Claim Your Offer
New semester, new challenges—but don’t stress, we’ve got your back! Get expert programming assignment help and breeze through Python, Java, C++, and more with ease. For a limited time, enjoy 10% OFF on all programming assignments with the Spring Special Discount! Just use code SPRING10OFF at checkout! Why stress over deadlines when you can score high effortlessly? Grab this exclusive offer now and make this semester your best one yet!
We Accept
- 1. Understand the Problem Requirements
- 2. Plan the Data Flow and Design
- 3. Implementing Efficient Algorithms
- 4. Testing and Debugging
- 5. Documentation
- Key Takeaways:
- Conclusion:
When tasked with a programming assignment that involves data structures, algorithms, and real-world data, such as analyzing historical stock market trends, students often face several challenges that require both technical proficiency and strategic problem-solving skills. These types of assignments not only demand a deep understanding of core computer science concepts—such as how to efficiently manipulate and process large datasets using appropriate algorithms and data structures—but also the ability to apply these concepts in a practical, real-world context where performance and efficiency are critical. Additionally, students must ensure that their solutions adhere to specific constraints, such as optimizing for time complexity, often with a requirement like linear runtime O(n), and minimizing memory usage to handle large volumes of data effectively.
One of the biggest hurdles students encounter is managing the complexity of real-world data while balancing the technical limitations of the programming language they are using, such as Java. It is essential to select or design custom data structures that are both efficient and tailored to the problem at hand. Furthermore, students need to develop robust algorithms that can process data in a single pass, avoiding multiple iterations over the dataset, which could lead to performance bottlenecks. Debugging and testing these solutions also become critical, as real-world datasets often contain anomalies or edge cases that could break a poorly constructed program.
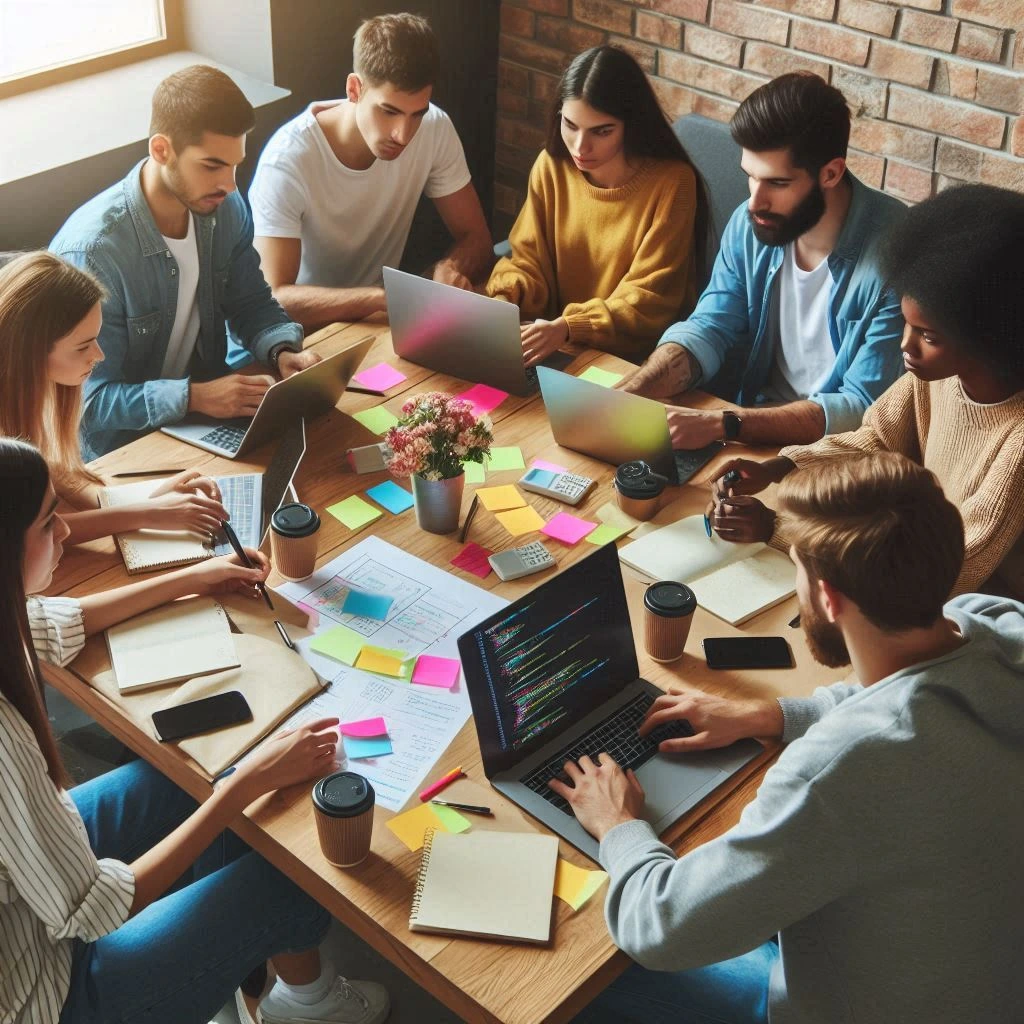
Beyond the technical aspects, students must also consider the broader scope of the assignment. This includes understanding the problem domain, identifying the key metrics required for output (such as percentage changes, streaks of gains or losses, etc.), and ensuring their program delivers accurate results while meeting the required specifications. In this blog, we will explore a structured and methodical approach to tackling these complex programming assignments. We will discuss how to break down the problem into manageable components, choose the right data structures, implement efficient algorithms, and thoroughly test the solution—all while maintaining clarity, organization, and performance. By following this approach, you can effectively utilize a Java assignment helper to meet the requirements without feeling overwhelmed or frustrated.
1. Understand the Problem Requirements
The first and most important step in tackling any programming assignment is to thoroughly understand the problem's requirements. This foundational step ensures that you are clear on the objectives, the expected outcomes, and the constraints of the problem. For example, in an assignment that involves analyzing the Dow Jones Industrial Average (DJIA) data over a long period, you might be tasked with identifying trends, such as the largest percentage one-day increases, the biggest drops, or the longest streaks of positive and negative gains. Without a clear understanding of what is expected, it is easy to go off course or overlook key elements of the assignment. Seeking help with programming homework can provide valuable insights and clarify any uncertainties, allowing you to stay focused and on track.
In assignments like these, pay close attention to the following key factors:
- Input Data: First, ensure you understand the format and structure of the input data file (in this case, a .csv file that contains financial data). Knowing the format of each field—such as dates, opening and closing prices, highs, and lows—is essential to correctly extracting and processing the data. Also, check if the data needs to be processed directly from the file or whether certain manipulations (such as filtering or sorting) are necessary.
- Output Requirements: It’s crucial to clearly outline what specific outputs are expected from the program. For instance, the assignment might ask for specific outputs like the 10 largest percentage changes, the longest streaks of positive or negative gains, and the dates on which they occurred. Understanding these outputs will guide the logic you implement in your algorithms.
- Constraints: Every programming problem comes with constraints that you must follow. In this case, you may be required to implement your solution in linear time O(n) meaning that your algorithm must traverse the data efficiently, ideally in a single pass. This constraint emphasizes the need to focus on algorithmic efficiency, avoiding unnecessary complexity or repeated iterations over the dataset.
By thoroughly understanding the problem’s requirements, input data, expected outputs, and constraints, you can lay a strong foundation for the next steps in the assignment, ensuring that your approach is both focused and efficient.
2. Plan the Data Flow and Design
Before diving into coding, it's essential to create a well-thought-out plan for how data will flow through your program and how your solution will be designed. Taking time to structure your approach upfront helps to ensure your solution is both efficient and scalable. Here are several important considerations when planning your data flow and design:
- Data Reading: In assignments involving large datasets, such as the Dow Jones Industrial Average (DJIA) data spanning decades, loading the entire file into memory can be inefficient and may exceed memory limits. A better approach is to read the file line by line. By doing so, you can process each record independently without overloading memory. This ensures that your program can handle large files efficiently, while also meeting any space constraints imposed by the problem.
- Choosing a Data Structure: One of the most critical decisions in solving a programming problem is selecting the right data structure. The choice depends on the specific requirements of the problem, such as the need to efficiently track the largest changes, streaks, or differences. For instance:
- Arrays: You might use arrays to store a fixed number of values (e.g., the top 10 percentage changes). Arrays are a good choice when you need constant-time access to elements, and you know the number of values you're working with in advance.
- Linked Lists: If the size of the data you’re tracking is not fixed, a linked list could be useful. This structure allows dynamic growth, but it's important to remember that accessing elements in a linked list is slower compared to arrays due to the need for traversal.
- Custom Data Structures: You might need to design custom data structures to handle the specific needs of the problem. For example, a priority queue or a min-heap could be ideal for keeping track of the top 10 largest changes in percentage, allowing you to efficiently insert new values and remove the smallest ones as new larger values are found.
- Minimizing Memory Usage: Since the problem specifies minimizing memory space, you should avoid retaining unnecessary data as you process each record. For example, instead of keeping every data point from the dataset, you can focus on storing only the relevant information—such as the current top 10 percentage changes, the current longest streaks, or the maximum percentage differences between highs and lows.
- Efficient Operations: Your chosen data structure should allow for easy insertion, comparison, and replacement of values as you parse through the records. For instance, if you're tracking the top 10 largest percentage changes, your data structure should allow you to compare new values against the smallest of the top 10, replacing it if the new value is larger. This ensures that your program performs efficiently, even when processing large datasets.
By planning the data flow and carefully selecting the right data structures, you set the stage for an optimized and efficient solution that adheres to the problem’s requirements while minimizing resource usage.
3. Implementing Efficient Algorithms
Efficiency is often a key requirement in real-world programming assignments, and ensuring that your solution adheres to time and space constraints is crucial. In many cases, you will need to process large datasets while maintaining both performance and accuracy. To achieve this, it's important to design an algorithm that operates efficiently, in this case with a linear time complexity O(n)O(n)O(n). Below are some essential strategies for implementing an efficient algorithm:
- One-Pass Algorithm: Instead of making multiple passes over the dataset, aim to design an algorithm that processes each piece of data as it is read. For example, while reading the DJIA data, calculate and update the top 10 largest one-day increases, decreases, and streaks in real-time, as the data flows in. This prevents the need for a second pass and ensures that the runtime complexity remains linear, as the number of operations is directly proportional to the size of the dataset.
- Updating on the Fly: As you parse each line of the data file, continuously update the metrics you are tracking. For instance, every time a new record is read, immediately compare its one-day percentage change with the current smallest entry in your list of top 10 largest changes. If the new value is larger, replace the smallest entry with the new one. Similarly, keep a running tally of the longest streaks of positive or negative gains and update these values as necessary. By processing the data on the fly, you avoid the need to store all records in memory, ensuring both memory efficiency and optimal performance.
- Efficient Data Structures: To support these real-time updates, use efficient data structures such as heaps or arrays. A min-heap could be ideal for maintaining the top 10 largest values, as it allows for constant time insertion and extraction of the smallest element. This approach ensures that your program remains responsive even with large datasets.
By designing your algorithm to process the data in a single pass and updating necessary values as you go, you ensure that your solution not only meets the performance requirements but also handles large datasets efficiently.
4. Testing and Debugging
Testing is a critical step in verifying the accuracy and performance of your code. After implementing your algorithm, you should create a smaller, manageable dataset from the DJIA data to test your solution. This allows you to manually calculate the expected results and compare them with your program’s output, ensuring that your logic is correct.
- Edge Cases: Always consider potential edge cases in your testing. For example, some days might show no change in stock value, or the dataset could contain very few records. Your code should be able to handle these cases without errors. Consider writing tests to ensure that the program behaves correctly when the input data is minimal or when specific conditions (like no price changes) occur.
- Debugging: As part of the testing process, thoroughly document your test cases and the results. This includes capturing screenshots of your test runs and explaining why the results match (or don’t match) the expected outputs. This documentation is not only useful for debugging but also demonstrates that you have rigorously tested your solution before submission.
By preparing test cases, particularly for edge scenarios, and documenting the debugging process, you ensure that your program is robust and can handle any unexpected inputs effectively.
5. Documentation
Proper documentation is an essential part of writing maintainable and understandable code. While working on complex assignments involving algorithms, custom data structures, or unique problem-solving approaches, it's important to include clear and concise comments throughout your code.
- Commenting: As you write your program, include comments explaining the purpose of each major section, as well as specific logic where necessary. Describe your thought process and why certain decisions were made (e.g., why you chose a particular data structure or algorithm). Clear documentation helps others understand your solution and allows you to revisit the code later with ease.
- Code Structure: Organize your code into logical sections, with each function or method clearly dedicated to a specific task. Use descriptive variable names that reflect their purpose within the code. Well-organized code, combined with clear comments, ensures that your solution is easy to read and maintain.
By documenting your code thoroughly, you enhance its clarity and make it easier for others (such as your instructor or future collaborators) to understand your approach and the logic behind your solution.
Key Takeaways:
- Understand the problem thoroughly: Begin by gaining a clear understanding of the problem requirements and output expectations.
- Optimize memory and time: Design a solution that minimizes memory usage and ensures optimal time complexity, especially for large datasets.
- Use one-pass algorithms: Implement algorithms that process data in a single pass, updating relevant values on the fly to improve efficiency.
- Test with smaller datasets: Use smaller, manageable datasets to thoroughly test your solution, including edge cases, to ensure accuracy.
- Document clearly: Write clear, concise documentation to explain your code, making it easy to understand and maintain for future use.
Conclusion:
When faced with a challenging programming assignment like analyzing historical data, it’s easy to feel daunted. Imagine a scenario where you’re navigating through decades of stock market data, hunting for trends and insights. At first glance, it seems like a monumental task—but by following a methodical approach, you can transform this overwhelming challenge into a manageable project.
The journey begins with a deep understanding of the problem. Much like a detective gathering clues, you need to focus on the input data, output expectations, and constraints. Once you have the full picture, it’s time to design your plan. Think of it like mapping out a route before setting off on a hike—without a clear path, you could easily lose your way. You streamline your design to ensure you’re using memory efficiently and processing each piece of data just once, like packing only the essentials for your journey.
When it’s time to implement, your one-pass algorithms come into play—collecting insights as you read the data line by line, much like a miner sifting through rock to extract gold. You don’t need to store everything, only what’s valuable. The power of an efficient algorithm lies in its ability to process huge amounts of data without getting bogged down.
And of course, every good adventurer tests their equipment before embarking on the real journey. You test your code on smaller datasets, preparing for the unexpected twists and turns. With your solution tested and refined, you’re ready to face the final hurdle: documenting the adventure. Clear documentation is the story you leave behind, ensuring that anyone who follows in your footsteps knows exactly how to navigate the path.
In the end, the assignment that seemed impossible has been broken down, understood, and conquered. By applying these principles—problem comprehension, efficient design, thorough testing, and careful documentation—you’re not just completing an assignment; you’re mastering a valuable process that will serve you in any future programming challenge.