concerns. In this blog, we’ll discuss how to approach designing and implementing a survey and test-taking system. While the assignment above provides a specific problem statement, this guide will be generalized to help you solve your software engineering assignment effectively.
We will start with the high-level design, breaking down the problem into manageable components, and then discuss the detailed implementation. We will use UML diagrams to visualize the structure of the system and provide code snippets to clarify the concepts. The goal is to provide a deep understanding of how to design such systems, which can be adapted to various programming languages and requirements. This content will also help with UML diagram assignments, offering practical insights into effective system modeling.
Introduction to Designing a Test and Survey System
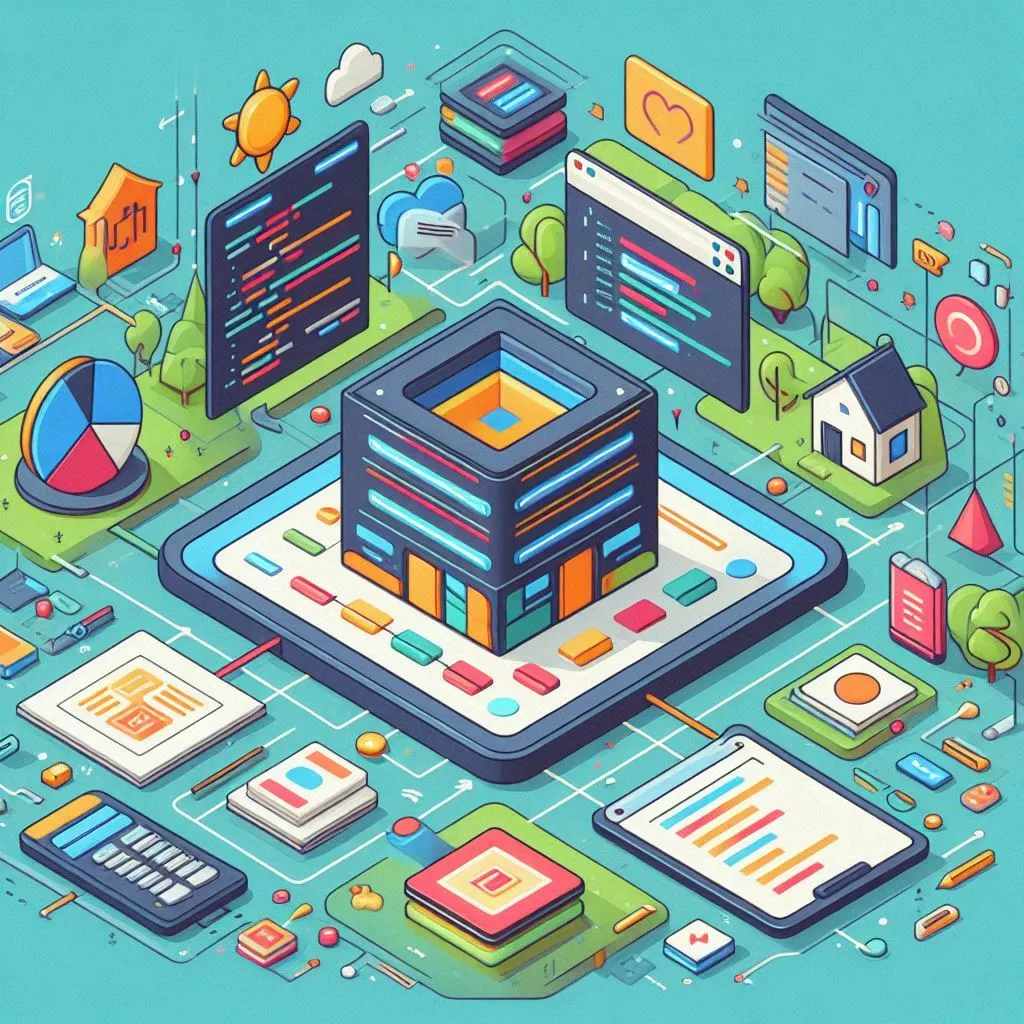
Understanding the Problem
Before diving into the solution, it’s important to understand the problem requirements. Here’s a breakdown of what we need:
1. Survey and Test Management:
- Surveys and tests should be created, modified, stored, loaded, and taken by users.
- A test is similar to a survey but includes correct answers and grading functionality.
2. Question Types:
- The system should support various types of questions: True/False, Multiple Choice, Short Answer, Essay, Matching, and Valid Date.
- Each question can accept single or multiple responses depending on its type.
3. Grading and Results:
- Tests should be graded based on predefined correct answers, except for essays.
- Survey results should be tabulated, and test results should include grading.
4. Persistence:
- Surveys and tests, along with user responses, should be stored using serialization.
High-Level Design
The first step is to establish the core components of the system. Here’s a breakdown of the primary classes and their responsibilities:
1. Survey and Test Classes:
- Survey: Manages a collection of questions but has no concept of correct answers.
- Test: Inherits from Survey but adds functionality to handle correct answers and grading.
2. Question Classes:
- Question: A base class for different types of questions.
- TrueFalseQuestion, MultipleChoiceQuestion, ShortAnswerQuestion, EssayQuestion, MatchingQuestion, ValidDateQuestion: Specific question types inheriting from Question.
3. Response Classes:
- SurveyResponse: Handles responses to surveys.
- TestResponse: Handles responses to tests, including the ability to grade.
4. Persistence Classes:
- FileManager: Handles saving and loading surveys, tests, and responses using serialization.
5. Utility Classes:
- GradingModule: Handles the grading logic for tests.
- ResultsModule: Tabulates and displays the results of surveys and tests.
UML Diagrams
To visualize the structure, let’s create some UML diagrams. We’ll focus on class diagrams and sequence diagrams.
Class Diagram
The class diagram outlines the relationships and hierarchies between the main components.
Sequence Diagram
A sequence diagram helps to visualize the flow of actions when a user takes a test and the system grades it.
Implementing the Solution
Survey and Test Classes
Survey Class
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
public class Survey implements Serializable {
private static final long serialVersionUID = 1L;
private List<Question> questions;
private String title;
public Survey(String title) {
this.title = title;
this.questions = new ArrayList<>();
}
public void addQuestion(Question question) {
questions.add(question);
}
public void removeQuestion(Question question) {
questions.remove(question);
}
public void displaySurvey() {
System.out.println("Survey: " + title);
for (Question q : questions) {
q.display();
}
}
public void takeSurvey() {
// Logic for taking the survey
}
// Getters and Setters
}
Test Class
import java.io.Serializable;
public class Test extends Survey implements Serializable {
private static final long serialVersionUID = 1L;
private transient Grading grading;
public Test(String title) {
super(title);
this.grading = new Grading();
}
@Override
public void takeSurvey() {
super.takeSurvey();
// Additional logic for test-taking
}
public void gradeTest() {
grading.grade(this);
}
// Additional methods specific to Test
}
2. Question Class and Subclasses
Question Abstract Class
import java.io.Serializable;
public abstract class Question implements Serializable {
private static final long serialVersionUID = 1L;
private String text;
public Question(String text) {
this.text = text;
}
public abstract void display();
// Getters and Setters
}
TrueFalse Class
public class TrueFalse extends Question {
private boolean answer;
public TrueFalse(String text, boolean answer) {
super(text);
this.answer = answer;
}
@Override
public void display() {
System.out.println(getText() + " (True/False)");
}
// Getters and Setters
}
MultipleChoice Class
import java.util.HashMap;
import java.util.Map;
public class MultipleChoice extends Question {
private Map<String, Boolean> choices;
public MultipleChoice(String text) {
super(text);
choices = new HashMap<>();
}
public void addChoice(String choice, boolean isCorrect) {
choices.put(choice, isCorrect);
}
@Override
public void display() {
System.out.println(getText());
for (Map.Entry<String, Boolean> entry : choices.entrySet()) {
System.out.println("- " + entry.getKey());
}
}
// Getters and Setters
}
3. Grading Class
public class Grading {
public void grade(Test test) {
// Implement grading logic here
}
}
4. Storage Class
Serialization and Deserialization
import java.io.*;
public class Storage {
public static void save(Object object, String filename) throws IOException {
try (ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream(filename))) {
oos.writeObject(object);
}
}
public static Object load(String filename) throws IOException, ClassNotFoundException {
try (ObjectInputStream ois = new ObjectInputStream(new FileInputStream(filename))) {
return ois.readObject();
}
}
}
5. Results Module
public class ResultsModule {
public void displayResults(Survey survey) {
// Display results logic
}
}
Testing and Validation
Unit Testing
Write unit tests to ensure that each component functions correctly. For example, test the Survey class to ensure questions can be added and removed properly:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
public class SurveyTest {
@Test
public void testAddQuestion() {
Survey survey = new Survey("Test Survey");
TrueFalse question = new TrueFalse("Is this a test?", true);
survey.addQuestion(question);
assertEquals(1, survey.getQuestions().size());
}
}
Integration Testing
Ensure that the Test class integrates properly with the Grading class:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
public class TestIntegrationTest {
@Test
public void testGradeTest() {
Test test = new Test("Sample Test");
// Add questions to test
test.gradeTest();
// Check results
}
}
Conclusion
This guide offers a comprehensive approach to designing and implementing a flexible survey and test-taking system, emphasizing modularity, abstraction, and separation of concerns. By breaking the problem down into manageable components—such as survey and test management, question types, grading, and data persistence—you can develop a system that is both robust and maintainable.
Leveraging object-oriented principles like inheritance and polymorphism ensures the system's extensibility, allowing for the seamless addition of new features, such as different question types or advanced grading mechanisms, without modifying the existing codebase. The clear separation of concerns between classes and modules not only simplifies the system's understanding and testing but also enhances its scalability, paving the way for future growth. This approach can be particularly useful if you need assistance with Java assignment focused on developing such a system.
In conclusion, the design principles and implementation strategies explored here extend beyond the specific context of surveys and tests, offering valuable insights for a wide array of software engineering challenges. Whether you're developing a straightforward survey tool, a complex assessment platform, or looking to solve your programming assignment, this approach equips you with the tools to create a system that is both robust and flexible, capable of meeting the diverse needs of users and stakeholders alike.