Claim Your Offer
New semester, new challenges—but don’t stress, we’ve got your back! Get expert programming assignment help and breeze through Python, Java, C++, and more with ease. For a limited time, enjoy 10% OFF on all programming assignments with the Spring Special Discount! Just use code SPRING10OFF at checkout! Why stress over deadlines when you can score high effortlessly? Grab this exclusive offer now and make this semester your best one yet!
We Accept
- Why Focus on Stacks and Queues?
- Stacks
- Queues
- Part 1: Evaluating Arithmetic Expressions Using a Stack
- Problem Overview
- Understanding Stacks
- Steps to Solve the Problem
- Sample Implementation in C++
- Detailed Breakdown of the Code
- Part 2: Implementing a Scheduler Using a Queue
- Problem Overview
- Understanding Queues
- Steps to Solve the Problem
- Sample Implementation in C++
- Detailed Breakdown of the Code
- Conclusion
Data structures are fundamental building blocks that help us manage and organize data efficiently. Two of the most versatile and commonly used data structures are stacks and queues. These structures are not just theoretical concepts but are used in practical applications ranging from expression evaluation to process scheduling.
Imagine you're faced with a complex arithmetic expression and need to determine the correct order of operations or consider the challenge of scheduling multiple processes to ensure each one gets fair CPU time. How would you approach these problems? The answer often lies in understanding and applying the right data structures—specifically stacks and queues.
This guide is designed to walk you through solving programming assignments that leverage these data structures. By breaking down complex problems into manageable components and demonstrating practical implementations, we aim to provide you with the tools and knowledge needed to tackle similar data structure assignments with confidence.
Why Focus on Stacks and Queues?
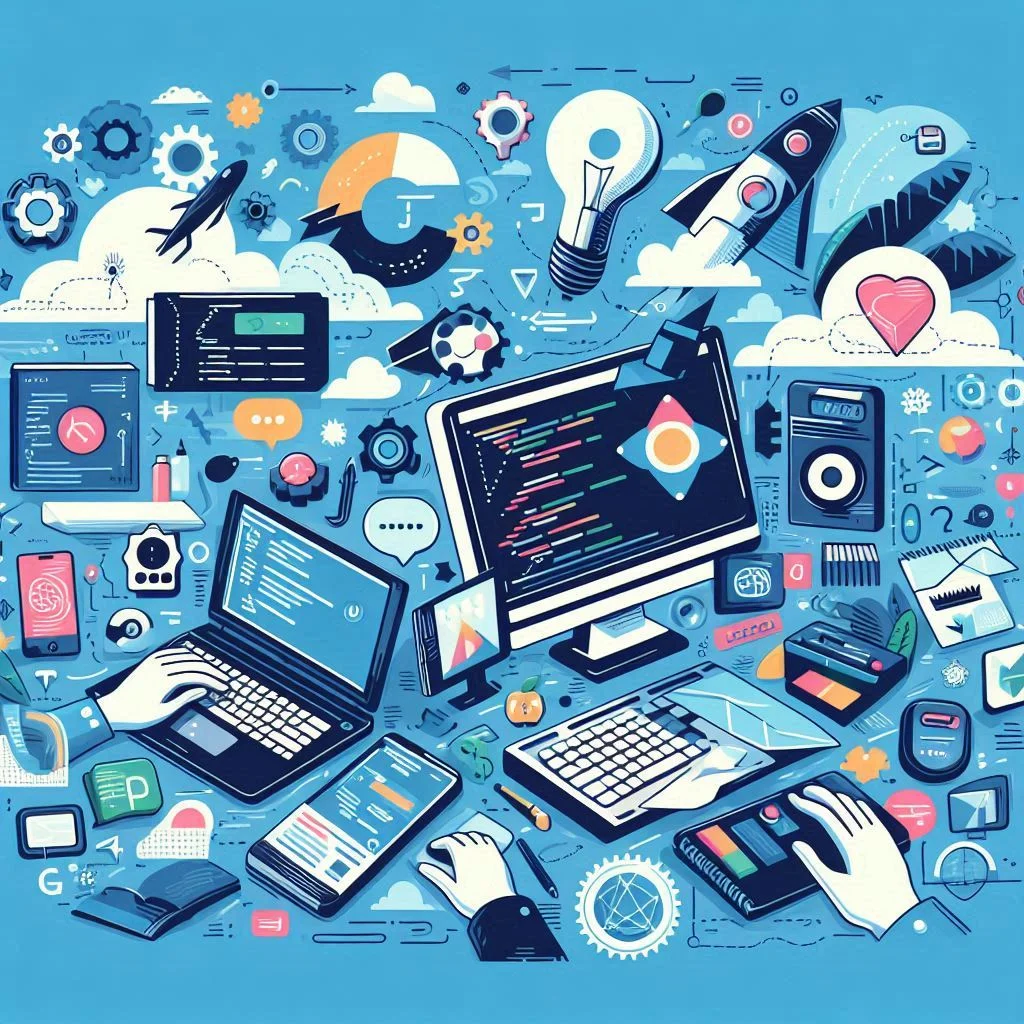
Stacks and queues are crucial in many computing scenarios. They help us manage data in ways that align with real-world processes and computational requirements:
Stacks
A stack is a linear data structure that follows the Last In, First Out (LIFO) principle. This means the last element added to the stack will be the first one to be removed. Think of a stack as a stack of plates where you can only add or remove the top plate.
Operations:
- Push: Add an item to the top of the stack.
- Pop: Remove the item from the top of the stack.
- Peek/Top: View the item on the top of the stack without removing it.
- IsEmpty: Check if the stack is empty.
Queues
A queue is a linear data structure that follows the First In, First Out (FIFO) principle. In a queue, the first element added is the first one to be removed, similar to a line at a ticket counter.
Operations:
- Enqueue: Add an item to the end of the queue.
- Dequeue: Remove the item from the front of the queue.
- Front: View the item at the front of the queue without removing it.
- IsEmpty: Check if the queue is empty.
Part 1: Evaluating Arithmetic Expressions Using a Stack
Problem Overview
Arithmetic expression evaluation is a fundamental problem in computer science. While simple for humans, automating this process with a program can be complex, particularly when parentheses are involved. The goal here is to determine the order of subexpression evaluation based on parentheses, ignoring operator precedence.
Understanding Stacks
A stack is a Last-In, First-Out (LIFO) data structure, meaning that the last element added is the first one to be removed. Stacks are useful for problems involving nested structures, such as parentheses in arithmetic expressions.
Steps to Solve the Problem
1. Algorithm Description:
- Initialization: Start by initializing a stack to keep track of the positions of opening parentheses.
- Traversal: Iterate through the arithmetic expression character by character.
- Handling Parentheses: When encountering an opening parenthesis '(', push its position onto the stack. When encountering a closing parenthesis ')', pop the stack to get the position of the matching opening parenthesis.
- Extracting Subexpressions: Print the substring between the matched parentheses, excluding the outermost parentheses.
2. Edge Cases and Validation:
- Empty Stack on Pop: Ensure the stack is not empty before attempting to pop, indicating mismatched parentheses.
- Unbalanced Parentheses: Check for unbalanced parentheses to handle incomplete expressions.
Sample Implementation in C++
Here's a detailed C++ implementation to evaluate arithmetic expressions using a stack:
#include <iostream>
#include <stack>
#include <string>
// Function to evaluate and print the order of subexpression evaluation
void evaluateExpression(const std::string &expression) {
std::stack<int> positions; // Stack to store positions of '('
for (size_t i = 0; i < expression.length(); ++i) {
if (expression[i] == '(') {
positions.push(i); // Push position of '('
} else if (expression[i] == ')') {
if (positions.empty()) {
std::cout << "Invalid expression" << std::endl;
return; // Exit if there is no matching '('
}
int start = positions.top();
positions.pop(); // Pop position of matching '('
std::string subexpression = expression.substr(start + 1, i - start - 1);
std::cout << subexpression << std::endl; // Print subexpression without outermost parentheses
}
}
if (!positions.empty()) {
std::cout << "Invalid expression" << std::endl; // Handle case of unbalanced parentheses
}
}
int main() {
std::string expression;
std::cout << "Enter an arithmetic expression: ";
std::getline(std::cin, expression); // Read the entire expression as a string
evaluateExpression(expression);
return 0;
}
Detailed Breakdown of the Code
1. Header Files:
- #include <iostream> and #include <stack> are necessary for input/output operations and stack data structure usage.
- #include <string> is required for string manipulation.
2. Function Definition:
- void evaluateExpression(const std::string &expression) defines a function to evaluate subexpressions.
3. Stack Initialization:
- std::stack<int> positions; initializes a stack to store positions of '(' characters.
4. Expression Traversal:
- A for loop iterates through each character in the expression.
5. Handling Parentheses:
- When encountering '(', the position is pushed onto the stack.
- When encountering ')', the position of the matching '(' is popped from the stack.
6. Subexpression Extraction:
- The substring between the matched parentheses is extracted and printed, excluding the outermost parentheses.
7. Edge Case Handling:
- The function checks if the stack is empty before popping to handle mismatched parentheses.
- After the loop, if the stack is not empty, it indicates unbalanced parentheses.
Part 2: Implementing a Scheduler Using a Queue
Problem Overview
Operating systems often need to manage multiple processes, ensuring each process gets a fair share of CPU time. This problem can be simplified using a queue, a First-In, First-Out (FIFO) data structure.
Understanding Queues
A queue is a FIFO data structure, meaning the first element added is the first one to be removed. Queues are ideal for scheduling problems where order and fairness are essential.
Steps to Solve the Problem
1. Class Design:
- Scheduler Class: Contains a queue of processes and methods to add processes, get the next process, and check if the queue is empty.
- PendingProcess Structure: Holds process details, including name and remaining execution time.
2. Algorithm Description:
- Reading Process List: Read a list of pending processes from a file.
- Adding Processes to Scheduler: Add each process to the scheduler's queue.
- Scheduling Processes: Continuously fetch the next process to run, decrement its remaining time, and re-add it to the queue if it still has time remaining.
3. Edge Cases and Validation:
- Empty Queue: Ensure the queue is not empty before fetching the next process.
- Process Execution Completion: Remove processes from the queue once their execution time is zero.
Sample Implementation in C++
Here's a detailed C++ implementation to manage process scheduling using a queue:
#include <iostream>
#include <queue>
#include <string>
#include <vector>
#include <fstream>
// Structure to represent a pending process
struct PendingProcess {
std::string name;
int timeRemaining;
PendingProcess(const std::string &n, int t) : name(n), timeRemaining(t) {}
};
// Class to represent a Scheduler
class Scheduler {
private:
std::queue<PendingProcess> processQueue; // Queue to store processes
public:
// Method to add a process to the queue
void add(const PendingProcess &process) {
processQueue.push(process);
}
// Method to get the next process to run
std::string next() {
if (processQueue.empty()) return ""; // Return empty string if no processes
PendingProcess current = processQueue.front();
processQueue.pop();
current.timeRemaining -= 1;
if (current.timeRemaining > 0) {
processQueue.push(current); // Re-add process if it still has time remaining
}
return current.name;
}
// Method to check if the queue is empty
bool empty() const {
return processQueue.empty();
}
};
// Function to read a list of pending processes from a file
std::vector<PendingProcess> readPendingProcessList(const std::string &filename) {
std::vector<PendingProcess> processes;
std::ifstream file(filename);
std::string name;
int time;
while (file >> name >> time) {
processes.push_back(PendingProcess(name, time));
}
return processes;
}
int main() {
std::string filename = "process_list.txt"; // Specify the input file
std::vector<PendingProcess> processes = readPendingProcessList(filename);
Scheduler scheduler;
for (const auto &process : processes) {
scheduler.add(process); // Add all processes to the scheduler
}
int second = 0;
while (!scheduler.empty()) {
std::cout << "Second " << second << ": " << scheduler.next() << std::endl;
second++;
}
return 0;
}
Detailed Breakdown of the Code
1. Header Files:
- #include <iostream>, #include <queue>, #include <string>, #include <vector>, and #include <fstream> are necessary for input/output operations, queue usage, string manipulation, vector storage, and file reading.
2. PendingProcess Structure:
- The structure holds process details, including name and remaining execution time.
3. Scheduler Class:
- Private Field: std::queue<PendingProcess> processQueue; stores the processes.
- Public Methods:
- void add(const PendingProcess &process) adds a process to the queue.
- std::string next() fetches the next process to run, decrements its time, and re-adds it if time remains.
- bool empty() const checks if the queue is empty.
4. Reading Process List:
- std::vector<PendingProcess> readPendingProcessList(const std::string &filename) reads processes from a file and returns a vector of PendingProcess objects.
5. Main Function:
- Reads the list of processes from a file, adds them to the scheduler, and continuously prints the process scheduled to run each second until the queue is empty.
Conclusion
By mastering stacks and queues, you gain powerful tools for solving a variety of C++ programming assignments. Whether you're dealing with complex expressions or scheduling tasks, understanding how to apply these data structures effectively is key to developing robust and efficient solutions. Practice with these concepts will enhance your problem-solving skills and prepare you for more advanced topics in computer science.