Claim Your Discount Today
Ring in Christmas and New Year with a special treat from www.programminghomeworkhelp.com! Get 15% off on all programming assignments when you use the code PHHCNY15 for expert assistance. Don’t miss this festive offer—available for a limited time. Start your New Year with academic success and savings. Act now and save!
We Accept
- 1. Understanding the Assignment Requirements
- 2. Setting Up Your Development Environment
- 3. Familiarizing Yourself with Python and Numpy
- 4. Breaking Down the Problem
- 5. Writing and Testing Code
- 6. Visualization and Verification
- 7. Preparing for Submission
- 8. Handling Common Issues
- 9. Continuous Learning and Practice
- Conclusion
Visual computing assignments in Python present both challenges and rewards. Mastering tasks such as managing data structures, implementing graphics pipelines, conducting image processing, and executing transformations requires a systematic approach. By adopting structured methods, students can effectively navigate these complexities. This guide aims to empower learners by offering strategies that enhance efficiency and comprehension. Whether you're refining basic concepts or delving into advanced techniques, understanding the underlying principles is crucial. Each step, from conceptualizing algorithms to debugging code, contributes to comprehensive skill development. Emphasis is placed on clarity in implementation and adherence to assignment specifications, ensuring robust solutions. Practical examples and clear explanations enable students to grasp intricate concepts more effectively. By fostering a problem-solving mindset and encouraging experimentation, this resource equips learners with the tools to solve their visual computing assignment using Python confidently. Through continuous practice and application, students can complete their programming assignment and enhance their Python proficiency, excelling in handling diverse visual computing assignments.
1. Understanding the Assignment Requirements
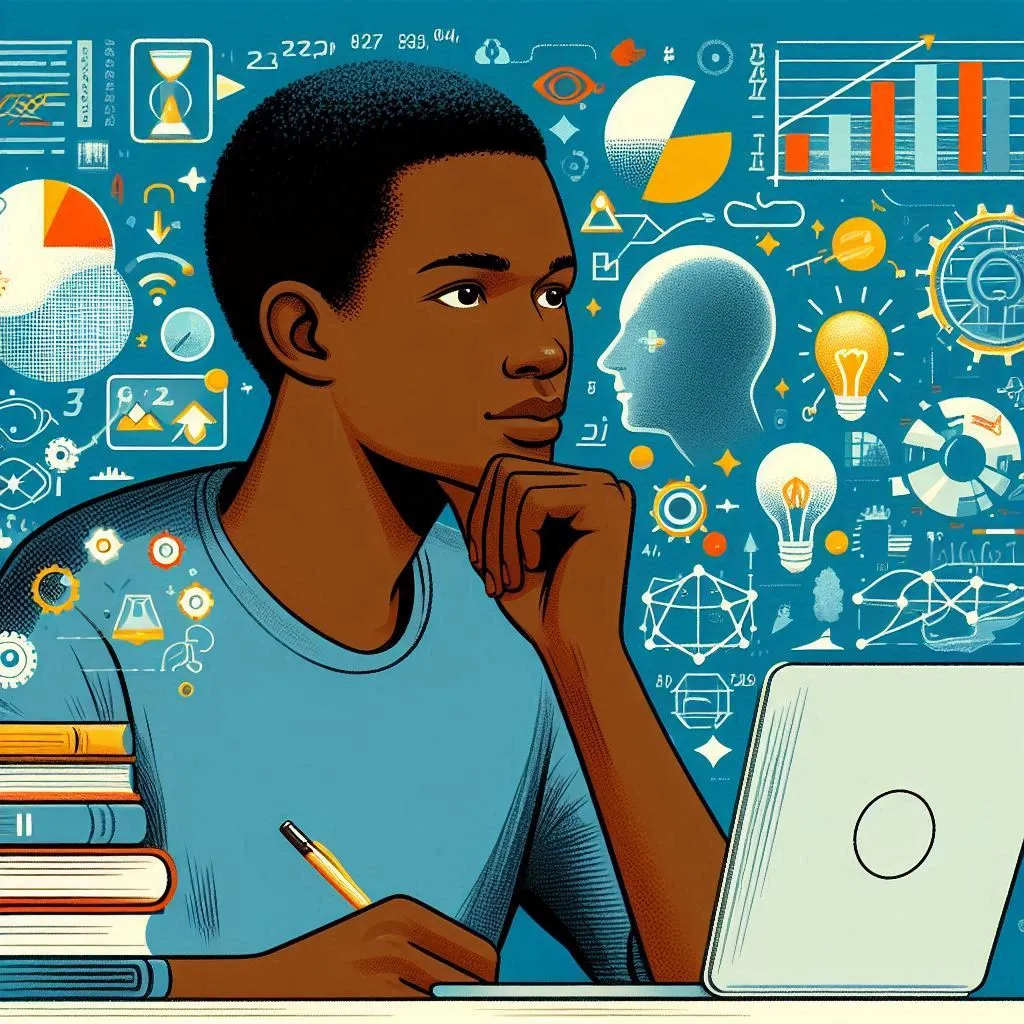
Before diving into coding, thoroughly read the assignment instructions. Pay attention to the following:
- Task Breakdown: Begin by identifying the main components and subtasks outlined in the assignment prompt. For example, a typical assignment may involve tasks related to Python basics, graphics pipelines, image processing, and transformations. Each component may require specific functions or modules to be implemented.
- Deliverables: Take note of the specific files you are required to submit, such as .py files or a complete Jupyter Notebook. Ensure that your code is organized according to the specified structure and naming conventions to facilitate evaluation.
- Evaluation Criteria: Familiarize yourself with how the assignment will be graded. Understand the grading rubric and prioritize tasks accordingly. Assignments often allocate points to different components, such as correctness of implementation, adherence to programming principles, and documentation clarity. This understanding guides you in allocating time and effort effectively to meet the assignment's requirements and maximize your score.
2. Setting Up Your Development Environment
A proper setup is crucial for smooth coding:
- Virtual Environment: Start by setting up a virtual environment to manage dependencies and ensure project isolation. You can create a virtual environment using tools like conda or Python's built-in venv module:
conda env create -f environment.yml
# or
python3 -m venv myenv
source myenv/bin/activate
pip install -r requirements.txt
This approach ensures that your project dependencies are isolated and easily reproducible across different environments.
- Code Editor: Choose a robust code editor such as Visual Studio Code (VSCode) tailored for Python development. VSCode offers powerful features including debugging capabilities, syntax highlighting, and extensions for enhanced productivity. Configuring VSCode with Python extensions provides additional functionalities like linting, code formatting, and integrated terminal access, optimizing your workflow and facilitating efficient code development and debugging.
3. Familiarizing Yourself with Python and Numpy
For many visual computing tasks, a solid understanding of Python and the Numpy library is essential:
- Python Basics: Start by refreshing your knowledge of fundamental Python concepts such as data structures (e.g., lists, dictionaries, sets) and control flow statements (e.g., loops, conditionals). These foundational skills are crucial for effective problem-solving and algorithm implementation in Python.
- Numpy: Delve into Numpy, a powerful library for numerical computing in Python. Familiarize yourself with its capabilities for performing vector and matrix operations efficiently. Numpy provides optimized functions for mathematical computations, making it indispensable for tasks ranging from basic arithmetic operations to advanced computations required in visual computing tasks like image processing and transformations. Mastery of Numpy allows you to leverage its extensive functionalities to manipulate and process large datasets with ease, enhancing the efficiency and performance of your Python programs.
4. Breaking Down the Problem
Divide the assignment into smaller, manageable parts:
- Data Structures: Begin by defining essential data structures using Numpy arrays. For example, create vectors and matrices based on input parameters such as a student ID. This step ensures that your data representation aligns with the assignment requirements and facilitates efficient computation.
- Algorithms: Implement the necessary algorithms according to assignment guidelines. If required, avoid using built-in functions and instead develop custom solutions. For instance, create functions for operations like dot product or cross product, demonstrating proficiency in algorithmic thinking and problem-solving.
- Graphics and Transformations: Gain a thorough understanding of graphics pipelines and transformation theories relevant to the assignment. Apply this theoretical knowledge to practical implementation in your code, ensuring that graphical transformations and manipulations are accurately reflected. This approach bridges theory with application, enhancing comprehension and enabling effective execution of visual computing tasks in Python.
5. Writing and Testing Code
Implement your solution incrementally and test each part thoroughly:
- Function Implementation: Begin by writing functions for each task outlined in the assignment, such as define_structures(), compute_lengths(), and compute_triangle_area(). Ensure that each function adheres to the specified requirements and effectively performs its intended operation.
- Unit Testing: Create comprehensive unit tests to validate the correctness of each function. Develop test cases that cover typical scenarios and edge cases, verifying outputs against expected results. Utilize Numpy functions for numerical validation, ensuring accuracy in computations and handling of data structures.
- Debugging: Employ debugging tools available in your code editor, such as breakpoints and variable inspection. Step through your code systematically to identify and resolve any errors or unexpected behaviors. Debugging aids in refining your implementation, enhancing code efficiency, and ensuring that your solution meets assignment specifications without runtime issues or logical errors.
6. Visualization and Verification
For assignments involving graphics or image processing:
- Plotting: Utilize libraries like Matplotlib to visualize data structures effectively. Plotting can be instrumental in illustrating concepts such as triangles or matrices, providing visual confirmation of your computational results and aiding in understanding complex data relationships.
- Image Processing: Experiment with different filters and transformations on images as specified by the assignment requirements. Implement these operations using Python libraries tailored for image processing, ensuring that each transformation yields the expected results. Testing various filters and transformations helps validate the robustness and accuracy of your implementations, ensuring they meet the desired objectives effectively.
7. Preparing for Submission
Before submitting your work:
- Code Review: Conduct a thorough review of your codebase to ensure readability and adherence to coding standards. Verify that variables are appropriately named, functions are well-documented with clear comments, and the overall structure follows best practices. A clean, organized codebase facilitates easier debugging and enhances maintainability.
- Functionality Check: Confirm that all required functions have been implemented according to the assignment specifications. Test each function to ensure it performs its intended task accurately and efficiently. Validate outputs against expected results to identify and address any discrepancies or logic errors.
- Final Testing: Execute comprehensive tests in the designated environment to verify the functionality and stability of your code. Ensure that your solution runs smoothly without encountering runtime errors or unexpected behaviors. Final testing guarantees that your submission meets all project requirements and is ready for evaluation.
8. Handling Common Issues
When encountering common challenges:
- Dependency Problems: Address issues related to library installations by consulting official documentation or seeking assistance from relevant forums and community support. Follow recommended procedures for installing and managing dependencies, ensuring compatibility and functionality within your development environment.
- Debugging Difficulties: Navigate debugging challenges using available online resources such as tutorials and documentation. Gain insights into error resolution techniques and leverage debugging tools provided by your integrated development environment (IDE) to pinpoint and rectify code errors effectively. Continuous learning and proactive problem-solving are key to overcoming debugging obstacles and refining your coding proficiency.
9. Continuous Learning and Practice
To continually improve:
- Online Resources: Make use of abundant online tutorials, comprehensive documentation, and active forums to expand your knowledge base. These resources offer valuable insights, troubleshooting tips, and advanced techniques that can enhance your understanding of Python and visual computing concepts.
- Practice: Regularly engage in hands-on practice by tackling similar assignments and projects. Applying theoretical knowledge in practical scenarios strengthens your problem-solving abilities and builds confidence in handling diverse visual computing tasks. Consistent practice not only reinforces fundamental concepts but also cultivates proficiency in implementing complex algorithms and techniques effectively.
Conclusion
By following these steps, you can systematically approach and solve visual computing problems using Python, ensuring a thorough understanding and effective implementation of your assignment. Begin by familiarizing yourself with basic Python concepts and gradually advance to more complex topics such as graphics pipelines, image processing, and transformations. Emphasize a structured approach to problem-solving, breaking down tasks into manageable steps and leveraging Python libraries like NumPy for efficient computation. Debugging and testing are integral to refining your solutions, ensuring they meet assignment requirements. Lastly, maintain documentation and seek clarification on unclear concepts to strengthen your understanding. With perseverance and practice, mastering visual computing in Python will not only enhance your programming skills but also prepare you for tackling future challenges in the field. Happy coding!