Claim Your Offer
New semester, new challenges—but don’t stress, we’ve got your back! Get expert programming assignment help and breeze through Python, Java, C++, and more with ease. For a limited time, enjoy 10% OFF on all programming assignments with the Spring Special Discount! Just use code SPRING10OFF at checkout! Why stress over deadlines when you can score high effortlessly? Grab this exclusive offer now and make this semester your best one yet!
We Accept
- Understanding the Assignment Requirements
- Reading the Assignment Brief
- Planning Your Approach
- Setting Up Your Development Environment
- Designing the Game Board
- Drawing the Game Board
- Implementing the Game Logic
- Drawing Players and Alternating Turns
- Implementing Special Features
- Adding Ladders and Snakes
- Implementing Double Moves and Extra Turns
- Handling Special Conditions
- Finalizing and Testing Your Game
- Testing for Bugs and Edge Cases
- Commenting and Documenting Your Code
- Following Submission Guidelines
- Detailed Steps to Approach a Board Game Programming Assignment
- Setting Up Your Development Environment
- Designing the Game Board
- Implementing Special Features
- Finalizing and Testing Your Game
- Tips for Success in Board Game Programming Assignments
- Stay Organized
- Test Incrementally
- Seek Feedback
- Practice Regularly
Programming assignments involving board games offer an exciting and educational way to enhance your coding skills. These projects require you to implement complex logic, nested loops, and graphical elements, making them an excellent exercise for budding programmers. In this blog, we'll explore a general approach to solve python assignments. This guide will help you navigate any similar board game programming task by providing a structured method and practical tips.
Understanding the Assignment Requirements
Before diving into the coding process, it's crucial to understand the assignment requirements thoroughly. This step will set the foundation for a successful project.
Reading the Assignment Brief
Carefully read the assignment brief to identify key tasks and requirements. Look for details about the grid size, types of dice, and specific game features you need to implement. For example, an assignment might ask you to:
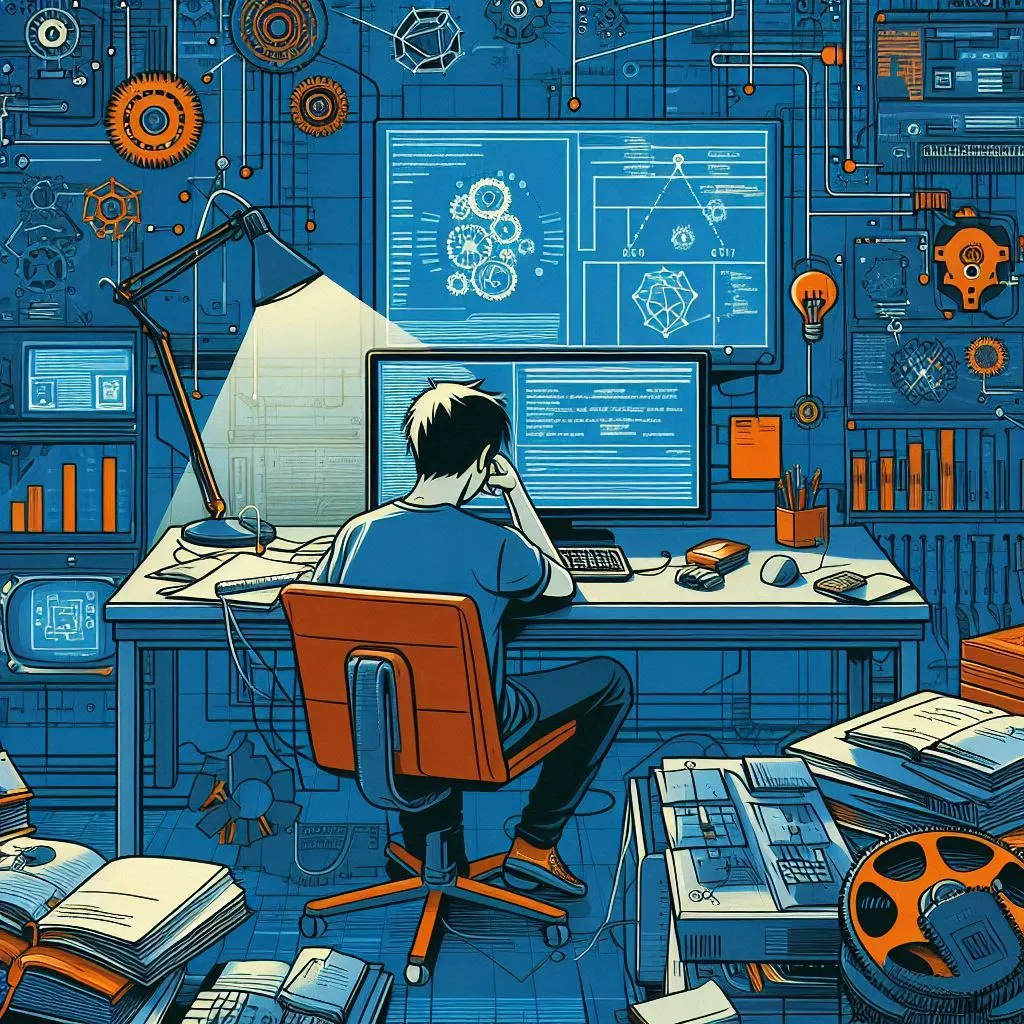
- Choose the grid size from options like 42, 56, 72, 90, 110, 132, 156.
- Decide on the number and type of dice.
- Implement at least two features such as ladders, snakes, double moves, or extra turns.
Planning Your Approach
Planning is essential to avoid confusion later. Break down the project into manageable steps. Decide on the grid size, dice type, and game features you want to implement. Sketch out the game logic and design on paper or a whiteboard before coding.
Setting Up Your Development Environment
Ensure your development environment is ready. For Python-based projects, you'll need libraries like pygame for graphics and random for dice rolls. Install these libraries and set up your coding environment to streamline your workflow.
import pygame
import random
Designing the Game Board
Once you've understood the requirements and planned your approach, it's time to start designing the game board. This involves creating a visual representation of the board and implementing the game logic.
Drawing the Game Board
Use nested loops to create the grid for your game board. pygame is a popular library for drawing graphics in Python. The following code snippet demonstrates how to draw a simple grid using pygame.
def draw_board(screen, grid_size):
for row in range(grid_size):
for col in range(grid_size):
pygame.draw.rect(screen, (255, 255, 255), (col * 20, row * 20, 20, 20), 1)
Implementing the Game Logic
Define the core logic of your game using loops and conditionals. A while loop can run the game until a player reaches the end. The game logic will include rolling dice, moving players, and checking for special conditions like ladders or snakes.
player1_position = 0
player2_position = 0
current_player = 1
while player1_position < grid_size ** 2 and player2_position < grid_size ** 2:
if current_player == 1:
roll = random.randint(1, 6) # Rolling a 6-sided dice
player1_position += roll
current_player = 2
else:
roll = random.randint(1, 6)
player2_position += roll
current_player = 1
Drawing Players and Alternating Turns
Keep track of each player’s position and alternate turns. Use pygame to draw the players on the board based on their positions.
def draw_players(screen, player1_position, player2_position, grid_size):
player1_row = player1_position // grid_size
player1_col = player1_position % grid_size
pygame.draw.circle(screen, (255, 0, 0), (player1_col * 20 + 10, player1_row * 20 + 10), 10)
player2_row = player2_position // grid_size
player2_col = player2_position % grid_size
pygame.draw.circle(screen, (0, 0, 255), (player2_col * 20 + 10, player2_row * 20 + 10), 10)
Implementing Special Features
Special features add complexity and interest to your board game. These can include ladders, snakes, double moves, and more. Implementing these features requires additional logic to handle specific game conditions.
Adding Ladders and Snakes
Ladders and snakes can significantly alter a player's progress. Use functions to check if a player has landed on a ladder or snake and adjust their position accordingly.
def check_ladders(position):
ladders = {3: 22, 5: 8, 11: 26, 20: 29}
return ladders.get(position, position)
def check_snakes(position):
snakes = {27: 1, 21: 9, 17: 4, 19: 7}
return snakes.get(position, position)
Implementing Double Moves and Extra Turns
Double moves and extra turns can add strategic depth to your game. Implement these features using conditionals that check for specific conditions.
def check_double_moves(position):
double_moves = {6, 18, 30}
return position in double_moves
def check_extra_turns(position):
extra_turns = {12, 24}
return position in extra_turns
Handling Special Conditions
Other special conditions, like exact requirements or missed turns, can be implemented similarly. These features enhance the gameplay experience and add variety.
def check_exact_requirements(position, grid_size):
return position == grid_size ** 2
def check_missed_turns(position):
missed_turns = {14, 28}
return position in missed_turns
Finalizing and Testing Your Game
After implementing the core logic and special features, it's time to finalize and test your game. Testing ensures your game runs smoothly and meets all assignment requirements.
Testing for Bugs and Edge Cases
Thoroughly test your game to catch any bugs or edge cases. Play through the game multiple times and check for unexpected behavior. Make sure all features work as intended and the game logic is sound.
Commenting and Documenting Your Code
Comment your code to explain key sections and logic. This makes your code easier to understand and maintain. Include comments that describe the purpose of each function and major code blocks.
# Check if player has landed on a ladder
player1_position = check_ladders(player1_position)
# Check if player has landed on a snake
player1_position = check_snakes(player1_position)
Following Submission Guidelines
Ensure your submission meets all guidelines provided in the assignment brief. This includes naming your file correctly and including all required elements. Double-check the instructions to avoid losing points on technicalities.
By following these steps, you can systematically approach and solve any board game programming assignment. This structured method not only helps in completing the assignment but also reinforces important programming concepts.
Detailed Steps to Approach a Board Game Programming Assignment
To further assist you, let's break down the detailed steps to approach a board game programming assignment. These steps will guide you through each phase of the project, from initial setup to final submission.
Setting Up Your Development Environment
Setting up your development environment correctly is crucial for a smooth coding experience.
Installing Necessary Libraries
Ensure you have all necessary libraries installed. For Python-based projects, pygame and random are commonly used.
import pygame
import random
Setting Up Your IDE
Choose a suitable Integrated Development Environment (IDE) like PyCharm, VSCode, or Jupyter Notebook. Set up your project folder and ensure your IDE is configured to run your code effectively.
Creating a Project Structure
Organize your project files into a clear structure. This makes it easier to manage your code and assets.
Designing the Game Board
Designing the game board involves creating a visual grid and implementing the game logic.
Drawing the Grid
Use nested loops to draw the grid. This creates the visual representation of the board.
def draw_board(screen, grid_size):
for row in range(grid_size):
for col in range(grid_size):
pygame.draw.rect(screen, (255, 255, 255), (col * 20, row * 20, 20, 20), 1)
Initializing Player Positions
Set initial positions for the players. Use variables to track their positions on the board.
player1_position = 0
player2_position = 0
Implementing Player Movement
Define the logic for player movement. Use a while loop to alternate turns and move players based on dice rolls.
current_player = 1
while player1_position < grid_size ** 2 and player2_position < grid_size ** 2:
if current_player == 1:
roll = random.randint(1, 6) # Rolling a 6-sided dice
player1_position += roll
current_player = 2
else:
roll = random.randint(1, 6)
player2_position += roll
current_player = 1
Implementing Special Features
Special features enhance gameplay by adding complexity and interest.
Adding Ladders and Snakes
Implement ladders and snakes to modify player positions based on their location on the board.
def check_ladders(position):
ladders = {3: 22, 5: 8, 11: 26, 20: 29}
return ladders.get(position, position)
def check_snakes(position):
snakes = {27: 1, 21: 9, 17: 4, 19: 7}
return snakes.get(position, position)
Implementing Double Moves and Extra Turns
Add logic for double moves and extra turns to create strategic opportunities for players.
def check_double_moves(position):
double_moves = {6, 18, 30}
return position in double_moves
def check_extra_turns(position):
extra_turns = {12, 24}
return position in extra_turns
Handling Special Conditions
Implement special conditions like exact requirements and missed turns to add variety to the game.
def check_exact_requirements(position, grid_size):
return position == grid_size ** 2
def check_missed_turns(position):
missed_turns = {14, 28}
return position in missed_turns
Finalizing and Testing Your Game
Finalizing and testing your game ensures it runs smoothly and meets all requirements.
Conducting Thorough Testing
Play through the game multiple times to catch any bugs or edge cases. Verify that all features work as intended and the game logic is correct.
Commenting Your Code
Add comments to explain key sections and logic in your code. This makes your code easier to understand and maintain.
# Check if player has landed on a ladder
player1_position = check_ladders(player1_position)
# Check if player has landed on a snake
player1_position = check_snakes(player1_position)
Preparing for Submission
Ensure your submission meets all guidelines provided in the assignment brief. Double-check the instructions to avoid losing points on technicalities.
Tips for Success in Board Game Programming Assignments
Finally, here are some additional tips to help you succeed in board game programming assignments.
Stay Organized
Keep your code and project files organized. Use clear naming conventions and maintain a tidy project structure.
Test Incrementally
Test your code incrementally as you develop it. This helps catch bugs early and ensures each part of your game works correctly before moving on.
Seek Feedback
Don't hesitate to seek feedback from peers or instructors. Constructive feedback can provide valuable insights and help you improve your code.
Practice Regularly
Regular practice is key to mastering programming. Work on similar projects and challenges to strengthen your coding skills.
By following this comprehensive guide, you can approach and solve any board game programming assignment with confidence. This structured method will help you complete the assignment efficiently while reinforcing important programming concepts.