Claim Your Offer
New semester, new challenges—but don’t stress, we’ve got your back! Get expert programming assignment help and breeze through Python, Java, C++, and more with ease. For a limited time, enjoy 10% OFF on all programming assignments with the Spring Special Discount! Just use code SPRING10OFF at checkout! Why stress over deadlines when you can score high effortlessly? Grab this exclusive offer now and make this semester your best one yet!
We Accept
- Assignment Overview
- Key Components
- 1. Setting Up Your Python File
- 2. Processing User Input
- 3. Implementing a Menu-Driven Interface
- 4. Writing Pseudocode
- Conclusion
- Key Takeaways:
Programming assignments often challenge students to blend various programming skills to develop functional and effective applications. These tasks frequently require a solid understanding of string manipulation, error handling, and the creation of user-friendly, menu-driven interfaces. Successfully tackling such assignments demands not only technical proficiency but also an organized and methodical approach to problem-solving. The ability to seamlessly integrate these elements can make a significant difference in the effectiveness and usability of your final program.
Understanding the intricacies of handling user input, formatting data, and implementing menu-driven interactions can significantly impact the functionality and usability of your program. It’s crucial to break down the assignment into manageable components. This includes creating functions to handle specific tasks, designing intuitive menus for user interaction, and incorporating robust error-checking mechanisms to handle unexpected inputs. By approaching your assignment with this structured methodology, you can streamline your coding process and enhance your problem-solving capabilities.
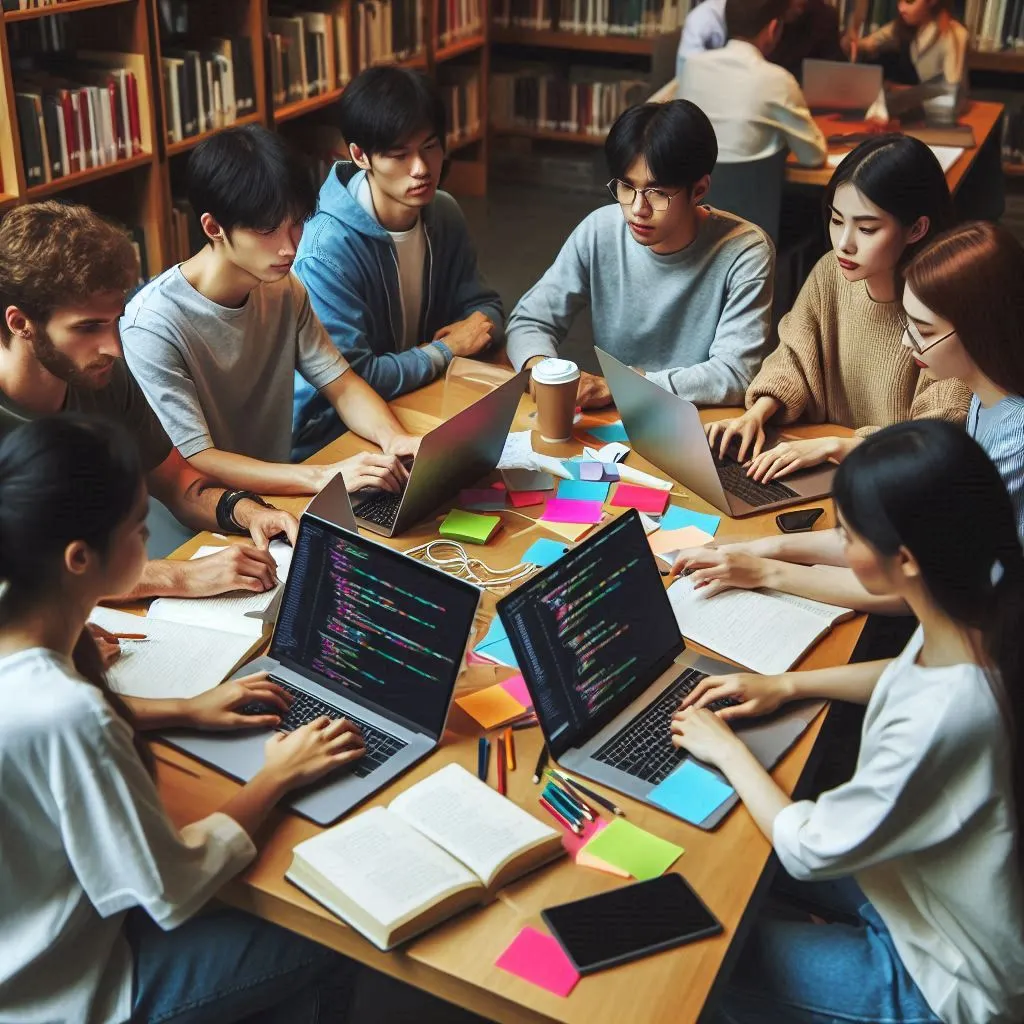
Moreover, attention to detail in how you process and format strings ensures that your program not only performs its intended functions but also does so in a way that is clear and accessible to users. Designing effective menus involves more than just presenting options; it requires anticipating user needs, providing clear instructions, and handling erroneous inputs gracefully. Error handling is equally important, as it helps maintain the robustness of your program, ensuring that it can manage and recover from unexpected situations without crashing or producing incorrect results.
In this blog, we will delve into the essential components of a typical programming assignment involving string manipulation and menu-driven interfaces. We’ll offer practical tips, detailed pseudocode, and sample code to guide you through similar tasks. Key aspects such as reading and formatting strings, designing user-friendly menus, and ensuring your program handles invalid inputs effectively will be covered in depth. These strategies are not just about completing assignments; they are about building a strong foundation in programming that will support you in tackling more complex challenges in the future.
By focusing on these foundational elements, you will gain a deeper understanding of how to create robust, user-friendly applications. This approach will not only help you complete your assignments more effectively but also enhance your overall programming expertise. With these skills, you will be better prepared for future programming tasks and able to approach new problems with greater confidence and efficiency. For additional support, consider leveraging python assignment help to further refine your skills and tackle complex assignments with ease.
Assignment Overview
Typically, these types of programming assignments may require you to perform several key tasks, each of which contributes to the development of a functional and user-friendly application. Here’s a detailed breakdown of what is generally expected, and how seeking programming assignment help can provide additional support in mastering these tasks:
- Create a Python Script: Start by setting up a Python file, where you’ll implement your solution. It’s crucial to include a title comment block at the beginning of your script. This block should provide a brief description of the project, the date, the course or module name, and your name. This not only helps in maintaining clarity but also makes your code easier to understand and review.
- Process Strings: Write a program that takes user input in a specific format and processes it accordingly. For instance, you might need to parse dates, format strings, or manipulate text in various ways. Your code should accurately handle the input data and transform it as required by the assignment’s specifications.
- Implement Menus: Develop an intuitive, menu-driven interface to enhance user interaction with your program. This involves creating a menu that presents users with various options and allows them to make selections. The menu should be designed to be clear and user-friendly, enabling smooth navigation through different functionalities of your program.
- Handle Errors: Ensure your program is robust by incorporating error handling mechanisms. This includes managing invalid input gracefully and providing meaningful error messages to guide users in correcting their mistakes. Proper error handling improves the user experience and ensures your program runs smoothly even when unexpected inputs are provided.
- Document Your Work: Document your code thoroughly by including pseudocode and comments. Pseudocode serves as a high-level outline of your program’s logic, while comments within the code explain the purpose of specific sections and functions. This documentation is essential for understanding your approach and logic, and it facilitates easier debugging and maintenance of your code.
By addressing these components comprehensively, you will be able to create well-structured, functional, and user-friendly applications that meet the requirements of your programming assignments.
Key Components
When tackling a programming assignment, particularly one that involves working with strings and creating interactive, menu-driven programs, it’s crucial to follow a structured approach. Understanding and executing several key components effectively will guide you through the process of setting up your Python environment, handling user input, implementing a user-friendly interface, and documenting your work thoroughly. This comprehensive approach not only helps in developing a functional and efficient program but also ensures that your code is clear, maintainable, and easy to understand.
1. Setting Up Your Python File
The initial step in any programming assignment is to create a Python file that is clearly named and organized. For example, if your name is Alex Smith, a suitable name for your file might be M6Lab_String_AlexSmith.py. This naming convention is crucial as it helps keep your work organized, especially when dealing with multiple files or collaborating with peers.
At the top of your Python file, include a title comment block. This block should contain:
- A brief description of the project or the assignment’s purpose.
- The date of creation or modification.
- The course or module name associated with the assignment.
- Your full name to identify the author of the code.
Including this comment block is not just a matter of good practice but also a way to provide important context for anyone who might review or work with your code in the future. It helps in understanding the project’s objective and the author’s identity, which is especially useful in collaborative environments or when submitting assignments for evaluation.
Here’s an example of what your title comment block might look like:
# A brief description of the project
# Date: [Insert Date]
# CSC121 M6Lab – Reading String
# Alex Smith
2. Processing User Input
In this part of the assignment, you will focus on writing a program that effectively interacts with users by processing their input. This involves several key steps:
- Prompt the User: Begin by asking the user to enter a date in the format mm/dd/yyyy. Ensure that your prompt is clear, precise, and user-friendly to avoid any potential confusion.
- Process and Format the Input: Convert the entered date into a more readable format, such as Month dd, yyyy. This step makes the date easier for users to understand and interpret.
Here’s a detailed pseudocode example to illustrate this process:
- Prompt the user to enter a date in the format mm/dd/yyyy.
- Split the date string into three components: month, day, and year.
- Use a predefined list of month names to convert the numeric month part into its corresponding name.
- Format the date and print it in the format Month dd, yyyy to enhance readability and user comprehension.
Sample Code:
# Function to convert month number to month name
def get_month_name(month_number):
# List of month names in order
months = ["January", "February", "March", "April", "May", "June",
"July", "August", "September", "October", "November", "December"]
# Return the month name corresponding to the month number
return months[month_number - 1]
# Main function to execute the program logic
def main():
# Prompt the user to enter a date
date_input = input("Enter a date (mm/dd/yyyy): ")
# Split the date input into month, day, and year
month, day, year = map(int, date_input.split('/'))
# Get the name of the month from the month number
month_name = get_month_name(month)
# Print the formatted date
print(f"{month_name} {day}, {year}")
if __name__ == "__main__":
main()
3. Implementing a Menu-Driven Interface
To make your program more interactive and user-friendly, you need to implement a menu-driven interface. This interface allows users to select from various options and navigate through different functionalities seamlessly. Here’s how you can approach creating this menu:
1. Display Menu Options: Present a set of choices to the user, including:
- Option 1: Prompt the user to enter a date.
- Option 2: Exit the program.
2. Handle User Choices:
- If the user selects option 1, execute the function that processes and formats the date.
- If the user selects option 2, terminate the program and display a farewell message.
- If the user enters an invalid choice, display an error message and prompt the user to select again.
3. Loop Until Exit: Continuously display the menu and handle user choices until the user decides to exit the program.
Sample Code:
def display_menu():
# Display menu options to the user
print("1. Enter a date")
print("2. Exit")
def main():
while True:
# Display the menu to the user
display_menu()
# Get the user's choice
choice = input("Enter your choice: ")
if choice == "1":
# Process the date input if choice is 1
date_input = input("Enter a date (mm/dd/yyyy): ")
month, day, year = map(int, date_input.split('/'))
month_name = get_month_name(month)
print(f"{month_name} {day}, {year}")
elif choice == "2":
# Exit the program if choice is 2
print("Exiting the program. Have a great day!")
break
else:
# Handle invalid choices
print("Invalid choice. Please select a valid option from the menu.")
if __name__ == "__main__":
main()
4. Writing Pseudocode
Before diving into actual coding, it is vital to draft pseudocode. Pseudocode serves as a planning tool that helps you outline the logic and structure of your program. It acts as a roadmap, allowing you to organize your thoughts and ensure that all necessary components are covered. Here’s an expanded pseudocode draft for a menu-driven program:
1. Define a Function to Convert Month Number to Name:
- Create a list of month names, ordered from January to December.
- Implement a function that returns the month name based on the provided month number.
2. Define the Main Function to Manage User Interaction and Program Flow:
- Display the menu options to the user.
- Handle user input based on the selected menu choices:
- If the user selects the option to enter a date, invoke the function that processes and formats the date.
- If the user selects the option to exit, end the program and display an exit message.
- If the user inputs an invalid choice, display an error message and prompt them to choose again.
- Continuously repeat the menu display and choice handling until the user decides to exit.
By incorporating these detailed components into your approach, you will be well-prepared to tackle programming assignments that involve string manipulation and menu-driven interfaces. This structured methodology will enhance your problem-solving skills and improve the overall quality and maintainability of your code. By practicing and applying these techniques, you’ll develop a deeper understanding of programming concepts, leading to more effective and efficient solutions in your future coding endeavors.
Conclusion
By adhering to these strategies and utilizing the provided pseudocode and sample code, you can confidently approach Python assignments that involve string manipulation and menu-driven programming. Each key component, from setting up your Python file and processing user input to implementing interactive menus and documenting your work, plays a vital role in developing a robust and user-friendly application.
Key Takeaways:
- File Setup: Properly naming and documenting your Python file ensures clarity and organization, making it easier for you and others to understand the purpose and context of your code.
- String Processing: Mastering string manipulation techniques is crucial for converting and formatting user input effectively, enhancing the readability and usability of your application.
- Menu-Driven Interface: Implementing a menu-driven interface improves user interaction, allowing for a more intuitive and engaging experience. It also helps in managing different functionalities within a single program.
- Error Handling: Graceful handling of invalid input and providing clear error messages contributes to a more reliable and user-friendly program.
- Documentation: Writing pseudocode and comments not only helps in planning and debugging your code but also aids others in understanding your logic and approach.
These strategies are not only applicable to the specific assignment at hand but are also foundational skills that will benefit you in various programming contexts. Practice these techniques regularly to build your confidence and proficiency in programming.
Feel free to adapt and modify the provided examples to better fit your unique assignment requirements and personal coding style. Experiment with different approaches and continuously seek to improve your coding practices.
With these tools and insights, you are well-prepared to tackle programming assignments effectively and to develop solutions that are both functional and well-structured. Embrace the learning journey and happy coding!