Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 10% off on your all programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
- Understanding the Problem
- Step-by-Step Guide to Solving the Problem
- 1. Flatten the Image:
- 2. Unflatten the Image:
- 3. Check Adjacency:
- 4. Recursive Pixel Flipping:
- 5. Generate All Possible Images:
- 6. Writing Images to a File:
- Conclusion
Programming assignments can often seem daunting, especially when they involve complex concepts like recursion. However, breaking down the problem into manageable steps and understanding the core principles can make tackling python assignments much more approachable. In this blog post, we will guide you through the process of solving a type of programming problem that involves recursively flipping pixels in an image. The objective is to generate all possible unique images from an input image by flipping pixels from 0 to 1, under certain constraints: a pixel can only be flipped if there is an adjacent pixel with the value of 1, and the number of pixels that can be flipped is limited by a budget. We will explore this process step-by-step, including flattening the image, checking adjacency, using recursion for pixel flipping, generating all possible images, and writing these images to a file. While the specific details of your assignment may vary, the general approach outlined here can be applied to similar problems, providing you with a structured method to tackle complex programming challenges. If you need assistance with programming assignment, this guide will help you navigate through the steps and understand the process better.
Understanding the Problem
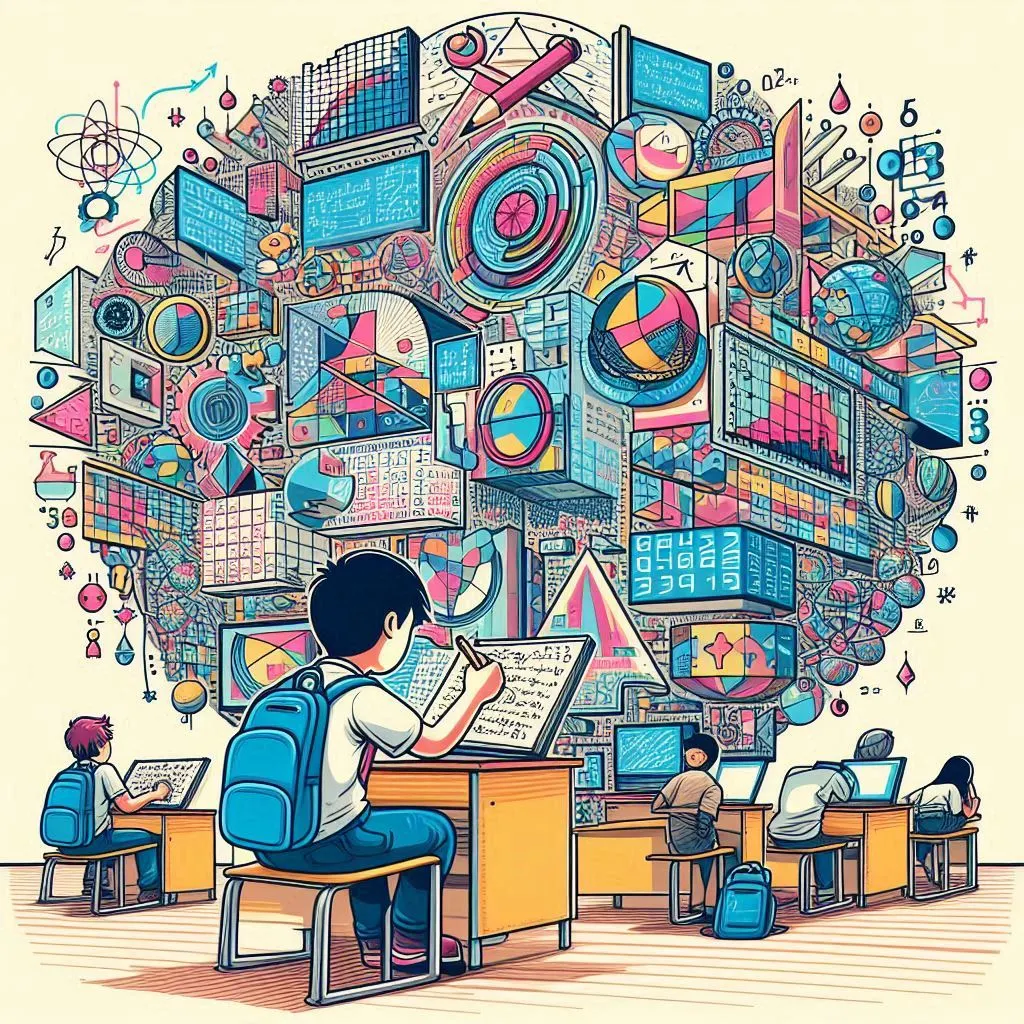
Before diving into code, it's essential to fully understand the problem statement. This involves thoroughly reading and analyzing the requirements, constraints, and objectives outlined in the assignment. Understanding the problem ensures you know exactly what is expected and helps in identifying the key elements that need to be addressed. It's important to consider all the conditions and constraints specified, such as adjacency rules and budget limitations for pixel flips in our case. Grasping the core problem allows you to conceptualize the overall approach and plan the necessary steps to implement an effective solution. This preliminary step is crucial as it lays the foundation for the entire coding process, making the subsequent steps of breaking down the task into manageable functions and using recursion more straightforward. Taking the time to fully comprehend the problem statement will ultimately save time and reduce errors as you work through the assignment, ensuring a more efficient and accurate solution. Here's a concise summary of the typical task:
- Objective: Generate all possible unique images from an input image by flipping pixels from 0 to 1. This task revolves around transforming an initial image by selectively altering pixels under specified conditions. The goal is to explore every potential configuration where pixels initially set to 0 can be flipped to 1, ensuring each resultant image maintains its uniqueness. This process is vital in computational imaging tasks where generating diverse variations of an image can aid in pattern recognition, data augmentation for machine learning, or exploring different visual outcomes in digital art.
- Constraints: A pixel can only be flipped from 0 to 1 if there is an adjacent pixel with the value of 1. The number of pixels that can be flipped is limited by a budget. These constraints impose logical boundaries on the transformation process. They ensure that any change to the image adheres to spatial continuity rules (adjacency constraint) and respects computational limits (budget constraint). By enforcing these rules, the integrity of the original image structure is preserved while allowing controlled modifications that expand the image's potential representations.
Let's consider a simple example to illustrate this:
```python
image = [
[1, 0, 0],
[1, 0, 0],
[1, 1, 1]
]
```
In this example, the objective would be to generate all possible configurations where pixels initially set to 0 can be flipped to 1, adhering to the adjacency and budget constraints. This practical example demonstrates how these constraints influence the transformation process and shape the outcomes of the pixel flipping algorithm.
Step-by-Step Guide to Solving the Problem
To effectively tackle a programming assignment involving recursive pixel flipping, it's crucial to approach it in a structured, step-by-step manner. This methodical approach starts with understanding the problem thoroughly, ensuring you are clear on the constraints and requirements. Next, break down the task into smaller, manageable functions. Begin by flattening the image for simpler manipulation, then implement a function to check for adjacent pixels with a value of 1, which is necessary for valid pixel flips. With these foundational functions in place, you can then use recursion to explore all possible valid flips within the given budget constraints. Each recursive call should process one pixel at a time, adhering to the adjacency and budget rules. Once you have all possible variations, convert the flattened images back to their original 2D form for easier verification and output. This systematic approach not only helps in solving the specific assignment at hand but also enhances your overall problem-solving skills in programming.
1. Flatten the Image:
Working with a 2D list can be complex due to the nested structure of rows and columns. Flattening the image into a 1D list simplifies indexing and manipulation by reducing the dimensional complexity. This transformation makes it easier to iterate through the pixels linearly and perform operations such as checking adjacency or applying recursive algorithms for pixel flipping. Moreover, a flattened representation is often more compatible with certain data processing tasks and algorithms that expect a linear sequence of data rather than a matrix-like structure.
def flatten_image(image):
return [pixel for row in image for pixel in row]
The flatten_image function iterates through each row of the 2D image matrix, appending each pixel sequentially to a 1D list. This process ensures that every pixel's value is included in a single linear list, preserving the order and arrangement of pixels from the original image.
2. Unflatten the Image:
Converting a 1D list back into a 2D list is essential for output and verification purposes, especially after performing operations on the flattened representation. The unflatten_image function reconstructs the original 2D structure from a flattened list, restoring the rows and columns of the image matrix. This transformation is crucial for visualizing and verifying the results of operations performed on the flattened image. It ensures that the spatial arrangement of pixels is accurately represented, which is particularly important in tasks such as image processing, where maintaining the original layout of pixels is necessary for correct interpretation and analysis.
def unflatten_image(flat_image):
size = int(len(flat_image) ** 0.5)
return [flat_image[i*size:(i+1)*size] for i in range(size)]
In the unflatten_image function, the size of the original image is inferred from the length of the flattened list. By dividing the flattened list into segments of equal length corresponding to the original image rows, each segment is reshaped into rows of pixels, reconstructing the 2D matrix. This process ensures that the integrity of the original image dimensions and pixel values is maintained throughout the transformation.
3. Check Adjacency:
The check_adjacent_for_one function is crucial in ensuring that a pixel can only be flipped if there is at least one adjacent pixel with a value of 1. This function calculates the row and column of a given pixel index within a flattened image representation. It then identifies adjacent indices based on the image size, checking in all four cardinal directions (above, below, left, right). By verifying adjacent pixels, this function enforces spatial continuity rules, which are essential for maintaining logical coherence in the transformed images. This validation step ensures that each pixel flip adheres to the specified adjacency constraint, thereby preserving the structural integrity of the original image during transformation.
def check_adjacent_for_one(flat_image, index):
size = int(len(flat_image) ** 0.5)
row, col = divmod(index, size)
adjacent_indices = []
if row > 0: adjacent_indices.append(index - size)
if row < size - 1: adjacent_indices.append(index + size)
if col > 0: adjacent_indices.append(index - 1)
if col < size - 1: adjacent_indices.append(index + 1)
return any(flat_image[i] == 1 for i in adjacent_indices)
4. Recursive Pixel Flipping:
The pixel_flip function employs recursion to systematically explore and generate all possible configurations of an image by flipping pixels from 0 to 1 within a specified budget. This recursive approach iterates through each pixel in the image, attempting to flip it if conditions permit (i.e., if the pixel is 0 and adjacent to a 1). For each recursive call, the function evaluates the possibility of flipping the current pixel and recursively explores subsequent pixels until either the budget is exhausted or all pixels have been considered. The results are accumulated in the results list, capturing all valid configurations that meet the flipping criteria. This methodical exploration using recursion ensures that every potential transformation of the image is considered, adhering to both the adjacency and budget constraints defined earlier.
def pixel_flip(current_image, original_image, flip_budget, results, pixel_index=0):
if flip_budget == 0 or pixel_index >= len(current_image):
results.append(current_image[:])
return
if current_image[pixel_index] == 0 and check_adjacent_for_one(original_image, pixel_index):
current_image[pixel_index] = 1
pixel_flip(current_image, original_image, flip_budget - 1, results, pixel_index + 1)
current_image[pixel_index] = 0
pixel_flip(current_image, original_image, flip_budget, results, pixel_index + 1)
These functions collectively form a robust framework for manipulating and transforming image data through recursive exploration and logical constraints, providing a structured approach to solving complex programming assignments involving pixel manipulation and transformation.
5. Generate All Possible Images:
The generate_new_images function initiates the process by first flattening the input image into a 1D list using the flatten_image function. This flattened representation simplifies the recursive pixel flipping process by providing a linear sequence of pixels to manipulate. The function then initializes an empty list results to store all possible configurations of the image that adhere to the specified budget and adjacency constraints. By calling the pixel_flip function with the flattened image and budget parameters, the function systematically explores and generates every valid image variation. Finally, the function converts each flattened result back into a 2D format using unflatten_image, ensuring that each generated image is presented in its original matrix structure.
def generate_new_images(image, budget):
flat_image = flatten_image(image)
results = []
pixel_flip(flat_image, flat_image, budget, results)
return [unflatten_image(result) for result in results]
6. Writing Images to a File:
The write_image function facilitates the practical output of generated images to files, ensuring that the transformations are saved and marked accordingly for verification and analysis. This function takes three parameters: orig_image, new_image, and file_name. It opens a file specified by file_name in write mode and iterates through corresponding rows of orig_image and new_image. For each row pair, it compares corresponding pixels: if a pixel in new_image differs from orig_image, it marks it with 'X'; otherwise, it writes the original pixel value. This marking mechanism visually indicates where pixels have been flipped during the transformation process, providing a clear representation of the changes made to the image data.
def write_image(orig_image, new_image, file_name):
with open(file_name, 'w') as file:
for orig_row, new_row in zip(orig_image, new_image):
file.write(''.join('X' if o != n else str(o) for o, n in zip(orig_row, new_row)) + '\n')
These functions together provide a comprehensive solution for generating and persisting multiple variations of an image based on specified transformation rules. They enable both theoretical exploration through recursive manipulation and practical application by storing results in a format that is easily accessible and verifiable. This structured approach supports the implementation of complex programming assignments involving image processing and manipulation, ensuring accuracy and efficiency in both exploration and output phases.
Conclusion
By following this structured approach, you can efficiently solve recursive pixel flipping problems or similar assignments. The key steps involve understanding the problem constraints, breaking down the task into simpler functions, and using recursion to explore all valid possibilities. This methodical approach not only helps in completing your python assignments but also strengthens your problem-solving skills in programming. Remember, while the specific details of your assignment may vary, the principles of flattening data, checking conditions, and using recursion are widely applicable. By mastering these techniques, you'll be better equipped to tackle a variety of complex programming challenges. Good luck with your programming assignments!