Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 10% off on your all programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
- Introduction to Reverse Engineering and Disassembly
- Understanding the Problem
- Processor Architecture
- Input and Output
- Understanding the Machine Architecture
- Phase 1: Basic Disassembly
- a. Reading the Binary File
- b. Processing the Binary Data
- c. Converting Words to Machine Code
- d. Disassembling Instructions
- e. Outputting Results
- Phase 2: Advanced Disassembly with Code Execution Tracing
- a. Initializing Execution State
- b. Tracing Execution
- c. Disassembling Reachable Code
- d. Managing Data vs. Code
- Example Implementation
- Testing and Validation
- Conclusion
Disassembling machine code involves converting binary data, which the computer understands, into human-readable assembly language instructions. This process is essential for analyzing and understanding low-level code, especially when dealing with reverse engineering tasks. Whether you’re working with a known architecture or a hypothetical one like the S20 machine, the principles remain consistent.
Computer architecture assignment that involves reverse engineering and disassembling can be among the most challenging tasks for students. These python assignments often require a deep understanding of both theoretical concepts and practical skills. If you’re dealing with an assignment similar to the one involving the S20 processor and binary file analysis, this guide will walk you through a detailed, step-by-step approach to help you effectively tackle such complex tasks.
Introduction to Reverse Engineering and Disassembly
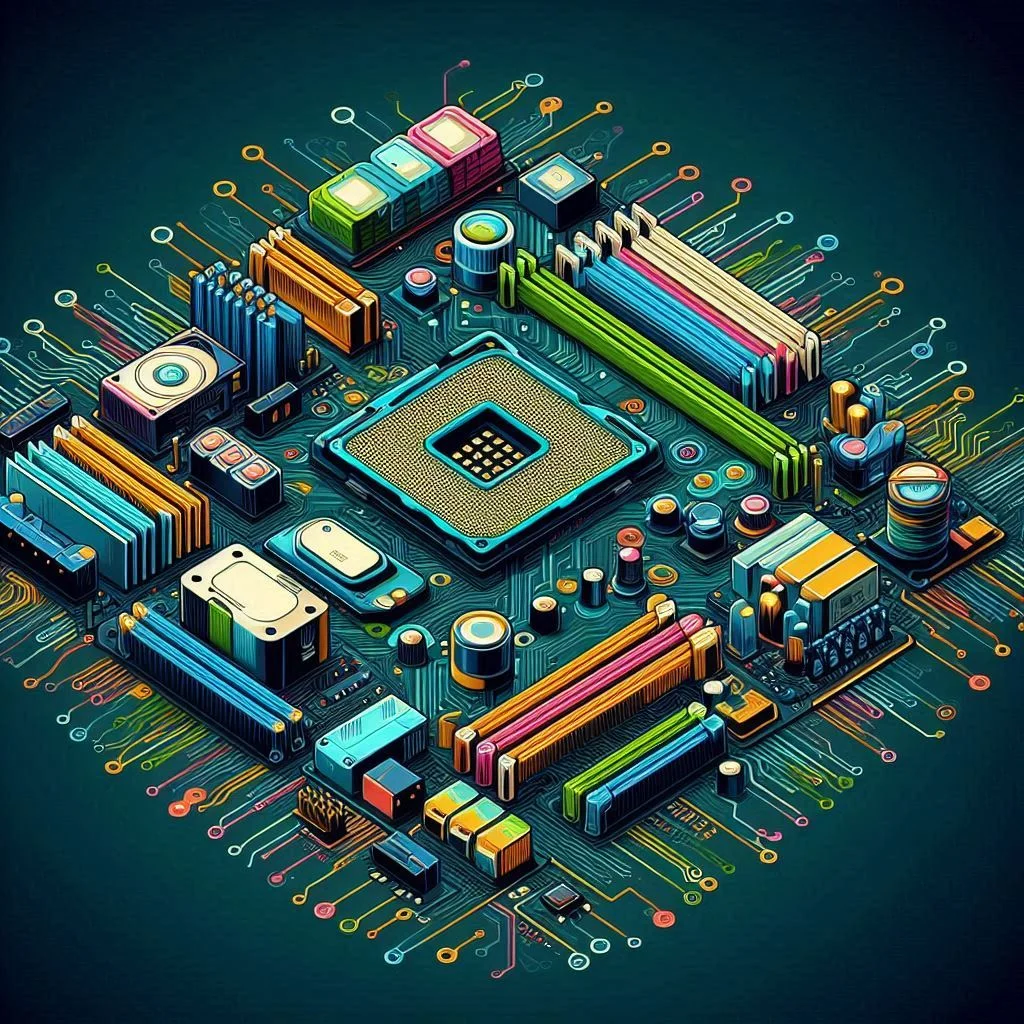
Reverse engineering is the process of analyzing a system to understand its components and functionality, often to reconstruct its design or functionality. Disassembly is a specific form of reverse engineering where you convert machine code (binary) back into human-readable assembly code. This process is crucial for understanding how a program operates at a low level, especially when source code is not available.
Assignments that involve disassembling machine code often require a solid grasp of computer architecture, binary data manipulation, and assembly language. Here’s a detailed breakdown of how to solve such programming assignments.
Understanding the Problem
Before diving into the implementation, let's break down the problem:
Processor Architecture
The S20 processor is a hypothetical processor with a specific instruction set and data format, detailed in a datasheet. Here's what you need to understand:
- Instruction Set: Defines how instructions are formatted and executed. It includes the opcode (operation code) and operands.
- Instruction Format: In this case, each instruction is 3 bytes long. The format includes how these bytes are arranged and interpreted.
- Endianness: The byte order in the binary file. We need to handle this correctly when interpreting the binary data.
Input and Output
- Input: A binary file containing machine code for the S20 processor. Each instruction is represented by a 3-byte sequence.
- Output: A human-readable assembly language representation of the binary data. This output should clearly distinguish executable code from non-executable data.
Understanding the Machine Architecture
Before diving into disassembly, it's crucial to understand the machine architecture of the processor you're working with. This includes:
- Instruction Set: The instruction set defines all the operations that the processor can perform. Each instruction corresponds to a specific opcode (operation code) and may include operands (data or addresses).
- Memory Model: Understand how the machine addresses and accesses memory. This includes whether the machine uses a flat memory model, segmented memory, or some other model.
- Endianness: Endianness determines the order in which bytes are stored in memory. In big-endian format, the most significant byte is stored at the lowest memory address, whereas in little-endian format, the least significant byte is stored first.
- Instruction Format: The format of instructions varies between architectures. You need to know how to interpret the bits of an instruction, including the opcode and operands.
Phase 1: Basic Disassembly
The first phase involves converting the binary file into assembly instructions without worrying about whether the code is reachable. This process generally involves the following steps:
a. Reading the Binary File
The first step is to read the binary file into a format that can be processed. In Python, you can use the built-in functions to handle binary data.
with open('binaryfile.bin', 'rb') as file:
byte_array = file.read()
Here, byte_array contains the raw bytes of the binary file.
b. Processing the Binary Data
The next step is to process the byte array into instruction words. For a hypothetical machine like the S20, where each instruction is 3 bytes long, you can split the byte array into chunks.
instructions = [byte_array[i:i+3] for i in range(0, len(byte_array), 3)]
Each chunk (3 bytes) represents one instruction.
c. Converting Words to Machine Code
Depending on the endianness of the machine, you may need to convert the byte chunks into a word. For a big-endian machine like the S20, the most significant byte comes first.
def to_word(bytes_):
return int.from_bytes(bytes_, byteorder='big')
This function converts a 3-byte chunk into a single integer word.
d. Disassembling Instructions
Once you have the word, you need to convert it into assembly instructions. This involves interpreting the opcode and operands based on the instruction set. For the S20, your disassembly function might look like this:
def disassemble(word):
opcode = (word >> 16) & 0xFF
operand = word & 0xFFFF
return f'OPCODE {opcode}, OPERAND {operand}'
This function extracts the opcode and operand from the word and returns a human-readable assembly instruction.
e. Outputting Results
Finally, you’ll want to output the results of your disassembly. You can either print them or save them to a file.
for inst in instructions:
word = to_word(inst)
print(disassemble(word))
Phase 2: Advanced Disassembly with Code Execution Tracing
The second phase involves tracing code execution to only disassemble reachable instructions. This phase is more complex and involves simulating how the code would execute starting from a known entry point.
a. Initializing Execution State
You need to keep track of which addresses have been visited to avoid disassembling the same code multiple times.
visited_addresses = set()
def mark_visited(address):
visited_addresses.add(address)
b. Tracing Execution
Starting from the entry point (usually address 0), disassemble instructions and follow any branches or jumps to other instructions. This requires simulating how the program would execute.
def trace_code(address):
if address in visited_addresses:
return
visited_addresses.add(address)
# Disassemble the instruction at this address
# Follow branches and trace further
c. Disassembling Reachable Code
For each address that you trace, disassemble the instruction and follow any branches to other instructions.
def disassemble_reachable_code(start_address):
trace_code(start_address)
# Output or process disassembled instructions based on reachability
d. Managing Data vs. Code
During the tracing process, you need to differentiate between code and data. Data sections are non-executable and should be labeled accordingly.
def is_data(address):
def is_data(address):
return address not in visited_addresses
Example Implementation
Here’s a simplified example of a disassembler in Python, assuming a hypothetical machine with a 3-byte instruction set:
def to_word(bytes_):
return int.from_bytes(bytes_, byteorder='big')
def disassemble(word):
opcode = (word >> 16) & 0xFF
operand = word & 0xFFFF
return f'OPCODE {opcode}, OPERAND {operand}'
def read_binary_file(filename):
with open(filename, 'rb') as file:
return file.read()
def process_binary_data(byte_array):
return [byte_array[i:i+3] for i in range(0, len(byte_array), 3)]
def main():
byte_array = read_binary_file('binaryfile.bin')
instructions = process_binary_data(byte_array)
for inst in instructions:
word = to_word(inst)
print(disassemble(word))
if __name__ == '__main__':
main()
Testing and Validation
After implementing your disassembler, it’s crucial to test it thoroughly. Use various binary files to ensure that your disassembler handles different scenarios correctly. Pay attention to:
- Code Coverage: Ensure that all reachable code is disassembled.
- Accuracy: Verify that the disassembled instructions match the expected assembly language instructions.
- Edge Cases: Test with different instruction lengths, data patterns, and endianness scenarios.
Conclusion
Disassembling machine code from binary files involves a blend of understanding machine architecture, processing binary data, and simulating code execution. Creating a disassembler for a hypothetical processor involves several steps: reading binary data, parsing instructions, simulating code execution, and distinguishing code from data. By following a structured approach, testing thoroughly, and seeking help when needed, you can effectively solve your programming assignments. This detailed guide provides a comprehensive approach to understand and solve programming assignments, preparing you for future challenges in programming and reverse engineering.