Claim Your Discount Today
Take your coding game to new heights with expert help at unbeatable prices. Got a tricky project or a tight deadline? We’ve got you covered! Use code PHHBF10 at checkout and save BIG. Don’t wait—this exclusive Black Friday deal won’t last long. Secure your academic success today!
We Accept
- Sequential Search Algorithm
- Steps for Sequential Search:
- Binary Search Algorithm
- Steps for Binary Search:
- Implementing the Algorithms
- Sequential Search Implementation
- Binary Search Implementation
- Measuring Performance
- Measuring Runtime
- Measuring Comparisons
- Data Collection and Analysis
- Generating Data
- Analyzing Data
- Example Graphs:
- Reporting Findings
- Example Summary:
- Conclusion
A search algorithm is a step-by-step procedure used to locate specific data among a collection of data. The efficiency and effectiveness of these algorithms directly impact the performance of various applications, from simple data lookups to complex data analysis. Programming assignments involving search algorithms are common in computer science education. They provide a deep dive into the efficiency of different search methods and help students understand algorithm performance in real-world scenarios. In this guide, we'll explore how to implement and analyze two fundamental search algorithms: sequential search and binary search. We'll also cover how to measure their performance, produce meaningful data, and report findings effectively.
Sequential Search Algorithm
Sequential search, also known as linear search, is the simplest search algorithm. It works by checking each element in a list one by one until it finds the target element or reaches the end of the list.
Steps for Sequential Search:
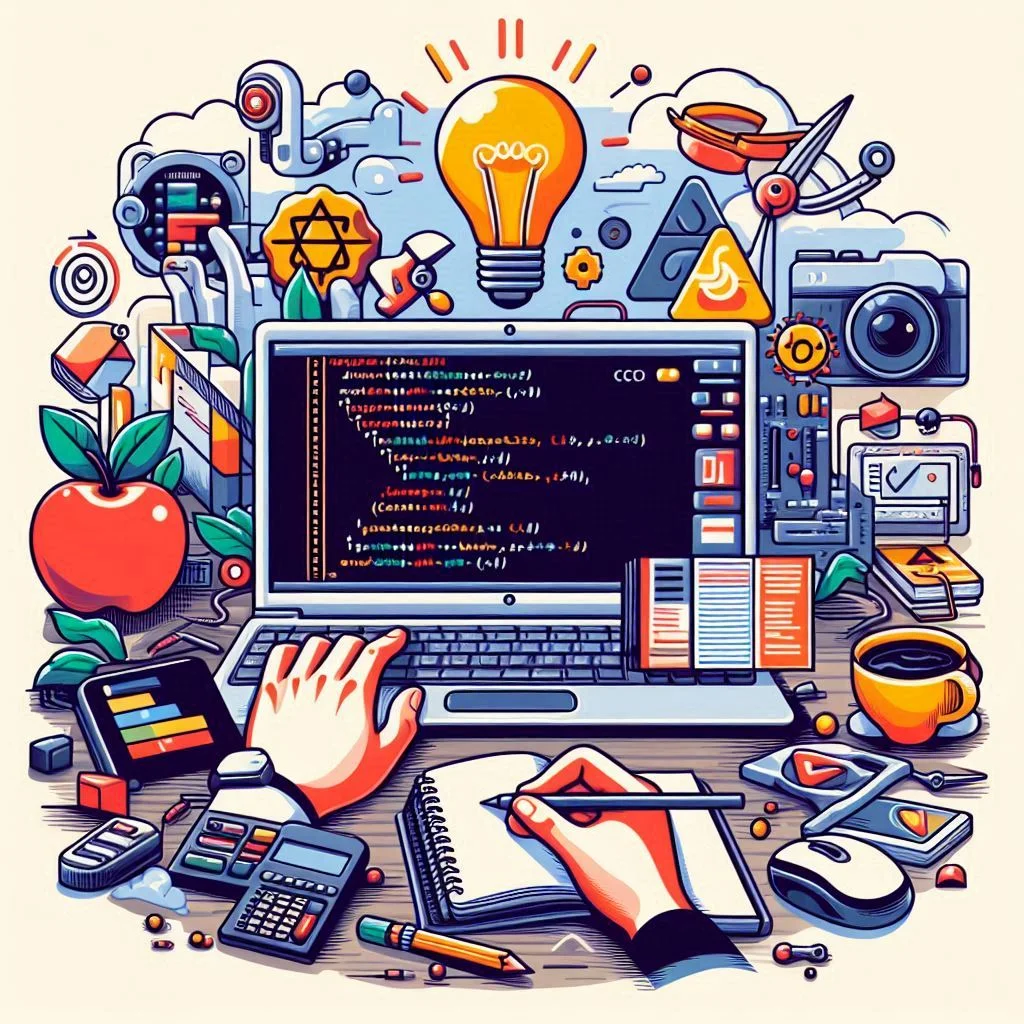
- Start at the beginning of the list.
- Compare each element with the target element.
- If a match is found, return the position of the element.
- If no match is found by the end of the list, return a sentinel value (e.g., -1 or an iterator to the end of the list).
Advantages:
- Simple to implement.
- Works on unsorted data.
Disadvantages:
- Inefficient for large lists as it may require checking every element.
Binary Search Algorithm
Binary search is a more efficient search algorithm but requires that the list be sorted. It works by repeatedly dividing the search interval in half.
Steps for Binary Search:
- Start with the full range of the list.
- Calculate the middle of the range.
- Compare the middle element with the target element.
- If a match is found, return the position of the element.
- If the target is less than the middle element, narrow the search to the lower half.
- If the target is greater, narrow the search to the upper half.
- Repeat until the element is found or the range is empty.
Advantages:
- Much faster than sequential search for large lists.
- Efficient with sorted data.
Disadvantages:
- Requires sorted data.
- More complex to implement.
Implementing the Algorithms
To gain a solid understanding, we need to implement both search algorithms. We'll use C++ for this demonstration, but the concepts are applicable to other languages as well.
Sequential Search Implementation
#include <vector>
#include <algorithm>
#include <functional>
#include <iostream>
// Sequential search algorithm
template<typename Iterator, typename Callable>
Iterator sequential_search(Iterator begin, Iterator end, Callable predicate) {
for (; begin != end; ++begin) {
if (predicate(*begin)) return begin;
}
return end;
}
// Callable function to test equality
struct EqualityTest {
int value;
bool operator()(int item) const {
return item == value;
}
};
In the above implementation:
- Iterator represents the type of the iterator used in the search.
- Callable is a function or function object used to check if an element matches the target.
Binary Search Implementation
#include <vector>
#include <algorithm>
#include <functional>
#include <iostream>
// Binary search algorithm
template<typename Iterator, typename Callable>
Iterator binary_search(Iterator begin, Iterator end, Callable compare) {
while (begin < end) {
Iterator mid = begin + (end - begin) / 2;
int cmp = compare(*mid);
if (cmp == 0) return mid;
if (cmp < 0) end = mid;
else begin = mid + 1;
}
return end;
}
// Callable function for binary search comparisons
struct ComparisonTest {
int value;
int operator()(int item) const {
if (item < value) return -1;
if (item == value) return 0;
return 1;
}
};
In this implementation:
- compare function returns -1, 0, or 1 to indicate whether the element is less than, equal to, or greater than the target value.
Measuring Performance
Performance measurement involves evaluating how each search algorithm performs in terms of execution time and the number of comparisons. This process includes:
- Runtime Measurement: Measure how long each search algorithm takes to complete.
- Comparison Counting: Track how many comparisons each algorithm makes.
Measuring Runtime
To measure runtime, you can use high-resolution timers. In C++, the <chrono> library provides tools for this purpose.
#include <chrono>
auto start = std::chrono::high_resolution_clock::now();
// Call your search function
auto end = std::chrono::high_resolution_clock::now();
std::chrono::duration<double> elapsed = end - start;
std::cout << "Elapsed time: " << elapsed.count() << " seconds" << std::endl;
Measuring Comparisons
To count comparisons, use a counter in your callable function. For both sequential and binary searches, this counter should increment each time a comparison is made.
struct Counter {
int count = 0;
int operator()(int item) {
++count;
// Add comparison logic here
return item < value ? -1 : (item == value ? 0 : 1);
}
};
Data Collection and Analysis
Collect data by running each search algorithm on lists of varying sizes. You'll need to:
- Generate Data: Create lists of random integers and measure the performance for different list sizes.
- Store Results: Save the results in a CSV file for easy analysis.
- Analyze Data: Use statistical and visualization tools to interpret the results.
Generating Data
Create a series of tests with increasing list sizes. For each size, measure the runtime and number of comparisons for each algorithm.
#include <fstream>
#include <vector>
#include <cstdlib>
void generate_data() {
std::ofstream file("results.csv");
file << "Size,MyFindTime,MyFindIter,StdFindTime,StdFindIter,MyBinTime,MyBinIter\n";
for (int size = 1000; size <= 1000000; size += 1000) {
std::vector<int> data(size);
// Fill data with random integers
for (int& value : data) {
value = rand() % (size * 10);
}
// Sequential search
// Measure time and comparisons
// Binary search (requires sorted data)
std::sort(data.begin(), data.end());
// Measure time and comparisons
file << size << "," << myFindTime << "," << myFindIter << "," << stdFindTime << "," << stdFindIter << "," << myBinTime << "," << myBinIter << "\n";
}
file.close();
}
Analyzing Data
Import the CSV file into a spreadsheet application like Excel or Google Sheets. Create graphs to visualize:
- Runtime vs. List Size: Plot runtime for each search algorithm against list size.
- Comparisons vs. List Size: Plot the number of comparisons for each algorithm against list size.
Example Graphs:
- Runtime Graph: X-axis represents list size, Y-axis represents runtime.
- Comparisons Graph: X-axis represents list size, Y-axis represents number of comparisons.
Reporting Findings
After analyzing the data, you need to compile a report summarizing your observations.
Key Components of the Report
- Introduction: Briefly describe the search algorithms and their significance.
- Methodology: Explain how you implemented the algorithms, measured performance, and collected data.
- Results: Present the data in tables and graphs. Discuss the runtime and comparison results for each algorithm.
- Analysis: Interpret the results. Discuss the efficiency of each algorithm and whether the data supports the hypothesis.
- Conclusion: Summarize the key findings and their implications. Reflect on the performance of sequential and binary searches.
Example Summary:
- Runtime: "Binary search is significantly faster than sequential search, especially as the list size grows. This is due to its logarithmic time complexity compared to the linear time complexity of sequential search."
- Comparisons: "Binary search requires fewer comparisons on average, as it halves the search space each step, unlike sequential search which checks each element."
Conclusion
Understanding and analyzing search algorithms is crucial for developing efficient software. By implementing sequential and binary search algorithms, measuring their performance, and analyzing the results, you gain insights into their efficiency and applicability. This comprehensive approach not only helps in academic assignments but also prepares you for real-world software development challenges.
This guide covers the fundamental steps for implementing, measuring, and analyzing search algorithms. With practice and careful analysis, you can effectively tackle similar programming assignments and enhance your problem-solving skills.