Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 10% off on your all programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
- Understanding the Assignment
- Step 1: Reading the Log File
- Step 2: Using Regular Expressions to Extract Data
- Explanation of the Regex Pattern
- Why Regular Expressions are Useful
- Step 3: Organizing Data with Dictionaries
- Step 4: Analyzing the Data
- Step 5: Structuring the Code with Functions
- Step 6: Conclusion
When working on programming assignments, it's crucial to have the right approach and a solid understanding of how to effectively use tools such as regular expressions, dictionaries, and file handling techniques. These tools are not only essential for extracting and manipulating data but also for organizing and analyzing information in a structured and efficient manner. Developing these skills will allow you to tackle complex data-driven tasks, process large datasets, and perform operations such as searching, filtering, and sorting efficiently. In this blog, we will explore key strategies and best practices that can help you not only successfully solve assignments similar to the one described but also enhance your overall problem-solving and programming abilities.
By breaking down the problem into manageable steps, leveraging data structures, and writing modular, reusable code, you can approach any assignment with confidence. This guide aims to walk you through common methodologies and patterns for tackling such projects, offering insights into how to think critically and abstractly when solving similar challenges. Whether you're handling log files, performing data analysis, or managing real-time processing tasks, these techniques will help you identify patterns, automate repetitive tasks, and optimize your code for performance. With the help of Python homework helper strategies, mastering these concepts will enable you to take on more complex programming tasks with greater ease and adaptability, broadening your skillset and deepening your understanding of efficient coding practices across various applications and industries.
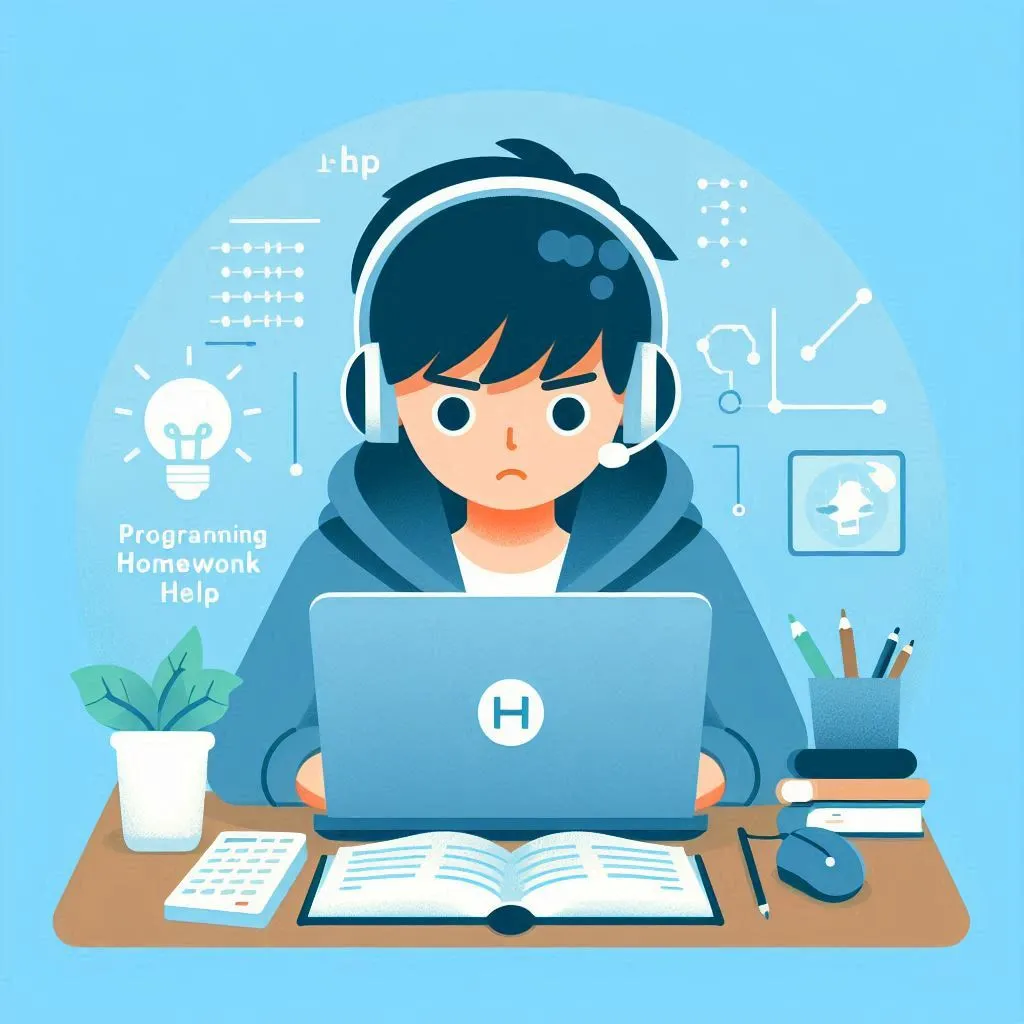
Understanding the Assignment
Assignments like this often involve analyzing a large dataset, such as a log file, and extracting meaningful information from it. In this case, the data comes from an access log file for an Apache server, which records various details of server requests. These types of projects are common in real-world scenarios, particularly in fields like data analysis, system administration, and cybersecurity. The primary tasks here include providing help with programming homework to develop skills in parsing, data extraction, and analysis. By mastering these techniques, you’ll be better equipped to handle similar assignments and real-world data challenges effectively.
Extracting data using regular expressions to parse specific parts of the log, such as IP addresses, resource URLs, and timestamps.
- Storing and managing data efficiently using dictionaries, lists, or tuples, which allow you to organize the extracted data and facilitate analysis.
- Finding patterns and relationships within the data, such as identifying the most frequently accessed resource or determining which requester made the most requests.
Completing assignments like this requires a combination of technical skills and analytical thinking. The key skills you’ll need to succeed include:
- Understanding file operations: Being able to read and write files is fundamental, especially when handling large datasets like log files. You should be familiar with opening, reading, and parsing files line by line to extract relevant information.
- Knowledge of regular expressions: Regular expressions (regex) are powerful tools that allow you to search, extract, and manipulate specific patterns in text, making them essential for parsing complex log data and isolating meaningful information, such as IP addresses or resource paths.
- Effective use of dictionaries: Dictionaries are crucial for storing and analyzing data in key-value pairs. For instance, you can use a dictionary to map IP addresses to the number of requests made or resources to the users accessing them. This allows for efficient lookups and data manipulation, helping you answer questions like, "What is the most accessed resource?" or "Which user made the most requests?"
By mastering these skills, you’ll be able to not only complete this specific assignment but also confidently tackle similar tasks in the future. These foundational techniques can be applied across various programming challenges, from analyzing server logs to performing data extraction in different formats, making them highly valuable in a wide range of programming and data analysis scenarios.
Step 1: Reading the Log File
Most log files, especially server access logs, are plain text files that record data in a structured format. The first step in processing these logs is to read the file line by line to extract relevant information. In Python, you can use the open() function to access the file and then iterate through its contents. For this example, we'll be working with an Apache server access log file, which records details about server requests, such as the time of the request, the IP address making the request, and the resource accessed.
Here's a simple example of how you can start by reading the file and storing the data:
with open('access_log', 'r') as log_file:
log_data = log_file.readlines()
In this code snippet, the open() function opens the file in read mode ('r'), and the readlines() method reads each line of the file and stores it in a list called log_data. Each line in the log file is now represented as a string in the list, which gives you a foundation to start analyzing the log information.
At this stage, the log data is unstructured and consists of raw strings. You’ll need to parse and extract specific information from these lines, such as the IP addresses, resource URLs, and HTTP status codes, which can be done using regular expressions. By reading the log file line by line, you're ensuring that even if the file is very large, your program can handle it efficiently without loading the entire file into memory all at once.
This step is crucial because it forms the base for processing and analyzing the log file. Once you have the log data in a manageable format, you can proceed with more complex operations, such as searching for patterns, identifying the most frequent requesters, and calculating access statistics for different resources. This approach also allows for flexibility, as you can easily add filters or conditions to handle specific parts of the log data that are relevant to your analysis.
Step 2: Using Regular Expressions to Extract Data
A log file, such as an Apache access log, follows a structured format, making it possible to extract specific information using regular expressions (regex). Regex is a powerful tool for pattern matching, and it allows you to extract data like IP addresses, requested resources, HTTP methods, and status codes from each log entry. In this step, we'll use regex to pull out key details from each line of the log file.
For example, an Apache access log entry typically looks something like this:
123.123.123.123 - - [12/Mar/2023:15:00:45 +0000] "GET /index.html HTTP/1.1" 200 1234
This line contains various pieces of information, including the IP address of the requester, the date and time of the request, the HTTP method (e.g., GET), the resource being accessed (e.g., /index.html), the HTTP protocol version, the status code (e.g., 200), and the size of the response in bytes. Regular expressions allow us to efficiently extract only the parts we care about.
Here’s a Python example of using regular expressions to extract the IP address and the resource requested:
import re
# Sample log entry
log_entry = '123.123.123.123 - - [12/Mar/2023:15:00:45 +0000] "GET /index.html HTTP/1.1" 200 1234'
# Regular expression pattern to extract the IP address and the resource
pattern = r'(\d{1,3}\.\d{1,3}\.\d{1,3}\.\d{1,3}) .* "GET (.*) HTTP'
# Search the log entry for the pattern
match = re.search(pattern, log_entry)
# If a match is found, extract the IP address and resource requested
if match:
ip_address = match.group(1) # First capture group is the IP address
resource_requested = match.group(2) # Second capture group is the resource requested
print(f"IP: {ip_address}, Resource: {resource_requested}")
Explanation of the Regex Pattern
- \d{1,3}\.\d{1,3}\.\d{1,3}\.\d{1,3}: This pattern matches an IP address. It looks for four sets of 1 to 3 digits separated by periods (e.g., 123.123.123.123).
- .*: This matches any characters between the IP address and the HTTP method (e.g., GET, POST).
- "GET (.*) HTTP: This captures the resource being accessed, such as /index.html. The parentheses around .* create a capture group, allowing us to extract the resource string.
Why Regular Expressions are Useful
- Precision: Regular expressions allow you to target specific patterns in each line, so you can efficiently pull out just the information you need without processing unnecessary data.
- Flexibility: You can easily modify the regex to capture other data, such as HTTP methods, status codes, or request timestamps, depending on the information required.
- Scalability: Regex can handle large datasets efficiently, making it ideal for parsing large log files that may contain thousands or even millions of entries.
By using regular expressions, you're able to extract meaningful data from raw log files in a structured way, which is a crucial step in solving assignments that involve log analysis or similar tasks. This approach ensures that you can automate the process of data extraction, allowing you to focus on more complex aspects of the assignment, such as analyzing patterns and drawing conclusions.
Step 3: Organizing Data with Dictionaries
Once you've extracted key information (like the IP address and resource), you can store it in dictionaries for easy access and counting.
You can use dictionaries to map requesters (IP addresses) to the resources they accessed and vice versa.
<code ignore--minify class="code-view">requesters = {} # Dictionary to map IP addresses to resources
resources = {} # Dictionary to map resources to IP addresses
for line in log_data:
match = re.search(pattern, line)
if match:
ip_address = match.group(1)
resource_requested = match.group(2)
# Count the number of requests per resource
if resource_requested not in resources:
resources[resource_requested] = []
resources[resource_requested].append(ip_address)
# Count the number of requests per requester
if ip_address not in requesters:
requesters[ip_address] = []
requesters[ip_address].append(resource_requested)
</code>
Here’s what the dictionaries represent:
- resources: Keys are the resources (e.g., /index.html), and the values are lists of IP addresses that accessed the resource.
- requesters: Keys are the IP addresses, and the values are lists of resources they requested.
Step 4: Analyzing the Data
Now that the data is organized, you can analyze it to answer the assignment questions. For example, to find the most requested resource and the top requester:
Finding the Most Accessed Resource:
most_accessed_resource = max(resources, key=lambda r: len(resources[r]))
times_accessed = len(resources[most_accessed_resource])
top_requester_for_resource = max(resources[most_accessed_resource], key=resources[most_accessed_resource].count)
print(f"Most accessed resource: {most_accessed_resource}")
print(f"Times accessed: {times_accessed}")
print(f"Top requester for this resource: {top_requester_for_resource}")
Finding the Most Frequent Requester:
most_frequent_requester = max(requesters, key=lambda r: len(requesters[r]))
total_requests = len(requesters[most_frequent_requester])
most_requested_resource = max(requesters[most_frequent_requester], key=requesters[most_frequent_requester].count)
print(f"Most frequent requester: {most_frequent_requester}")
print(f"Total requests: {total_requests}")
print(f"Most requested resource by this requester: {most_requested_resource}")
Step 5: Structuring the Code with Functions
To maintain clarity, organize your code into functions. For example:
def parse_log(log_line):
pattern = r'(\d{1,3}\.\d{1,3}\.\d{1,3}\.\d{1,3}) .* "GET (.*) HTTP'
return re.search(pattern, log_line)
def update_dictionaries(ip, resource, requesters, resources):
if resource not in resources:
resources[resource] = []
resources[resource].append(ip)
if ip not in requesters:
requesters[ip] = []
requesters[ip].append(resource)
def analyze_logs(log_data):
requesters = {}
resources = {}
for line in log_data:
match = parse_log(line)
if match:
ip_address, resource = match.groups()
update_dictionaries(ip_address, resource, requesters, resources)
return requesters, resources
Step 6: Conclusion
Working through assignments like this can significantly boost your problem-solving and programming skills, especially when handling real-world data like server logs. These tasks require you to break down large, unstructured datasets and extract meaningful insights using a combination of Python’s powerful libraries and tools like regular expressions, dictionaries, and efficient file handling techniques.
By following a systematic approach, as outlined in the previous steps, you can tackle similar programming assignments with confidence. First, reading and understanding the data helps you get a clear view of what needs to be processed. Using regular expressions allows you to extract specific details from the data, while dictionaries and other data structures help organize and analyze that information. By carefully designing your code with functions, you ensure modularity and ease of debugging.
In the case of this particular type of assignment, learning to work with server logs not only strengthens your Python skills but also familiarizes you with tasks often encountered in fields like web development, cybersecurity, and IT. These logs often record crucial information about user behavior, system performance, and potential security threats, making log analysis a critical skill for many tech professionals.
Moreover, these assignments help you learn how to automate tasks—such as identifying the most frequent requesters or most accessed resources—that would be nearly impossible to do manually, especially when dealing with large datasets. Through practice, you’ll become adept at writing algorithms to efficiently sift through large amounts of data, a skill that's invaluable in today’s data-driven world.
In conclusion, assignments involving data extraction, pattern recognition, and analysis are an excellent way to sharpen your programming skills. By using techniques such as file operations, regular expressions, and dictionaries, you can efficiently solve complex problems. As you practice, you'll become more proficient at breaking down assignments into manageable steps, ensuring success in similar future projects. Keep experimenting with different methods, and soon you'll find yourself mastering the art of data handling and analysis, which will benefit you in a wide range of programming and real-world applications.