Claim Your Discount Today
Ring in Christmas and New Year with a special treat from www.programminghomeworkhelp.com! Get 15% off on all programming assignments when you use the code PHHCNY15 for expert assistance. Don’t miss this festive offer—available for a limited time. Start your New Year with academic success and savings. Act now and save!
We Accept
- Understanding Regular Expressions (Regex)
- What Are Regular Expressions?
- Problem Statement Overview
- General Approach to Regex Problems
- Solution Approach for Problem A
- Solution Approach for Problem B
- Solution Approach for Problem C
- Understanding Deterministic Finite Automata (DFAs)
- What Is a DFA?
- General Approach to DFA Problems
- DFA Problem 1: Strings Without the Substring "ABBA"
- Solution:
- DFA File: dfa1.txt
- DFA File: dfa2.txt
- DFA File: dfa3.txt
- How to Run
- Conclusion
Regular Expressions (Regex) and Deterministic Finite Automata (DFA) are core tools in computer science that play a crucial role in pattern recognition, text processing, and language parsing. Whether you're validating input, searching for specific patterns in large datasets, or building state machines for automating decision-making, understanding how to effectively use Regex and DFAs is essential.
In programming assignments, students are often required to write solutions that rely on these concepts to solve real-world problems. Regular expressions enable you to define concise yet powerful patterns for matching, searching, and manipulating text, while DFAs provide a structured method for modeling the behavior of systems with finite states, enabling efficient pattern recognition.
This blog will guide you through the process of solving common assignments involving Regular Expressions and DFAs. We'll walk through examples, explain how to think about these problems and provide strategies for crafting your own solutions. By the end, you’ll have the skills to tackle similar assignments with confidence and solve your computational theory assignment effectively gaining a deeper understanding of how these fundamental tools can be applied to a wide range of computational problems.
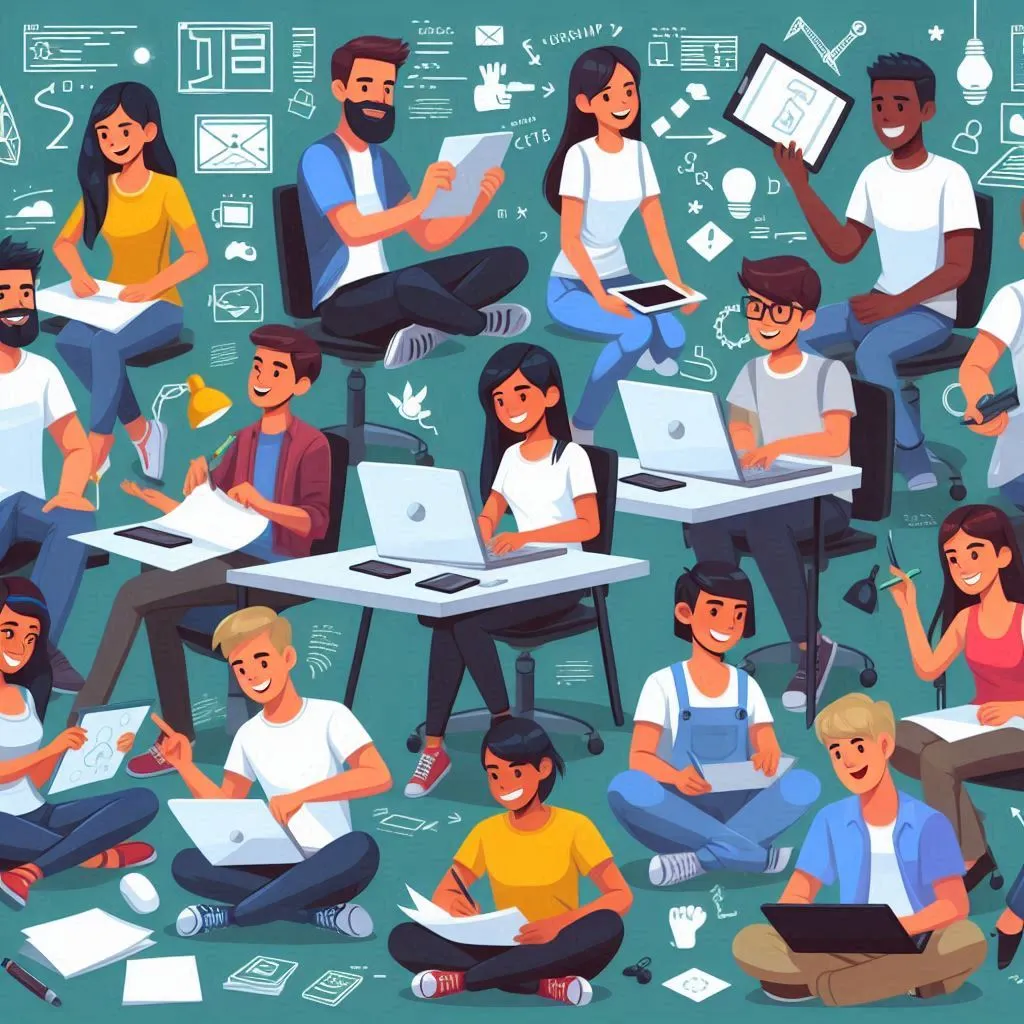
Understanding Regular Expressions (Regex)
What Are Regular Expressions?
Regular expressions (regex) are sequences of characters that form a search pattern, often used for pattern matching within strings. They're powerful tools for text processing, allowing you to define complex string patterns with simple syntax.
In most programming languages, regex is a built-in library or function that can be imported and utilized to match, search, or substitute specific patterns in strings.
Problem Statement Overview
We’ll start by breaking down the typical types of problems that involve regular expressions. Here’s an example of a problem statement:
1. A string over the alphabet {a, b} that contains no more than one pair of consecutive b’s.
- Acceptable Strings: abba, aa, aab
- Rejected Strings: bbbab, abbba
2. A string of odd length that contains digits and lowercase letters, where the only even number allowed is 4.
- Acceptable Strings: sample4, a1943bc
- Rejected Strings: sample6, another1b3
3. A function that swaps the last two subdirectories of a Seattle University URL if the URL contains two or more subdirectories.
We’ll solve these problems using Python’s re library for regex handling.
General Approach to Regex Problems
1. Understand the Problem Requirements:
Break down the problem's requirements into clear statements. For instance, if a problem specifies that a string should have no more than one pair of consecutive b's, that immediately tells you two things: the string is limited to certain characters (likely {a, b}), and you need a condition for managing consecutive characters.
2. Design the Pattern:
Once you know what you're looking for, start building the regular expression piece by piece. Begin with simpler patterns and test them incrementally. Often, problems require the use of special regex characters like:
- o . (any character),
- o * (zero or more occurrences),
- o + (one or more occurrences),
- o [] (character set),
- o | (alternation, similar to OR),
- o ^ (start of string),
- o $ (end of string).
3. Test the Regex with Multiple Cases:
Test the regex using both positive (matching) and negative (non-matching) examples. Make sure your test strings are diverse to cover various edge cases.
4. Refine and Optimize:
Optimize the regex for performance, especially if you're dealing with large strings. While regex is efficient, overly complex patterns can slow down matching.
Solution Approach for Problem A
Step 1: Analyzing the Problem
The problem requires us to create a regular expression that matches a string containing at most one occurrence of consecutive b’s. This means we have to ensure that:
- The string can have zero or one bb, but not more.
- The string can contain any number of a’s.
Step 2: Writing the Regular Expression
To write the regular expression, we can think about the problem in terms of how many occurrences of bb are allowed:
- The string can be composed of a sequence of a’s and b’s, with the only constraint being that the bb pair should appear at most once.
The following regular expression can be constructed:
import re
# Regular expression for the problem
pattern = re.compile(r'^(a|b)*bb?(a|b)*$')
# Test strings
accepted = ["abba", "aa", "aab"]
rejected = ["bbbab", "abbba", "bbb"]
# Testing the regex
for test_str in accepted:
if pattern.match(test_str):
print(f"Accepted: {test_str}")
else:
print(f"Rejected (wrongly): {test_str}")
for test_str in rejected:
if not pattern.match(test_str):
print(f"Correctly Rejected: {test_str}")
else:
print(f"Accepted (wrongly): {test_str}")
Explanation
- The regular expression r'^(a|b)*bb?(a|b)*$' works as follows:
- o ^(a|b)*: The string can start with any number of a’s or b’s.
- o bb?: Matches zero or one occurrence of consecutive b’s.
- o (a|b)*$: The string can end with any combination of a’s or b’s.
The regular expression ensures that there is no more than one pair of consecutive b’s by limiting bb? to one occurrence in the pattern.
Step 3: Testing the Regex
The test cases show that the pattern matches strings like abba or aa, but correctly rejects strings with multiple occurrences of consecutive b’s, such as abbba.
Solution Approach for Problem B
Step 1: Analyzing the Problem
Here, the string must:
- Be of odd length.
- Contain digits and lowercase letters.
- Only allow the digit 4 as the even number.
Step 2: Writing the Regular Expression
We’ll construct a regular expression to match the criteria. This includes checking the string's length and ensuring the only allowed even digit is 4.
import re
# Regular expression for the problem
pattern = re.compile(r'^[a-z\d]*[4][a-z\d]*$')
# Test strings
accepted = ["sample4", "abcde4", "1943another"]
rejected = ["sample6", "another!13"]
# Testing the regex
for test_str in accepted:
if pattern.match(test_str):
print(f"Accepted: {test_str}")
else:
print(f"Rejected (wrongly): {test_str}")
for test_str in rejected:
if not pattern.match(test_str):
print(f"Correctly Rejected: {test_str}")
else:
print(f"Accepted (wrongly): {test_str}")
Solution Approach for Problem C
The third problem involves creating a function that swaps the last two subdirectories of a Seattle University URL if the URL contains two or more subdirectories.
Step 1: Writing the Function
Here’s how we can implement the fixURL function:
import re
def fixURL(url):
pattern = re.compile(r'(https?://[a-zA-Z0-9\-_.]+/)([a-zA-Z0-9_\-/.]+/)([a-zA-Z0-9_\-/.]+/)$')
# Check if the URL matches the pattern
match = pattern.match(url)
if match:
# Swap the last two subdirectories
new_url = f"{match.group(1)}{match.group(3)}{match.group(2)}"
return new_url
else:
return url
Explanation
- The regular expression matches any valid URL with at least two subdirectories.
- The function swaps the last two subdirectories if the match is successful.
Understanding Deterministic Finite Automata (DFAs)
What Is a DFA?
A Deterministic Finite Automaton (DFA) is a state machine that processes a string of input symbols and determines if the string is accepted by the machine. It consists of:
- A finite set of states.
- An input alphabet.
- A transition function that maps states to other states based on input symbols.
- A start state and one or more accepting states.
General Approach to DFA Problems
1. Understand the Problem Specification:
Read the description carefully to determine the input alphabet and the acceptance condition. For example, "All strings that do not contain ABBA."
2. Design the DFA:
- Define the States: Identify different states based on the requirement. For the "no ABBA" condition, think about how each prefix of ABBA (like A, AB, ABB) affects the state transitions.
- Define Transitions: Map out the transitions for each input symbol in the alphabet.
- Mark Accepting and Rejecting States: Identify which states represent acceptance or rejection of the input.
3. Write the DFA File:
The DFA file typically consists of:
- Input Alphabet: A list of symbols used by the DFA.
- States: Lines for each state, indicating whether it's accepting or rejecting and listing the next state for each symbol.
4. Test the DFA:
Create a test file with input strings and check if the DFA correctly accepts or rejects them.
DFA Problem 1: Strings Without the Substring "ABBA"
Step 1: Designing the DFA
We want to design a DFA that accepts strings from the alphabet {A, B, C} that do not contain the substring ABBA.
To solve this, we will:
- Create states representing the progress toward forming the substring ABBA.
- Ensure that the DFA rejects any string that completes the sequence ABBA.
Solution:
DFA Construction
1. States:
- S0: Initial state (no part of "ABBA" seen)
- S1: Seen "A"
- S2: Seen "AB"
- S3: Seen "ABB"
- S4: Seen "ABBA" (rejecting state)
2. Transitions:
- From S0:
- On 'A': go to S1
- On 'B': go to S0
- On 'C': go to S0
- From S1:
- On 'A': go to S1
- On 'B': go to S2
- On 'C': go to S0
- From S2:
- On 'A': go to S3
- On 'B': go to S0
- On 'C': go to S0
- From S3:
- On 'A': go to S4 (rejecting state, seen "ABBA")
- On 'B': go to S0
- On 'C': go to S0
- From S4:
- On 'A': go to S4
- On 'B': go to S4
- On 'C': go to S4
3. Accepting States:
- S0, S1, S2, and S3 are accepting states.
DFA File: dfa1.txt
A B C
+ 1 0 0
- 1 2 0
- 3 0 0
- 4 0 0
- 4 4 4
DFA for Strings that Do Not Contain "ABBA"
Python Program: generate_dfa1.py
def generate_dfa1():
with open('dfa1.txt', 'w') as f:
# Alphabet
f.write("A B C\n")
# State 0
f.write("+ 1 0 0\n")
# State 1
f.write("- 1 2 0\n")
# State 2
f.write("- 3 0 0\n")
# State 3
f.write("- 4 0 0\n")
# State 4 (rejecting state)
f.write("- 4 4 4\n")
generate_dfa1()
DFA Problem 2: Strings with an Even Number of the Substring "XY"
This DFA needs to count how many times the substring XY occurs in the input string. If the number of occurrences is even, the DFA should accept the string.
Solution:
DFA Construction
1. States:
- S0: Initial state (even number of "XY")
- S1: Seen "X" (waiting for 'Y' to form "XY")
- S2: Seen "XY" (odd number of "XY")
- S3: Seen "XYX" (waiting for 'Y' to form another "XY")
2. Transitions:
- From S0:
- On 'X': go to S1
- On 'Y': go to S0
- From S1:
- On 'X': go to S1
- On 'Y': go to S2
- From S2:
- On 'X': go to S3
- On 'Y': go to S2
- From S3:
- On 'X': go to S1
- On 'Y': go to S0
3. Accepting States:
- S0 and S2 are accepting states (even number of "XY").
DFA File: dfa2.txt
X Y
+ 1 0
- 2 1
+ 3 2
- 0 3
DFA for Strings with Even Number of "XY"
Python Program: generate_dfa2.py
def generate_dfa2(): with open('dfa2.txt', 'w') as f: # Alphabet f.write("X Y\n") # State 0 f.write("+ 1 0\n") # State 1 f.write("- 2 1\n") # State 2 f.write("+ 3 2\n") # State 3 f.write("- 0 3\n") generate_dfa2()
DFA Problem 3: All strings on Σ = {A, B, C, D} that adhere to the regular expression `^(D?(B|CD)*A)$`
Solution:
DFA Construction
1. States:
- S0: Initial state (no input processed)
- S1: Seen "D" (optional prefix)
- S2: Seen "B" (waiting for more or end with "A")
- S3: Seen "CD" (waiting for "A" or more "CD")
- S4: Seen "B" followed by "A"
- S5: Seen "CD" followed by "A"
- S6: Accepting state (valid end)
- 2. Transitions:
- From S0:
- On 'D': go to S1
- On 'B': go to S2
- On 'C': go to S0
- On 'A': go to S0
- From S1:
- On 'B': go to S2
- On 'C': go to S3
- On 'D': go to S1
- On 'A': go to S6
- From S2:
- On 'B': go to S2
- On 'C': go to S0
- On 'D': go to S0
- On 'A': go to S4
- From S3:
- On 'B': go to S2
- On 'C': go to S3
- On 'D': go to S0
- On 'A': go to S5
- From S4:
- On 'A': go to S6
- On any other character: go to S0
- From S5:
- On 'A': go to S6
- On any other character: go to S0
- From S6:
- On any character: go to S0
3. Accepting States:
- S6 is the accepting state.
DFA File: dfa3.txt
A B C D
+ 1 2 0 1
- 2 3 0 1
+ 2 2 0 2
+ 0 3 0 0
- 0 0 0 0
DFA for Strings Matching ^(D?(B|CD)*A)$
Python Program: generate_dfa3.py
def generate_dfa3():
with open('dfa3.txt', 'w') as f:
# Alphabet
f.write("A B C D\n")
# State 0
f.write("+ 1 2 0 1\n")
# State 1
f.write("- 2 3 0 1\n")
# State 2
f.write("+ 2 2 0 2\n")
# State 3
f.write("+ 0 3 0 0\n")
# State 4 (rejecting state)
f.write("- 0 0 0 0\n")
generate_dfa3()
Explanation
- DFA for Strings That Do Not Contain "ABBA":
- S0: Start state; transitions based on input characters and tracks if we see the start of "ABBA".
- S1: After seeing "A".
- S2: After seeing "AB".
- S3: After seeing "ABB".
- S4: After seeing "ABBA" (rejecting state).
- DFA for Strings with Even Number of "XY":
- S0: Start state; even count of "XY".
- S1: Seen "X" waiting for "Y".
- S2: Seen "XY" (odd count of "XY").
- S3: Seen "XYX" waiting for next "Y".
- DFA for Strings Matching ^(D?(B|CD)*A)$:
- S0: Start state; can be empty or start with "D".
- S1: After seeing "D"; transitions to handle patterns of "B" and "CD".
- S2: Seen "B" (possibly more "B"s).
- S3: Seen "CD" (transition to accept or reject based on pattern completion).
- S4: Final accepting state.
How to Run
- Generate DFA Files: Run each of the Python programs (generate_dfa1.py, generate_dfa2.py, generate_dfa3.py) to create the respective DFA files.
- Test DFA Files: Use the DFA simulator as follows:
python3 dfa.py dfa1.txt test_strings.txt
python3 dfa.py dfa2.txt test_strings.txt
python3 dfa.py dfa3.txt test_strings.txt
Ensure test_strings.txt contains strings to test against each DFA.
Feel free to adjust the DFA description files or test strings as needed for your specific requirements or to test different cases.
Conclusion
By following these steps, you can systematically solve your Python assignment related to regular expressions or DFA (Deterministic Finite Automaton). The key lies in understanding the problem requirements, breaking them down into smaller parts, and iterating through design and testing. With practice, you will develop the skills to solve increasingly complex problems in these domains.
Regular expressions and DFAs are powerful tools for pattern matching and string recognition. By breaking down problems into manageable steps, you can design effective solutions for both regex and DFA-related tasks. Regular expressions allow you to define complex search patterns, while DFAs provide a structured way to process input and determine acceptance or rejection of strings.
By applying the strategies outlined in this guide, you'll be able to tackle similar assignments confidently. Regular expressions and DFAs play significant roles in various fields, from web development to computational theory, and mastering these concepts will enhance your problem-solving skills. If you ever find yourself in need of assistance with programming assignments, leveraging these strategies will prove invaluable.