Claim Your Discount Today
Ring in Christmas and New Year with a special treat from www.programminghomeworkhelp.com! Get 15% off on all programming assignments when you use the code PHHCNY15 for expert assistance. Don’t miss this festive offer—available for a limited time. Start your New Year with academic success and savings. Act now and save!
We Accept
- Understanding the Problem Statement
- Carefully Reading the Requirements
- Breaking Down the Problem
- Planning Your Approach
- Reviewing the Starter Code and Existing Structures
- Understanding the Provided Code
- Linked List Structures
- Leveraging Existing Functions
- Implementing the Core Components
- Implementing Linked List Operations
- Handling File I/O Operations
- Working with Bitwise Operations
- Testing, Debugging, and Optimization
- Testing Your Code
- Debugging Your Code
- Optimizing Your Code
- Conclusion,
Programming assignments can be complex and challenging, especially when they involve intricate concepts such as linked lists, file I/O, and bitwise operations. These assignments test your understanding of both theoretical and practical aspects of programming, pushing you to apply your knowledge in real-world scenarios. However, with a structured approach and a clear understanding of the core principles, you can effectively solve your C assignment, even if it seems daunting.
In this blog, we will explore how to approach and solve programming assignments that involve linked lists, file I/O, and bitwise operations. We will break down the process into manageable steps, helping you navigate through each phase of the assignment. By the end of this guide, you should feel confident in your ability to handle similar assignments, armed with a deeper understanding of the concepts and techniques required.
Understanding the Problem Statement
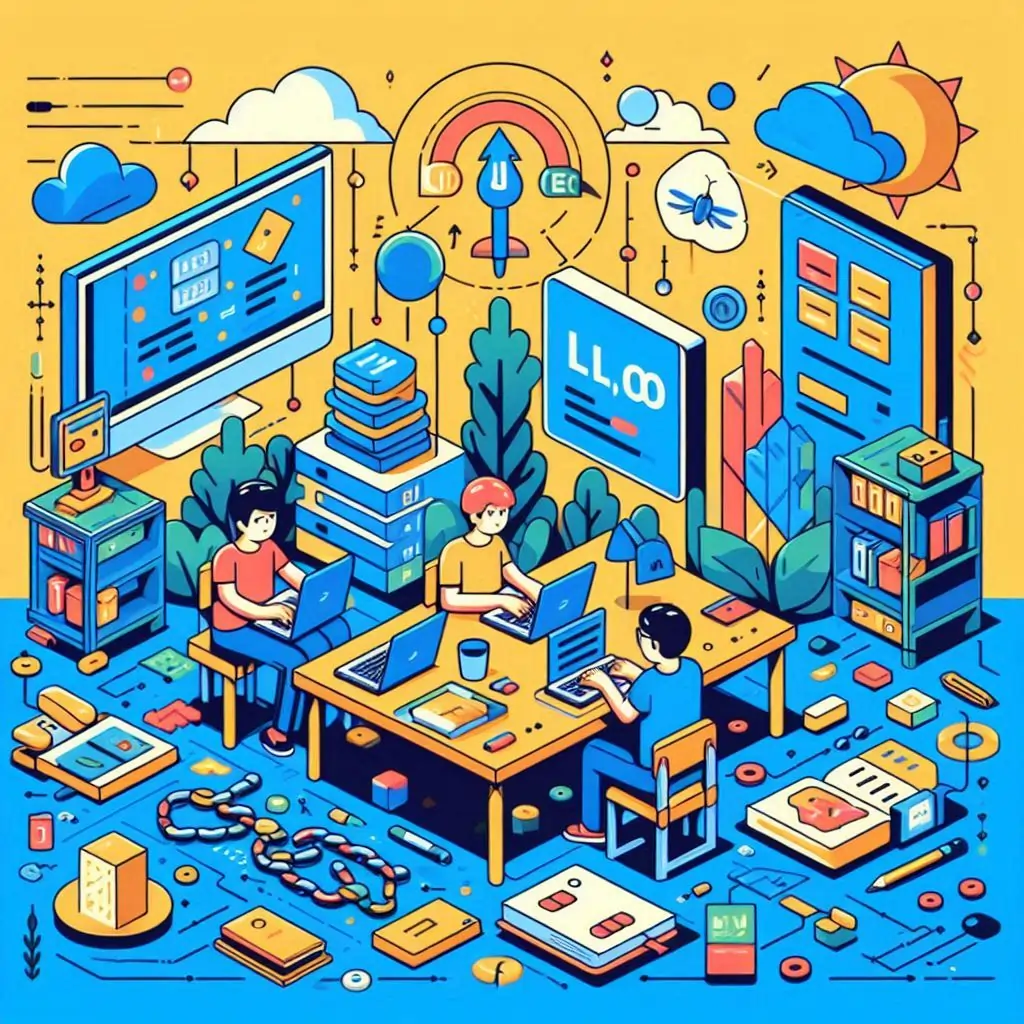
The first and most crucial step in solving any programming assignment is to thoroughly understand the problem statement. This section will guide you through the process of breaking down the problem, analyzing the requirements, and planning your approach.
Carefully Reading the Requirements
The first step in solving a programming assignment is to carefully read and understand the requirements. This might seem obvious, but many students overlook this crucial step and rush into coding without fully grasping what is being asked of them.
When you receive a programming assignment, take your time to read through the entire problem statement. Pay close attention to the key tasks you need to accomplish. For example, in an assignment involving linked lists, file I/O, and bitwise operations, you might be required to implement functions for manipulating linked lists, reading and parsing files, and performing bitwise operations on data.
It's important to identify the specific tasks and the order in which they need to be completed. For instance, you might first need to implement basic linked list operations before moving on to file I/O or bitwise operations. Understanding the sequence of tasks will help you plan your approach and ensure that you don’t miss any critical steps.
Breaking Down the Problem
Once you have a clear understanding of the requirements, the next step is to break down the problem into smaller, more manageable tasks. This approach makes it easier to tackle complex assignments by focusing on one task at a time.
For example, let’s say your assignment involves implementing a linked list to store data read from a file. You can break down the problem into the following tasks:
- Implement basic linked list operations, such as adding, searching, and deleting nodes.
- Write functions to open and read data from a file.
- Parse the data and store it in the linked list.
- Implement bitwise operations to manipulate the data as required.
By breaking down the problem in this way, you can approach each task individually, making the overall assignment less overwhelming. Additionally, this method allows you to test and debug each part of your code incrementally, ensuring that each component works correctly before moving on to the next.
Planning Your Approach
With the problem broken down into smaller tasks, the next step is to plan your approach. This involves deciding on the tools, techniques, and algorithms you will use to solve the problem.
Start by considering the data structures you will need. In assignments involving linked lists, you’ll need to understand how linked lists work, including how nodes are created, linked, and manipulated. If the assignment involves file I/O, you’ll need to be familiar with file handling functions in your chosen programming language. For bitwise operations, you should review the relevant operators and how they can be applied to manipulate data.
Once you have a clear idea of the data structures and algorithms required, outline your plan step by step. This plan will serve as a roadmap, guiding you through the implementation process and helping you stay on track.
Reviewing the Starter Code and Existing Structures
Many programming assignments provide starter code or predefined structures that you need to work with. Understanding this code is essential for successfully completing the assignment. This section will cover how to review and understand the starter code, with specific emphasis on linked list structures.
Understanding the Provided Code
Before you start writing your own code, it’s crucial to thoroughly review any starter code provided with the assignment. This code often includes essential structures and functions that you’ll need to build upon, so understanding it is key to your success.
Begin by examining the header files and any existing source code files. For instance, if the assignment involves a linked list, you might find a file like lc4_memory.h that defines the structure of a linked list node. This file might also include function prototypes for basic linked list operations, such as adding or searching for nodes.
Take your time to understand these structures and functions. Look at the data types used, the parameters passed to each function, and how the functions are intended to work together. If there are comments or documentation within the code, read them carefully to gain insight into the purpose of each component.
Understanding the provided code will not only help you avoid errors but also give you a solid foundation to build upon. It can also provide hints about the best way to approach the assignment, as the starter code is often designed to guide you toward the correct solution.
Linked List Structures
Linked lists are a common data structure used in many programming assignments, particularly those involving dynamic data management. Understanding the structure of a linked list is essential for implementing and manipulating it effectively.
A linked list consists of nodes, where each node contains data and a pointer to the next node in the list. In some cases, nodes may also include additional fields, such as pointers to previous nodes (in a doubly linked list) or extra data fields.
For example, in the assignment you provided, the linked list nodes might be defined in a structure like this:
typedef struct row_of_memory {
unsigned short address;
char* label;
unsigned short contents;
char* assembly;
struct row_of_memory* next;
} row_of_memory;
In this structure:
- address stores the memory address.
- label is a string that stores a label for the memory address.
- contents holds the actual data at the memory address.
- assembly stores the disassembled assembly code corresponding to the contents.
- next is a pointer to the next node in the linked list.
Understanding this structure is crucial because it defines how you will store and access data within the linked list. When implementing functions to manipulate the linked list, you’ll need to consider how to update these fields, how to traverse the list, and how to insert or delete nodes.
Leveraging Existing Functions
Many assignments provide starter functions that are partially implemented or that serve as placeholders for you to complete. Leveraging these functions effectively can save you time and effort.
For example, you might be given a function prototype for adding a node to the linked list:
void add_to_list(row_of_memory** head, unsigned short address, unsigned short contents);
Your task might be to implement the logic within this function. Since the function prototype and parameters are already defined, you can focus on writing the code that adds a new node to the list.
When implementing such functions, consider how they will interact with other parts of the code. Ensure that your implementation is consistent with the rest of the program and that it adheres to any guidelines provided in the assignment.
By understanding and leveraging existing functions, you can streamline your coding process and ensure that your implementation aligns with the overall structure of the assignment.
Implementing the Core Components
Once you have a solid understanding of the problem and the existing code, it’s time to start implementing the core components of your solution. This section will guide you through implementing linked list operations, handling file I/O, and working with bitwise operations.
Implementing Linked List Operations
Linked lists are a fundamental data structure in computer science, and implementing them is a common requirement in programming assignments. In this section, we’ll explore how to implement the core linked list operations that you’ll likely need.
Adding Nodes to the Linked List
The first and most basic operation in a linked list is adding nodes. In most cases, you’ll need to add nodes in a specific order, such as in ascending order of memory addresses.
To add a node to the linked list, you’ll need to allocate memory for the new node, populate its fields with the appropriate data, and then insert it into the list at the correct position. Here’s a basic outline of how to do this:
- Allocate Memory: Use a function like malloc to allocate memory for the new node.
- Populate Fields: Set the address, contents, label, and assembly fields based on the data you’re adding.
- Insert Node: Traverse the linked list to find the correct position for the new node, then update the pointers to insert it into the list.
Here’s an example of what this might look like in code:
void add_to_list(row_of_memory** head, unsigned short address, unsigned short contents) {
row_of_memory* new_node = malloc(sizeof(row_of_memory));
new_node->address = address;
new_node->contents = contents;
new_node->label = NULL;
new_node->assembly = NULL;
new_node->next = NULL;
if (*head == NULL || (*head)->address > address) {
new_node->next = *head;
*head = new_node;
} else {
row_of_memory* current = *head;
while (current->next != NULL && current->next->address < address) {
current = current->next;
}
new_node->next = current->next;
current->next = new_node;
}
}
This function adds a new node to the linked list, maintaining the order of nodes by address.
Searching for Nodes
Another common operation is searching for nodes within the linked list. This might be necessary when you need to find a node with a specific address or when you need to update a node’s contents.
Searching a linked list typically involves traversing the list and comparing each node’s address (or other fields) with the target value. If a match is found, you can return a pointer to the node; otherwise, return NULL.
Here’s an example of a search function:
row_of_memory* search_list(row_of_memory* head, unsigned short address) {
row_of_memory* current = head;
while (current != NULL) {
if (current->address == address) {
return current;
}
current = current->next;
}
return NULL;
}
This function traverses the list and returns a pointer to the node with the matching address.
Deleting Nodes
Finally, you might need to delete nodes from the linked list. This operation involves updating the pointers of the surrounding nodes to remove the target node from the list and then freeing the memory allocated for that node.
Here’s an example of a delete function:
void delete_node(row_of_memory** head, unsigned short address) {
row_of_memory* current = *head;
row_of_memory* previous = NULL;
while (current != NULL && current->address != address) {
previous = current;
current = current->next;
}
if (current == NULL) {
return; // Node not found
}
if (previous == NULL) {
*head = current->next; // Node to be deleted is the head
} else {
previous->next = current->next; // Bypass the node to be deleted
}
free(current);
}
This function removes a node with a specific address from the linked list and frees the associated memory.
Handling File I/O Operations
File input/output (I/O) is a common requirement in programming assignments, especially when you need to read data from files and store it in a data structure like a linked list. This section will cover how to implement file I/O operations effectively.
Opening and Reading Files
The first step in handling file I/O is opening the file you need to read. In C, you can use the fopen function to open a file, specifying the mode (e.g., "r" for reading). If the file cannot be opened, it’s important to handle the error gracefully and provide an appropriate error message.
Once the file is open, you can read its contents using functions like fgets or fscanf, depending on the format of the data. Here’s an example of how to open a file and read its contents line by line:
FILE* file = fopen("data.txt", "r");
if (file == NULL) {
printf("Error opening file.\n");
return;
}
char buffer[256];
while (fgets(buffer, sizeof(buffer), file) != NULL) {
printf("%s", buffer);
}
fclose(file);
This code opens a file named "data.txt", reads its contents line by line, and prints each line to the console.
Parsing File Data
Once you’ve read data from a file, the next step is to parse that data and store it in your linked list. Parsing involves extracting the relevant information from each line of the file and using it to populate the fields of your linked list nodes.
For example, if each line of the file contains an address and a corresponding value, you might parse the data as follows:
unsigned short address;
unsigned short contents;
if (sscanf(buffer, "%hx %hx", &address, &contents) == 2) {
add_to_list(&head, address, contents);
}
In this code, sscanf is used to extract two hexadecimal values from the buffer, which are then passed to the add_to_list function to create a new node in the linked list.
Working with Bitwise Operations
Bitwise operations are an essential part of many programming assignments, especially those that involve low-level data manipulation or working with binary data. This section will cover how to perform bitwise operations and apply them to your assignment.
Understanding Binary Representation
Before diving into bitwise operations, it’s important to understand how data is represented in binary. Each bit in a binary number represents a power of 2, with the rightmost bit being the least significant bit (LSB) and the leftmost bit being the most significant bit (MSB).
For example, the binary number 1101 represents the decimal value 13:
- The LSB is 1 (2^0 = 1)
- The next bit is 0 (2^1 = 2)
- The next bit is 1 (2^2 = 4)
- The MSB is 1 (2^3 = 8)
Adding these values together gives 8 + 4 + 0 + 1 = 13.
Understanding this binary representation is crucial for performing bitwise operations, as it allows you to manipulate individual bits within a number.
Using Bitwise Operators
In C, several bitwise operators allow you to manipulate individual bits within a number:
- & (AND): Compares each bit of two numbers and returns a 1 only if both bits are 1.
- | (OR): Compares each bit of two numbers and returns a 1 if either bit is 1.
- ^ (XOR): Compares each bit of two numbers and returns a 1 if the bits are different.
- ~ (NOT): Inverts all the bits in a number.
- << (left shift): Shifts all the bits in a number to the left by a specified number of positions.
- >> (right shift): Shifts all the bits in a number to the right by a specified number of positions.
These operators are incredibly powerful and can be used for tasks like setting, clearing, or toggling specific bits within a number. Here’s an example of how to use some of these operators:
unsigned short value = 0x0F; // 00001111 in binary
value = value << 2; // Shift left by 2: 00111100
value = value & 0x3C; // AND with 00111100: 00111100
value = value | 0x03; // OR with 00000011: 00111111
In this example, the value is first shifted left by two positions, then masked with 0x3C to clear the unwanted bits, and finally combined with 0x03 to set the last two bits.
Applying Bitwise Operations to Your Assignment
Bitwise operations are often used in programming assignments to manipulate data at the bit level. For example, you might need to extract specific bits from an instruction word to determine the opcode or the operand.
Here’s an example of how to extract an opcode from a 16-bit instruction:
unsigned short instruction = 0xA123; // Example instruction
unsigned short opcode = (instruction >> 12) & 0xF; // Extract the top 4 bits
In this code, the instruction is first shifted right by 12 bits, bringing the top 4 bits (the opcode) into the LSB positions. The result is then masked with 0xF to isolate those 4 bits.
Testing, Debugging, and Optimization
Once you’ve implemented the core components of your assignment, the next step is to test, debug, and optimize your code. This section will guide you through these critical stages of development.
Testing Your Code
Testing is an essential part of the development process. It ensures that your code works as expected and helps you identify and fix any issues before they become problematic.
Testing Incrementally
One of the most effective testing strategies is to test your code incrementally. Instead of waiting until the entire assignment is complete, test each component as you implement it.
For example, after implementing the add_to_list function for your linked list, write a simple test program that creates a linked list, adds a few nodes, and then prints the list to verify that the nodes are being added correctly.
By testing incrementally, you can catch errors early and avoid the frustration of trying to debug a large, complex program all at once.
Writing Test Cases
To test your code effectively, you’ll need to write test cases that cover a range of scenarios, including both typical and edge cases. For instance, when testing a linked list implementation, you should write test cases for:
- Adding nodes to an empty list
- Adding nodes to the beginning, middle, and end of the list
- Searching for nodes that exist and don’t exist in the list
- Deleting nodes from the beginning, middle, and end of the list
- Handling cases where the list is empty
By covering a wide range of scenarios, you can ensure that your code is robust and handles all possible inputs correctly.
Debugging Your Code
Despite your best efforts, bugs are likely to creep into your code. When this happens, effective debugging techniques are crucial for identifying and fixing the issues.
Using Debugging Tools
There are many debugging tools available that can help you find and fix bugs in your code. One of the most commonly used tools is gdb, the GNU Debugger. gdb allows you to step through your code line by line, inspect the values of variables, and set breakpoints to pause execution at specific points.
Here’s a basic example of how to use gdb:
gcc -g -o myprogram myprogram.c
gdb ./myprogram
In gdb, you can set a breakpoint at the beginning of the main function with the command break main, and then start the program with the command run. As you step through the code with the next command, you can inspect variables using the print command, e.g., print myvariable.
Using gdb or similar tools can significantly speed up the debugging process by allowing you to pinpoint the exact location and cause of a bug.
Analyzing Error Messages
Error messages and warnings from your compiler or runtime environment can provide valuable clues about what went wrong. Instead of ignoring these messages, take the time to analyze them and understand what they mean.
For example, if you receive a segmentation fault, it typically indicates that your program is trying to access memory that it shouldn’t. This could be due to an out-of-bounds array access, dereferencing a null pointer, or other memory-related issues.
By carefully analyzing error messages, you can often quickly identify the root cause of a bug and take steps to fix it.
Optimizing Your Code
Once your code is working correctly, the final step is to optimize it for performance. Optimization involves making your code run faster, use less memory, or be more efficient in other ways.
Identifying Bottlenecks
The first step in optimization is to identify bottlenecks in your code. These are the parts of your program that are consuming the most time or resources. You can use profiling tools like gprof to analyze your code and identify these bottlenecks.
Once you’ve identified the bottlenecks, you can focus your optimization efforts on these areas. For example, if you find that a particular loop is taking up a significant amount of time, you might be able to optimize it by reducing the number of iterations or by using a more efficient algorithm.
Optimizing Data Structures
Choosing the right data structures can have a significant impact on the performance of your code. For instance, if your linked list implementation is slow, you might consider switching to a different data structure, such as a hash table, if it’s more appropriate for the problem at hand.
Similarly, if you’re working with large amounts of data, consider using more memory-efficient data structures or algorithms to reduce your program’s memory footprint.
Code Refactoring
Finally, consider refactoring your code to make it cleaner, more efficient, and easier to maintain. Refactoring involves restructuring your code without changing its external behavior. This might involve breaking up large functions into smaller, more manageable ones, eliminating duplicate code, or improving the readability of your code.
By refactoring your code, you can often improve its performance and maintainability without making major changes.
Conclusion,
Successfully completing programming assignments involving linked lists, file I/O, and bitwise operations requires a clear understanding of the problem, careful planning, and diligent testing and debugging. By following the steps outlined in this guide, you can approach these assignments with confidence, knowing that you have the tools and techniques needed to succeed. Whether you’re adding nodes to a linked list, reading data from a file, or performing bitwise operations, the key is to break the problem down into manageable tasks, test your code thoroughly, and continuously refine your approach. Utilizing a programming assignment helper can also provide valuable insights and guidance as you develop the skills needed to tackle even the most complex programming challenges. With practice and persistence, you’ll be well-equipped to succeed.