Claim Your Offer
New semester, new challenges—but don’t stress, we’ve got your back! Get expert programming assignment help and breeze through Python, Java, C++, and more with ease. For a limited time, enjoy 10% OFF on all programming assignments with the Spring Special Discount! Just use code SPRING10OFF at checkout! Why stress over deadlines when you can score high effortlessly? Grab this exclusive offer now and make this semester your best one yet!
We Accept
- 1. Understanding Binary Trees
- What is a Binary Tree?
- Types of Binary Trees
- 2. Core Binary Tree Operations
- Counting Leaves
- Counting Non-Leaf Nodes
- Calculating Height
- 3. Special Properties of Binary Trees
- Checking if a Tree is Perfect
- Checking if a Tree is Degenerate
- 4. Bonus: Converting Degenerate Trees to Lists
- 5. Practical Considerations
- Edge Cases
- Efficiency
- Testing
- Conclusion
Recursive binary trees are a fundamental concept in computer science, crucial for various algorithms and data structures. Understanding binary trees involves grasping their structure, operations, and special properties. This comprehensive guide will explore binary trees in depth, covering fundamental operations like counting leaves, nodes, and calculating height, as well as special properties such as perfect, degenerate trees, and techniques for solving Haskell assignments involving recursive data types like binary trees. We will also delve into a bonus function for converting degenerate trees to lists.
This data structure assignment requires implementing several functions to work with a recursive binary tree data structure. The tasks involve counting leaves and non-leaf nodes, calculating the height of the tree, and checking for special properties such as whether the tree is perfect or degenerate. There is a problem that requires converting a degenerate tree into a list.
1. Understanding Binary Trees
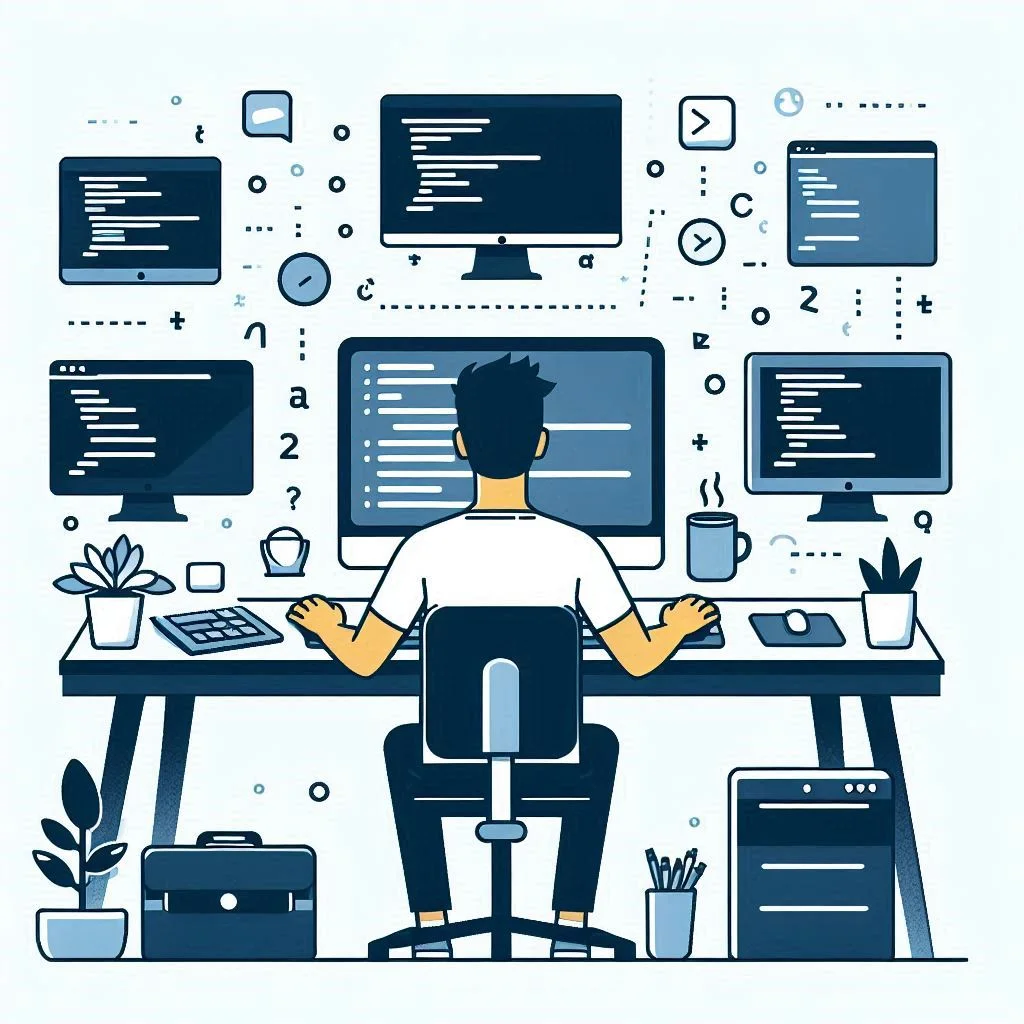
What is a Binary Tree?
A binary tree is a hierarchical structure where each node has up to two children, known as the left and right children. The topmost node is called the root, and nodes with no children are called leaves.
Basic Structure:
A
/ \
B C
/ \
D E
In this example:
- A is the root.
- B and C are children of A.
- D and E are children of B, making D and E leaves.
Types of Binary Trees
- Full Binary Tree: Every node has either 0 or 2 children. All nodes have exactly two children except the leaves.
- Complete Binary Tree: All levels, except possibly the last, are fully filled. The last level is filled from left to right.
- Perfect Binary Tree: All internal nodes have two children, and all leaves are at the same level.
- Degenerate (Pathological) Tree: Each node has either zero or one child, resembling a linked list.
2. Core Binary Tree Operations
Counting Leaves
Definition: Leaves are nodes with no children. To count them, traverse the tree and count nodes that do not have any left or right children.
Recursive Approach:
A recursive function for counting leaves would check if a node is a leaf and then recursively count leaves in the left and right subtrees.
Implementation:
-- Define the Tree type
data Tree a = Leaf | Node a (Tree a) (Tree a)
-- Function to count the number of leaves
leafCount :: Tree a -> Int
leafCount Leaf = 1
leafCount (Node _ left right) = leafCount left + leafCount right
Explanation:
- Leaf: If the node is a Leaf, return 1 because it's a leaf.
- Node: If the node is a Node, recursively count the leaves in the left and right subtrees and sum them.
Example:
-- Function to count non-leaf nodes
nodeCount :: Tree a -> Int
nodeCount Leaf = 0
nodeCount (Node _ Leaf Leaf) = 0
nodeCount (Node _ left right) = 1 + nodeCount left + nodeCount right
Counting Non-Leaf Nodes
Definition: Non-leaf nodes are nodes with at least one child. To count these, traverse the tree and count nodes that are not leaves.
Implementation:
-- Function to calculate the height of the tree
height :: Tree a -> Int
height Leaf = 0
height (Node _ left right) = 1 + max (height left) (height right)
Explanation:
- Leaf: If the node is a Leaf, return 0.
- Node with Leaves: If the node has no children (a leaf), return 0.
- Node with Children: For other nodes, count the current node plus the number of non-leaf nodes in the left and right subtrees.
Example:
let tree = Node 1 (Node 2 Leaf Leaf) (Node 3 Leaf Leaf)
height tree -- Returns 1 (Height from root to leaves)
Calculating Height
Definition: The height of a binary tree is the length of the longest path from the root to a leaf. For a tree consisting only of leaves, the height is 0.
Implementation:
-- Helper function to compute the depth of a leaf
depth :: Tree a -> Int
depth Leaf = 0
depth (Node _ left right) = 1 + max (depth left) (depth right)
-- Function to check if a tree is perfect
perfect :: Tree a -> Bool
perfect tree = let d = depth tree
in all (== d) (leafDepths tree 0)
-- Helper function to get the depths of all leaves
leafDepths :: Tree a -> Int -> [Int]
leafDepths Leaf d = [d]
leafDepths (Node _ left right) d = leafDepths left (d + 1) ++ leafDepths right (d + 1)
Explanation:
- Leaf: A Leaf has a height of 0.
- Node: For a Node, the height is 1 plus the maximum height of the left and right subtrees.
Example:
let tree1 = Node 1 (Node 2 (Node 4 Leaf Leaf) (Node 5 Leaf Leaf)) (Node 3 (Node 6 Leaf Leaf) (Node 7 Leaf Leaf))
perfect tree1 -- Returns True (All leaves are at depth 2)
let tree2 = Node 1 (Node 2 Leaf Leaf) (Node 3 (Node 4 Leaf Leaf) Leaf)
perfect tree2 -- Returns False (Leaves are at different depths)
3. Special Properties of Binary Trees
Checking if a Tree is Perfect
Definition: A perfect tree is one where all leaves are at the same depth. This means that every level of the tree is fully filled.
Implementation:
To check if a tree is perfect:
- Compute the depth of the tree.
- Ensure all leaves are at this depth.
-- Helper function to compute the depth of a leaf
depth :: Tree a -> Int
depth Leaf = 0
depth (Node _ left right) = 1 + max (depth left) (depth right)
-- Function to check if a tree is perfect
perfect :: Tree a -> Bool
perfect tree = let d = depth tree
in all (== d) (leafDepths tree 0)
-- Helper function to get the depths of all leaves
leafDepths :: Tree a -> Int -> [Int]
leafDepths Leaf d = [d]
leafDepths (Node _ left right) d = leafDepths left (d + 1) ++ leafDepths right (d + 1)
Explanation:
- Depth Function: Computes the maximum depth of the tree.
- Perfect Function: Uses leafDepths to collect all leaf depths and checks if they are all equal to the computed depth.
Example:
let tree1 = Node 1 (Node 2 (Node 4 Leaf Leaf) (Node 5 Leaf Leaf)) (Node 3 (Node 6 Leaf Leaf) (Node 7 Leaf Leaf))
perfect tree1 -- Returns True (All leaves are at depth 2)
let tree2 = Node 1 (Node 2 Leaf Leaf) (Node 3 (Node 4 Leaf Leaf) Leaf)
perfect tree2 -- Returns False (Leaves are at different depths)
Checking if a Tree is Degenerate
Definition: A degenerate tree is one where each node has either zero or one child. This forms a structure similar to a linked list.
Implementation:
-- Function to check if a tree is degenerate
degenerate :: Tree a -> Bool
degenerate Leaf = True
degenerate (Node _ Leaf right) = degenerate right
degenerate (Node _ left Leaf) = degenerate left
degenerate (Node _ left right) = False
Explanation:
- Leaf: A leaf is trivially degenerate.
- Single Child: If a node has only one child (either left or right), continue checking the child.
- Two Children: If a node has two children, the tree is not degenerate.
Example:
let tree1 = Node 1 (Node 2 (Node 3 (Node 4 Leaf Leaf) Leaf) Leaf) Leaf
degenerate tree1 -- Returns True (Tree is a linked list)
let tree2 = Node 1 (Node 2 Leaf Leaf) (Node 3 Leaf Leaf)
degenerate tree2 -- Returns False (Tree has nodes with two children)
4. Bonus: Converting Degenerate Trees to Lists
Definition: For a degenerate tree, convert it to a list where each node’s value is added to the list in the order they appear from top to bottom.
Implementation:
-- Function to convert a degenerate tree to a list
list :: Tree a -> Maybe [a]
list tree
| degenerate tree = Just (treeToList tree)
| otherwise = Nothing
-- Helper function to convert a degenerate tree to a list
treeToList :: Tree a -> [a]
treeToList Leaf = []
treeToList (Node v left right) = v : treeToList left ++ treeToList right
Explanation:
- List Function: Checks if the tree is degenerate and, if so, converts it to a list.
- TreeToList Function: Traverses the tree in a pre-order manner (root, left, right) and constructs the list.
Example:
let tree1 = Node 1 (Node 2 (Node 3 (Node 4 Leaf Leaf) Leaf) Leaf) Leaf
list tree1 -- Returns Just [1, 2, 3, 4]
let tree2 = Node 1 (Node 2 Leaf Leaf) (Node 3 Leaf Leaf)
list tree2 -- Returns Nothing (Tree is not degenerate)
5. Practical Considerations
Edge Cases
- Empty Trees: Ensure functions handle empty trees gracefully. For instance, the height of an empty tree is 0, and it should be properly managed in functions like leafCount and nodeCount.
- Single Node Trees: Test functions with trees consisting of only one node to ensure they work correctly with minimal input.
Efficiency
Recursive approaches are elegant but can be inefficient for large trees due to potential stack overflow or excessive recursion. Consider iterative approaches or optimizations if performance becomes an issue. For example, use tail recursion where possible or iterative methods to avoid deep recursion.
Testing
Thorough testing is crucial. Consider various tree structures, including:
- Perfect Trees: Trees where all leaves are at the same depth.
- Degenerate Trees: Trees that resemble linked lists.
- Random Trees: Trees with a mix of nodes and leaves at various depths.
Conclusion
Recursive binary trees are a versatile and important data structure in computer science. Mastering operations such as counting leaves, nodes, and calculating height provides a foundation for understanding more complex tree algorithms. Special properties like perfect and degenerate trees offer additional insights into tree structures and their behavior. The bonus function for converting degenerate trees to lists illustrates how binary trees can be transformed into other data representations.
This computer science assignment covers a range of operations and properties related to recursive binary trees. By implementing these functions, students will gain a solid understanding of tree traversal, recursive algorithms, and tree properties. The use of foldT in this assignment highlights its power in abstracting tree operations and simplifying tree manipulations. These bonus problems further enhance your understanding by linking tree structures to other data representations to solve programming assignments.
By carefully implementing and testing each function, you’ll develop a comprehensive grasp of binary trees and their operations, which are foundational for many advanced topics in computer science.