Claim Your Discount Today
Ring in Christmas and New Year with a special treat from www.programminghomeworkhelp.com! Get 15% off on all programming assignments when you use the code PHHCNY15 for expert assistance. Don’t miss this festive offer—available for a limited time. Start your New Year with academic success and savings. Act now and save!
We Accept
- Understanding Screen Management
- 1. What is Screen Management?
- 2. Why is Screen Management Important?
- Setting Up Your Screen Manager
- 1. Create a Base Screen Class
- 2. Implement Specialized Screens
- 3. Implement a Screen Manager
- 4. Integrate Screen Manager into Main Loop
- Detailed Implementation and Best Practices
- 1. Creating the Base Screen Class
- 2. Implementing Specialized Screens
- 3. Developing the Screen Manager
- 4. Integrating with the Main Loop
- Common Pitfalls and Troubleshooting
- 1. Redefinition Errors
- 2. Incorrect Code Placement
- 3. Resource Management
- 4. Debugging Tips
- Additional Features and Extensions
- 1. Adding More Screens
- 2. Handling User Input
- 3. Optimizing Performance
- 4. Enhancing Visuals
- 5. Testing and Iteration
- Conclusion
In game development, managing different screens or states is a crucial aspect that influences both the design and user experience. Whether you're working on a complex game framework or a simpler project with multiple views, a robust screen management system helps you handle various game states efficiently. This guide will delve deeply into the principles and implementation of screen management, offering a detailed approach to creating, updating, and rendering different screens or levels within your game. By following this guide, you can solve your game design assignment with ease, ensuring a seamless transition between different game states and enhancing the overall player experience.
Understanding Screen Management
1. What is Screen Management?
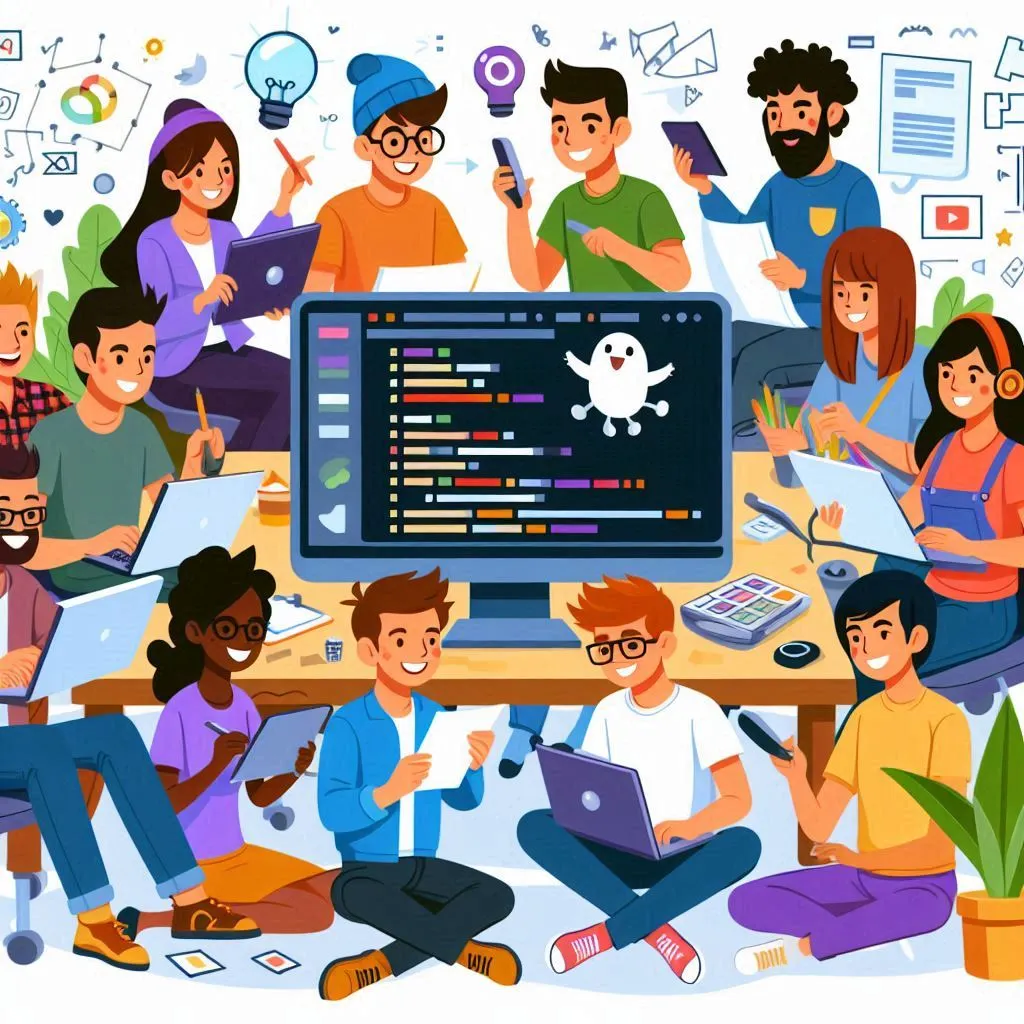
Screen management refers to the organization and handling of different screens or states within a game. These screens could include the main menu, gameplay levels, pause menus, game over screens, and more. Each screen represents a unique state or phase of the game, and managing transitions between these states is essential for a cohesive gaming experience.
2. Why is Screen Management Important?
Proper screen management ensures that your game can transition smoothly between various states. It helps in:
- Organizing Code: Keeps the codebase modular and manageable by separating different game states into distinct classes.
- Improving Maintainability: Makes it easier to update and maintain individual screens or levels without affecting others.
- Enhancing User Experience: Provides a seamless and intuitive flow between different parts of the game.
Setting Up Your Screen Manager
To manage screens effectively, you need a structured approach that involves creating a base screen class, implementing specialized screens, and managing transitions between them. Here’s a step-by-step guide to setting up your screen manager.
1. Create a Base Screen Class
The base screen class serves as the foundation for all other screens. It should provide a common interface for rendering and updating screens, ensuring that each screen follows a consistent structure.
class GameScreen {
protected:
SDL_Renderer* m_renderer; // Renderer for drawing on the screen
public:
GameScreen(SDL_Renderer* renderer) : m_renderer(renderer) {}
virtual ~GameScreen() { m_renderer = nullptr; }
// Pure virtual functions to be overridden by derived classes
virtual void Render() = 0;
virtual void Update(float deltaTime, SDL_Event e) = 0;
};
In this class:
- m_renderer is a pointer to the SDL renderer used for drawing.
- Render and Update are pure virtual functions, meaning derived classes must provide implementations for these functions.
2. Implement Specialized Screens
Each specific screen or level in your game should be a subclass of GameScreen. Implement the Render and Update methods to handle the unique functionality of each screen.
class GameScreenLevel1 : public GameScreen {
private:
Texture2D* m_background_texture; // Pointer to the background texture
public:
GameScreenLevel1(SDL_Renderer* renderer) : GameScreen(renderer), m_background_texture(nullptr) {}
~GameScreenLevel1() { delete m_background_texture; }
void Render() override {
if (m_background_texture) {
m_background_texture->Render(Vector2D(), SDL_FLIP_NONE);
}
}
void Update(float deltaTime, SDL_Event e) override {
// Update level-specific logic here
}
bool SetUpLevel() {
m_background_texture = new Texture2D(m_renderer);
if (!m_background_texture->LoadFromFile("Images/test.bmp")) {
std::cout << "Failed to load background texture!" << std::endl;
return false;
}
return true;
}
};
In GameScreenLevel1:
- m_background_texture is a pointer to the texture used as the background.
- Render method draws the background texture.
- Update method can be used to handle level-specific updates.
- SetUpLevel method loads the background texture and initializes the level.
3. Implement a Screen Manager
The screen manager is responsible for handling transitions between different screens. It maintains a reference to the current screen and provides functionality to switch screens.
class GameScreenManager {
private:
SDL_Renderer* m_renderer; // Renderer used by the screen manager
GameScreen* m_current_screen; // Pointer to the current screen
public:
GameScreenManager(SDL_Renderer* renderer, SCREENS initialScreen) : m_renderer(renderer), m_current_screen(nullptr) {
SwitchScreen(initialScreen);
}
~GameScreenManager() {
delete m_current_screen;
}
void SwitchScreen(SCREENS new_screen) {
delete m_current_screen;
m_current_screen = nullptr;
switch (new_screen) {
case SCREEN_LEVEL1:
m_current_screen = new GameScreenLevel1(m_renderer);
static_cast<GameScreenLevel1*>(m_current_screen)->SetUpLevel();
break;
// Add cases for other screens here
default:
// Handle default case
break;
}
}
void Render() {
if (m_current_screen) {
m_current_screen->Render();
}
}
void Update(float deltaTime, SDL_Event e) {
if (m_current_screen) {
m_current_screen->Update(deltaTime, e);
}
}
};
In GameScreenManager:
- SwitchScreen method changes the current screen based on the provided enum value.
- Render method calls the Render function of the current screen.
- Update method calls the Update function of the current screen.
4. Integrate Screen Manager into Main Loop
Finally, integrate the screen manager into your main game loop to handle rendering and updating of screens.
Uint32 g_old_time;
GameScreenManager* game_screen_manager;
if (InitSDL()) {
game_screen_manager = new GameScreenManager(g_renderer, SCREEN_LEVEL1);
g_old_time = SDL_GetTicks();
}
void Render() {
game_screen_manager->Render();
}
void Update(SDL_Event e) {
Uint32 new_time = SDL_GetTicks();
float deltaTime = (float)(new_time - g_old_time) / 1000.0f;
game_screen_manager->Update(deltaTime, e);
g_old_time = new_time;
}
Here:
- g_old_time tracks the previous time to calculate deltaTime.
- game_screen_manager manages screen transitions and updates.
Detailed Implementation and Best Practices
1. Creating the Base Screen Class
The GameScreen class is an abstract base class that defines the essential interface for all screens. It ensures that every derived class implements the Render and Update methods.
2. Implementing Specialized Screens
Derived classes like GameScreenLevel1 must implement the Render and Update methods to handle their specific functionality. Each screen should manage its resources, such as textures or sounds, and clean them up in the destructor to prevent memory leaks.
3. Developing the Screen Manager
The GameScreenManager class handles the logic for switching between screens. It manages the lifecycle of screens, ensuring that only one screen is active at a time. The SwitchScreen method deletes the current screen and creates a new one based on the provided SCREENS enum.
4. Integrating with the Main Loop
Integrating the screen manager into your main loop involves calling the Render and Update methods of the GameScreenManager in the main game loop. This setup ensures that the current screen is updated and rendered appropriately.
Common Pitfalls and Troubleshooting
1. Redefinition Errors
If you encounter redefinition errors, ensure that all header files use include guards. Include guards prevent multiple inclusions of the same header file, which can cause redefinition errors.
2. Incorrect Code Placement
Double-check that code snippets are placed in the correct locations. Misplaced code can lead to compilation errors or incorrect behavior. Follow the structure outlined in this guide to ensure proper placement.
3. Resource Management
Properly manage resources such as textures and sounds. Ensure that resources are loaded correctly and released when no longer needed. Failure to manage resources can lead to memory leaks or crashes.
4. Debugging Tips
- Check for Errors: Use debugging tools to check for runtime errors or crashes.
- Verify Logic: Ensure that screen transitions and updates are handled correctly.
- Review Code: Go through each step carefully and verify that all code is implemented as intended.
Additional Features and Extensions
1. Adding More Screens
To add more screens, extend the SCREENS enum and add corresponding cases in the SwitchScreen method of the GameScreenManager. Implement new classes for each screen, following the same pattern as GameScreenLevel1.
2. Handling User Input
Implement user input handling in the Update method of each screen. For example, you can detect key presses or mouse clicks to switch screens or interact with the game.
3. Optimizing Performance
Optimize performance by minimizing resource loading and unloading during screen transitions. Use efficient algorithms for updating and rendering to ensure smooth gameplay.
4. Enhancing Visuals
Enhance visuals by implementing advanced rendering techniques or adding animations. Improve the visual appeal of each screen by using high-quality textures and effects.
5. Testing and Iteration
Thoroughly test each screen and transition to ensure that they work as expected. Gather feedback from playtesting and iterate on the design to improve the overall experience.
Conclusion
Effective screen management is essential for developing a well-structured and enjoyable game. By creating a base screen class, implementing specialized screens, and managing transitions through a screen manager, you can build a flexible and organized game framework. This approach not only improves code maintainability but also enhances the gameplay experience by providing a seamless flow between different game states. To complete your C++ assignment, consider integrating these principles to ensure a smooth and efficient game development process.
Implementing screen management requires careful planning and attention to detail. Follow the principles outlined in this guide to create a robust system that meets the needs of your project. With practice and iteration, you'll be able to build complex and engaging games with smooth transitions and a cohesive structure. If you need assistance with programming assignments related to screen management, don't hesitate to seek help to ensure your implementation is both efficient and effective.