Claim Your Discount Today
Ring in Christmas and New Year with a special treat from www.programminghomeworkhelp.com! Get 15% off on all programming assignments when you use the code PHHCNY15 for expert assistance. Don’t miss this festive offer—available for a limited time. Start your New Year with academic success and savings. Act now and save!
We Accept
- Understanding the Shunting-Yard Algorithm
- Infix and Postfix Notation
- Key Concepts
- 1. Operator Precedence:
- 2. Associativity:
- 3. Parentheses:
- Implementing the Shunting-Yard Algorithm
- 1. Tokenizing the Input
- 2. Implementing the Shunting-Yard Algorithm
- Algorithm Steps:
- 3. Evaluating the RPN Expression
- 4. Putting It All Together
- Practical Tips and Considerations
- 1. Handling Edge Cases:
- 2. Error Handling:
- 3. Testing:
- 4. Performance:
- Conclusion
Parsing and evaluating mathematical expressions efficiently is a fundamental skill in computer science. Whether you're working on a computer science assignment or developing software like calculators or compilers, understanding how to handle expressions is crucial. The shunting-yard algorithm, developed by Edsger Dijkstra, is a powerful tool for converting infix expressions (the standard notation where operators are placed between operands) into postfix notation (Reverse Polish Notation or RPN), which simplifies the evaluation process.
In this guide, we’ll dive into the shunting-yard algorithm, breaking down its concepts and steps to help you solve C++ programming assignments effectively. We will start by exploring infix and postfix notations, move on to the details of the shunting-yard algorithm, and provide a thorough implementation guide.
Understanding the Shunting-Yard Algorithm
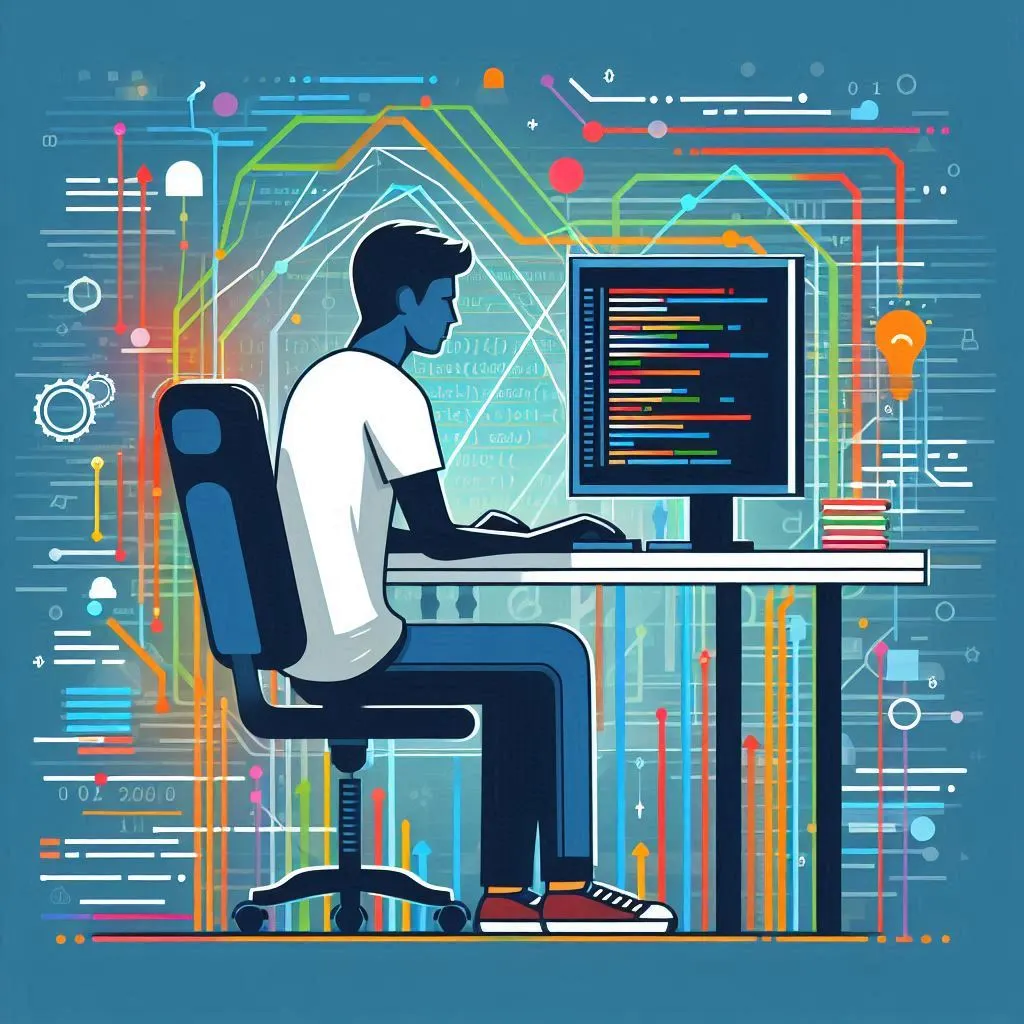
The shunting-yard algorithm, devised by Edsger Dijkstra, is a method for parsing mathematical expressions specified in infix notation and converting them to postfix notation (Reverse Polish Notation). This algorithm is particularly useful because it simplifies the evaluation of expressions by removing the need for parentheses and operator precedence rules during the computation phase.
Infix and Postfix Notation
Before diving into the algorithm, let's clarify the two types of notations involved:
1. Infix Notation:
In infix notation, operators are placed between operands. For example:
- A + B
- C * (D + E)
2. Postfix Notation (Reverse Polish Notation, RPN):
In postfix notation, operators are placed after their operands. This notation does not require parentheses to denote precedence, which simplifies computation:
- A B +
- C D E + *
Example Conversion:
Let's convert the infix expression 1 + 5 * 8 into postfix notation.
- Infix Notation: 1 + 5 * 8
- Postfix Notation: 1 5 8 * +
In the postfix notation, you perform the multiplication operation first and then the addition, which reflects the correct order of operations without needing parentheses.
Key Concepts
To implement the shunting-yard algorithm effectively, you need to understand several key concepts:
1. Operator Precedence:
Operators have different levels of precedence which determine the order in which operations are performed. Higher precedence operators should be evaluated before lower precedence ones. For instance, in the expression 3 + 4 * 5, the multiplication (*) has higher precedence than addition (+).
2. Associativity:
Operators also have associativity, which determines the order of operations when two operators of the same precedence appear. Most operators, including +, -, *, and /, are left-associative, meaning they are evaluated from left to right. For example, a - b - c is evaluated as (a - b) - c.
3. Parentheses:
Parentheses are used in infix notation to alter the natural precedence of operators. They indicate which operations should be performed first.
Implementing the Shunting-Yard Algorithm
Now that we understand the concepts, let's dive into implementing the shunting-yard algorithm. The algorithm processes tokens from the input expression and uses a stack to manage operators and parentheses. The goal is to output a list of tokens in postfix notation that can then be easily evaluated.
1. Tokenizing the Input
The first step is to tokenize the input expression. Tokenization involves splitting the input string into meaningful components: numbers, operators, and parentheses.
Example Code:
#include <sstream>
#include <string>
#include <vector>
std::vector<std::string> tokenize(const std::string &expression) {
std::vector<std::string> tokens;
std::istringstream stream(expression);
std::string token;
while (stream >> token) {
tokens.push_back(token);
}
return tokens;
}
This function reads the input expression line by line and splits it into tokens using whitespace as a delimiter.
2. Implementing the Shunting-Yard Algorithm
With the tokens ready, the next step is to apply the shunting-yard algorithm. The algorithm uses two data structures:
- Operator Stack: To temporarily hold operators and parentheses.
- Output Queue (or Vector): To hold the final output in postfix notation.
Algorithm Steps:
- Operands: Directly add operands (numbers) to the output queue.
- Operators: Pop operators from the operator stack to the output queue based on precedence and associativity, then push the current operator onto the stack.
- Parentheses: Handle opening parentheses by pushing them onto the operator stack. For closing parentheses, pop from the stack to the output queue until an opening parenthesis is encountered.
Example Code:
#include <stack>
#include <map>
#include <stdexcept>
std::vector<std::string> infixToRPN(const std::vector<std::string> &tokens) {
std::stack<std::string> operators;
std::vector<std::string> output;
std::map<std::string, int> precedence = { {"+", 1}, {"-", 1}, {"*", 2}, {"/", 2}, {"%", 2} };
for (const std::string &token : tokens) {
if (std::isdigit(token[0]) || token[0] == '-' && token.length() > 1) {
output.push_back(token);
} else if (token == "(") {
operators.push(token);
} else if (token == ")") {
while (!operators.empty() && operators.top() != "(") {
output.push_back(operators.top());
operators.pop();
}
if (operators.empty()) throw std::runtime_error("Mismatched parentheses");
operators.pop();
} else {
while (!operators.empty() && precedence[operators.top()] >= precedence[token]) {
output.push_back(operators.top());
operators.pop();
}
operators.push(token);
}
}
while (!operators.empty()) {
output.push_back(operators.top());
operators.pop();
}
return output;
}
3. Evaluating the RPN Expression
Once you have the RPN expression, the next step is to evaluate it. This involves using a stack to process operands and operators. When an operator is encountered, pop the required number of operands, apply the operator, and push the result back onto the stack.
Example Code:
#include <iostream>
#include <sstream>
#include <stack>
#include <stdexcept>
double evaluateRPN(const std::vector<std::string> &rpn) {
std::stack<double> stack;
for (const std::string &token : rpn) {
if (std::isdigit(token[0]) || token[0] == '-' && token.length() > 1) {
stack.push(std::stod(token));
} else {
double b = stack.top(); stack.pop();
double a = stack.top(); stack.pop();
if (token == "+") stack.push(a + b);
else if (token == "-") stack.push(a - b);
else if (token == "*") stack.push(a * b);
else if (token == "/") stack.push(a / b);
else if (token == "%") stack.push(std::fmod(a, b));
else throw std::runtime_error("Unknown operator");
}
}
return stack.top();
}
4. Putting It All Together
Finally, integrate the tokenization, conversion, and evaluation functions into a complete program that reads expressions, processes them, and outputs results.
Example Code:
int main() {
std::string line;
while (std::getline(std::cin, line) && !line.empty()) {
std::vector<std::string> tokens = tokenize(line);
std::vector<std::string> rpn = infixToRPN(tokens);
double result = evaluateRPN(rpn);
for (const std::string &token : rpn) {
std::cout << token << " ";
}
std::cout << "= " << result << std::endl;
}
return 0;
}
Practical Tips and Considerations
1. Handling Edge Cases:
Ensure your implementation can handle various edge cases, such as:
- Single operands (42)
- Expressions with only operators and no operands (e.g., 2 +)
- Empty lines or invalid inputs (though it's mentioned that the input will be well-formed)
2. Error Handling:
Implement robust error handling to manage unexpected scenarios, such as mismatched parentheses or invalid operators.
3. Testing:
Thoroughly test your implementation with a range of expressions to ensure correctness. Use both simple and complex expressions to verify that the shunting-yard algorithm and RPN evaluation work as expected.
4. Performance:
The shunting-yard algorithm and RPN evaluation are efficient with a time complexity of O(n), where n is the number of tokens. Ensure that your implementation handles large expressions gracefully.
Conclusion
Mastering the shunting-yard algorithm and its implementation is a valuable skill for handling mathematical expressions in programming assignments. By breaking down the problem into manageable steps and understanding key concepts, you can effectively solve programming assignments involving infix to postfix conversion and evaluation. Practice, thorough testing, and attention to edge cases will help ensure that your implementation is robust and accurate.
With this comprehensive guide, you are well-equipped to handle similar programming assignments and deepen your understanding of expression parsing and evaluation.